The `repmat` function in MATLAB replicates and tiles an array, allowing you to create larger matrices from smaller ones efficiently.
A = [1, 2; 3, 4]; % Original matrix
B = repmat(A, 2, 3); % Replicate A into a 2x3 block
Understanding the Syntax of `repmat`
Basic Syntax
The basic syntax for the MATLAB `repmat` function is quite straightforward. It is used to replicate an array along specified dimensions. The function signature looks like this:
B = repmat(A, m, n)
In this function:
- A is the input array that you want to replicate.
- m is the number of times you want to replicate `A` in the row dimension.
- n is the number of times you want to replicate `A` in the column dimension.
For example, if you have a matrix `A`, and you want to duplicate it 2 times along rows and 3 times along columns, you would do the following:
A = [1, 2; 3, 4];
B = repmat(A, 2, 3); % This creates a matrix B that replicates A
Additional Syntax Options
You can also use an alternative syntax with a single vector argument:
B = repmat(A, sz)
Here, sz is a 1-by-N vector that specifies the replication counts for each dimension.
For example, to replicate a 2D array into a 3D array, you can specify the replication for all dimensions in one go:
A = [1, 2; 3, 4];
B = repmat(A, [1, 2, 2]);
The above code replicates matrix `A` once in the first dimension, twice in the second dimension, and twice in a hypothetical third dimension.
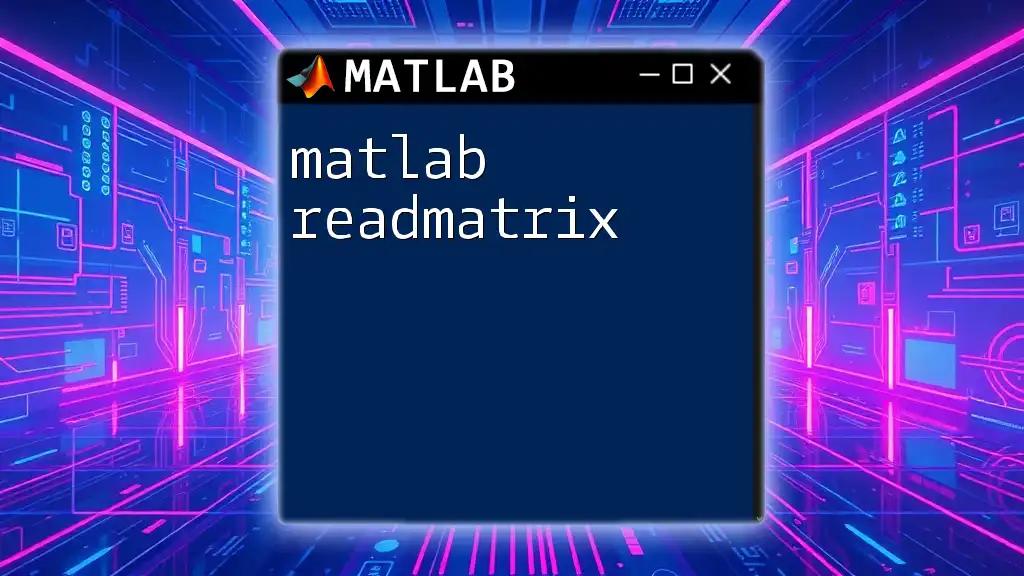
Why Use `repmat`?
Eliminating Loops
One of the significant advantages of using `repmat` is that it eliminates the need for loops when creating larger matrices. MATLAB is optimized for matrix operations, and utilizing `repmat` can drastically improve the performance of your code.
Consider a scenario where you might want to replicate a vector multiple times. Instead of writing a loop, you can achieve the same result succinctly with `repmat`, making your code cleaner and faster.
Efficiency in Code
When working with large datasets, writing efficient code is crucial. The use of `repmat` not only leads to more readable code, but also allows MATLAB to optimize memory allocation and computational tasks behind the scenes. This results in improved execution times especially when dealing with larger arrays.
