The `break` command in MATLAB is used to exit a loop prematurely when a specific condition is met.
for i = 1:10
if i == 5
break; % Exit the loop when i equals 5
end
disp(i);
end
Understanding Control Flow in MATLAB
The Role of Control Flow Statements
Control flow statements are vital in programming as they dictate the order in which code executes. In MATLAB, control flow statements can be categorized into two primary types:
- Conditional Statements: These include `if`, `else`, and `switch`. They allow your program to execute specific code blocks based on a condition.
- Looping Statements: The most common are `for` and `while` loops, which enable repetitive tasks to be performed until a certain condition is met.
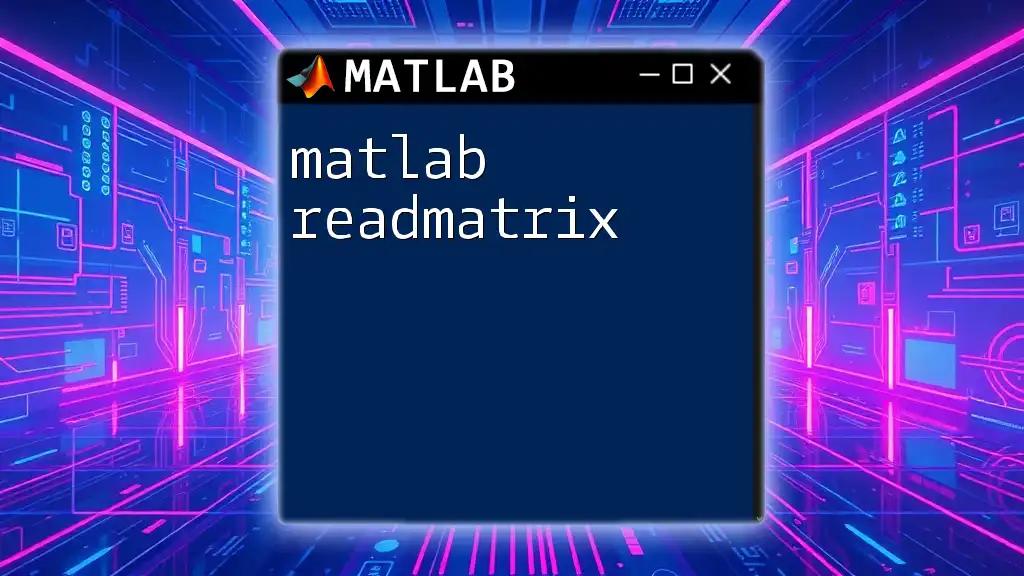
The `break` Command
Definition and Purpose
The `break` command in MATLAB is used to terminate the execution of the nearest enclosing loop, whether it’s a `for` or `while` loop. This command is essential when you want to exit a loop prematurely based on a specific condition.
When to Use the `break` Command
There are several situations where using the `break` command becomes beneficial:
- Exiting Loops Based on Conditions: If a specific condition is met while the loop is running, and you want to stop further iterations, `break` allows for this.
- Improving Program Efficiency: By skipping unnecessary iterations, `break` helps optimize your program's performance. For instance, if you're searching for a value in an array, you can exit the loop once the value is found.
