The `freqz` function in MATLAB calculates and plots the frequency response of a digital filter defined by its numerator and denominator coefficients.
Here’s a simple code snippet demonstrating the use of `freqz`:
% Define the filter coefficients
b = [1 0.5]; % Numerator coefficients
a = [1 -0.8]; % Denominator coefficients
% Compute and plot the frequency response
freqz(b, a);
Understanding the Basics of freqz
What is freqz? The `freqz` function in MATLAB is a powerful tool for analyzing the frequency response of digital filters. Its utility in signal processing makes it indispensable for engineers and researchers who need to understand how filters behave in the frequency domain.
When to Use freqz? You typically use `freqz` when working with FIR (Finite Impulse Response) and IIR (Infinite Impulse Response) filters. It is particularly useful for determining how different frequencies are affected—whether they are passed, attenuated, or completely blocked by the filter.
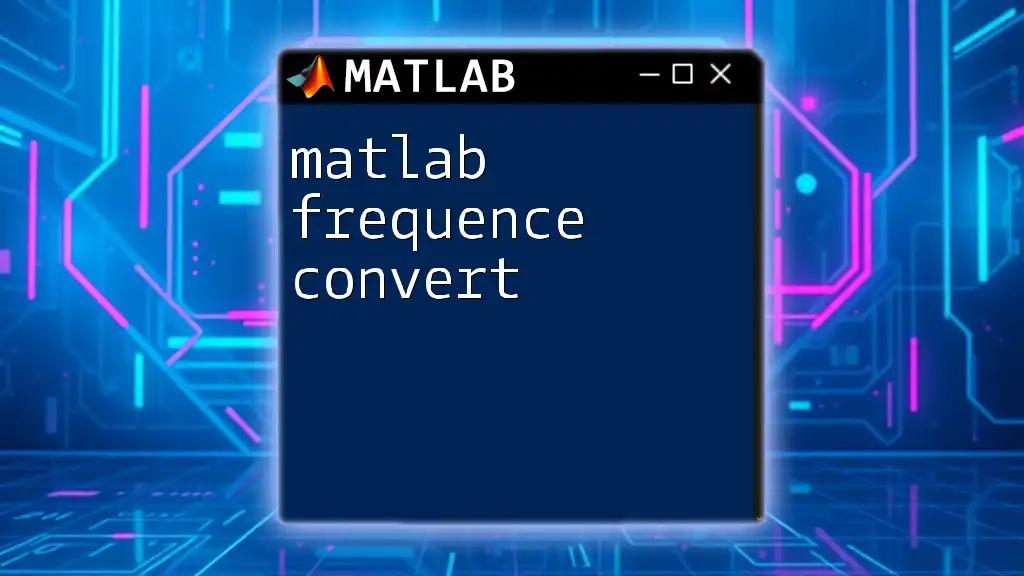
Parameters of freqz
`freqz` takes in several parameters, which primarily include:
- b: The filter coefficients.
- a: The denominator coefficients, which are often set to 1 for FIR filters.
- N: The number of frequency points to return, defaulting to 512 if unspecified.
An important point to understand is that `freqz` can generate a frequency vector that corresponds to the range of frequency responses calculated, which allows for a comprehensive analysis of filter behavior.
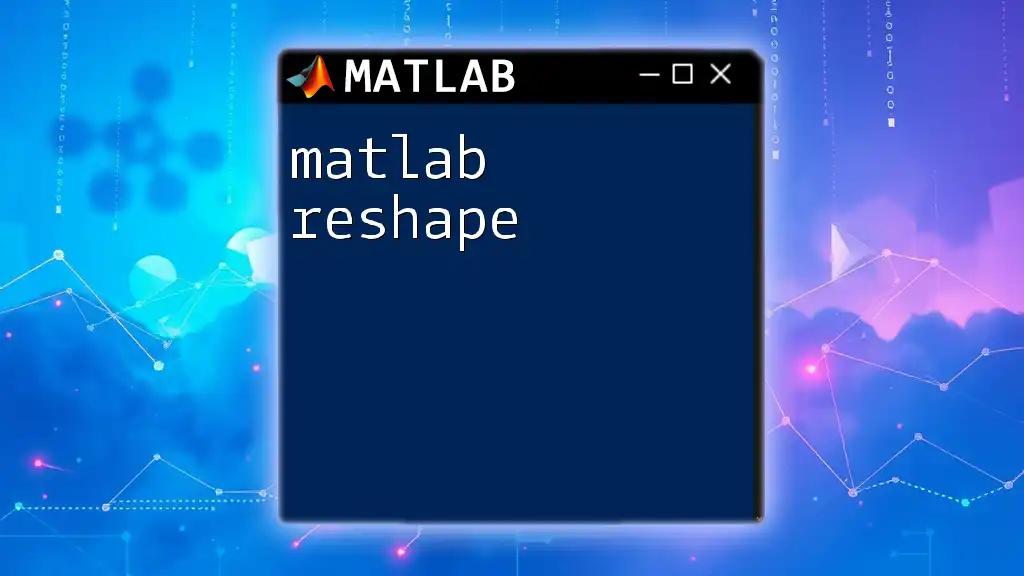
Output of freqz
The output of the `freqz` function is two key components:
- H: The complex frequency response, which contains both magnitude and phase information, allowing for detailed insight into filter performance.
