In MATLAB, you can create a histogram with the y-axis displayed as percentages by normalizing the histogram data and using the `histogram` function with the `'Normalization'` option set to `'probability'`.
Here's a code snippet demonstrating how to do this:
data = randn(1000,1); % Generate random data
histogram(data, 'Normalization', 'probability'); % Create histogram with y-axis as percentage
ylabel('Percentage'); % Label y-axis
title('Histogram with Y-Axis as Percentage'); % Title of the histogram
What is a Histogram?
A histogram is a graphical representation that organizes a group of data points into specified ranges, known as bins. The height of each bin reflects the frequency of data points within that range, making it an effective tool for visualizing data distributions. Histograms provide insight into the underlying frequency distribution of a dataset, helping to identify patterns, trends, and outliers.
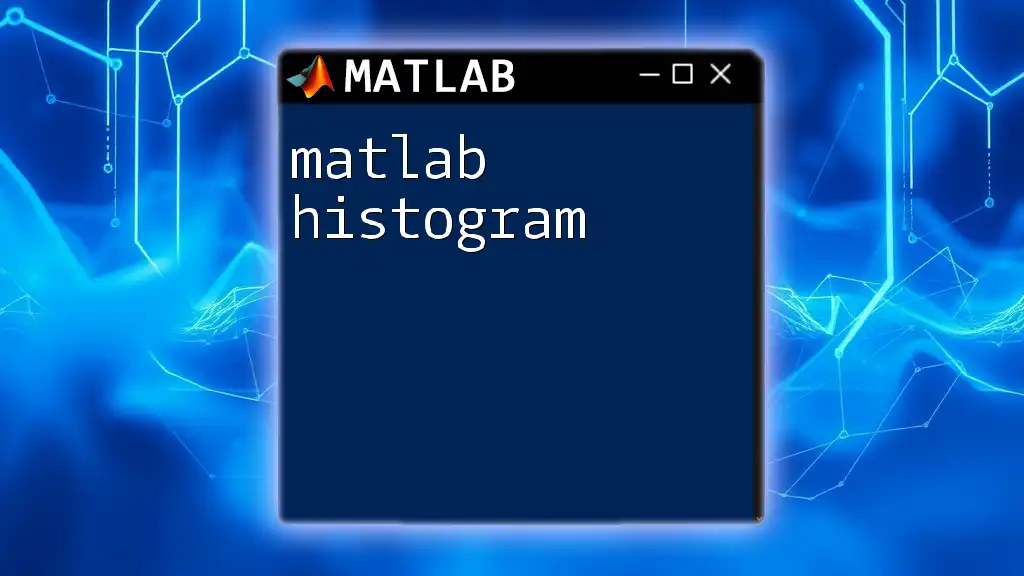
Why Use Percentage on the Y-Axis?
When working with histograms, you may choose to display the Y-axis as a percentage instead of the raw frequency count. This approach offers several benefits:
- Standardization: Percentage provides a way to compare different datasets more easily, regardless of their sample sizes.
- Interpretability: Interpreting data becomes more straightforward when you think in terms of percentages, especially when presenting findings to an audience.
- Communication: It helps to convey probabilities, making it easier to understand likely outcomes from the data.
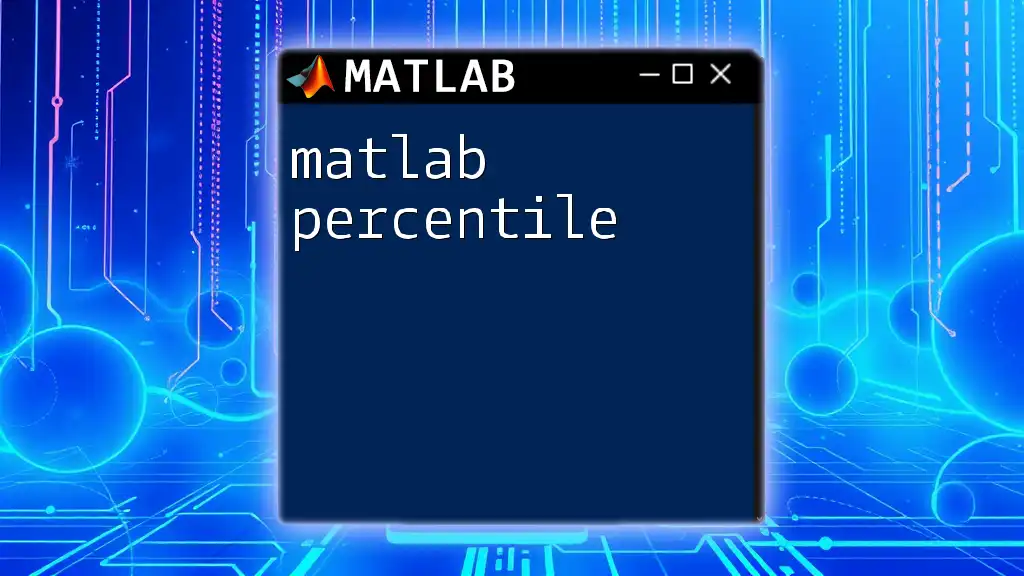
Understanding MATLAB Histograms
Basics of Creating a Histogram in MATLAB
To create a histogram in MATLAB, the `histogram` function is employed. This function allows you to customize various aspects of the histogram, from the number of bins to the appearance of the graph.