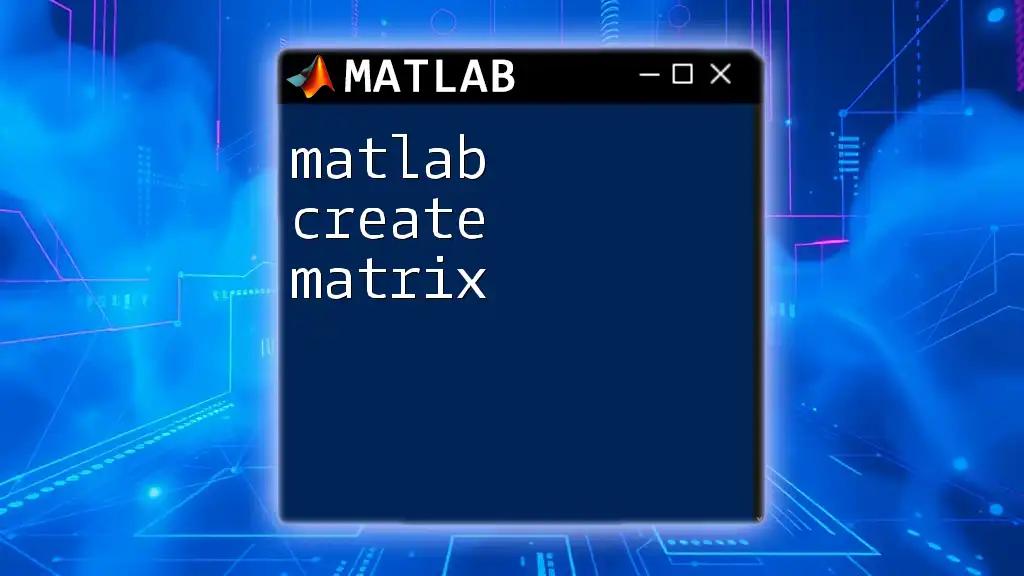
Syntax of the `break` Command
Basic Syntax
To illustrate the basic usage of the `break` command, consider the following code snippet:
% Basic Loop Example
for i = 1:10
if i == 5
break;
end
disp(i);
end
In this example, the loop will display the numbers 1 through 4. Once `i` reaches 5, the `break` statement is triggered, and the loop exits, skipping any further iterations.
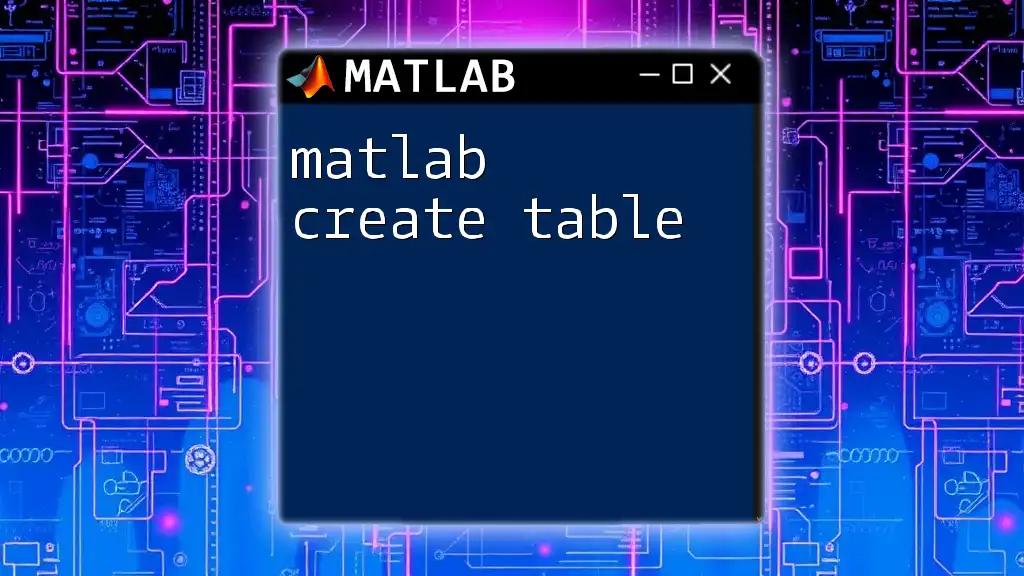
Practical Examples
Example 1: Simple Loop with `break`
Let's delve into a straightforward example:
% Simple Example Using Break
for i = 1:10
if i > 5
break; % Exit when i exceeds 5
end
fprintf('Current number: %d\n', i);
end
This code iterates from 1 to 10, but the output will only display numbers from 1 to 5. Once `i` exceeds 5, the `break` statement halts the loop.
Example 2: Nested Loops with `break`
Nested loops can also utilize the `break` command effectively. Here’s how:
% Nested Loops with Break
for i = 1:3
for j = 1:3
if j == 2
break; % Exits the inner loop
end
fprintf('i: %d, j: %d\n', i, j);
end
end
In this case, when `j` equals 2, the inner loop is terminated, but the outer loop continues. Thus, the output consists of pairs (i, j) where `j` prints 1 before exiting the inner loop.
