Matlab array indexing allows you to access and manipulate elements of an array using their row and column positions, enabling efficient data handling.
% Example of indexing in a 2D array
A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % Create a 3x3 array
element = A(2, 3); % Access the element in 2nd row, 3rd column (value is 6)
What is Array Indexing?
MATLAB array indexing refers to the method of accessing and manipulating elements within an array using their position or index. Indexing allows users to retrieve specific items, modify them, or extract subsets of data from arrays. Understanding how to efficiently index arrays is crucial in MATLAB, especially when working with large datasets or conducting numerical analyses.
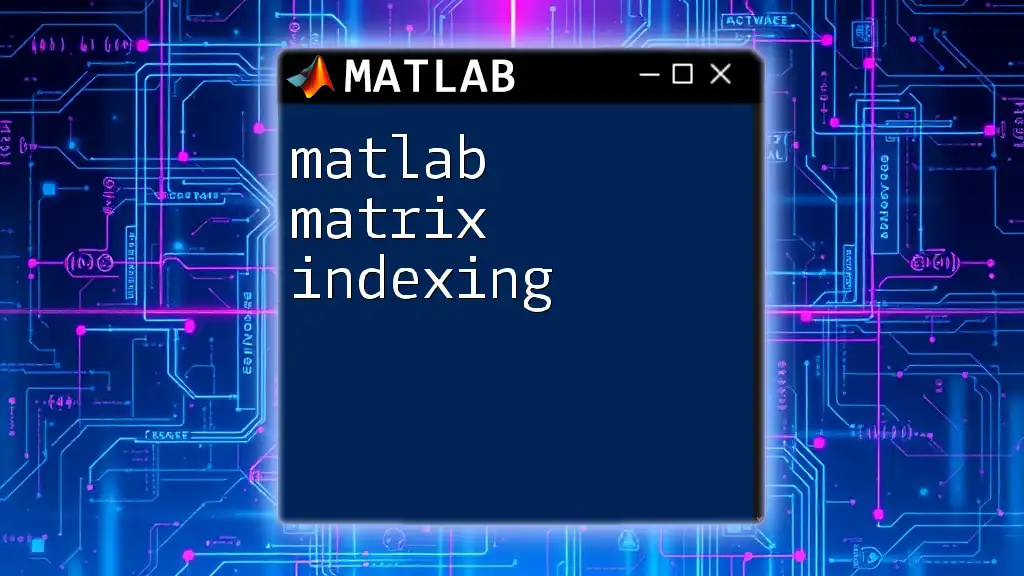
Why is Indexing Important?
Indexing plays a vital role in data manipulation and retrieval. It allows users to pinpoint specific elements or a group of elements without having to process the entire dataset. For instance, in scientific computing and engineering applications, practitioners often work with matrices and need precise data points for calculations and simulations. Efficient indexing can lead to more streamlined code, facilitating both readability and speed when working with MATLAB scripts.
