The MATLAB `function` command allows you to define a custom function that can take inputs and return outputs, promoting code reusability and organization.
Here’s a quick example of a simple function that adds two numbers:
function result = addNumbers(a, b)
result = a + b;
end
What is a Function in MATLAB?
A function in MATLAB is essentially a block of code designed to perform a specific task. Functions allow you to encapsulate code, making it reusable and easier to manage. The basic syntax for defining a function is as follows:
function [outputs] = functionName(inputs)
Outputs are the results returned by the function, while Inputs are the parameters you pass to it. Utilizing functions not only promotes code reuse but also improves readability and simplifies debugging. Additionally, functions help manage namespaces, preventing variable name collisions.
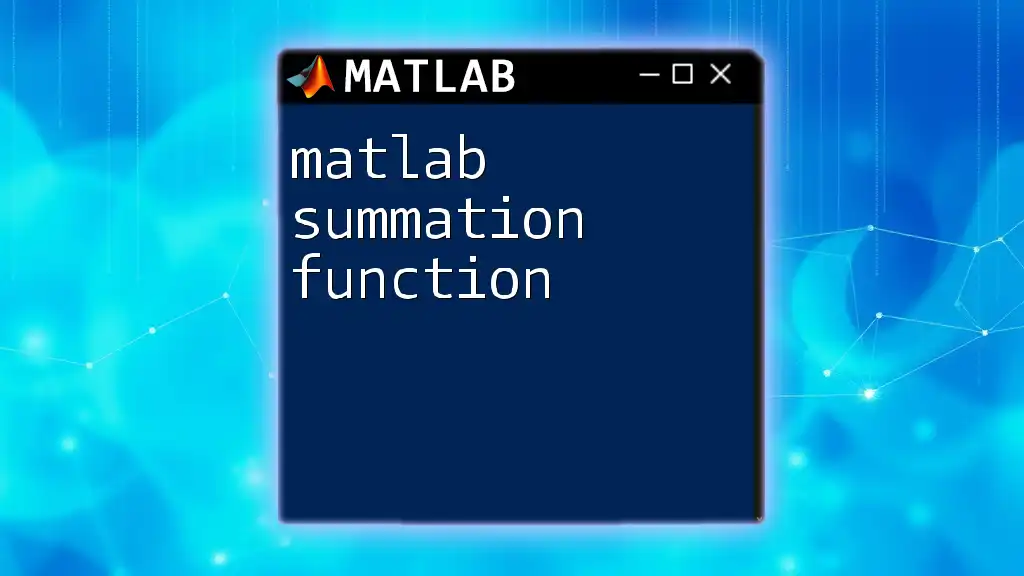
Creating a Basic Function
Syntax of a MATLAB Function
To create a function, you start with the `function` keyword followed by the output variables in square brackets, the function name, and the input variables in parentheses.
Example of a Simple Function
For instance, let’s create a function that adds two numbers:
function sum = addNumbers(a, b)
sum = a + b;
end
In this code snippet:
- The function name is `addNumbers`, and it takes two input arguments `a` and `b`.
- The output variable `sum` is computed as the sum of `a` and `b`.
To call this function, simply use:
result = addNumbers(5, 10);
The variable `result` will now hold the value `15`.
