The `connectlayers` function in MATLAB allows you to establish connections between layers in a 3D convolutional neural network, enabling seamless information flow between different stages of the model.
Here's a code snippet demonstrating how to connect layers in a 3D convolutional network:
lgraph = layerGraph(); % Create an empty layer graph
conv3dLayer = convolution3dLayer(3, 16, 'Name', 'conv3d_1'); % Define a 3D convolution layer
lgraph = addLayers(lgraph, conv3dLayer); % Add the 3D convolution layer to the graph
% Connect the input layer to the 3D convolution layer
lgraph = connectLayers(lgraph, 'input_1', 'conv3d_1');
Understanding Layers in MATLAB
What are Layers?
In the context of neural networks, layers are the building blocks that process data. Each layer consists of parameters that transform inputs into outputs, helping the model learn and adapt. Understanding different types of layers is crucial when building a neural network in MATLAB.
Commonly Used Layers
Some of the most common layers you will encounter in MATLAB include:
- Fully Connected Layer: A layer where each neuron is connected to every neuron in the previous layer, primarily used for classification tasks.
- Convolutional Layer: A layer that applies a convolution operation over the input data to extract features.
- Pooling Layer: A layer that reduces the dimensionality of the input by summarizing the features, making the computation more efficient.
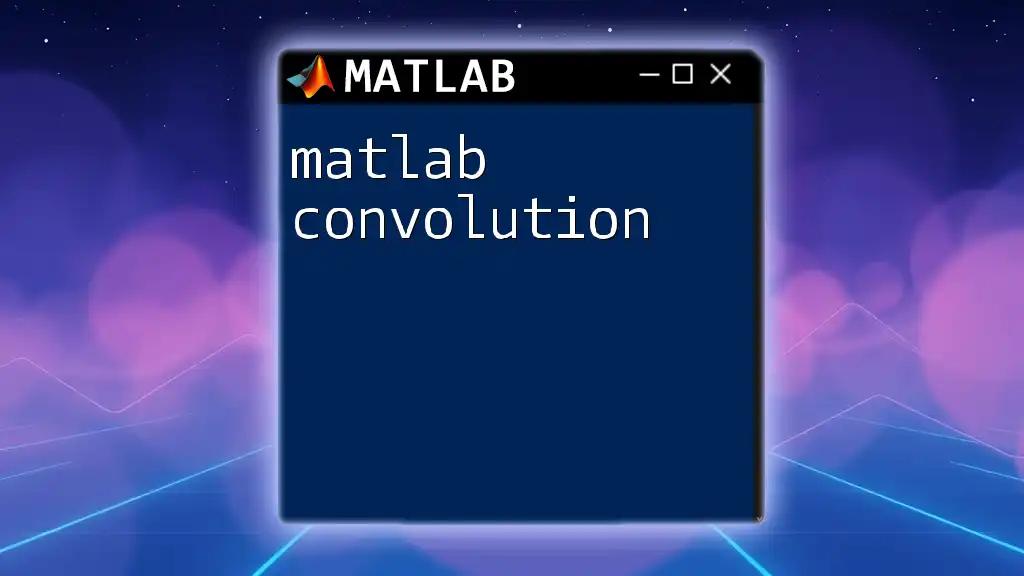
Getting Started with connectLayers
What is connectLayers?
The `connectLayers` function in MATLAB is essential for linking different layers within a neural network. This function allows you to specify how information should flow from a source layer to a destination layer, enabling you to create complex architectures.
Syntax of connectLayers
The general syntax for the `connectLayers` function is as follows:
layer = connectLayers(layers, sourceLayerName, destinationLayerName)
- layers: A group of layers that forms the network structure.
- sourceLayerName: The name of the layer that is being connected from.
- destinationLayerName: The name of the layer that is receiving the connection.