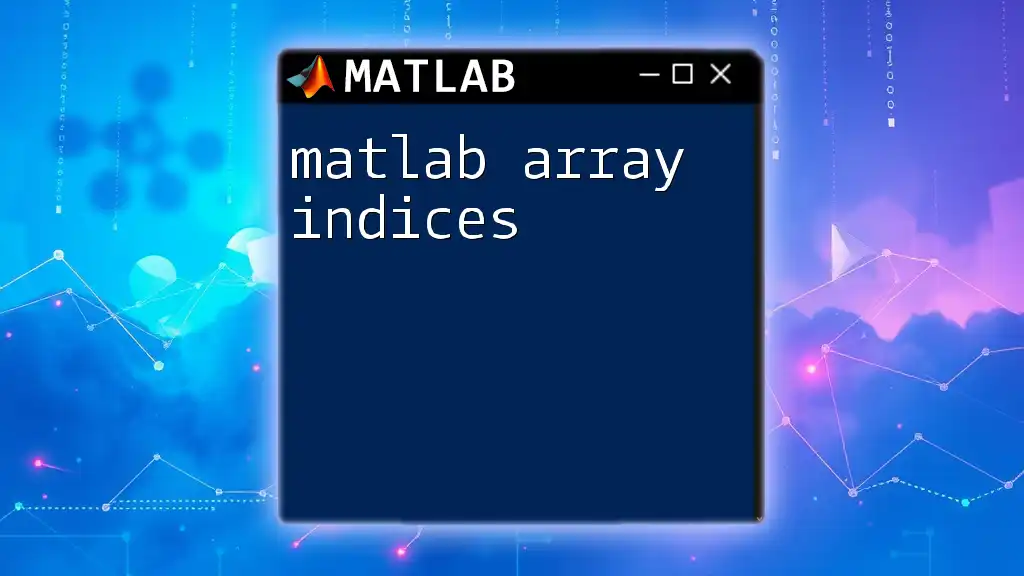
Understanding Array Basics
Types of Arrays in MATLAB
Numerical Arrays: These are the most commonly used arrays in MATLAB. They can be one-dimensional (vectors), two-dimensional (matrices), or multi-dimensional arrays. For example, you can create a 2D matrix with the command:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
Cell Arrays: These arrays can hold different types of data, including numbers, strings, or even other arrays, each in separate cells. A cell array can be initialized with the following syntax:
C = {1, 'MATLAB', [1, 2, 3]};
Struct Arrays: Structured arrays can store data of varying types, with fields that are accessible using a dot notation. Here is an example of creating a struct array:
S(1).name = 'John';
S(1).age = 28;
S(2).name = 'Anna';
S(2).age = 24;
Creating Arrays
Creating Numeric Arrays: MATLAB provides several functions to create arrays quickly. For instance, you can initialize arrays with zeros, ones, and random values effortlessly:
A = zeros(3, 3); % 3x3 matrix of zeros
B = ones(2, 2); % 2x2 matrix of ones
C = rand(4); % 4x4 matrix with random values
Creating Cell and Struct Arrays: You can easily create cell and struct arrays using curly braces and dot notation, as shown previously.
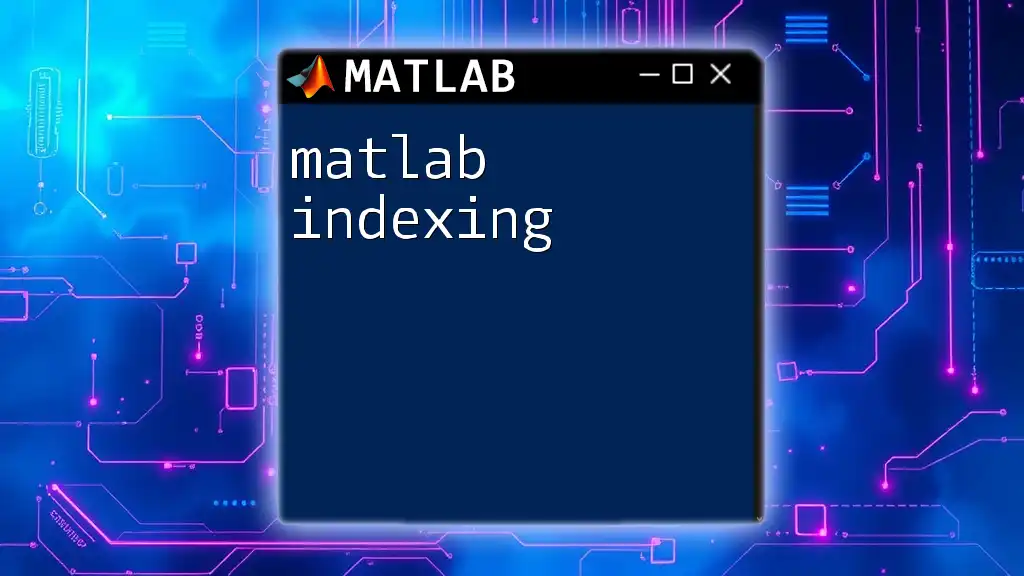
Array Indexing Syntax
Basic Indexing
Accessing Elements: To access individual elements of an array, you can use the syntax `array(row, column)`. For example, to access the element in the 2nd row and 3rd column of matrix A:
value = A(2, 3);
Accessing Rows and Columns: You can also retrieve entire rows or columns by leaving a section of the index empty. To access the 1st row of A:
first_row = A(1, :); % All columns in the first row
To get the 2nd column:
second_column = A(:, 2); % All rows in the second column
Advanced Indexing Techniques
Logical Indexing
Introduction to Logical Indexing: Logical indexing involves accessing array elements that satisfy a given condition. This technique is particularly useful for filtering data.
