In MATLAB, you can invert a matrix using the `inv()` function, which computes the inverse of a square matrix if it is non-singular.
A = [1 2; 3 4]; % Define a 2x2 matrix
A_inv = inv(A); % Calculate the inverse of the matrix
Understanding Matrix Inversion
What is Matrix Inversion?
Matrix inversion is a fundamental concept in linear algebra where we find a matrix A's inverse, denoted as A^-1. A matrix is invertible if it satisfies the condition:
A \ A^-1 = I*
where I represents the identity matrix. Only square matrices (same number of rows and columns) are subject to inversion, and a crucial requirement is that the matrix's determinant must not be zero. In other words, a matrix must be non-singular to have an inverse.
Applications of Inverted Matrices
Many real-world applications require matrix inversion, such as solving systems of linear equations, optimization problems, and understanding transformations in multiple dimensions. For instance, in engineering, matrix inverses are often used in control systems to analyze system stability. In data science, inverting covariance matrices is essential for algorithms like linear regression.
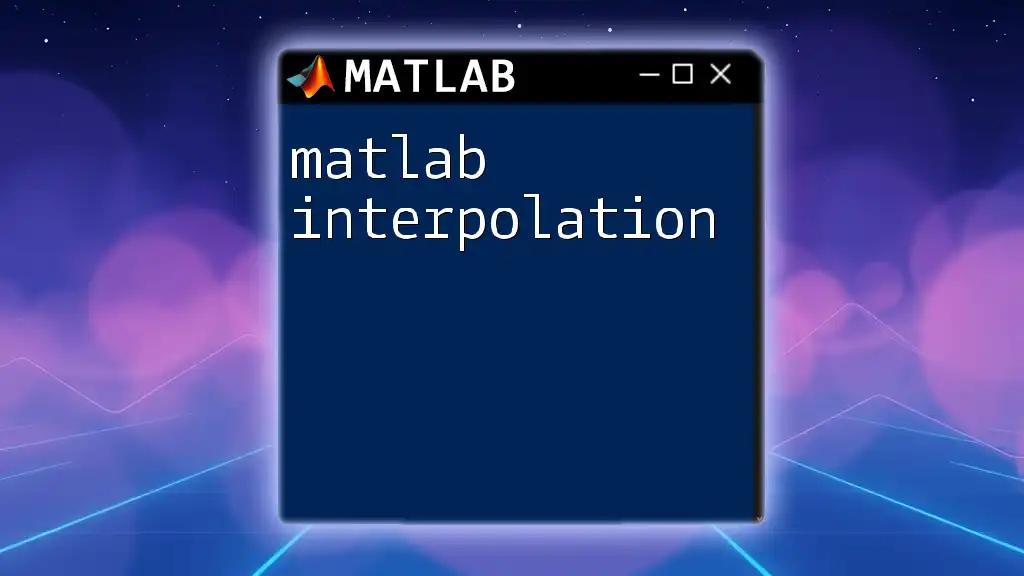
Theoretical Background
Determinant and Invertibility
The determinant of a square matrix is a scalar value that provides crucial information about the matrix's properties. If the determinant is zero, the matrix is singular, indicating that it does not have an inverse. You can compute the determinant in MATLAB using the `det()` function. For example:
A = [1, 2; 3, 4];
d = det(A);
disp(d);
Key insight: If `d` equals zero, the warning is clear—matrix A is not invertible.
Properties of Inverted Matrices
Understanding the properties of inverted matrices is essential for more advanced applications. Notably, the multiplication of a matrix by its inverse yields the identity matrix:
(A * A^-1 = I)
This property implies that each element from A and its inverse A^-1 interacts in a way that produces the neutral element of multiplication, the identity matrix. Additionally, the transpose of an inverse has the property that:
(A^-1)′ = (A′)−1
This means the inverse of the transpose of a matrix equals the transpose of the inverse, facilitating easier calculations in certain scenarios.