Example 3: Using `break` in `while` Loops
The `break` command is equally useful in `while` loops:
% While Loop Example
count = 1;
while count <= 10
if count == 7
break; % Exit when count is 7
end
disp(count);
count = count + 1;
end
Here, the `while` loop continues until `count` reaches 7, at which point `break` is executed, and the loop ceases. The output will display numbers from 1 to 6.
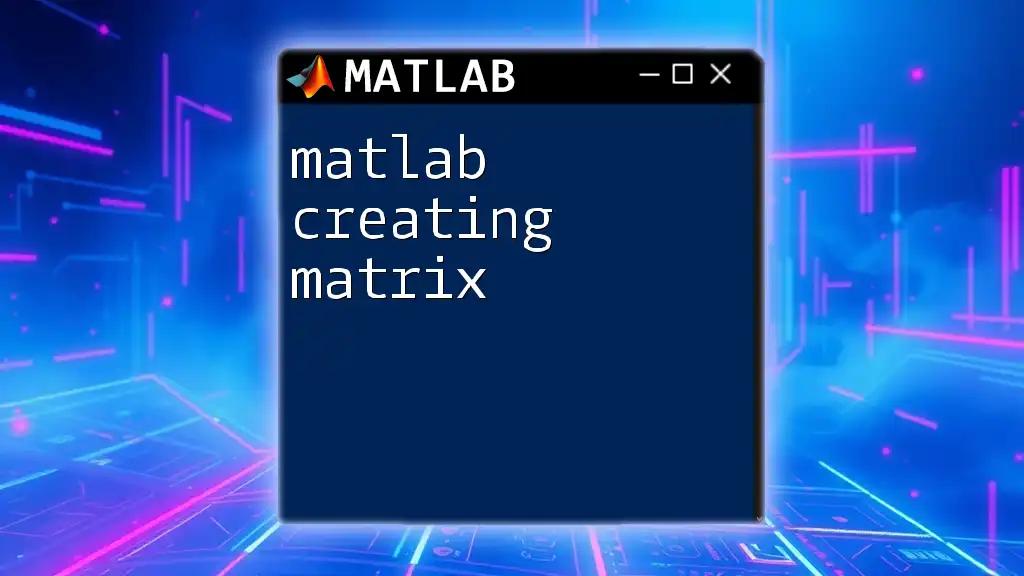
Common Mistakes and How to Avoid Them
Using `break` Incorrectly
Beginners often misuse the `break` command, leading to confusion in control flow. One common mistake is forgetting that `break` exits only the innermost loop. Consequently, it can lead to scenarios where the outer loop continues running unintentionally.
For instance, if you have nested loops both using `break`, you must ensure which loop you intend to exit. Failure to understand this can lead to unexpected behaviors in your program.
Best Practices for Using `break`
To use `break` efficiently:
- Be Clear with Conditions: Document your usage of `break` by providing comments in your code for clarity.
- Limit Its Use: While `break` is helpful, relying excessively on it can complicate logic. Ensure that your code structure can communicate intent without needing multiple `break` commands.
