In MATLAB, user input can be captured using the `input` function, which allows you to prompt the user for data during program execution. Here's a code snippet to illustrate this:
userAge = input('Enter your age: ');
Understanding User Input in MATLAB
What is User Input?
User input refers to the data that users provide to a program while it is running. In MATLAB, user input is critical for creating interactive applications. It allows users to influence the behavior and outcomes of scripts and functions by entering values dynamically. MATLAB provides various methods to collect and process user input, making it easier to tailor applications to specific needs.
Types of User Input
Numeric Input
Numeric input is one of the most straightforward forms of input in MATLAB. It enables users to enter numerical values, which can then be utilized for calculations or analysis. Here’s a simple example of how to collect a single numeric value from the user:
% Getting a single numeric value
userNum = input('Enter a number: ');
For multiple values, you can prompt the user to enter an array:
% Getting multiple numeric values
userNums = input('Enter multiple numbers (e.g., [1, 2, 3]): ');
In this case, the user enters an array directly, and you can perform operations like summation or averaging on that array later in your code.
String Input
String input is essential when you want to gather information that consists of text, such as names, addresses, or any other alphanumeric data. The syntax for gathering a string input in MATLAB includes an additional `'s'` argument in the input function:
% Getting a string input
userString = input('Enter your name: ', 's');
This command will prompt the user to enter their name. The value will then be stored in the variable `userString` for further processing.
Character Input
In MATLAB, character input relates to obtaining a single character, potentially for decision-making. Here’s how you can prompt for a character input:
% Getting a character input
userChar = input('Enter a single character: ', 's');
This input is similar to a string but is often used when only one character is necessary. You can use it for checks, such as menu selection or specific commands.
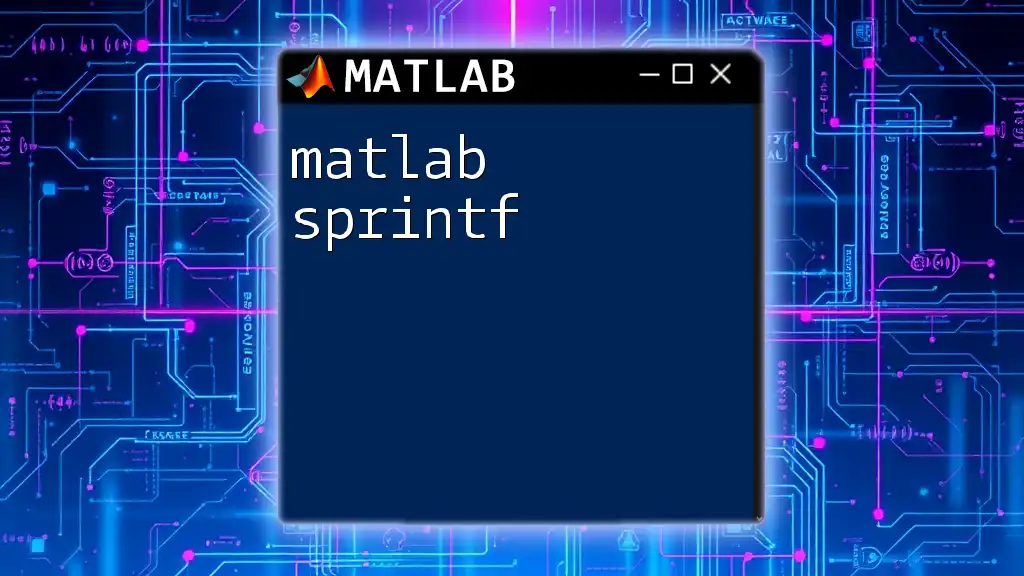
Techniques for Handling User Input
With the Input Function
The `input` function is versatile and can handle various data types based on the context of the input. To demonstrate its effectiveness, consider this combined approach for collecting both numeric and string inputs:
% Example of combined input
age = input('Enter your age: ');
name = input('What is your name? ', 's');
fprintf('Hello %s, you are %d years old.\n', name, age);
This snippet engages the user for their name and age, illustrating how easily these inputs can be processed and displayed.