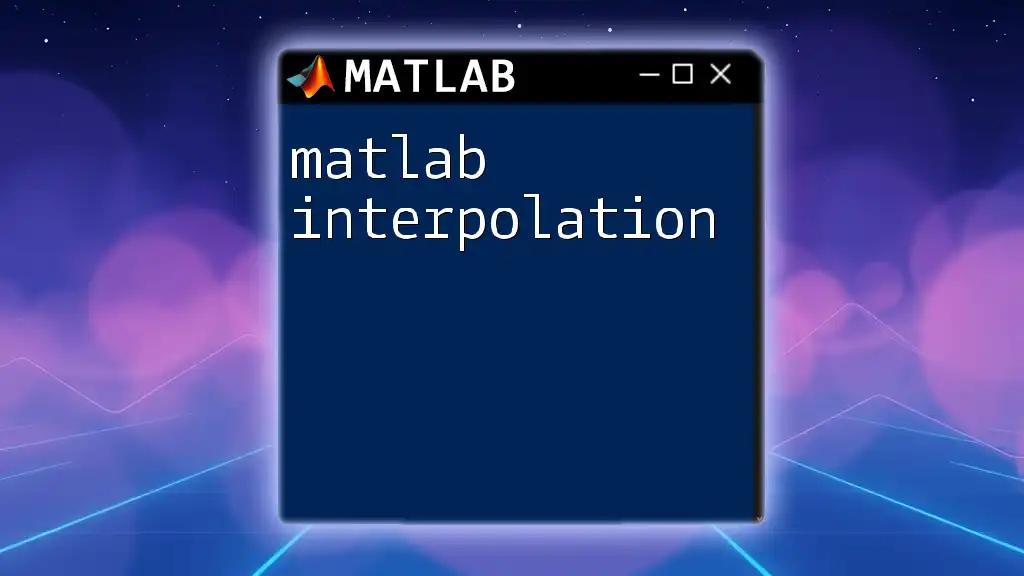
Convolutional Layers in MATLAB
What is a Convolution Layer?
Convolution layers play a critical role in processing 3D data and are pivotal for feature extraction in deep learning. By applying a convolution operation, these layers detect patterns and spatial hierarchies in multidimensional data.
Introduction to convolution3dLayer
The `convolution3dLayer` is specifically designed for three-dimensional data inputs, such as videos or volumetric images. It allows you to define various parameters that tailor the layer to your specific data needs.
The syntax for defining a `convolution3dLayer` is:
layer = convolution3dLayer(filterSize, numFilters, Name, Value)
- filterSize: Defines the size of the convolution filter, which determines how much of the input data is processed at a time.
- numFilters: Specifies the number of filters to be applied, essential for determining the depth of the output feature map.
- Name, Value: Additional customizable options to adjust layer behavior.
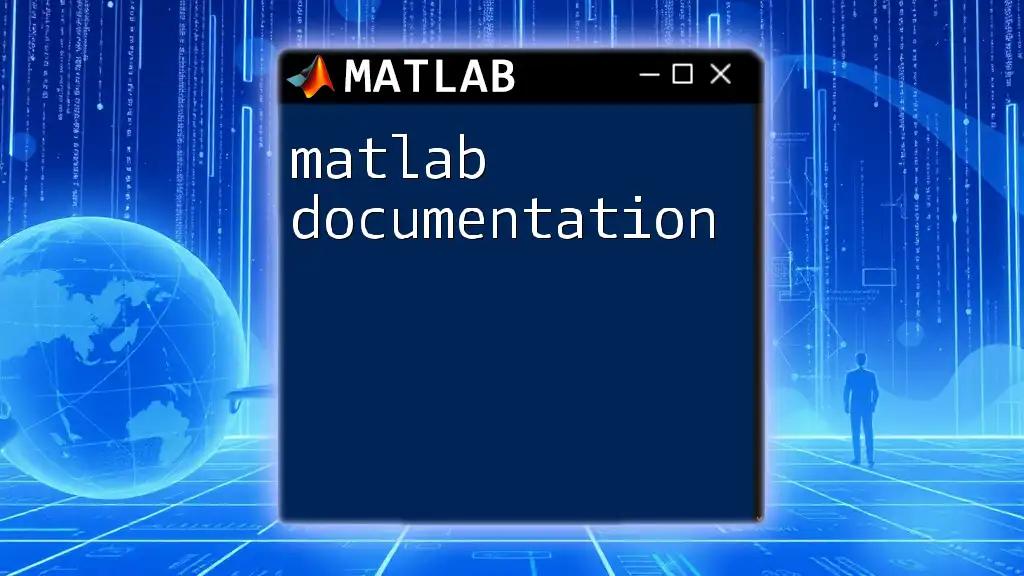
Creating a Simple 3D Convolutional Network
Step 1: Define Convolution Layers
To start, you'll want to define your convolution layers. For example, you can create a convolution layer with the following code:
convLayer1 = convolution3dLayer(3, 8, 'Padding', 'same', 'Name', 'conv1');
In this code:
- 3 is the size of the convolution filter.