- F: The frequencies at which the responses are computed, normalized between 0 and π radians/sample.
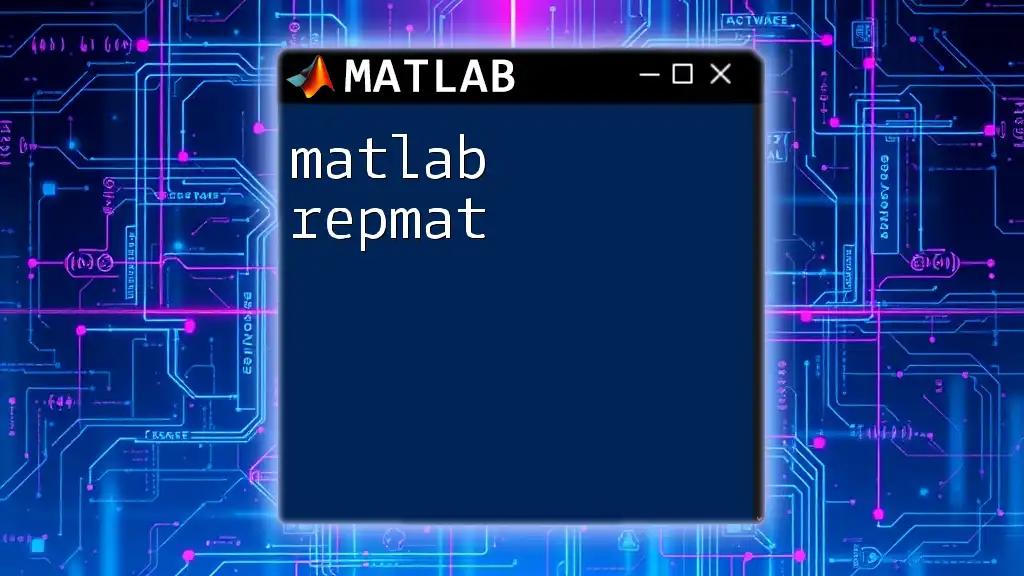
Setting Up MATLAB for freqz
To get started, you need to ensure you have MATLAB properly set up:
-
Installing MATLAB: Make sure to install the necessary toolboxes, particularly the Signal Processing Toolbox, for accessing `freqz` and other related functions.
-
Basic Commands to Get Started: Open the command window and create sample signals to test the function. It’s also essential to familiarize yourself with MATLAB’s editor to write and save scripts for your analyses.