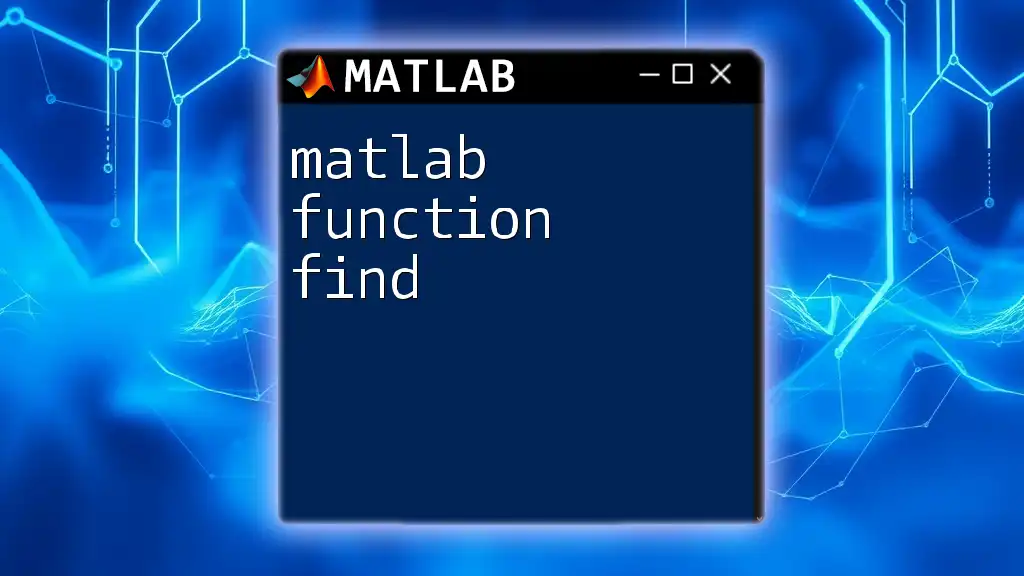
Function Outputs and Inputs
Input Arguments
Input arguments can be positional or optional. Positional arguments must be provided in the order defined, while optional arguments can have default values. Consider the following example that multiplies two numbers but gives a default value if only one argument is provided:
function result = multiplyNumbers(a, b)
if nargin < 2 % Check if two arguments are provided
b = 1; % Assign default value to b
end
result = a * b;
end
In this function, if you call `multiplyNumbers(5)`, `b` defaults to `1`, and the result will be `5`.
Output Arguments
A MATLAB function can return multiple outputs. This is done by defining multiple variables in square brackets. For instance, the following function computes both the sum and product of two numbers:
function [sum, product] = computeValues(a, b)
sum = a + b;
product = a * b;
end
When you call this function:
[totalSum, totalProduct] = computeValues(4, 5);
`totalSum` will store `9` and `totalProduct` will store `20`.
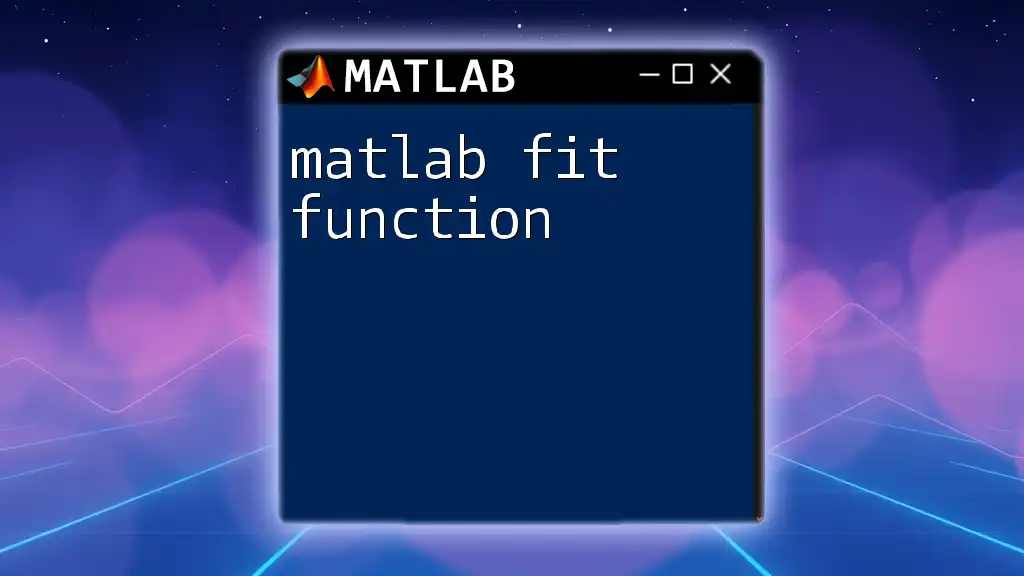
Function Files vs. Scripts
Understanding Function Files
A MATLAB function file is an M-file that contains function definitions, distinct from scripts which execute a sequence of MATLAB commands. While scripts operate on variables in the active workspace, functions have their own local workspace.