To get started, let’s look at a basic example. Here’s how you can create a simple histogram:
data = randn(1000, 1); % Example data: normally distributed
histogram(data);
title('Basic Histogram');
xlabel('Value');
ylabel('Frequency');
In this example, `randn(1000, 1)` generates 1,000 random numbers drawn from a standard normal distribution. The `histogram` function automatically determines the number of bins and displays the frequency of data points.
Customizing the Histogram
Once you've generated your basic histogram, you can customize it by:
- Changing the number of bins: Use the second argument in the `histogram` function to define the number of bins explicitly.
- Setting edges and limits: Customizing the bin edges enables you to focus on specific ranges of your data.
- Adding labels and titles: Ensuring your histogram is properly titled and labeled improves its clarity and effectiveness.
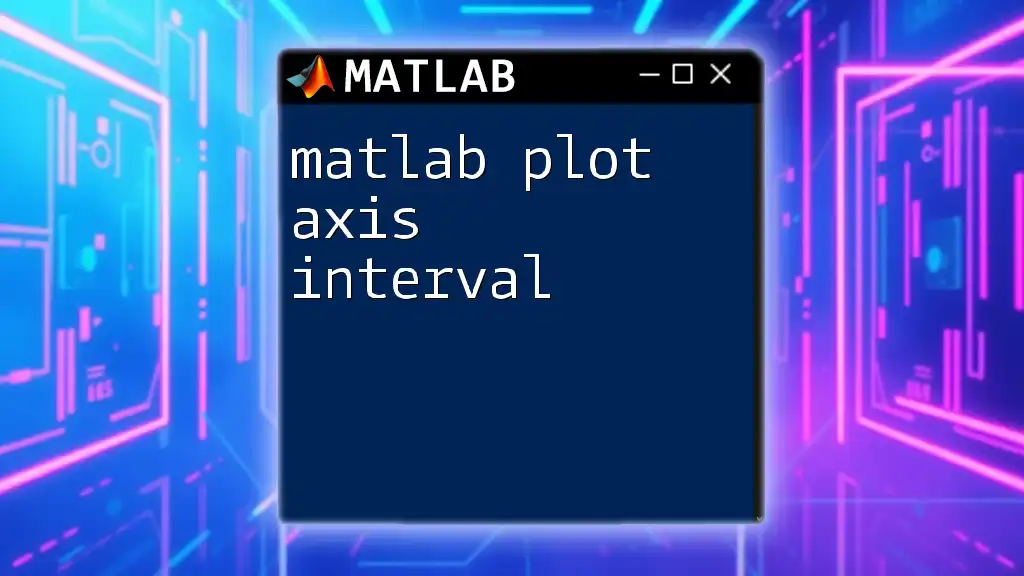
Converting to Percentage Y-Axis
Understanding the Concept of Normalization
Normalization refers to the process of adjusting values measured on different scales to a common scale. In the context of histograms, it means converting frequency counts into probabilities or percentages. This is particularly important when your data varies significantly in size or when you want to present a clear interpretation based on percentages.