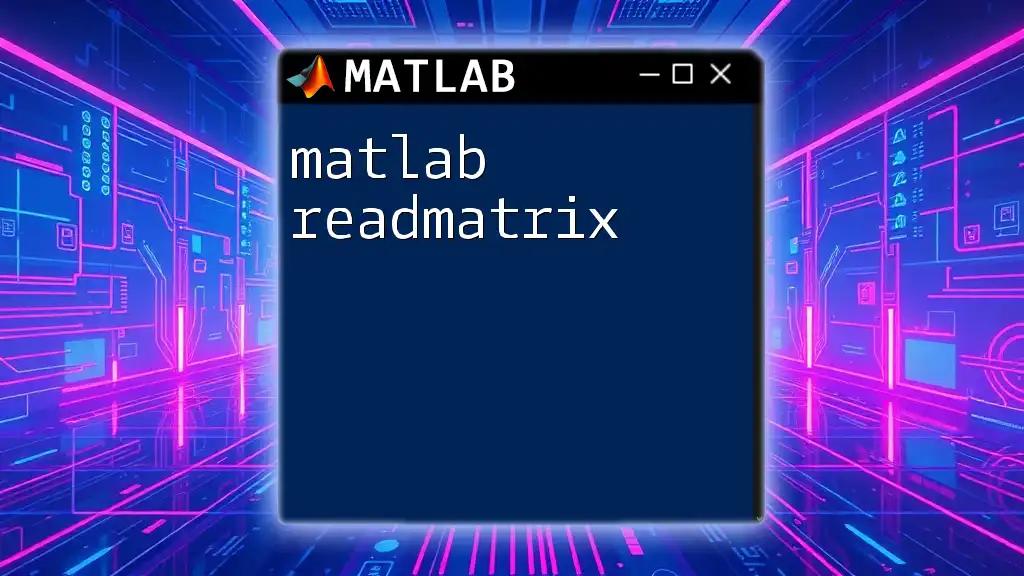
Inverting Matrices in MATLAB
Using the `inv()` Function
MATLAB provides the `inv()` function specifically for matrix inversion. The syntax is simple:
A_inv = inv(A);
Here is an example of inverting a 2x2 matrix:
A = [1, 2; 3, 4];
A_inv = inv(A);
disp(A_inv);
Explanation of Output: The resultant matrix `A_inv` is the inverse of matrix A, and it should satisfy the property mentioned above when multiplied back with A.
Alternative Methods to Calculate the Inverse
Matrix Division
Another way to compute an inverse is through matrix division. In MATLAB, we can use left or right division for this purpose. For instance, solving linear equations can be conveniently expressed as:
x = A \ B; % Equivalent to A_inv * B
This syntax often proves to be more numerically stable than directly computing the inverse with `inv()`.
Using the `rref` Function
The reduced row echelon form (RREF) can also be used to find the inverse of a matrix. To apply RREF, you augment the matrix with the identity matrix. Consider the following example:
augmented_matrix = [A, eye(size(A))]; % Augment matrix A with identity matrix
A_inv = rref(augmented_matrix);
Once you apply `rref`, the resulting augmented matrix will yield the inverse on the right side if it exists. This approach is particularly useful for educational purposes and clarity in demonstration.
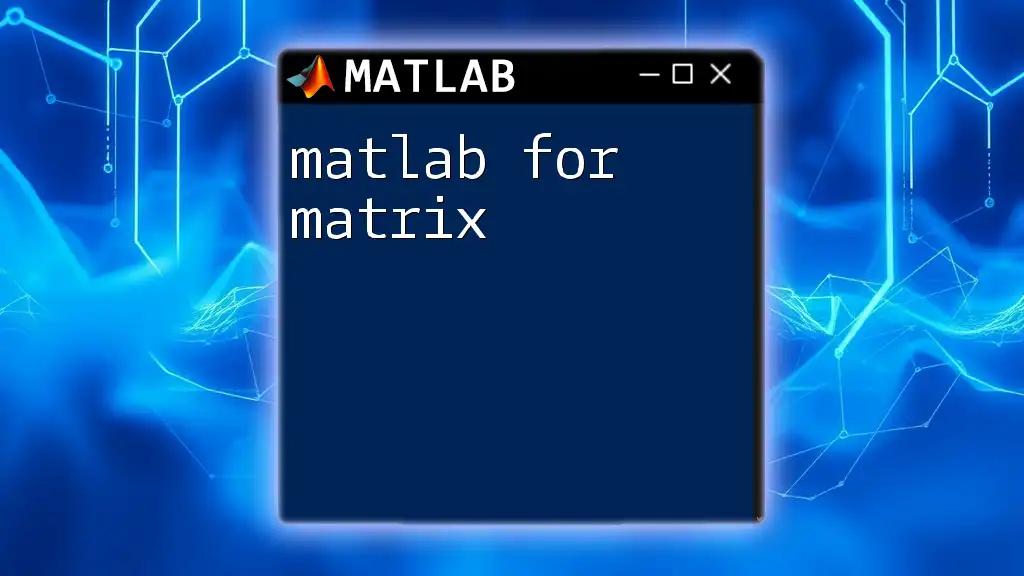
Practical Examples
Inverting a 2x2 Matrix
Let's consider a specific example of inverting a 2x2 matrix. Take the matrix:
A = [2, 3; 5, 7];
A_inv = inv(A);
disp(A_inv);
This will yield matrix A_inv, the inverse of A. Always check your results by multiplying back to confirm that the product equals the identity matrix.
