"MATLAB programming online offers a convenient way to learn and practice essential MATLAB commands and techniques through interactive platforms and resources."
Here's a simple code snippet to demonstrate a basic MATLAB command that creates a plot:
x = 0:0.1:10; % Define the range of x from 0 to 10 with increments of 0.1
y = sin(x); % Calculate the sine of each value in x
plot(x, y); % Create a plot of y vs. x
title('Sine Wave'); % Add a title to the plot
xlabel('X-axis'); % Label the x-axis
ylabel('Y-axis'); % Label the y-axis
grid on; % Turn on the grid for better visualization
Getting Started with MATLAB Online
Choosing the Right Online Platform
When embarking on your journey of MATLAB programming online, the first step is to select the right platform. Various platforms offer courses and resources tailored to MATLAB learners, including:
- Coursera: Offers a variety of courses created by top universities. You can find beginner to advanced topics covered here.
- edX: Similar to Coursera, it provides access to university-level courses, including specialized MATLAB programs.
- MATLAB Academy: An official site by MathWorks dedicated to providing comprehensive MATLAB training through interactive courses.
Each platform has its strengths—some provide a more structured learning path, while others offer flexibility in course selection. Compare their features, instructors, and user reviews to find the one that meets your needs.
Setting Up Your MATLAB Environment
Once you've chosen a platform, it’s time to set up your MATLAB Online environment.
-
Signing Up for MATLAB Online: Visit the MATLAB website and create an account. You might qualify for a free trial, or your educational institution may provide free access.
-
Overview of MATLAB Online Features: MATLAB Online allows you to write code directly in your web browser without software installation. Explore the key features, such as the Command Window, the Workspace, and the Editor.
-
Basic User Interface Walkthrough: Familiarize yourself with the interface, focusing on how to create scripts and run commands. Practice using the Command Window to execute simple commands.

Understanding MATLAB Commands
Basic MATLAB Syntax
A solid grasp of MATLAB syntax is crucial for efficient programming. Understanding how to format and organize your code will enhance clarity and maintainability.
When writing MATLAB code, always remember to use comments. Comments in MATLAB start with a `%` symbol and help explain what your code does.
Example: Here’s a simple script to display "Hello, World!"
% Simple script to display "Hello, World!"
disp('Hello, World!')
Executing this code will output "Hello, World!" in the Command Window, helping you verify that your environment is working correctly.
Common Commands and Their Usage
MATLAB offers a vast array of commands and functions. Here are some basic commands for arithmetic operations and variable management:
Arithmetic Operations
MATLAB allows you to perform arithmetic calculations easily.
Example: Performing calculations with variables.
a = 5;
b = 3;
c = a + b;
disp(c) % Output: 8
In this example, we assigned values to variables `a` and `b`, added them, and displayed the result.
Variable Creation and Assignment
Creating variables is straightforward in MATLAB. You can assign various data types, such as strings, numbers, and arrays.
Example: Creating and using different data types.
str = 'MATLAB'; % String
num = 42; % Integer
arr = [1, 2, 3]; % Array
By storing data in variables, you make your code reusable and organized.