Example Usage of a Function File
To use a function, save it as a `.m` file, for example, `addNumbers.m`. You can then call this function from either the command window or another script as shown previously.
Using functions helps you create modular code, making it easier to organize and maintain.
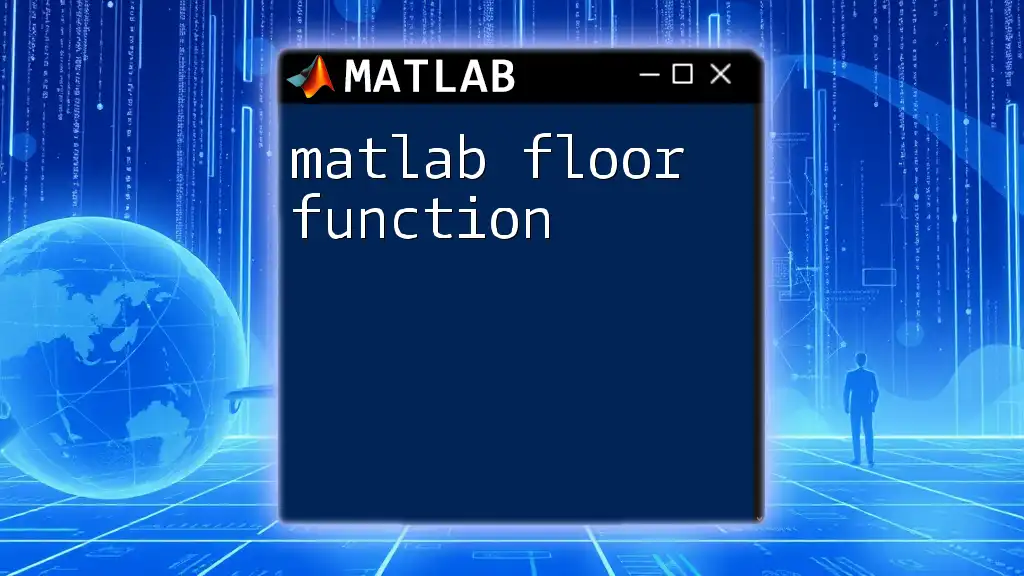
Scope of Variables in Functions
Local vs Global Variables
In MATLAB, variables defined inside a function are local, which means they are only accessible within that function. In contrast, global variables can be accessed from multiple functions or the command line.
Code Example Demonstrating Variable Scope
Here is an example:
function demoScope()
a = 10; % Local variable
global b;
b = 20; % Global variable
end
When you call `demoScope()`, `a` cannot be accessed outside the function, but `b` can be accessed globally after initializing it within `demoScope()`.
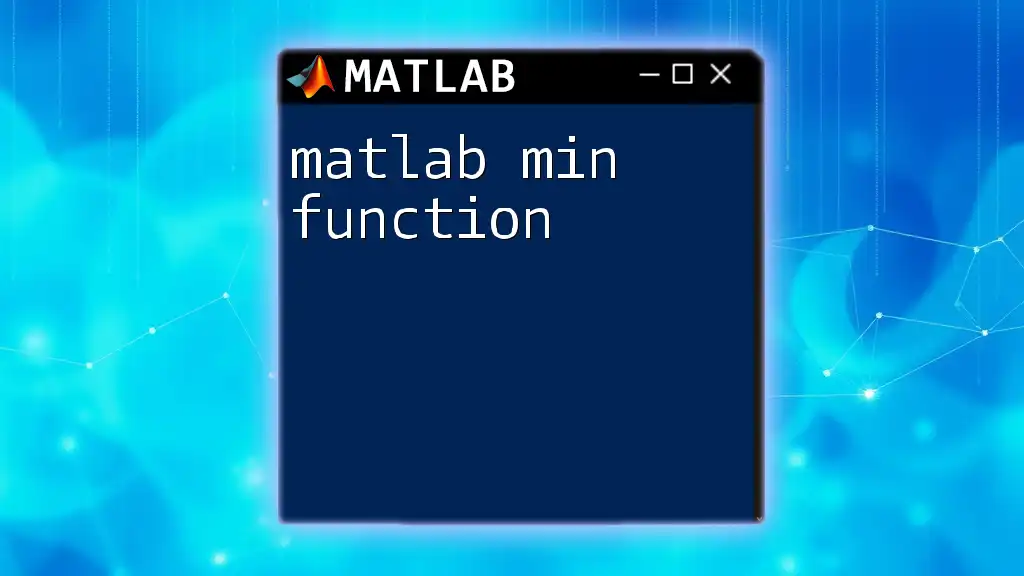
Function Nesting
What is Function Nesting?