Inverting a 3x3 Matrix
For a more complex scenario, we can invert a 3x3 matrix. The following code illustrates this:
A = [1, 2, 3; 0, 1, 4; 5, 6, 0];
A_inv = inv(A);
disp(A_inv);
As with the 2x2 case, validate the output to ensure A \ A_inv = I* holds true.
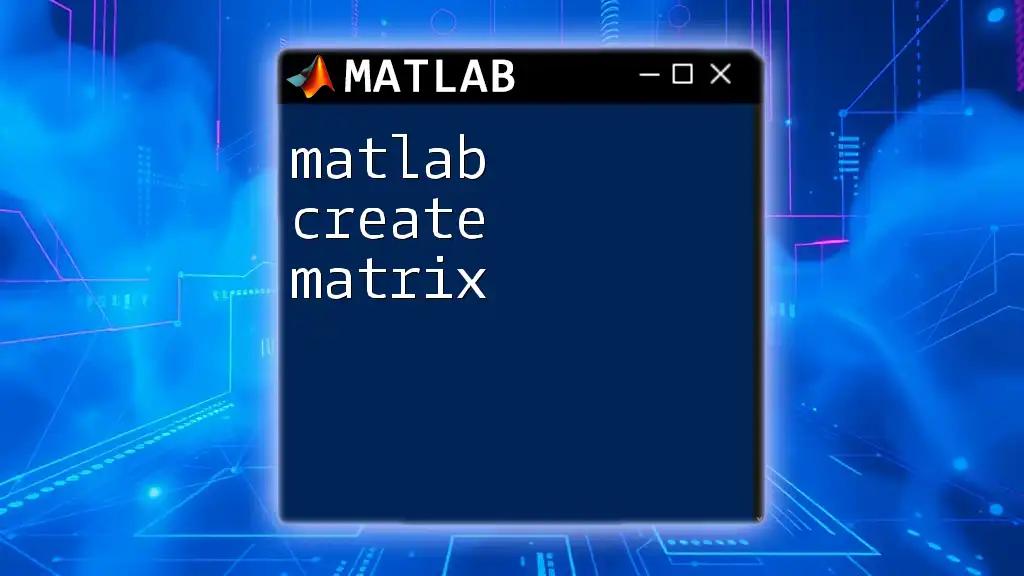
Common Errors and Troubleshooting
Singular Matrices
A common stumbling block when working with matrix inversion is encountering a singular matrix. Matrices that do not have an inverse fall into this category. You can easily check if a matrix is singular in MATLAB using the determinant:
if det(A) == 0
disp('Matrix is singular and cannot be inverted.');
end
This alert serves as a quick diagnostic tool.
Error Messages in MATLAB
While working in MATLAB, specific error messages may prompt issues during matrix inversion. For example, "Matrix is singular" appears if your matrix fails the invertibility condition. Always check the dimensions, as errors can also arise if matrices do not align for operations.
