The `regionprops` function in MATLAB measures the properties of labeled image regions, enabling analysis of features such as area, centroid, and bounding box.
Here's an example of how to use `regionprops`:
% Load a binary image
bw = imread('binary_image.png');
% Label connected components in the binary image
[labeledImage, numRegions] = bwlabel(bw);
% Measure properties of the labeled regions
props = regionprops(labeledImage, 'Area', 'Centroid', 'BoundingBox');
Understanding `regionprops`
What is `regionprops`?
The `regionprops` function in MATLAB is a powerful tool for measuring various properties of labeled regions in binary images. Primarily, it extracts meaningful quantitative information from segmented image data, which is essential in image analysis tasks across multiple fields such as biology, robotics, and manufacturing. Understanding `regionprops` allows users to efficiently analyze physical attributes of objects depicted in images, facilitating data-driven decisions.
Key Features of `regionprops`
The core strength of `regionprops` lies in its ability to extract and quantify a diverse set of properties. Some commonly measured properties include:
- Area: The total number of pixels within the region.
- Bounding Box: The smallest rectangle that contains the region, represented by a vector of `[x, y, width, height]`.
- Centroid: The center point of the region, typically calculated as the mean of the coordinate values of all pixels in the region.
- Major and Minor Axis Length: These indicate the lengths of the axes of the ellipse that fits the region.
- Eccentricity: A measure of the deviation of the ellipse from being circular (ranging from 0 for a circle to 1 for a line).
- Orientation: The angle (in degrees) of the major axis of the ellipse relative to the x-axis.
Each of these properties provides essential insights into the characteristics of the segmented objects, enabling various analyses depending on the domain of application.

Prerequisites for Using `regionprops`
Image Processing Basics
To effectively use `regionprops`, a basic understanding of image processing concepts is crucial. This includes knowledge of:
- Grayscale vs. Binary Images: `regionprops` works with binary images where pixels representing objects of interest are set to 1 (true), and all other pixels are set to 0 (false).
- Importance of Labeling Regions: The function requires that regions are labeled either through segmentation algorithms or by using MATLAB's built-in functions like `bwlabel`.
Required Toolboxes
Using `regionprops` necessitates having the Image Processing Toolbox installed in your MATLAB environment. Ensure your license includes this toolbox, as it provides essential functions for analyzing and visualizing image data.

Using `regionprops` in MATLAB
Basic Syntax
The syntax for `regionprops` can often be succinct and straightforward. The fundamental function call is structured as follows:
stats = regionprops(BW)
In this command, `BW` represents the binary image input, while `stats` is a structure array that holds the calculated properties for each labeled region. The properties returned can be tailored based on specific requirements during function invocation.
Common Use Cases
Measuring Object Properties
One of the most prevalent use cases for `regionprops` is measuring properties of connected components in binary images. Here’s how you can implement it:
BW = imread('image.png'); % Load binary image
stats = regionprops(BW, 'Area', 'Centroid'); % Measure properties
In this example, `stats` will contain the areas and centroids of all objects in the binary image. Each property can be accessed by referencing the specific field in the `stats` structure.
Analyzing Shapes in Images
In fields like medicine, analyzing shapes in images can provide insights into conditions or phenomena. A typical usage involves overlaying centroids on the original binary image:
imshow(BW);
hold on;
for k = 1:numel(stats)
plot(stats(k).Centroid(1), stats(k).Centroid(2), 'r*');
end
hold off;
This code snippet enhances the binary image visualization by marking the centroid of each region, facilitating further analysis.
Advanced Features of `regionprops`
Customizing Property Measurements
`regionprops` offers flexibility in property selection based on analytical needs. To extract multiple properties at once, you can specify them in a cell array:
stats = regionprops(BW, 'Area', 'BoundingBox', 'Eccentricity');
This will return a structure containing only the specified properties, which can simplify data analysis by focusing on what’s relevant to your research.