Using Logical Conditions to Index: For example, to find all elements in a matrix greater than 5, you can use:
greater_than_five = A(A > 5);
This returns an array of elements in A that are greater than 5.
Linear Indexing
Explaining Linear Indexing: Linear indexing allows you to access elements of an array as if it were a one-dimensional array, making it easy to work with multidimensional arrays.
Examples with 1D and 2D arrays: For a matrix, the indices are counted column-wise. For example, using the matrix A defined earlier, you can access the element in the 2nd row and 3rd column (6) using:
element = A(6);
This retrieves the 6th element in a linear index.
Concatenation and Indexing
Combining Arrays: You can concatenate arrays using the `cat` function or by directly using square brackets. After concatenation, you can still access elements using the same indexing techniques.
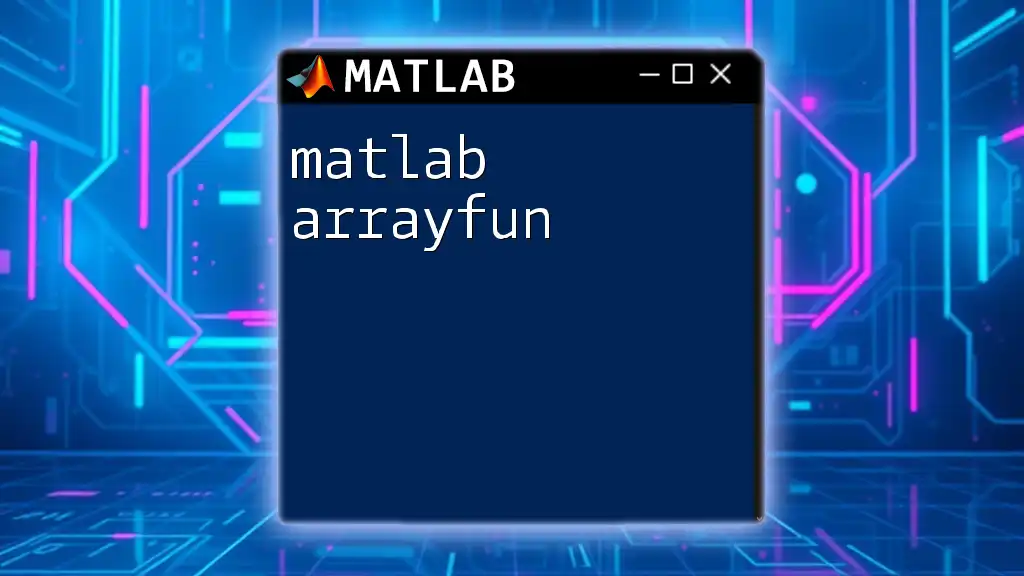
Slicing and Reshaping
Slicing Arrays
Extracting Subarrays: Slicing allows you to obtain a defined subset of an array. For example, to extract a 2x2 submatrix from A:
submatrix = A(1:2, 2:3); % Gets the top left 2x2 section starting from row 1, column 2
Reshaping as an Indexing Technique
Using `reshape` Function: You can change the dimensions of an array while keeping the total number of elements the same using the `reshape` function:
B = reshape(A, 3, 3); % Reshapes A into a 3x3 matrix
This allows you to apply different indexing methods on resized arrays.