Nested functions are functions defined within another function, allowing the inner function to access the variables of the outer function. This encapsulation can be beneficial in maintaining state without cluttering the global namespace.
Example of Nested Functions
Consider the following example:
function outerFunction()
function innerFunction()
disp('This is a nested function');
end
innerFunction();
end
When `outerFunction()` is called, it will display `"This is a nested function"`. Here, `innerFunction` is only accessible within `outerFunction`.
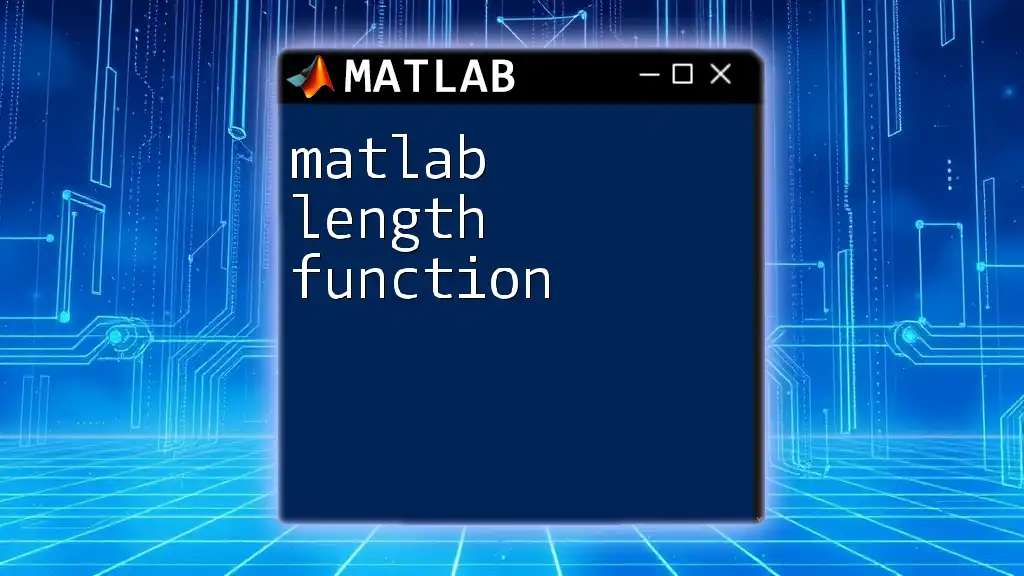
Anonymous Functions
Definition of Anonymous Functions
Anonymous functions are a concise way to create functions without having to define them in a separate file. The syntax is compact, and they are particularly useful for simple computations.
Code Example of an Anonymous Function
You can create an anonymous function that squares a number as follows:
squared = @(x) x^2; % Creating an anonymous function
result = squared(5);
In this case, calling `squared(5)` will return `25`. Anonymous functions are especially useful when you need quick calculations, such as passing a simple function to other functions like `arrayfun`.