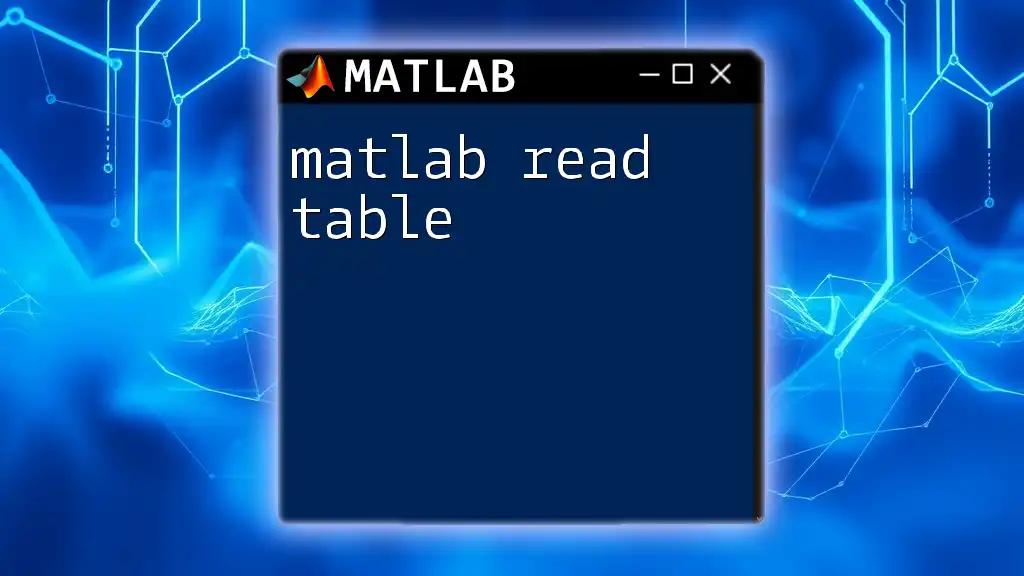
Alternatives to the `break` Command
Using Flags
An alternative to using `break` involves employing flags. Flags provide a means to manage loop execution without direct termination. Here is an example:
% Using Flags Instead of Break
shouldContinue = true;
i = 1;
while shouldContinue
if i > 5
shouldContinue = false; // Effectively exits the loop
end
disp(i);
i = i + 1;
end
In the above example, a flag variable (`shouldContinue`) controls whether the loop continues. When `i` exceeds 5, the flag is switched to `false`, allowing the loop to exit seamlessly.
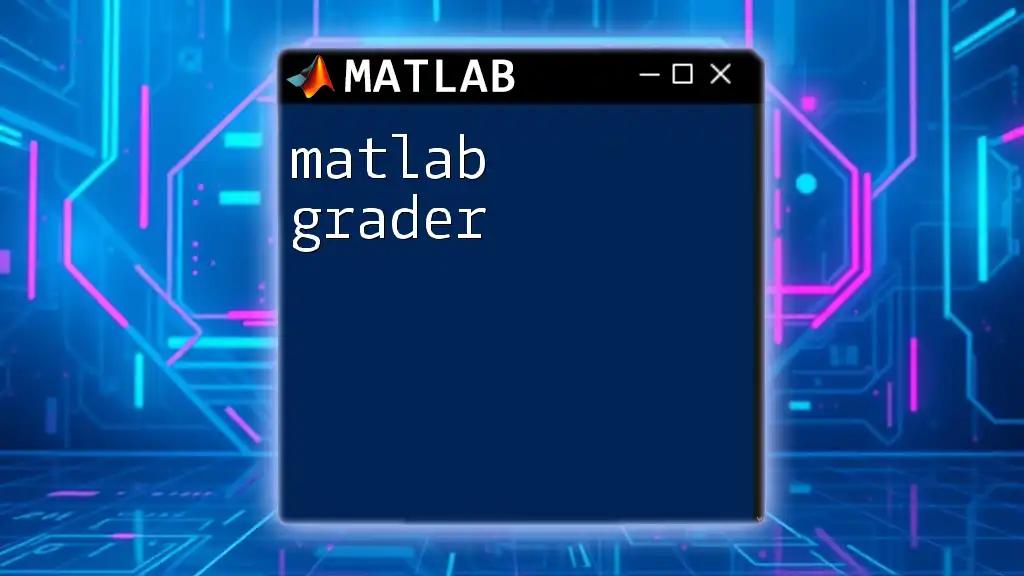
Conclusion
The `break` command is a powerful tool in MATLAB for controlling loop execution. Its proper implementation can significantly enhance program efficiency and make code cleaner and more manageable. As you continue to work with MATLAB, practicing the usage of the `break` command in various scenarios will deepen your understanding of control flow.