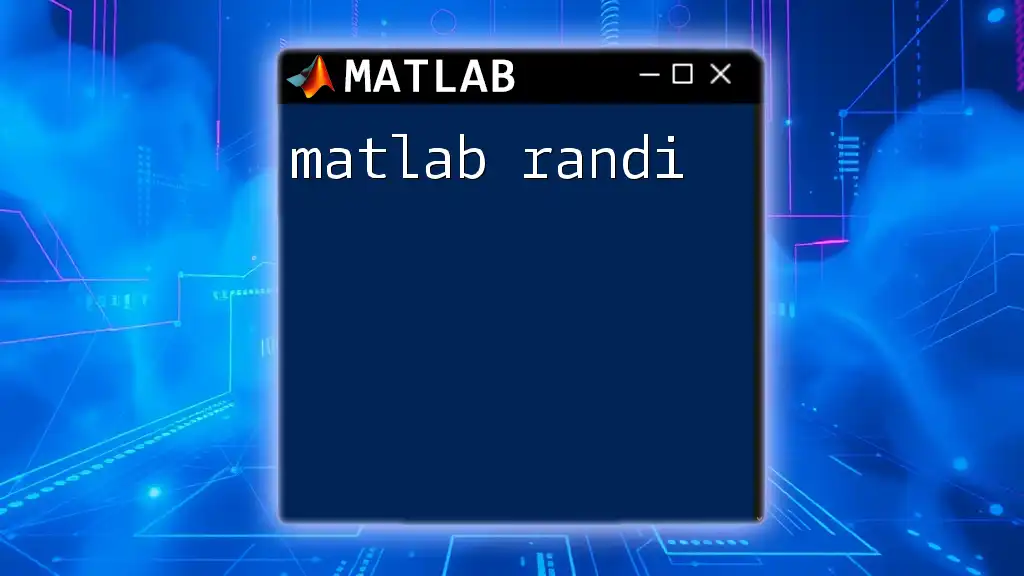
Special Indexing Cases
Indexing with `end`
Utilizing the `end` Keyword: The `end` keyword can be extremely handy in accessing the last element of an array. For example:
last_element = A(end); % Retrieves the last element of a linear index
last_row = A(end, :); % Retrieves the last complete row
Indexing in Multi-dimensional Arrays
Accessing Elements in Multi-dimensional Arrays: When dealing with more than two dimensions, you can still use typical indexing techniques, extending the usage of commas. For instance, given a 3D array:
D = rand(4, 4, 4);
element = D(2, 3, 4); % Accesses element in the 2nd slice, 3rd row, and 4th column
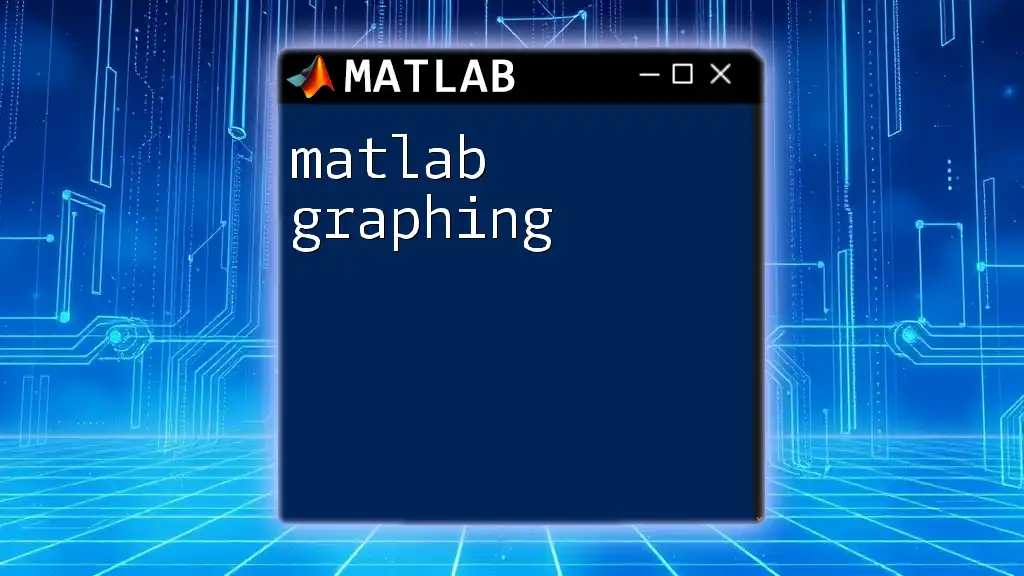
Common Indexing Errors
Mistakes and How to Avoid Them
Index Out of Bounds: One common error encountered in MATLAB is attempting to access elements outside the array bounds, leading to an "Index exceeds matrix dimensions" error. For example, if A is a 3x3 matrix, attempting to access `A(4, 2)` will fail.