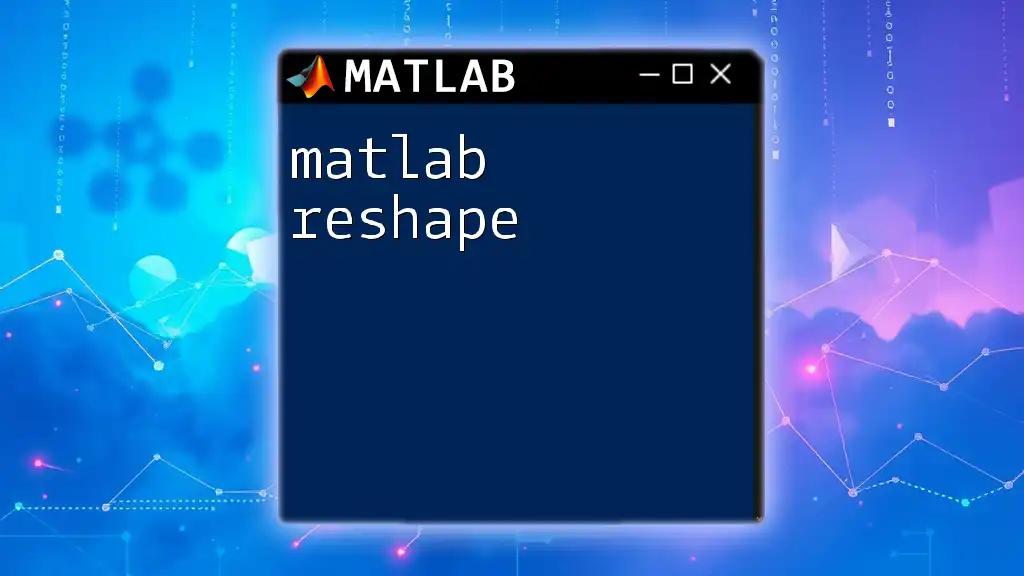
Use Cases for `repmat`
Creating Test Data
`repmat` is particularly useful in generating test data for simulations or testing algorithms. For instance, suppose you need a matrix where a specific pattern is repeated. `repmat` can create that pattern quickly and efficiently.
Example:
A = [1; 2; 3]; % Column vector
B = repmat(A, 1, 4); % Create a matrix by replicating A in a single row four times
This results in:
B =
1 2 3 1 2 3 1 2 3 1 2 3
Image Processing Applications
In image processing, you often need to manipulate the color channels of images. Each color channel may need to be repeated to match the size of another image. The `repmat` function excels in such scenarios, allowing for quick adjustments.
Matrix Operations
In linear algebra, replicating matrices can prepare them for calculations. For example, if you need a matrix that fits a model's requirement for dimensions, using `repmat` can prepare your data accordingly.
For instance:
A = [1, 2; 3, 4];
B = repmat(A, 2, 2); % A 4x4 matrix is created
This approach is not just for visualization; it aids in computations that require matrices of specific dimensions.
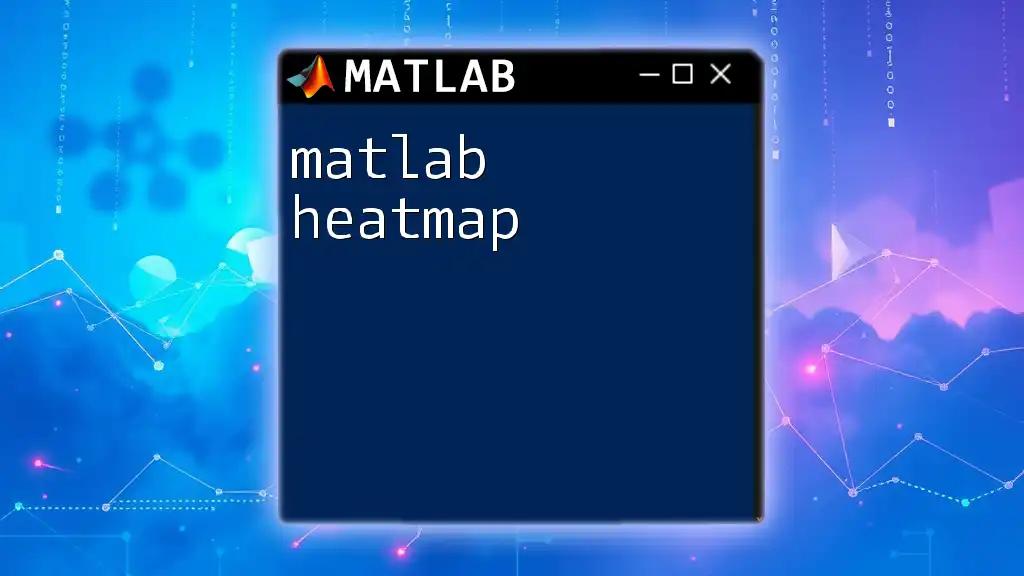
Examples of `repmat`
Basic Example
Consider a simple demonstration of `repmat`:
A = [5, 10; 15, 20];
B = repmat(A, 2, 3);
disp(B);
This code will replicate the matrix `A` to create a larger 4x6 matrix `B`.