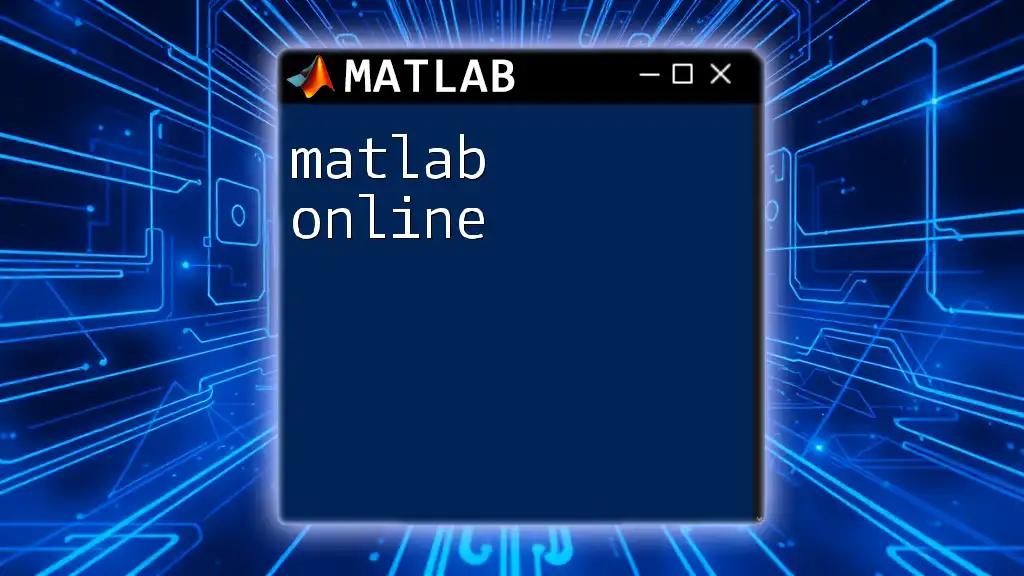
Learning Advanced MATLAB Programming
MATLAB Functions
Function programming is vital for structuring your code. Functions let you encapsulate logic that can be reused multiple times, making your code modular.
Example: Writing a simple function to calculate the square of a number.
function result = squareNumber(x)
result = x^2;
end
In this function, you can call `squareNumber(4)` to get the result of `16`. The importance of functions is amplifying as your projects become more complex, allowing easier debugging and clarity.
Control Flow in MATLAB
Control flow structures enable your program to execute commands conditionally and iteratively.
Using Conditional Statements
MATLAB supports `if` and `else` statements for decision-making processes.
Example: Using conditional statements.
if a > b
disp('a is greater than b');
else
disp('b is greater than or equal to a');
end
Here, the program checks the condition and displays a message based on the outcome.
Looping Structures
Loops are crucial for executing repetitive tasks. `for` and `while` loops are commonly used in MATLAB to iterate over arrays or run a block of code multiple times.
Example: Displaying numbers using a `for` loop.
for i = 1:5
disp(i);
end
This loop will display numbers from 1 to 5 in the Command Window.
Working with Matrices and Arrays
Matrices are a fundamental part of MATLAB. You can easily perform various operations with matrices, which is essential for mathematical computations and data analysis.
Example: Adding and multiplying matrices.
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
C = A + B; % Matrix addition
D = A * B; % Matrix multiplication
The ability to manipulate matrices efficiently is a key reason why MATLAB is widely used in engineering and scientific applications.
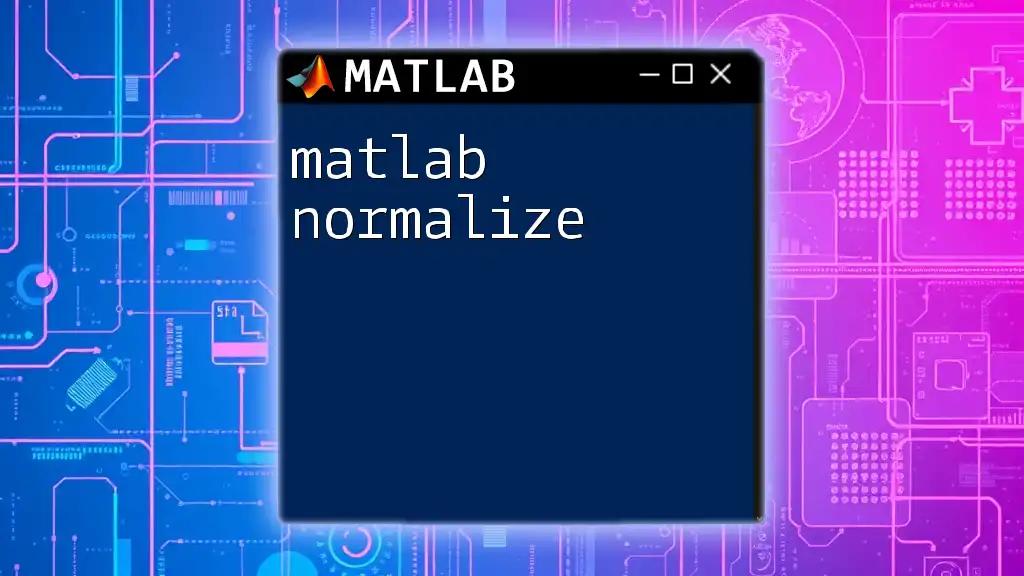
Resources for Learning MATLAB Online
Free and Paid Courses
Many resources are available for learning MATLAB programming online. Take advantage of both free and paid options based on your learning preferences.