Scalar vs. Vector Indexing Confusion: New users often confuse how MATLAB treats scalar and vector indexing, especially when the output is not as expected. Ensuring you know whether you want a single value or a subarray is crucial in avoiding this error.
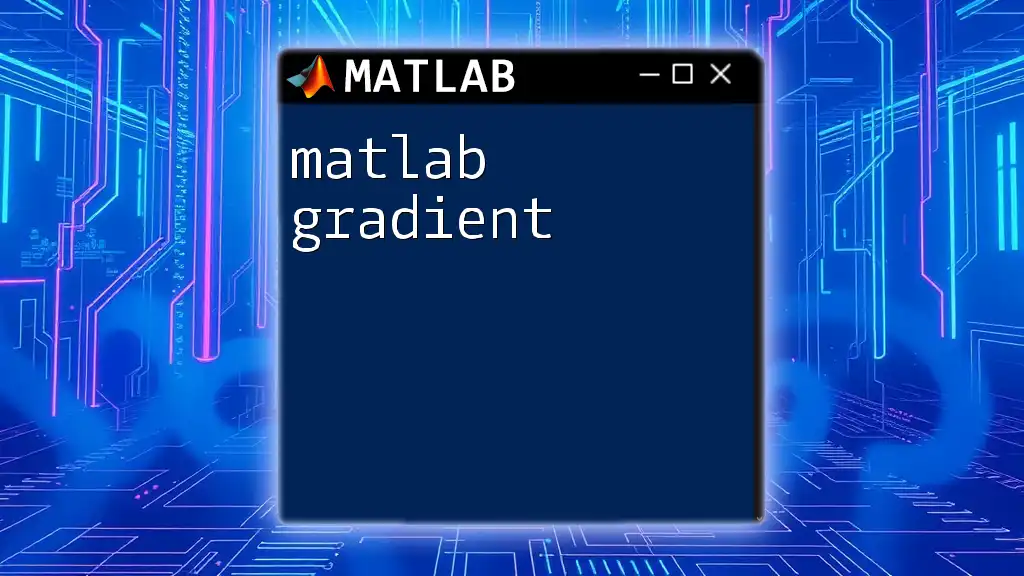
Best Practices for Array Indexing
Efficient Indexing Techniques
Vectorization: Embracing vectorized operations allows MATLAB to optimize performance significantly. Instead of using loops to manipulate array data, applying operations directly to arrays (like matrix multiplication or element-wise operations) is preferred.
Readability in Code
Writing Clean and Readable Code: Clear indexing enhances code readability. Using meaningful variable names and consistent indexing methods improves the maintainability of your scripts. Avoid overly complex indexing expressions that may confuse the reader.
