The `resample` function in MATLAB is used to change the sample rate of a signal by resampling it to a new frequency, which can be particularly useful in signal processing.
Here's a simple code snippet demonstrating its usage:
% Original signal
t = 0:0.01:1; % Time vector
x = sin(2*pi*10*t); % Sampled at 100 Hz
% Resampling
p = 2; % Upsampling factor
q = 1; % Downsampling factor
y = resample(x, p, q); % Resampling the signal
Understanding Resampling in MATLAB
What is Resampling?
Resampling is a method used to change the sampling rate of a discrete signal. It may involve increasing or decreasing the number of samples in a dataset and is essential in many fields such as signal processing, time series analysis, and image processing. The significance of resampling lies in the ability to interpolate or decimate data effectively, ensuring that the analysis remains accurate and relevant.
Types of Resampling Techniques
Resampling techniques can generally be classified into two categories: interpolation and decimation.
- Interpolation refers to the method of estimating unknown values that fall within the range of known data points. This technique allows us to create new data points from existing data.
- Decimation is the process of reducing the number of samples in a dataset while preserving its essential characteristics.
Several methods are commonly used for resampling:
- Linear Interpolation: Simple and fast, it creates new points linearly connecting two known points.
- Spline Interpolation: More complex, it uses polynomial functions for smoother results.
- Other methods may include nearest-neighbor, piecewise constant, and more.
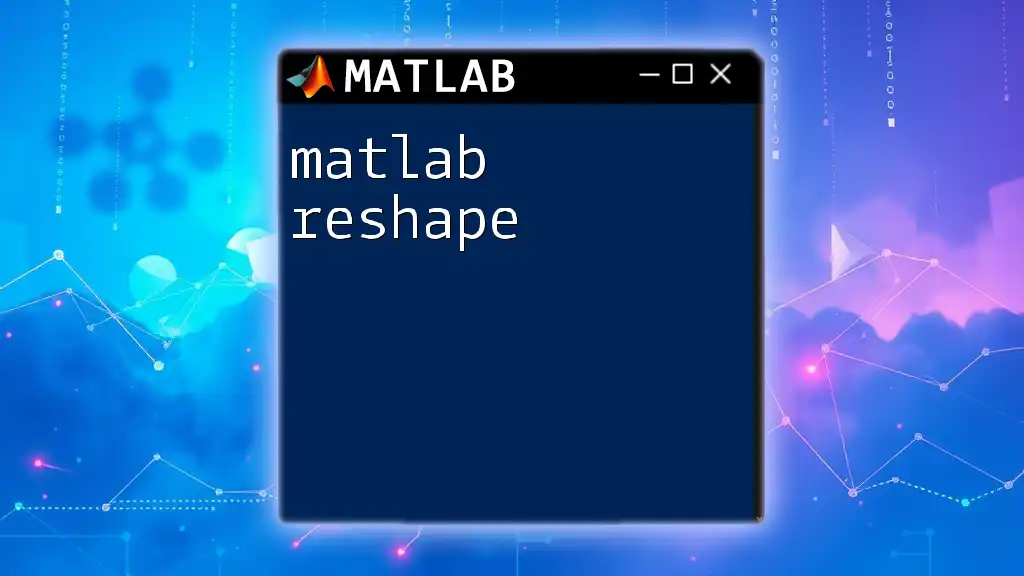
Getting Started with MATLAB Resample Function
Introduction to the `resample` Function
In MATLAB, the built-in `resample` function streamlines the resampling process for signals and sequences. The basic syntax for the `resample` function is:
[y, t_new] = resample(y, t, p, q)
Here:
- `y` is your original signal.
- `t` is the original time vector.
- `p` represents the new sampling frequency (numerator).
- `q` represents the original sampling frequency (denominator).
Basic Usage of `resample`
Using the `resample` function is straightforward. For instance, consider a simple sine wave signal that we want to resample:
t = 0:0.01:2*pi;
y = sin(t);
p = 2;
q = 1;
[y_resampled, t_resampled] = resample(y, t, p, q);
In this example, we defined a time vector `t` and a sine wave signal `y`. We set the new sampling rate to be double the original by using `p = 2` and `q = 1`. The resulting `y_resampled` will have more points than the original `y`, expanding our observation without losing the wave's integrity.