To transform frequency counts into percentages, you would calculate the percentage counts:
\[ \text{Percentage} = \left( \frac{\text{Frequency}}{\text{Total Count}} \right) \times 100 \]
Applying Percentage to Y-Axis in MATLAB
To display the Y-axis as a percentage in MATLAB, you can use the normalization parameter in the `histogram` function. Here’s how you can do that:
data = randn(1000, 1);
h = histogram(data, 'Normalization', 'probability'); % Normalized histogram
title('Histogram with Y-Axis as Percentage');
xlabel('Value');
ylabel('Percentage');
In this code snippet, the `Normalization` option is set to `'probability'`, which automatically scales the heights of the bars to represent their proportionate frequency in relation to the total count.
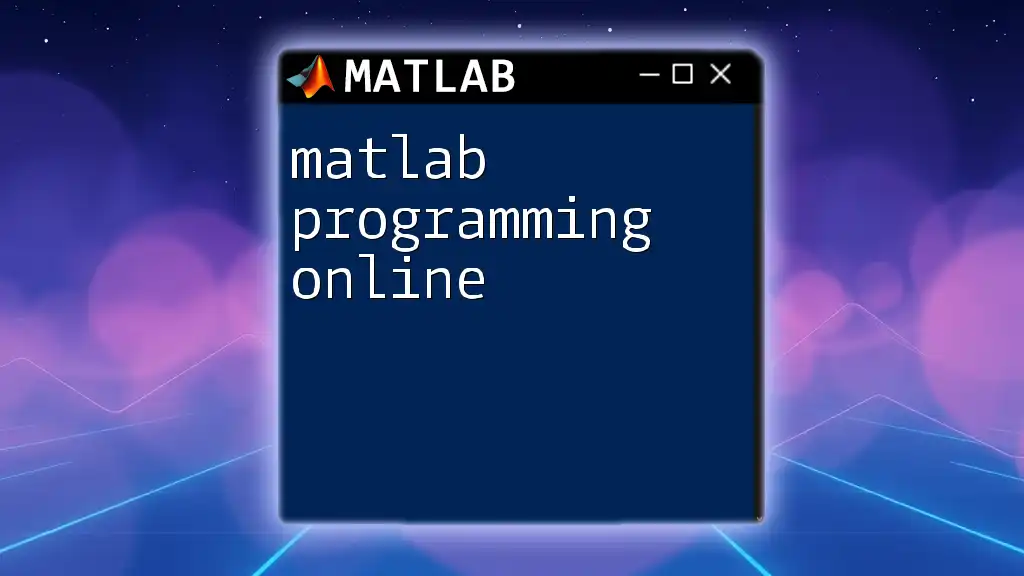
Advanced Histogram Customizations
Customizing the Appearance
To improve the aesthetic appeal of your histogram, consider customizing:
- Bar colors: Alter the color of your bars for clarity and visual interest.
- Opacity: Adjust transparency using the `'FaceAlpha'` property for layers of information.
- Grid lines: Adding grid lines enhances readability, especially in crowded histograms.
Adding a Cumulative Histogram
A cumulative histogram illustrates the cumulative distribution of the data, allowing viewers to see the total number of data points up to each bin. To create a cumulative histogram with a percentage Y-axis, use the cumulative distribution function (CDF):
h = histogram(data, 'Normalization', 'cdf'); % Cumulative distribution function
title('Cumulative Histogram with Y-Axis as Percentage');
xlabel('Value');
ylabel('Cumulative Percentage');
By setting `'Normalization'` to `'cdf'`, you show the cumulative probability, providing a complete view of the data distribution over the defined range.