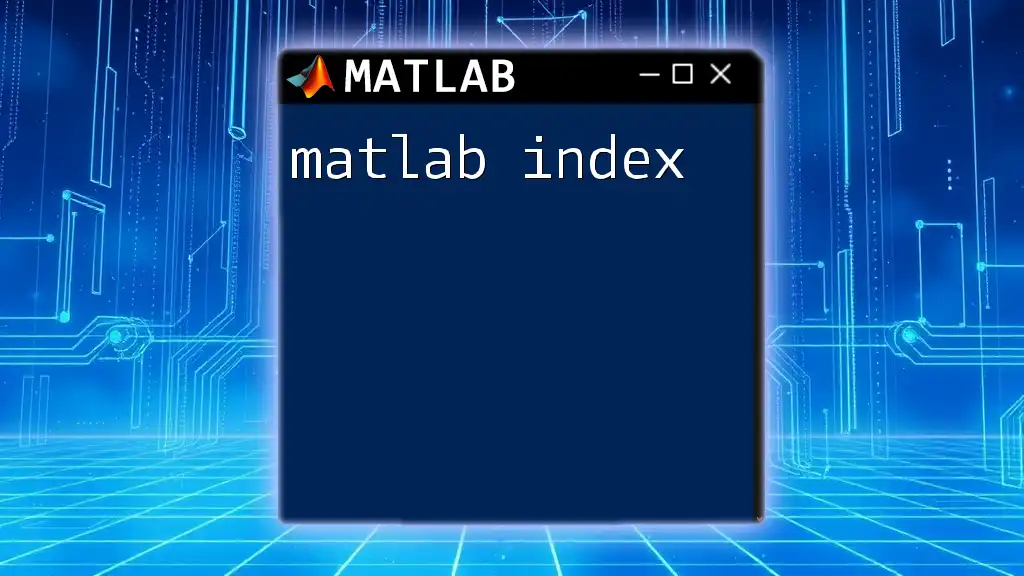
Conclusion
In summary, MATLAB array indexing is a powerful tool that greatly enhances your ability to manipulate and analyze data efficiently. By mastering both basic and advanced indexing techniques, you can leverage MATLAB's full potential and streamline your workflows.
It’s crucial to practice these concepts regularly to build proficiency. Experiment with different indexing methods, and challenge yourself with various datasets to solidify your understanding.
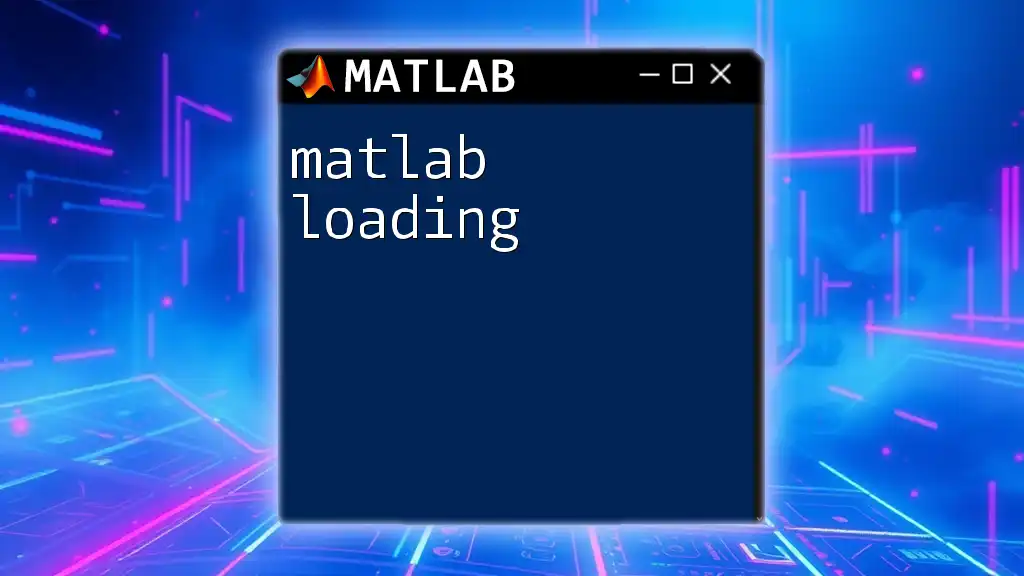
Additional Resources
To further enhance your understanding of MATLAB array indexing, refer to the official documentation and online tutorials. Engage with practice problems to reinforce your skills and discover innovative indexing techniques that can optimize your MATLAB experience.