The "MATLAB area" refers to the calculation of geometric areas using MATLAB commands, which can be efficiently executed with built-in functions to simplify the process.
Here’s a code snippet to calculate the area of a circle given its radius:
radius = 5; % Radius of the circle
area = pi * (radius^2); % Area calculation
disp(['The area of the circle is: ', num2str(area)]);
Understanding MATLAB Area Calculations
What is MATLAB?
MATLAB, which stands for Matrix Laboratory, is a high-level programming language and interactive environment used extensively for numerical computation, data analysis, and algorithm development. Its powerful matrix-oriented capabilities make it particularly suitable for engineering tasks, scientific research, and advanced data visualizations. Mastering MATLAB commands is essential for effective problem-solving within various domains.
The Concept of Area in MATLAB
In both mathematical and programming contexts, the area refers to the measurement of a surface or a specific two-dimensional space within defined boundaries. Understanding area calculations in MATLAB is crucial, as these calculations apply widely in fields like engineering, physics, and even finance. The ability to swiftly compute areas can significantly enhance your analytical skills and the precision of your modeling efforts.
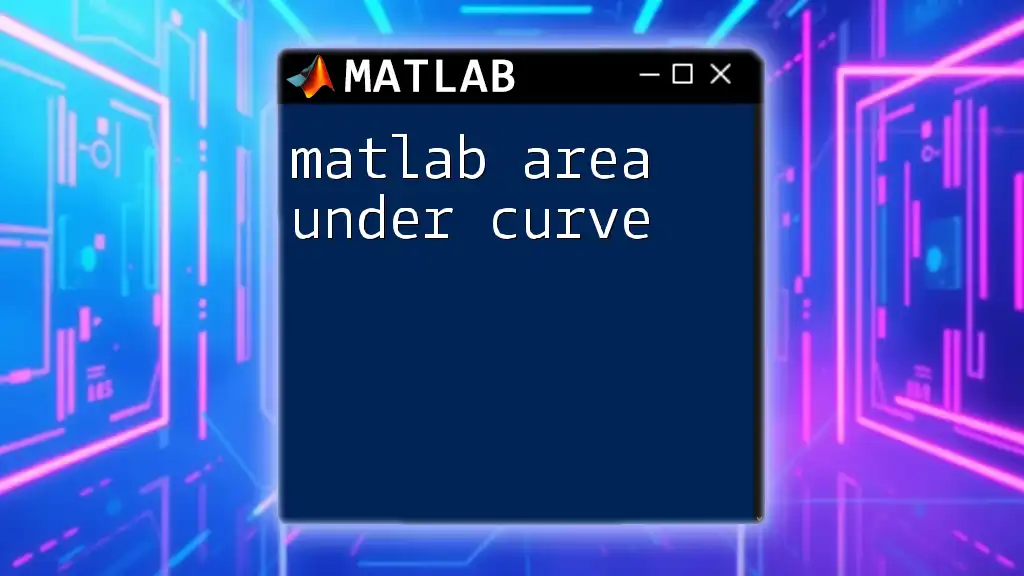
Basic Area Calculations
Area of Common Shapes
When calculating area in MATLAB, you can easily compute the area of common geometric shapes by applying simple formulas. Here are a few examples:
-
Rectangle The area of a rectangle is calculated using the formula: Area = length × width. Here’s how to implement it in MATLAB:
length = 5; % in units width = 3; % in units area_rectangle = length * width; fprintf('Area of Rectangle: %.2f\n', area_rectangle);
-
Circle To find the area of a circle, the formula is: Area = π × radius². This is straightforward to implement:
radius = 4; % in units area_circle = pi * radius^2; fprintf('Area of Circle: %.2f\n', area_circle);
-
Triangle The area of a triangle can be found using the formula: Area = 0.5 × base × height. You can compute this in MATLAB as follows:
base = 6; % in units height = 4; % in units area_triangle = 0.5 * base * height; fprintf('Area of Triangle: %.2f\n', area_triangle);
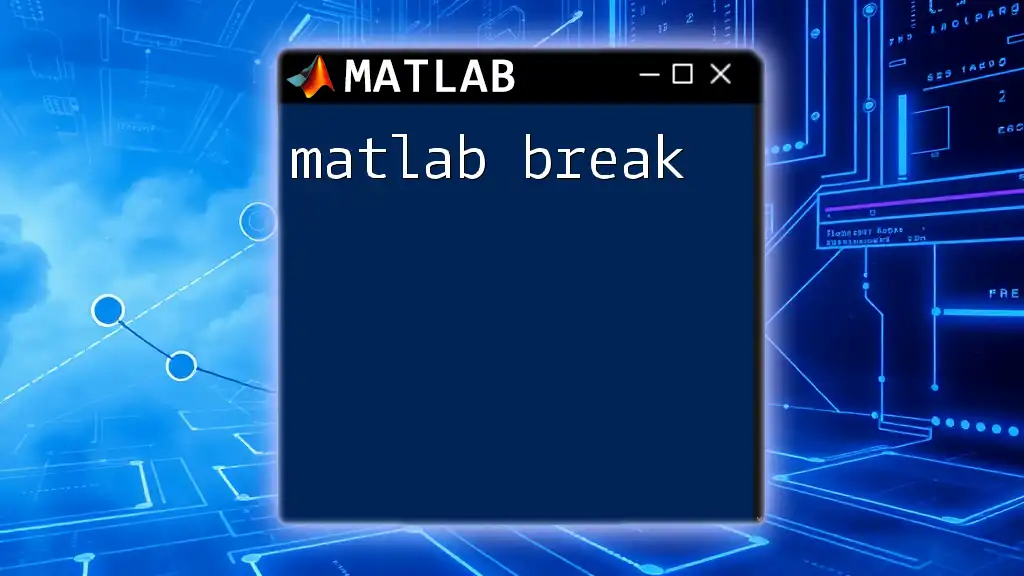
Advanced Area Calculations
Area Under Curves
When dealing with more complex shapes or functions, you may need to calculate the area under a curve, which requires integrating the function over a specific interval.