Example with 3D Arrays
For users who want to extend their array manipulations into three dimensions, here is how you would do so with `repmat`:
A = [1, 2; 3, 4];
B = repmat(A, [1, 2, 2]);
disp(B);
This results in an array where each layer of the third dimension consists of the 2D matrix `A`, replicated across two layers.

Advanced Topics Related to `repmat`
Combining with Other Functions
`repmat` can be combined with other MATLAB functions to achieve complex data structures. Functions like `reshape`, `cat`, and even `bsxfun` can work hand-in-hand with `repmat` for powerful data manipulations.
For instance, `repmat` can be used before `reshape` to prepare an array for reshaping into a different dimensional structure.
Performance Considerations
While `repmat` is efficient, it is also important to consider the array sizes being handled. Replicating very large arrays can lead to significant memory usage. Understanding MATLAB's memory management and preallocating your matrices whenever possible can help mitigate performance issues.
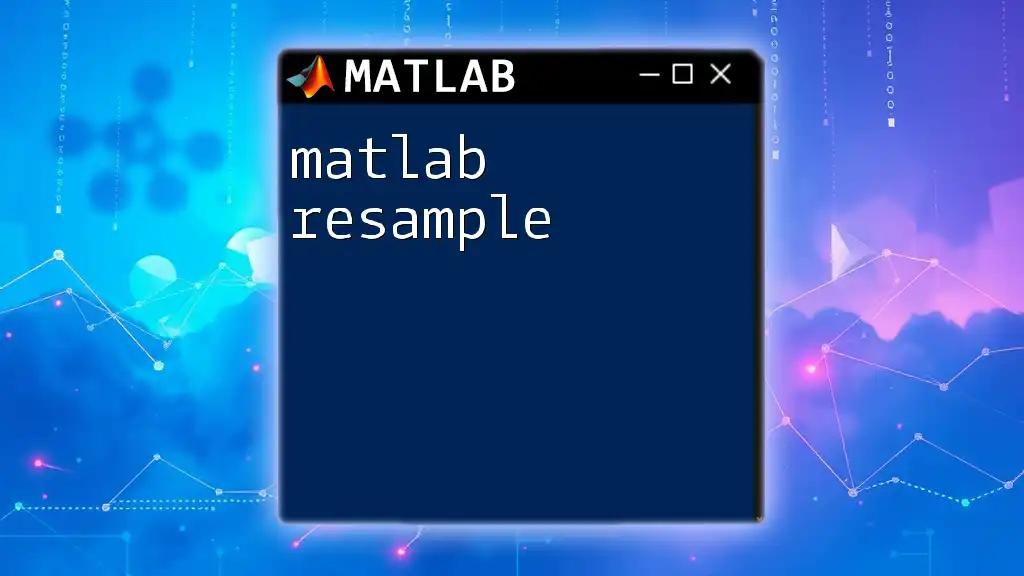
Common Mistakes with `repmat`
Mismatched Dimensions
One common mistake is attempting to replicate matrices where the sizes are incompatible. This can lead to unexpected results or errors. Always ensure that the dimensions you are replicating are suitable for the intended manipulation.
Not Preallocating Memory
Preallocation can save time and resources. If you are generating a large matrix, consider whether you can define its size before using `repmat`. Skipping this step can lead to slower performance.