Using `regionprops` with Custom Functions
For even more specialized analyses, users can create custom property functions. By integrating `regionprops` with custom logic, researchers can extract unique metrics tailored to their project requirements:
function customStats = myPropertyFunction(BW)
stats = regionprops(BW, 'Area', 'Perimeter');
customStats = struct('Area', {stats.Area}, 'Perimeter', {stats.Perimeter});
end
With this custom function, users can collate specific metrics relevant to their project, facilitating targeted analysis without extraneous data clutter.
Performance Considerations
When working with large images or multiple datasets, performance and memory management become crucial.
- Best Practices: Efficiently manage variable usage and clear off unnecessary temporary variables from workspace using `clear`.
- Batch Processing: If analyzing multiple images, consider vectorized operations or processing them in batches to minimize overhead.
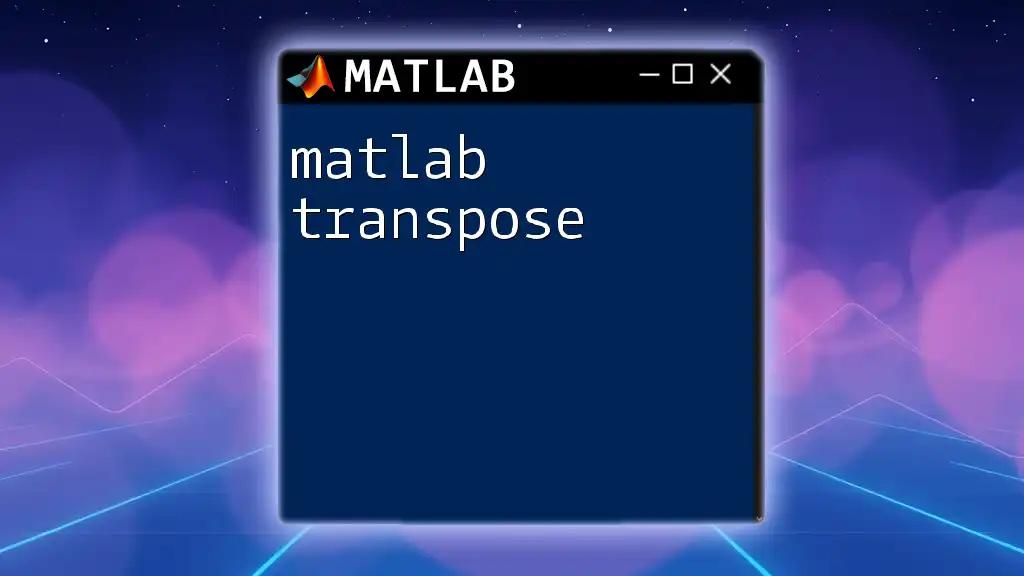
Practical Applications of `regionprops`
Applications in Various Fields
`regionprops` finds extensive applications across different domains:
- Healthcare: In medical imaging, `regionprops` is used to analyze tumors, allowing for precise measurements that assist in diagnosis and treatment planning.
- Manufacturing: In quality control, it analyzes the shape and dimensions of products to identify defects.
- Robotics: For machine vision tasks, it helps robots understand their environment better, allowing for more effective navigation and interaction with objects.
Case Study Example
An in-depth case study might focus on the analysis of tumor images. By applying a segmentation algorithm to extract tumors from MRI scans, one could use `regionprops` to summarize the area, centroid, and shape features. Through this analysis, practitioners can derive critical insights regarding tumor growth patterns and their implications.