- 8 is the number of filters, indicating the layer will produce an output with depth equal to 8.
- The padding option is set to 'same', ensuring that the output size remains the same as the input.
Step 2: Add Additional Layers
With your convolution layer defined, you can now add other layers to your network. Here’s an example of how to do this:
layers = [
imageInputLayer([32 32 32 1], 'Name', 'input')
convLayer1
reluLayer('Name', 'relu1')
fullyConnectedLayer(10, 'Name', 'fc')
softmaxLayer('Name', 'softmax')
classificationLayer('Name', 'output')
];
In this example:
- imageInputLayer defines the input layer for a 3D volumetric input of size 32x32x32 with a single channel.
- A ReLU layer is added for activation, followed by a fully connected layer for classification purposes.
- The softmax layer and classification layer are included for final output interpretation.
Step 3: Connecting Layers
Now that you've set up your layers, you can use `connectLayers` to establish connections. This is how you do it:
lgraph = layerGraph(layers);
lgraph = connectLayers(lgraph, 'conv1', 'relu1');
This snippet creates a layer graph from your previously defined layers and connects `conv1` to `relu1`, specifying how the output of the convolution layer feeds into the ReLU layer.
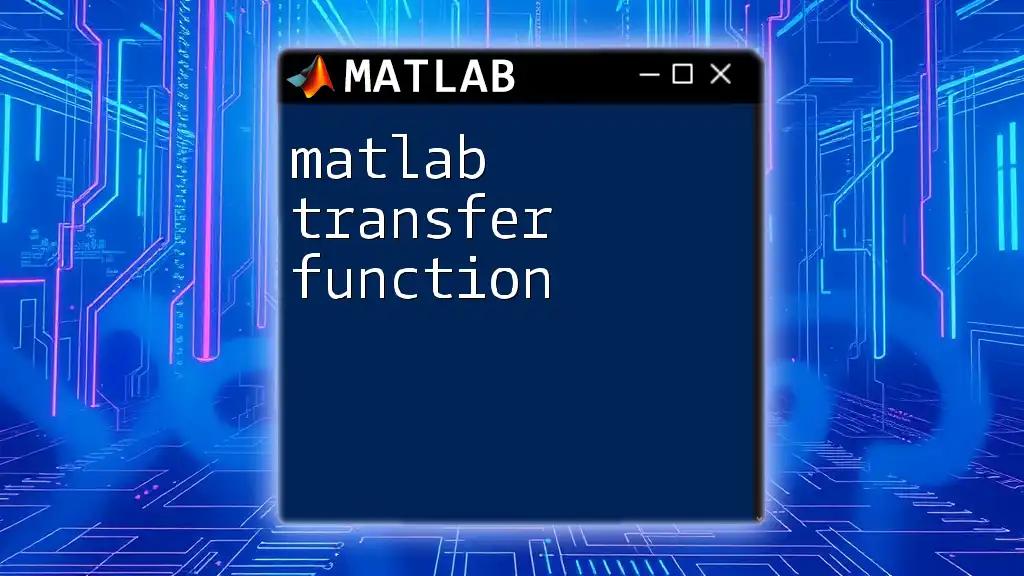
Training the 3D Convolutional Network
Configuring the Training Options
Before you begin training, you need to configure your training parameters. Here’s an example setup:
options = trainingOptions('adam', ...
'MaxEpochs', 4, ...
'InitialLearnRate', 1e-4, ...
'Shuffle', 'every-epoch', ...
'Verbose', false, ...
'Plots', 'training-progress');
In this code:
- MaxEpochs determines how many times the training algorithm will iterate over the entire dataset.
- InitialLearnRate sets the learning rate for the optimization algorithm.
- Shuffle indicates that the data should be shuffled every epoch to enhance the training process.
Running the Training Process
Once you've defined your network and training options, you can initiate the training with the following command:
net = trainNetwork(trainingData, layers, options);
Here, `trainingData` is the dataset you will use to train the model, layers is your defined layer structure, and options are the training configurations you just set up.

Conclusion
In this guide, we've explored the essential components of using MATLAB's connectLayers and convolution3dLayer. Understanding these concepts is crucial for building advanced neural networks tailored to your specific data requirements. As you experiment with different configurations and architectures, you'll gain deeper insights into how these layers work together to process 3D data effectively.
Dive into MATLAB's extensive documentation, tutorials, and community forums to further enhance your knowledge and skills in deep learning and neural network design.