Using Check Conditions
To enhance the robustness of your MATLAB applications, it's crucial to validate user inputs. Employing conditions can guide users to provide valid entries. Here’s a code example for validating numeric input:
% Validating numeric input
while true
userValue = input('Enter a positive number: ');
if userValue > 0
break;
else
disp('Input must be a positive number. Please try again.');
end
end
This loop enables users to retry until they provide acceptable input, increasing the overall integrity of the program.
Using Input Dialogs
For a more user-friendly approach, MATLAB provides graphical input dialogs using the `inputdlg` function, allowing for interactive input. Here’s a simple example:
% Using input dialog for inputs
prompt = {'Enter your name:', 'Enter your age:'};
dlgtitle = 'User Information';
dims = [1 35];
definput = {'John Doe', '30'};
answer = inputdlg(prompt, dlgtitle, dims, definput);
name = answer{1};
age = str2double(answer{2});
fprintf('Hello %s, you are %d years old.\n', name, age);
This script opens a dialog box prompting users to enter their name and age, making it visually appealing and intuitive.
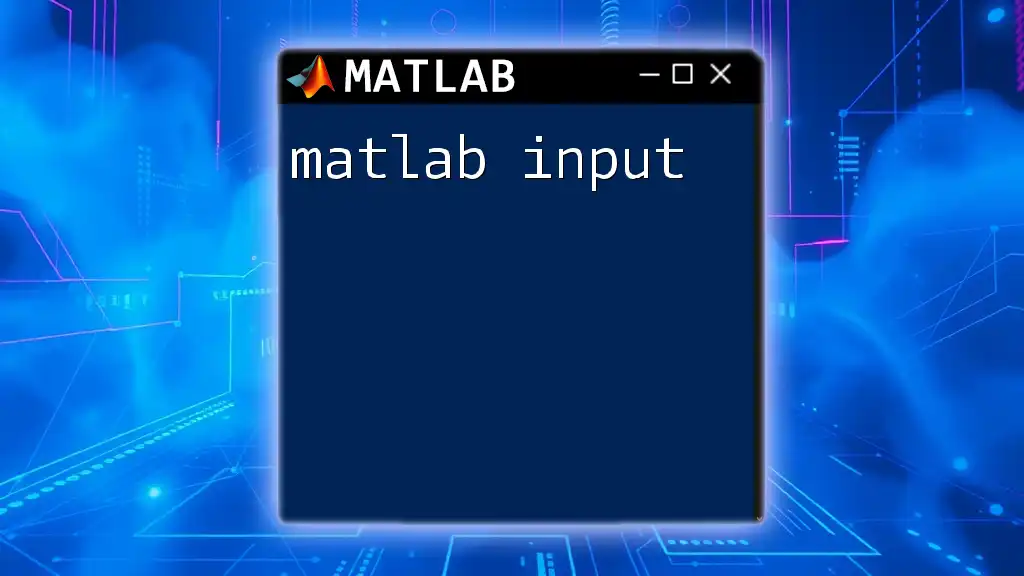
Customizing User Input Experience
User Instructions and Prompts
Crafting clear and concise prompts is vital for ensuring users understand what input is needed. Effective instructions enhance the user experience and reduce the likelihood of incorrect or irrelevant data entry. Remember to write prompts that are specific and easily understood.
Error Handling and Feedback
Incorporating error handling into your user input processes is essential. Providing informative error messages when users input invalid data can dramatically improve user satisfaction. For instance:
% Error handling for user input
userInput = input('Enter a number between 1 and 10: ');
if userInput < 1 || userInput > 10
disp('Error: Input must be between 1 and 10.');
else
disp('Thank you for your input.');
end
This feedback mechanism ensures users are aware of the input constraints, allowing them to correct their input.