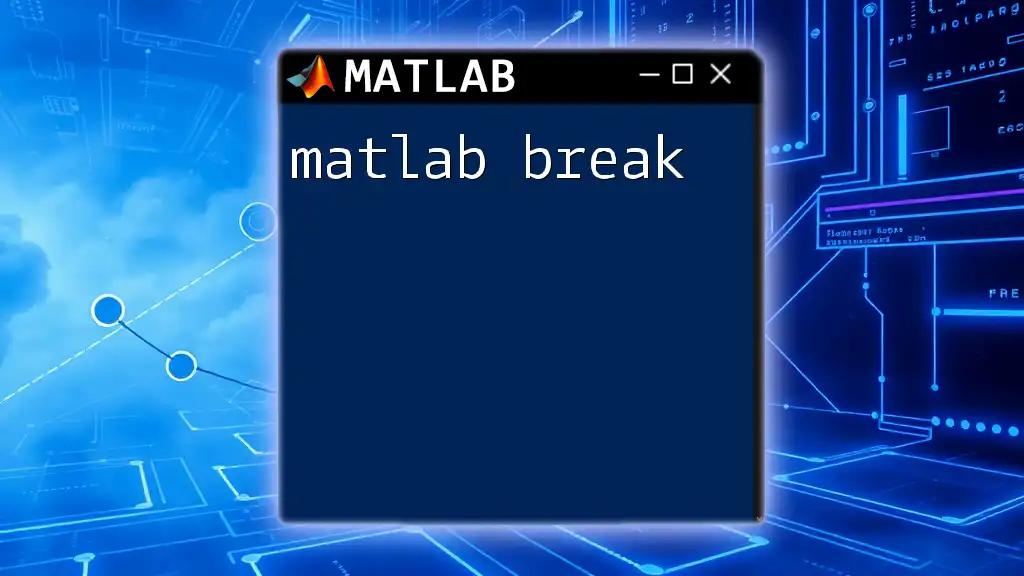
Using freqz in Basic Applications
Step 1: Designing a Filter
Before you can analyze a filter's frequency response, you'll need to design one. MATLAB provides several functions to create various filter types but let's focus on a simple FIR filter:
- Filter Types in MATLAB: FIR filters are generally easier to design and implement compared to IIR filters, making them a common choice for beginners.
To design a simple FIR filter, you can use the following code snippet:
N = 20; % Filter order
Fc = 0.4; % Cutoff frequency
b = fir1(N, Fc); % Create filter coefficients
Visualizing Filter Coefficients
Once you have your filter coefficients, you can visualize the frequency response using `freqz`:
freqz(b, 1); % Visualize the magnitude and phase response
Executing this command will display a plot that illustrates how the filter behaves across various frequencies.
Step 2: Analyzing Frequency Response
To analyze the frequency response of your designed filter in more depth, you can extract both the magnitude and phase information by calling `freqz` functionually:
[H, F] = freqz(b, 1, 512); % Compute frequency response
plot(F/pi, abs(H)); % Plot magnitude response
title('Magnitude Response of FIR Filter');
xlabel('Normalized Frequency (\times\pi rad/sample)');
ylabel('Magnitude');
This code provides a clean representation of the magnitude response, making it easy to observe how your FIR filter will affect incoming signals.
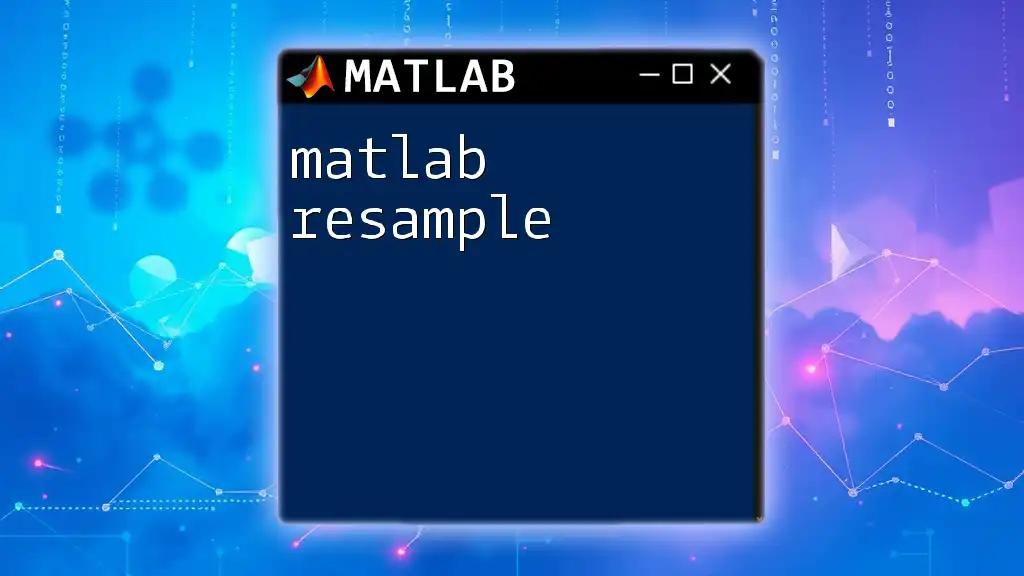
Advanced Usage of freqz
Customizing the Frequency Response Plot
Customizations enhance readability and presentation:
figure;
freqz(b, 1); % Generate default plot
set(gca, 'Color', 'lightgray'); % Custom background color
grid on; % Adding grid for better readability
These modifications can significantly improve your visual outputs, thereby making analyses easier to communicate to others.