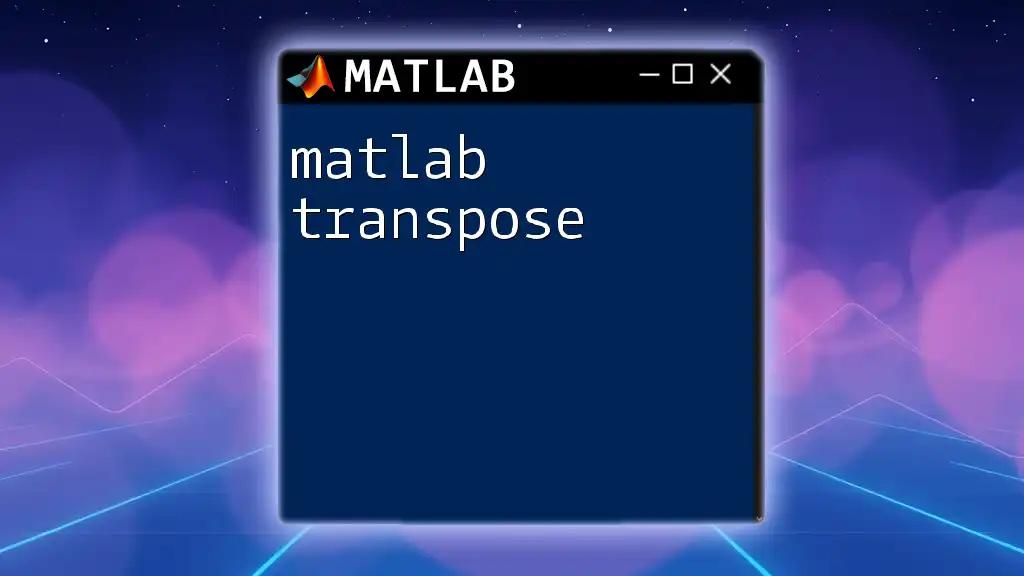
Additional Resources
For further readings and tutorials on the `break` command and control flow in MATLAB, refer to the official MATLAB documentation and consider enrolling in online courses to enhance your skills further.
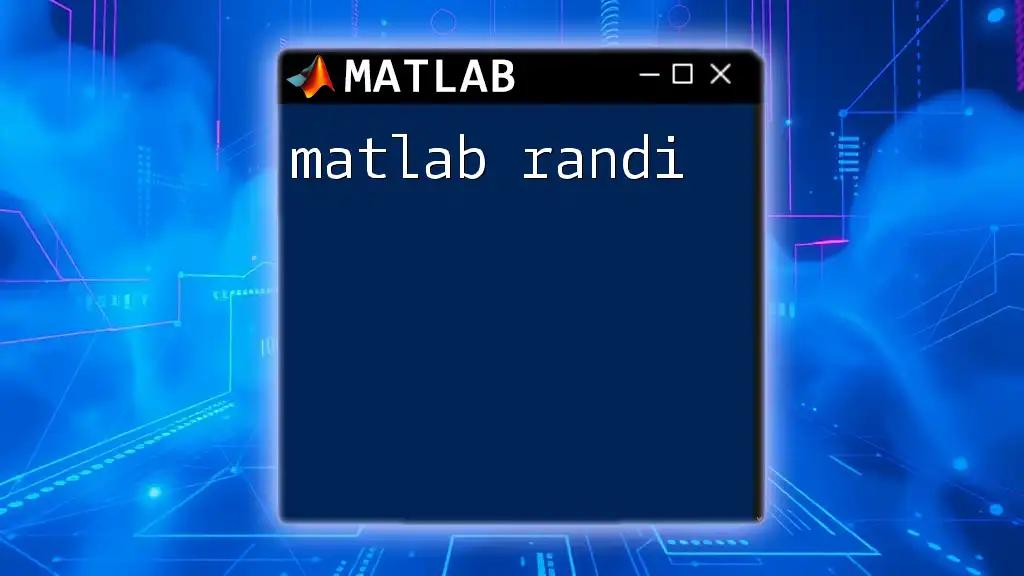
FAQs about the `break` Command
Why Use `break` Instead of `return`?
While both `break` and `return` serve to exit execution, they operate differently. `break` is used to leave the nearest loop, while `return` will exit a function and return control to the calling function. Understanding this distinction is crucial for employing the correct command based on your programming needs.
Can I Use `break` in Functions?
Yes, you can use the `break` command within functions. However, be mindful that it will only exit loops within the function's scope, not the function itself. This distinction is important for maintaining appropriate control flow in your functions.
By experimenting with these concepts and consistently applying them, you will master the `break` command and enhance your MATLAB programming capabilities.