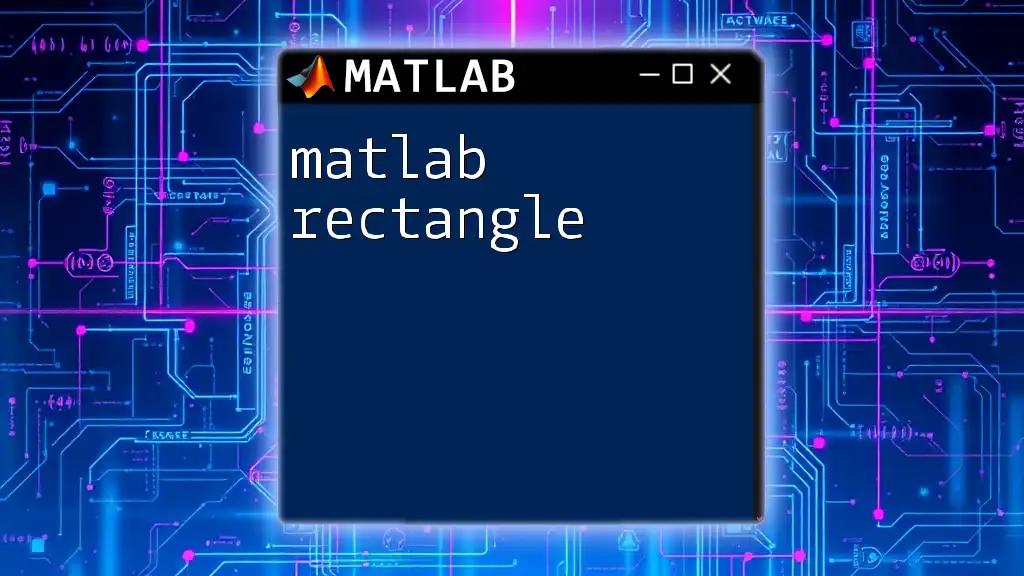
Conclusion
In this comprehensive guide, we have explored the MATLAB `repmat` function and its practical applications. By understanding its syntax, advantages, and use cases, you can employ `repmat` to simplify your coding tasks and enhance your data processing efficiency. We encourage you to practice its implementation in your projects to fully grasp its capabilities and explore the various ways you can manipulate data more efficiently in MATLAB.
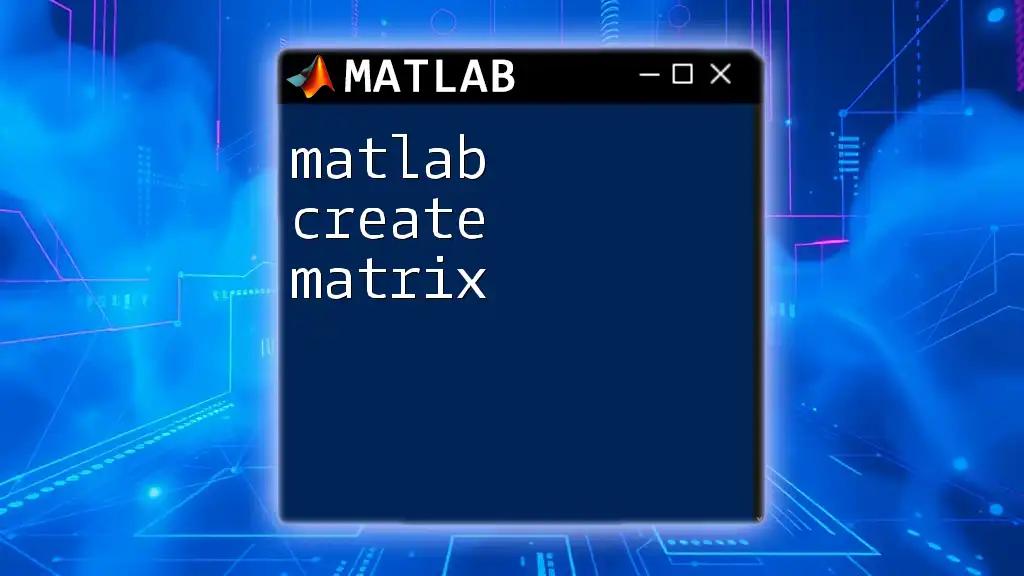
Additional Resources
For more in-depth knowledge and understanding, consider visiting the official MATLAB documentation on `repmat`. You may also want to explore tutorials and exercises available on various platforms to further reinforce your learning.