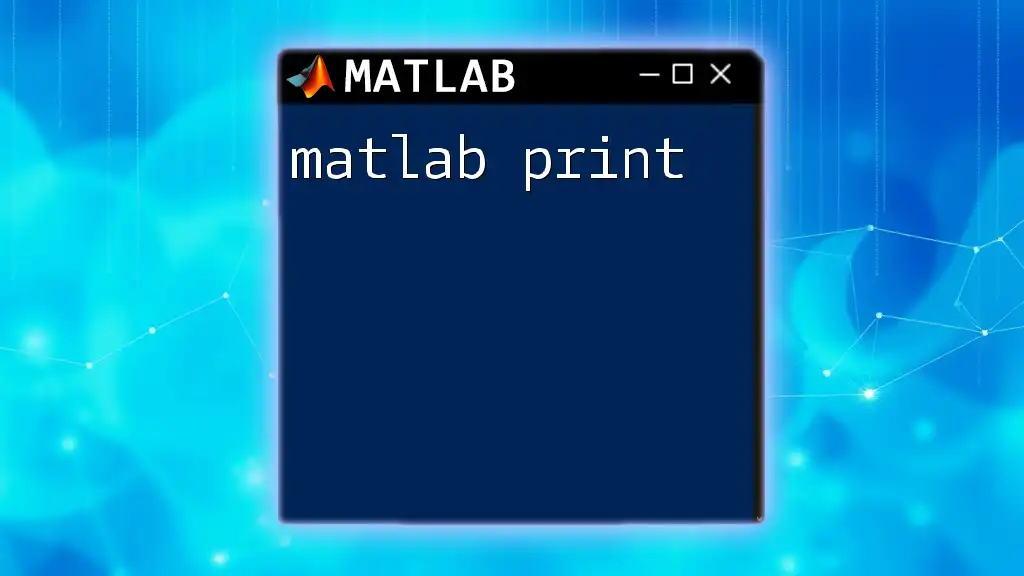
Practical Applications of User Input
Creating Simple Interactive Programs
MATLAB user input can be utilized to create interactive applications. A practical example is a basic calculator that performs arithmetic operations:
% Simple calculator example
operation = input('Enter operation (+, -, *, /): ', 's');
num1 = input('Enter first number: ');
num2 = input('Enter second number: ');
switch operation
case '+'
result = num1 + num2;
case '-'
result = num1 - num2;
case '*'
result = num1 * num2;
case '/'
result = num1 / num2;
otherwise
disp('Invalid operation. Please try again.');
end
fprintf('The result is: %f\n', result);
This interactive calculator takes two numbers and an operation type from the user, providing immediate results and demonstrating how user input can drive application logic.
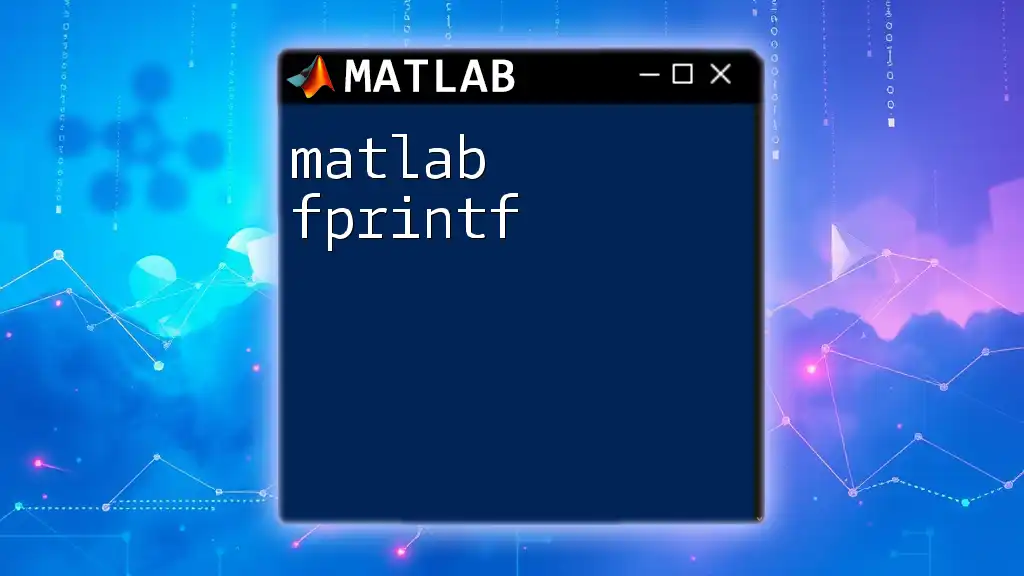
Conclusion
The ability to gather and handle MATLAB user input is crucial for building effective, interactive applications. This comprehensive guide covered various types of user input, techniques for effective handling, and practical applications. By implementing the strategies discussed in this guide, you can create user-friendly MATLAB applications that engage users and allow for dynamic input handling. Engaging with user input opens up new horizons in MATLAB programming, enhancing the overall functionality and interactivity of your projects.