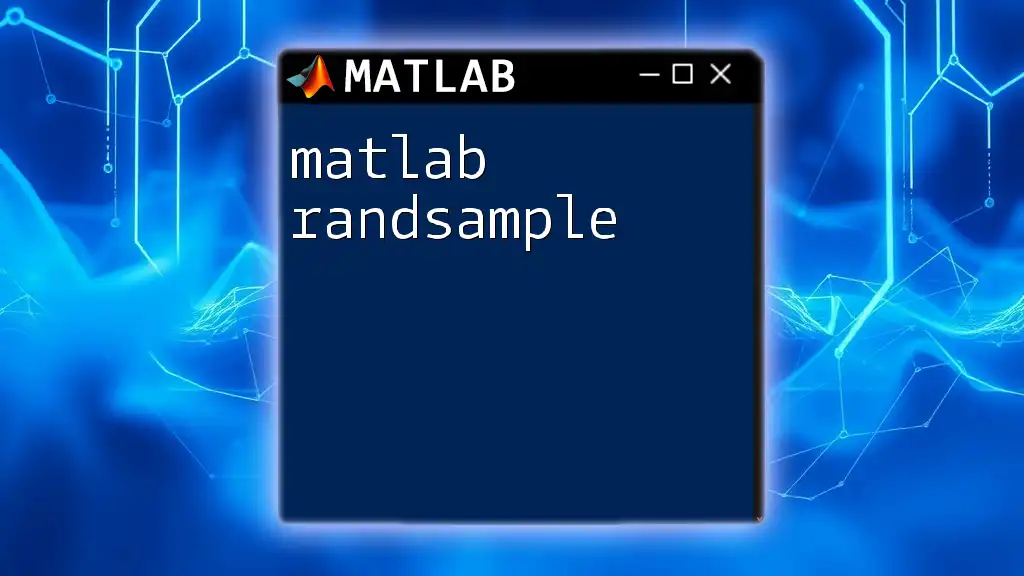
Advanced Resampling Techniques
Using Different Interpolation Methods
While `resample` covers many cases effectively, sometimes other interpolation methods, like the `interp1` function, may yield better results based on the nature of your data. For example, using linear interpolation to fill gaps can be implemented like this:
y_interp = interp1(t, y, t_new, 'linear');
This approach allows you to fill in gaps between your dataset more accurately, especially when dealing with time series data. The comparison of `resample` versus `interp1` is crucial in understanding when to choose one method over the other according to the specific requirements of your analysis.
Handling Non-Uniformly Sampled Data
Resampling isn't just limited to uniformly sampled data; it also applies to non-uniformly sampled data, which can present unique challenges. When resampling such data, you may encounter issues related to data sparsity or uneven distribution.
For example, let’s see how to handle and resample non-uniform data:
t_nonuniform = sort(rand(1, 100) * 10);
y_nonuniform = sin(t_nonuniform);
new_t = 0:0.1:10;
[y_resampled_nonuniform, t_new_nonuniform] = resample(y_nonuniform, t_nonuniform, 5, 1);
In this code snippet, we generated a non-uniform time vector `t_nonuniform` and calculated the corresponding sine values. The result from `resample` adjusts the data to fit a uniform time vector `new_t`, accommodating the inconsistencies of the original data while preserving its characteristics. It’s pivotal to visualize the resampled data to ensure accuracy, especially with non-uniform datasets.
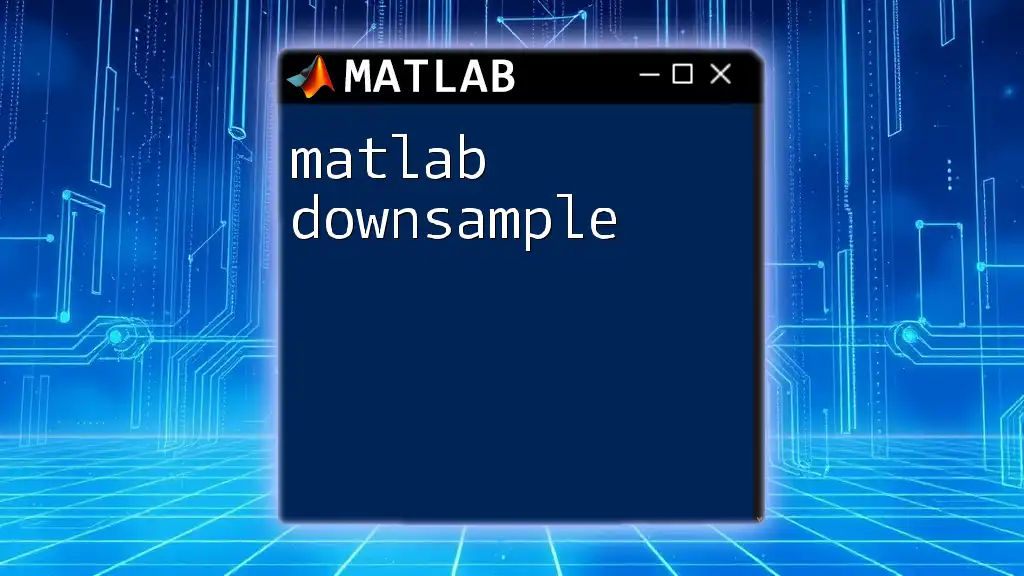
Practical Applications of Resampling
Time Series Analysis
In fields like finance and economics, accurate data interpolation is essential to perform meaningful time series analysis. For instance, when you have daily stock prices, but need to study trends on a monthly basis, resampling can help. You can apply `resample` to aggregate the data meaningfully while maintaining key characteristics.
Audio Signal Processing
Resampling is also extensively used in the field of audio signal processing. Changing the sample rate can enhance audio quality and optimize playback for various devices. For example, consider this code to change the sampling frequency of an audio file:
[x, fs] = audioread('audiofile.wav');
x_resampled = resample(x, 44, 22); % Changing sampling frequency from 22 kHz to 44 kHz
By utilizing `resample` in this scenario, one can ensure that the sound quality is not compromised during the process while still adapting the signal to match newer standards or capabilities.