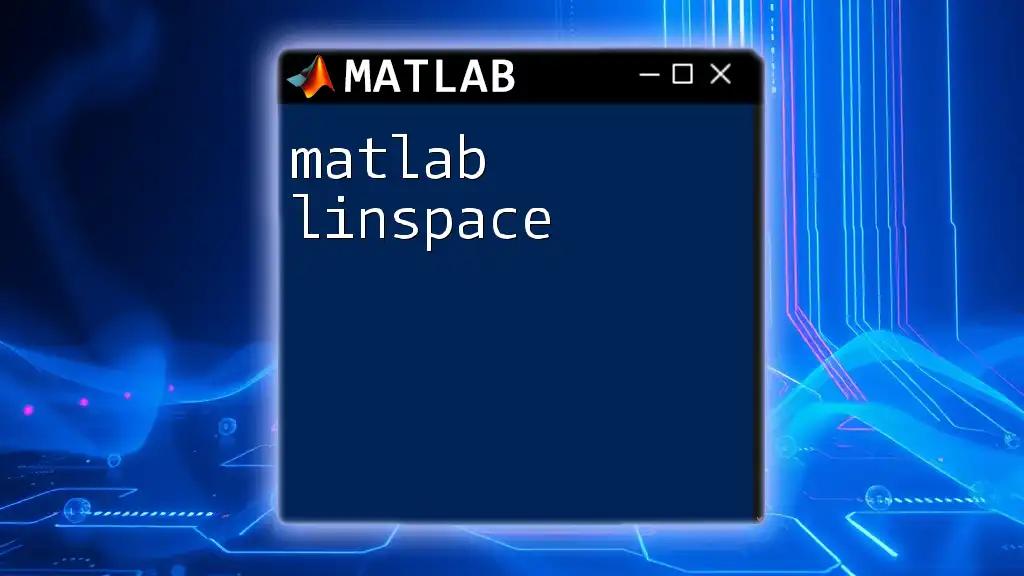
Troubleshooting Common Issues
Common Errors and Fixes
When using `regionprops`, users might encounter typical errors, such as:
-
Image Format Issues: Ensure the input is a binary image, as `regionprops` requires labeling to function correctly. If you try inputting a grayscale image directly, errors will arise.
-
Invalid Property Names: Make sure that the properties requested exist. Check MATLAB documentation to confirm variable names.
Optimization Tips
To optimize performance and ensure your analysis scales well:
- Handle Large Datasets with Care: Evaluate whether you need to process every pixel or region. Sometimes dilating or eroding the image prior to processing can yield better performance.
- Use Functions Efficiently: Combine `regionprops` with functions like `bwconncomp` for even more control over connected components.
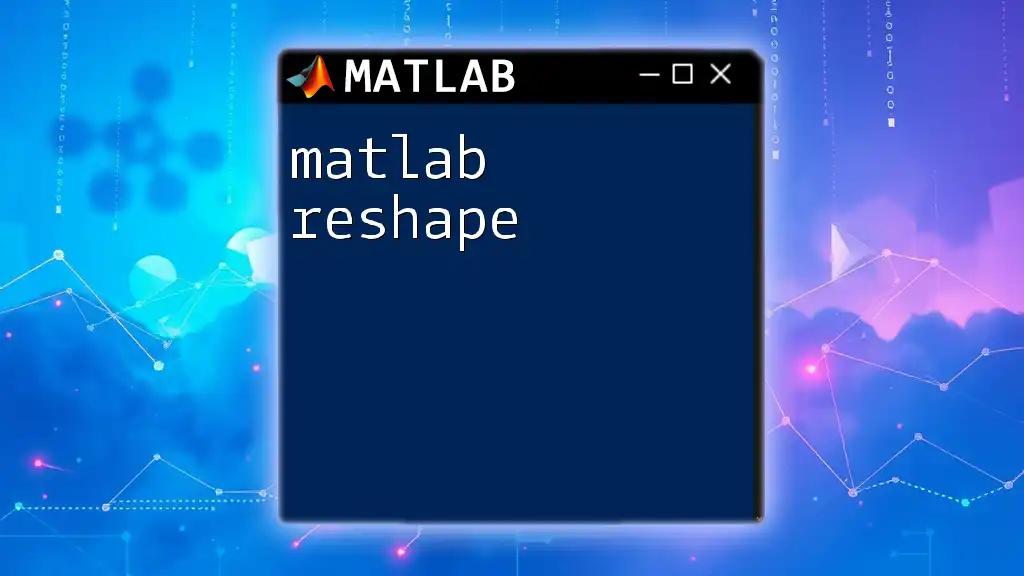
Conclusion
In summary, `regionprops` in MATLAB is an indispensable function for analyzing the geometric properties of various objects within images. Mastering its applications and functionalities equips users to undertake extensive analyses across multiple domains, enhancing decision-making processes based on quantitative data. Exploring this function opens up numerous possibilities in image analysis and interpretation, making it a fundamental tool for practitioners in the field.