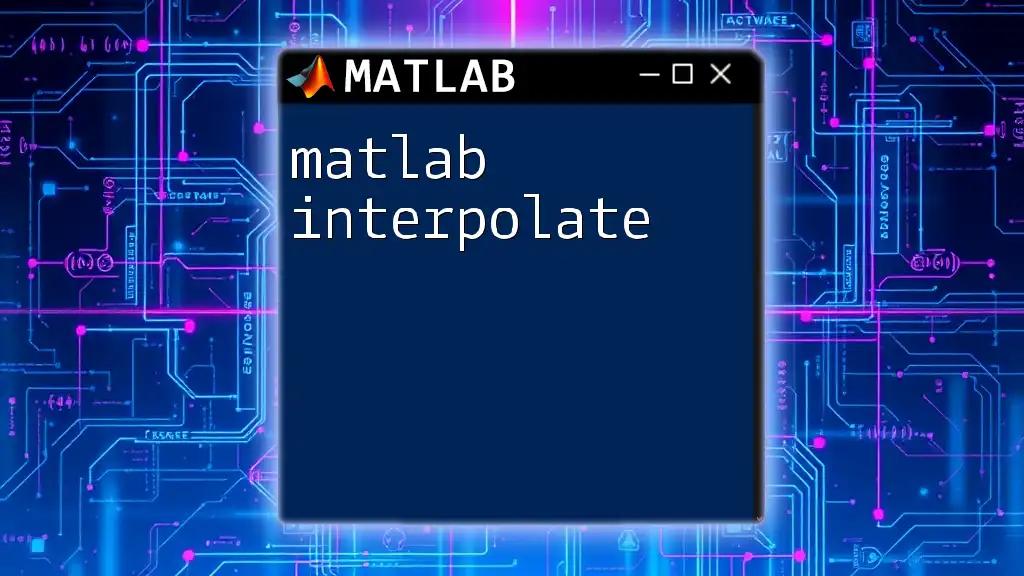
Performance Considerations
Computational Complexity
The time complexity of matrix inversion tends to increase significantly with larger matrices. In practice, Storing and computational overhead can lead to inefficiencies, particularly when matrices grow dense or high-dimensional.
Best Practices
Alternatives to Direct Inversion: Whenever possible, avoid using the inverse directly. Instead, rely on methods such as matrix factorization or decomposition for solving linear equations—these methods remain more efficient and robust, particularly for large datasets.
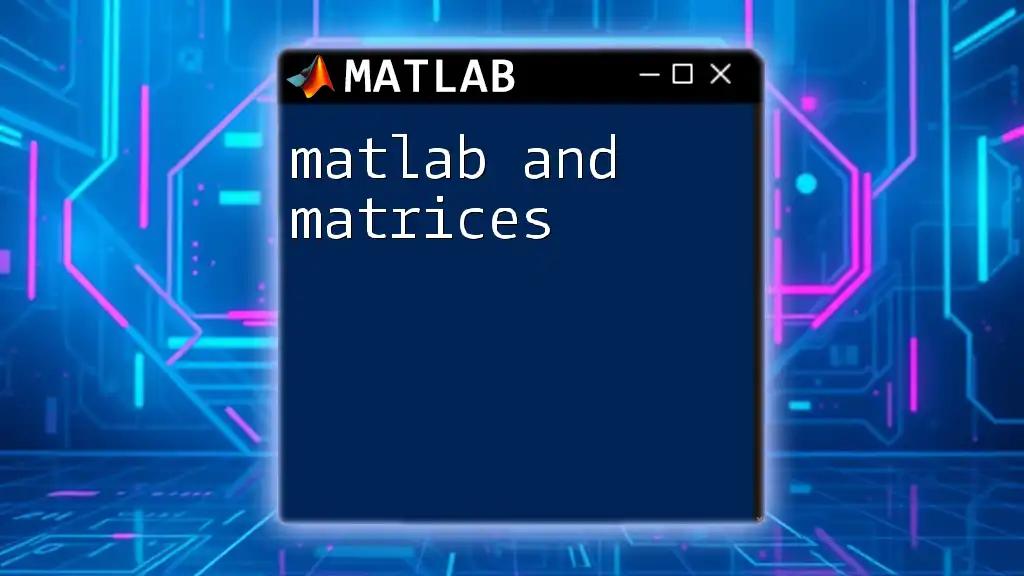
Conclusion
In this guide, we delved into the topic of how to matlab invert matrix efficiently. You learned the theoretical foundations, practical implementations, and common pitfalls associated with matrix inversion. Keep practicing with the provided examples to enhance your MATLAB skills. Consider exploring additional resources to deepen your understanding, and don't hesitate to reach out for more tutorials or insights into MATLAB usage.