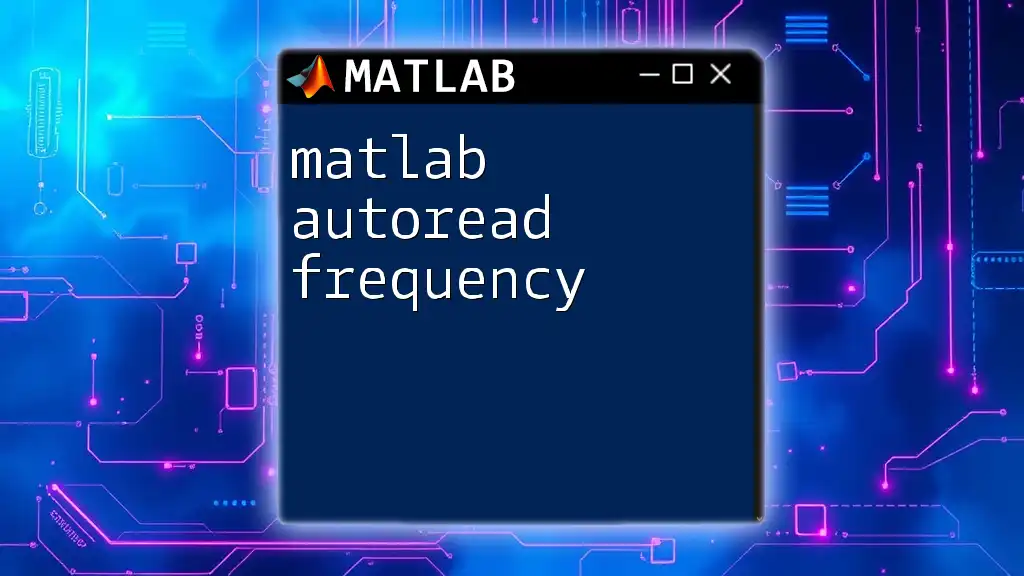
Practical Applications of Percentage Histograms
Percentage histograms are widely used in various fields, including:
- Finance: Analysts use them to visualize returns, risk distributions, or customer segments.
- Healthcare: They can help in understanding the distribution of patient outcomes or treatment effectiveness.
- Academia: Researchers often use them to present statistical findings, making trends easier to evaluate.
Case studies often illustrate the effective use of percentage histograms in analyzing performance metrics in business or studying research data in universities.
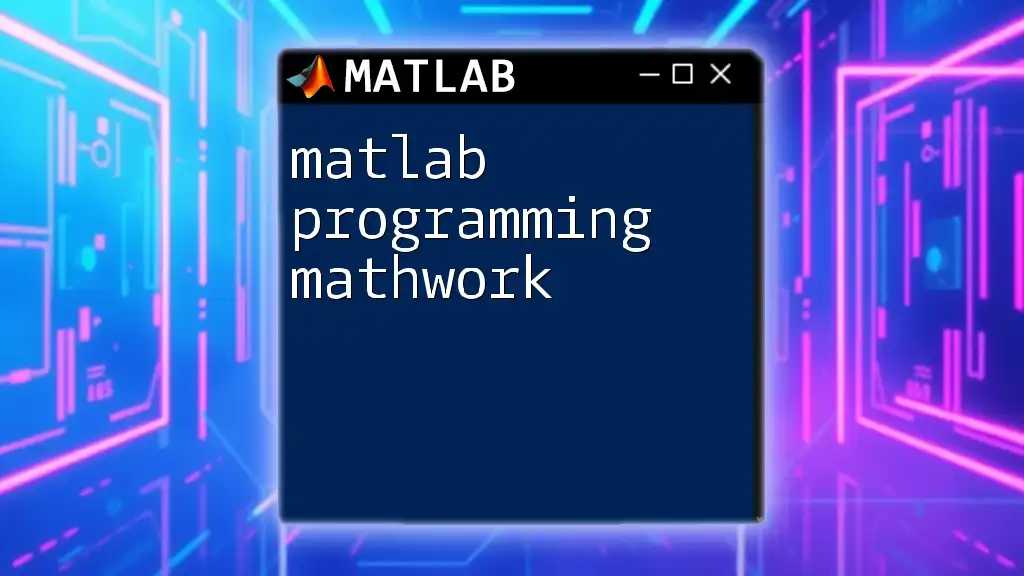
Troubleshooting Common Issues
When working with histograms in MATLAB, you might encounter common pitfalls, such as:
- Improper bin sizes: Too few or too many bins may obscure the true data distribution. Adjust accordingly.
- Incorrect normalizations: Ensure you're using the proper normalization type to accurately reflect your data's intent.
- Validation of outputs: Compare against known distributions or check with summary statistics to confirm histogram accuracy.
Tips for validating outputs include reviewing the data set distribution, checking summary statistics like mean and standard deviation, and confirming that graphical displays match numerical insights.