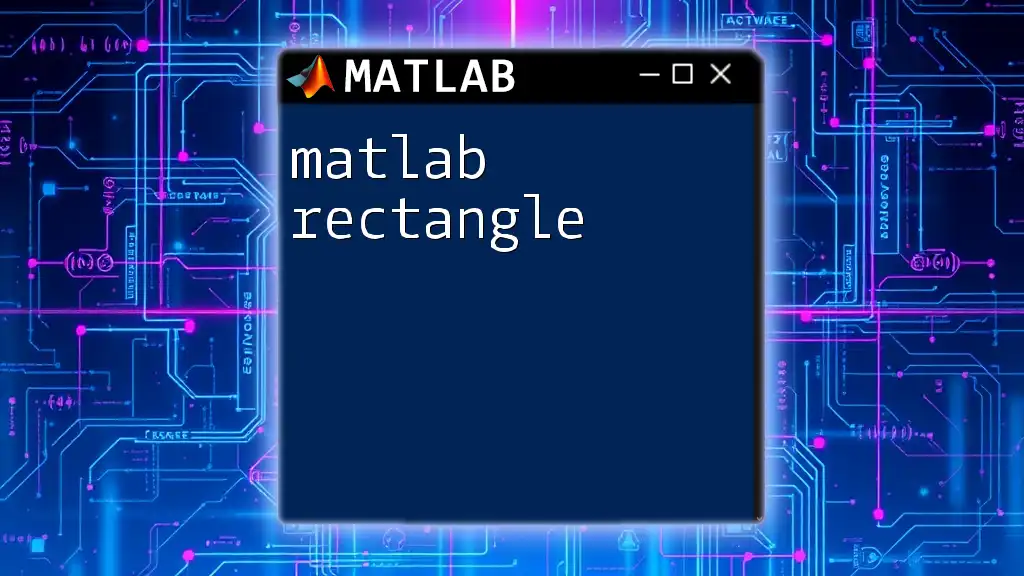
Troubleshooting Common Issues with Resampling
Common Errors
When using the MATLAB resample function, beginners often encounter several pitfalls, such as dimension mismatches between the input signals or incorrectly defined parameters. To troubleshoot, always check that your input signal dimensions align with the specified time vector and resampling rates.
Performance Considerations
When working with large datasets, performance can become an issue, impacting processing time and resource allocation. To improve performance, use vectorized operations when possible and preallocate memory for outputs. Understanding how MATLAB handles data can significantly speed up your analysis.
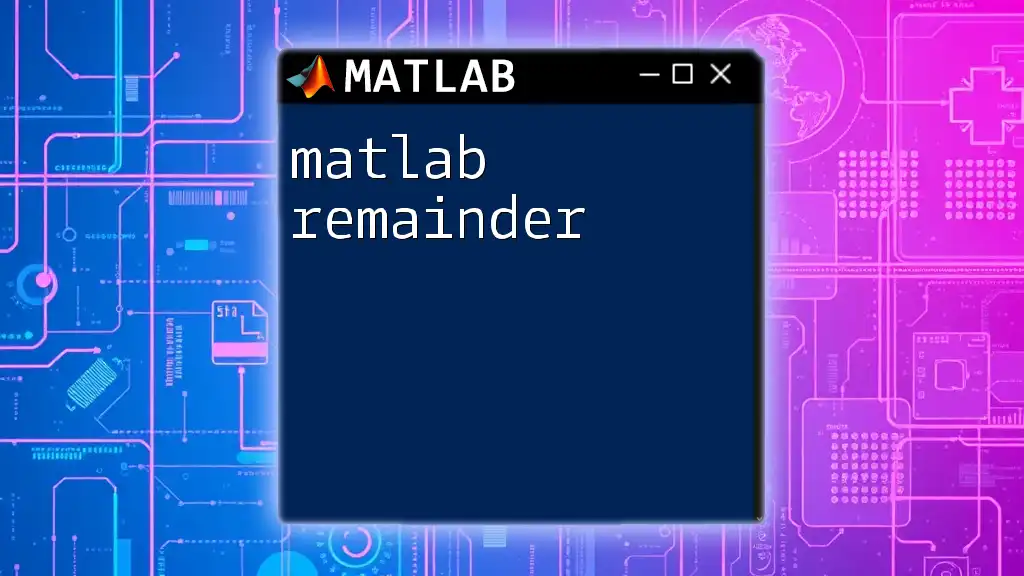
Conclusion
Summary of Key Points
In this comprehensive guide, we have explored the essence of MATLAB resample, looking at its function, variations of resampling techniques, practical applications, and how to troubleshoot common issues. Mastering the `resample` function will undoubtedly enhance your data analysis capabilities.
Call to Action
Start practicing with sample datasets to harness the power of MATLAB resample in real-world scenarios. Engage with community forums and share your experiences as you navigate through various resampling challenges. Remember, the more you practice, the more proficient you will become!