Multiple Filters Comparison
Sometimes you may want to compare different filters side by side. This can be achieved with just a few lines of code:
b1 = fir1(20, 0.4); % First filter coefficients
b2 = fir1(20, 0.6); % Second filter coefficients
[H1, F] = freqz(b1, 1);
[H2, F] = freqz(b2, 1);
plot(F/pi, abs(H1), 'r', 'LineWidth', 1.5); % Plot first filter
hold on;
plot(F/pi, abs(H2), 'b', 'LineWidth', 1.5); % Plot second filter
legend('Filter 1 - 0.4', 'Filter 2 - 0.6');
title('Comparison of Multiple FIR Filters');
hold off;
This code showcases how two different filters respond across the same range of frequencies, offering valuable insight into their relative performance.
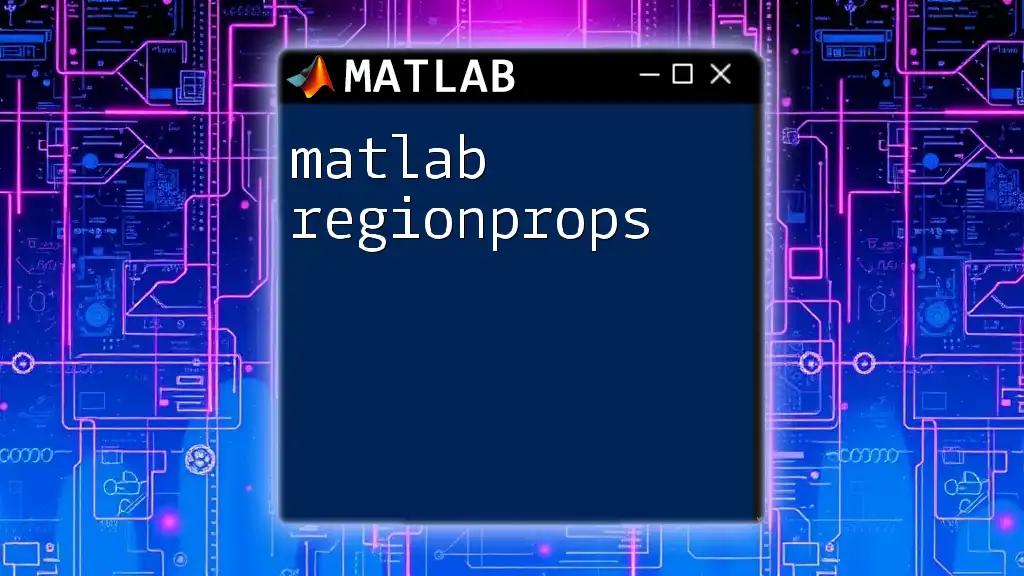
Practical Applications of freqz
Real-World Example: Audio Signal Processing
For practical applications, suppose you are working with audio signals. Filtering out noise from an audio stream is a common use case. You can filter an audio signal using a designed FIR filter and analyze the output using `freqz` to evaluate its effectiveness.