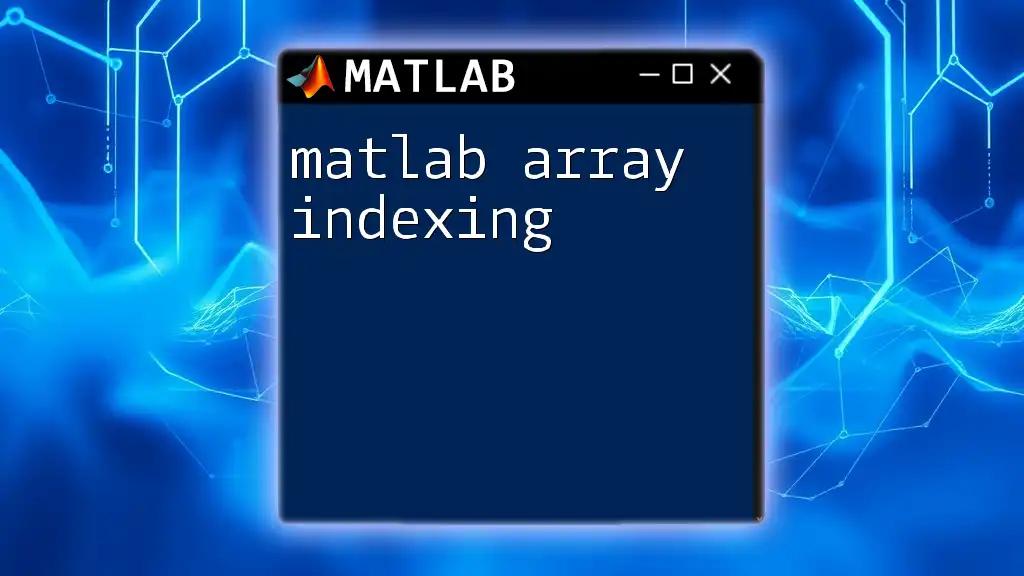
Conclusion
In summary, transforming your histogram's Y-axis to display percentages instead of raw frequencies using MATLAB enhances data interpretation, improves comparisons, and boosts presentation quality. By utilizing the `histogram` function and customizing your visualizations effectively, you can convey significant insights and results from your data.
For those eager to deepen their MATLAB skills, continuing to experiment with histograms and other visualization techniques will provide immense value in both analysis and presentation. Join our community for more tips on using MATLAB effectively!
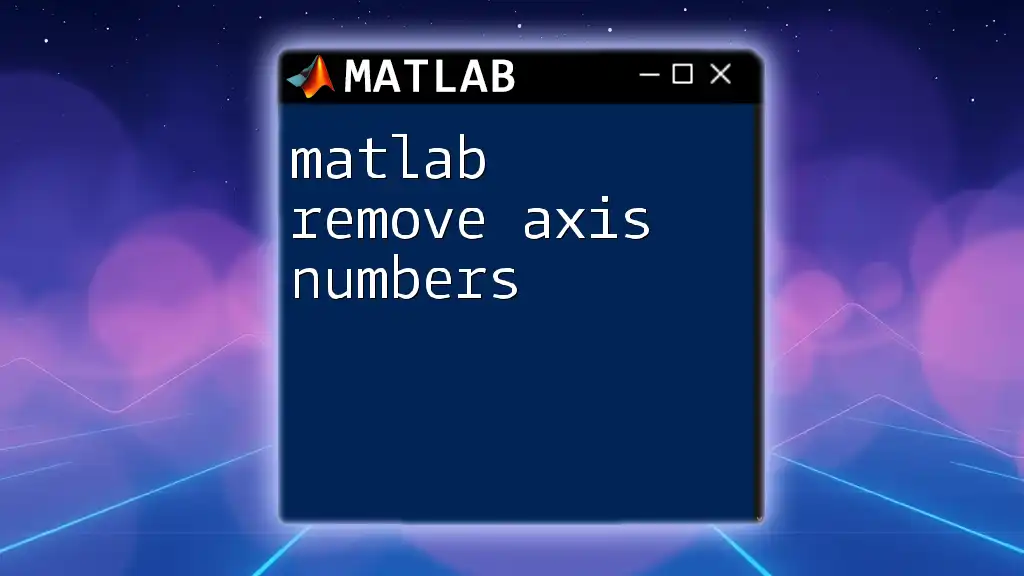
Further Reading Resources
For more information on MATLAB histogram functions, check the official MATLAB documentation and explore other tutorials that dive into advanced data visualization techniques.