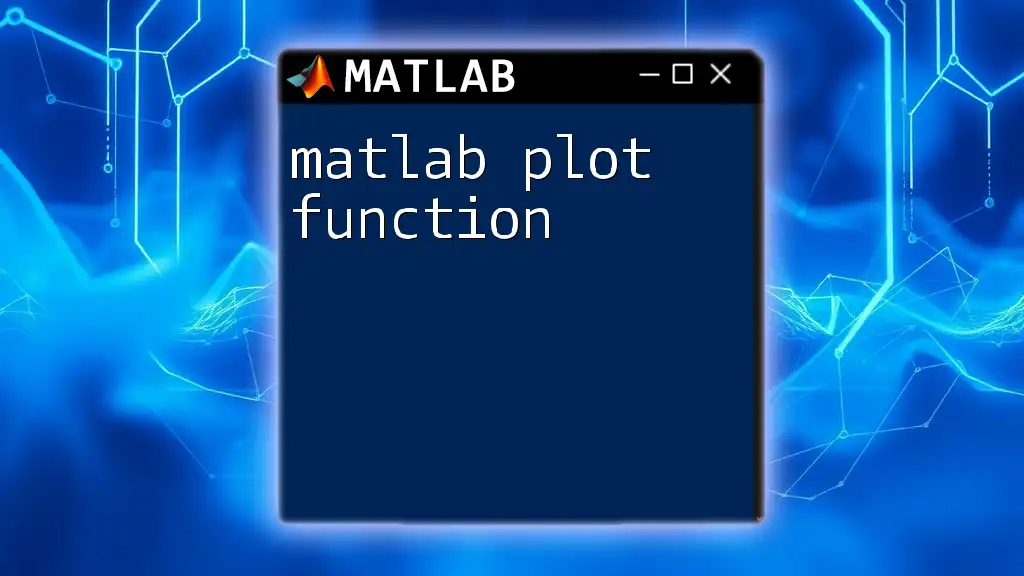
Conclusion
In summary, understanding the MATLAB function function is crucial for anyone looking to enhance their programming skills within MATLAB. Functions improve code reusability, readability, and management, making your scripts cleaner and more efficient.
Practice creating and using functions to get the most out of MATLAB. We encourage you to experiment with various examples and come up with your own functions. With consistent practice, you'll quickly grasp the full potential of functions in MATLAB.
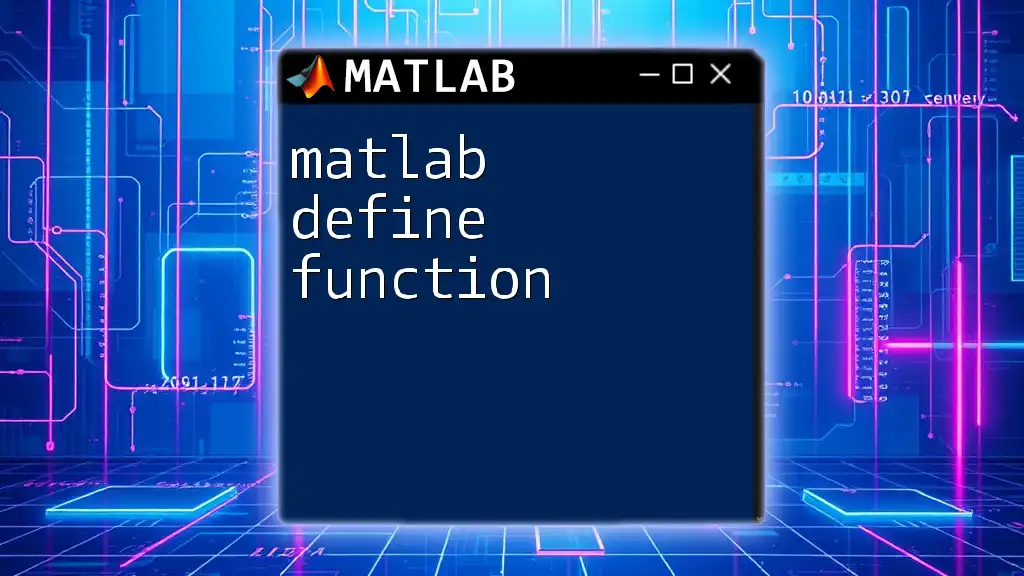
Additional Resources
For further reading, refer to the official MATLAB documentation on functions, and consider exploring recommended books and online courses that delve deeper into the topic. Engaging with the MATLAB community through forums and group discussions can also provide valuable insights and support as you continue your MATLAB journey.