Sample Code to Filter Audio Signals
% Assume 'audio_signal' is your input audio and 'b' is your filter coefficients
filtered_signal = filter(b, 1, audio_signal);
sound(filtered_signal, fs); % Where fs is the sampling frequency
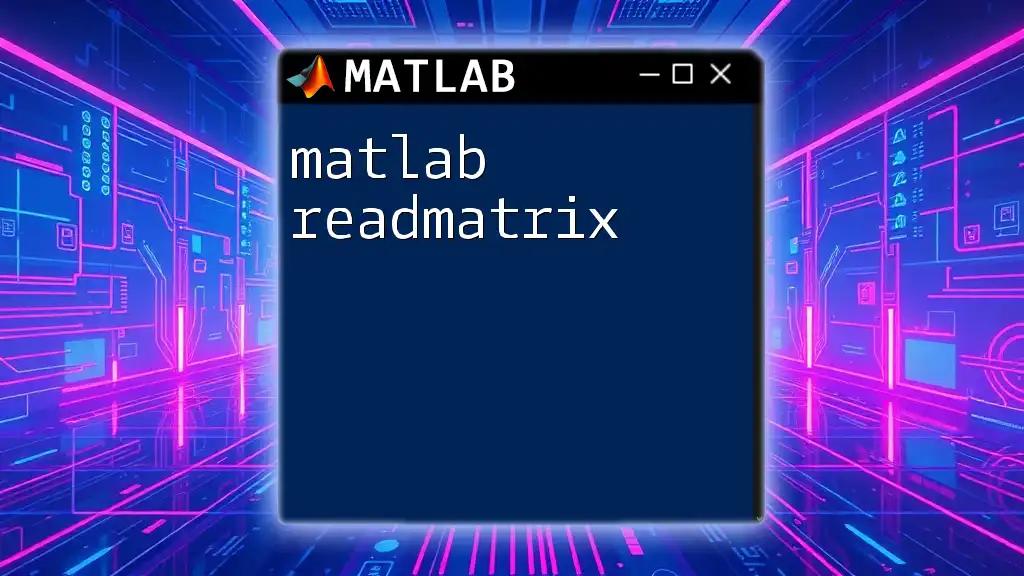
Conclusion
In summary, understanding and using `matlab freqz` can dramatically enhance your ability to analyze and design filters effectively. This function not only provides a deep insight into the frequency response characteristics but also allows for easy comparison between different filter designs.
Encouragement to Experiment: I encourage you to experiment with different filter designs, tweaking coefficients, and observing the resulting frequency responses using `freqz`. The hands-on approach will deepen your understanding of filter operations profoundly.
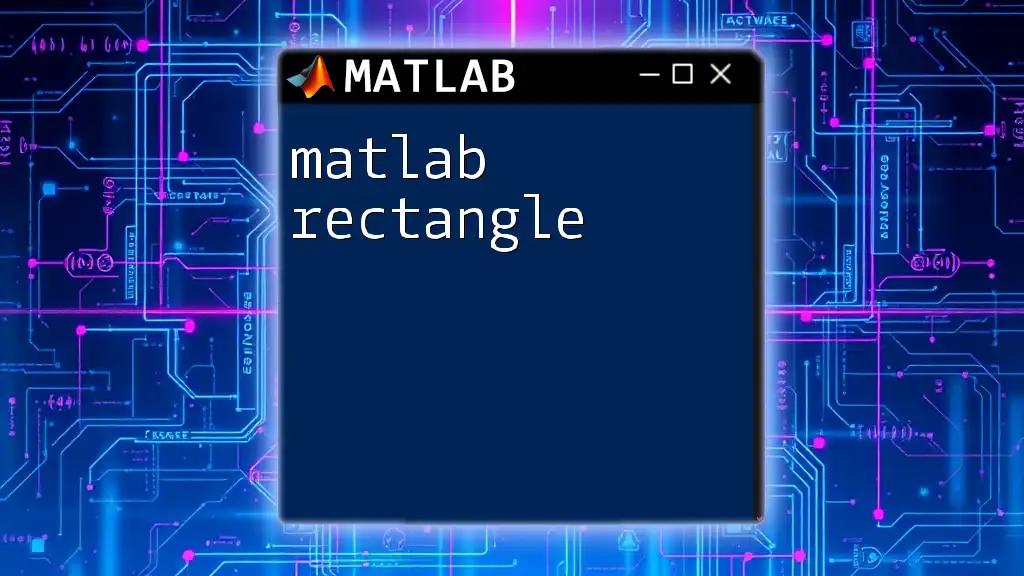
FAQs About freqz
The following are some common questions that may arise when working with `freqz`:
- How do I determine filter stability? Understanding the poles and zeros of the filter may require tools like `zpk` and `pole` functions alongside `freqz`.
- What if my response seems incorrect? Double-check your coefficients and ensure the correct structure for FIR or IIR filters based on your design requirements.

Additional Resources
For further exploration and learning, refer to the following:
- Official MATLAB Documentation: Dive deeper into the complete functionalities available with `freqz` and its parameters from the MATLAB website.
- Suggested Tutorials and Videos: These can provide visual learning experiences that complement the written guide and offer practical demonstrations of using `freqz` effectively.