-
Introduction to Integration Integration is a fundamental concept in calculus that allows you to determine the area under a curve. There are two types of integrals: definite (which produces a numerical result) and indefinite (which results in a function).
-
Using MATLAB for Numerical Integration MATLAB makes it easy to perform numerical integration using the `integral` function. For example, to find the area under the curve defined by the function f(x) = x² between the limits of 0 and 3, you could use:
f = @(x) x.^2; % function for the curve area_under_curve = integral(f, 0, 3); fprintf('Area under the curve: %.2f\n', area_under_curve);
Working with Complex Shapes
MATLAB also provides tools to compute the area of irregular shapes using their vertices.
- Using Polygon Area Function
The `polyarea` function allows you to calculate the area of a polygon given the coordinates of its vertices. For example:
x = [0 1 0.5]; % x-coordinates of vertices y = [0 0 1]; % y-coordinates of vertices area_polygon = polyarea(x, y); fprintf('Area of Polygon: %.2f\n', area_polygon);
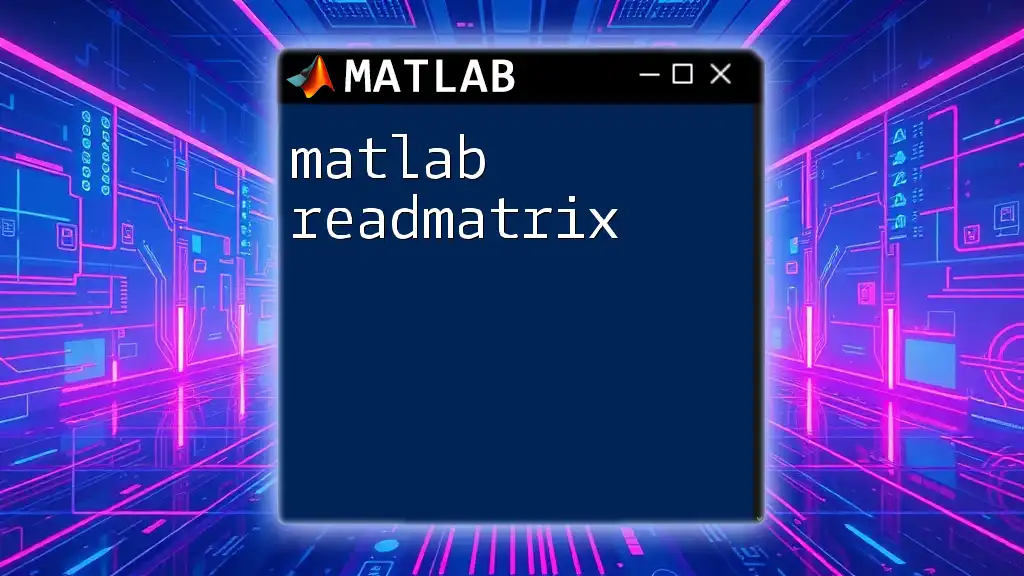
Visualizing Areas in MATLAB
Plotting Basic Shapes
A visual representation of the shapes and areas can enhance understanding. MATLAB’s `fill` function enables users to plot filled shapes easily. For instance, to visualize a rectangle:
x_rectangle = [0, 5, 5, 0];
y_rectangle = [0, 0, 3, 3];
fill(x_rectangle, y_rectangle, 'r');
title('Rectangle');
Area Under Curves Visualization
Graphing functions aids in visualizing area calculations. To illustrate the area under the curve of the function y = x², you would use:
fplot(@(x) x.^2, [0, 3]); % function plot
hold on;
area([0, 3], [0, 9], 'FaceColor', 'cyan', 'FaceAlpha', 0.5);
title('Area Under y=x^2');
hold off;
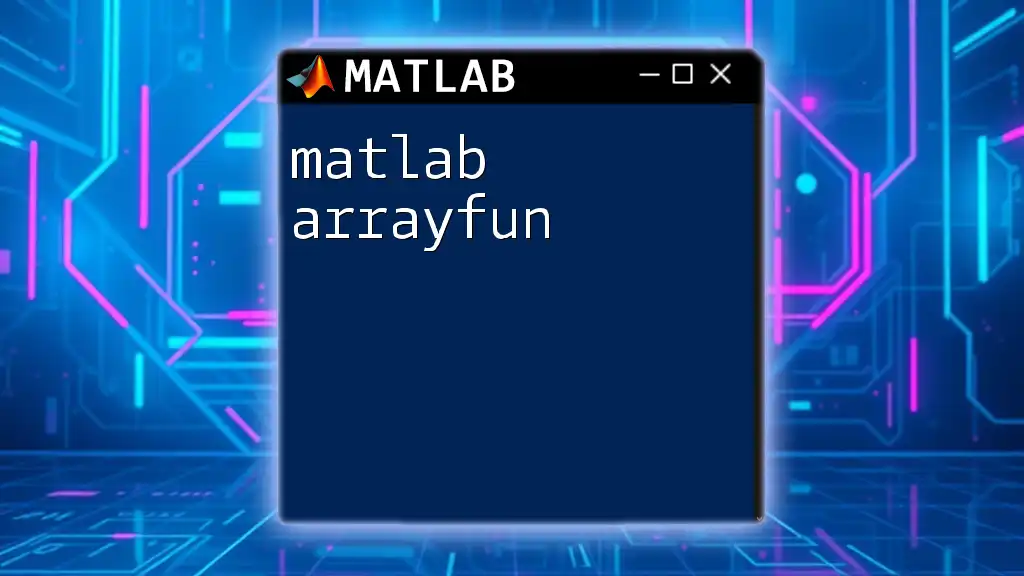
Practical Applications of Area Calculations
Engineering Applications
In engineering, accurate area calculations play a pivotal role in designing and analyzing structures. Whether calculating the cross-sectional area of materials or determining load distributions, the precise computation of areas can influence material selection, cost estimates, and overall design efficiency.
Scientific Research
In scientific contexts, area calculations can be instrumental for statistical analysis, including assessing probabilities in histograms or even in the field of ecological studies to quantify land areas. An example would be employing MATLAB to calculate areas in research involving environmental data, where area computations might represent regions of habitat loss or expansion.
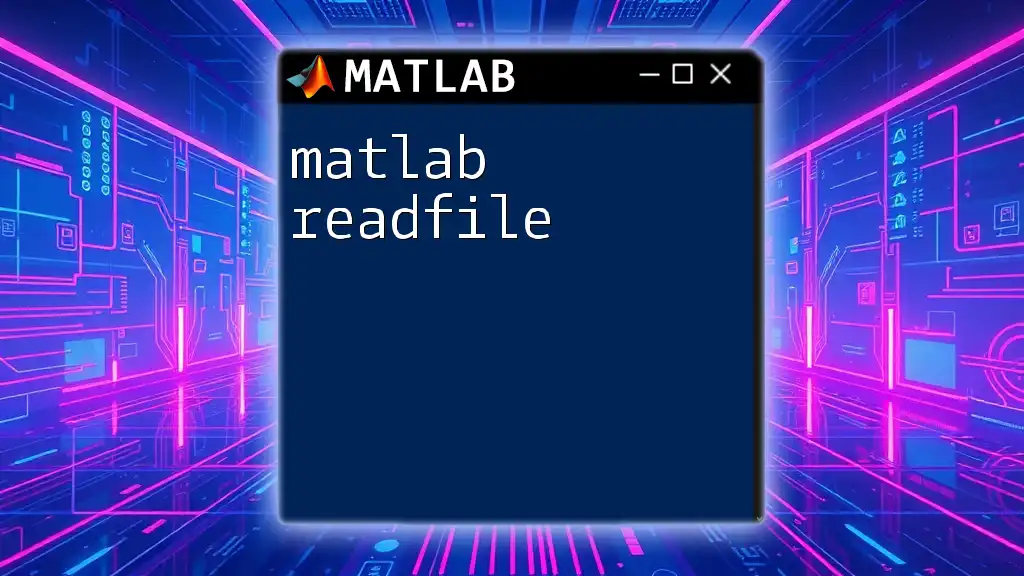
Conclusion
Summary of Key Points
Through this article, we’ve explored various techniques for calculating areas in MATLAB, from basic shapes to more intricate curves. The use of built-in functions simplifies calculations, making MATLAB a powerful ally in mathematical and engineering tasks.
Further Resources
For additional learning, consider exploring the official MATLAB documentation or enrolling in comprehensive online courses that dive deeper into MATLAB's capabilities.
Call to Action
To enhance your skills in MATLAB area calculations, practice using the provided examples, and feel free to reach out to the community for specific inquiries or further guidance. Engaging actively with others who are learning MATLAB can greatly accelerate your learning process and deepen your understanding.