-
Free Resources: You can find several free introductory courses on platforms like YouTube. Some other websites also offer free MATLAB tutorials and documentation.
-
Best Paid Courses: If you’re looking for structured learning, consider paid courses on Coursera and edX, which provide more comprehensive and interactive learning experiences.
Engaging with the MATLAB Community
Joining forums, discussion groups, or even social media communities will enrich your learning experience. Engaging with fellow learners allows you to ask questions, share your experiences, and gain insight from others.
Explore platforms like the MATLAB Central community where users often share experience, coding tips, and troubleshooting advice.
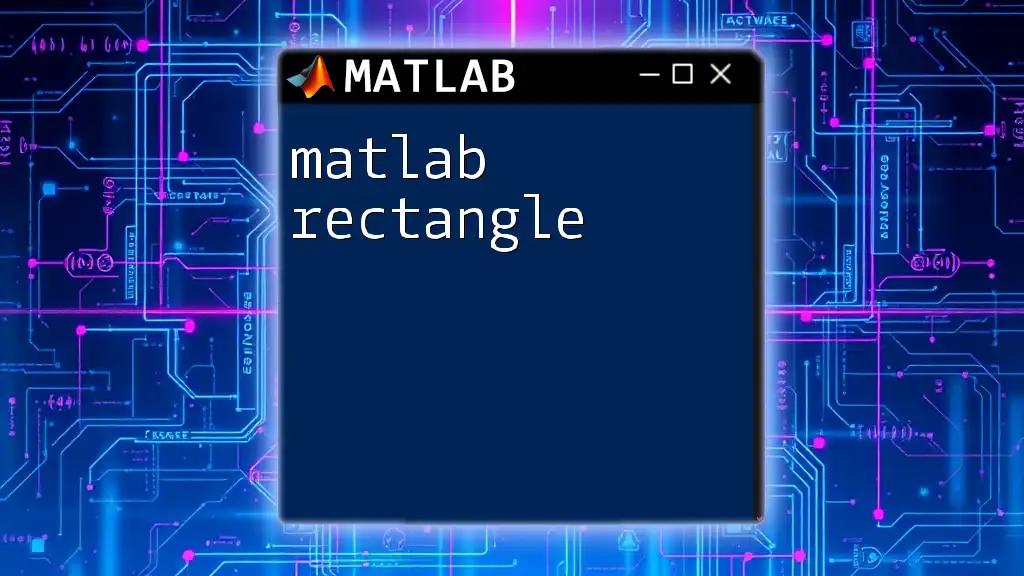
Tips for Effective Online Learning
Creating a Study Plan
To maximize the effectiveness of your online learning, it’s essential to create a structured study plan. Outline what you want to learn weekly and set aside dedicated time for practice.
Practicing Regularly
Hands-on experience is key to mastering MATLAB. Make it a habit to solve problems, engage in self-directed projects, or complete assignments from your courses. The more you practice, the more confident you’ll become in your programming skills.

Conclusion
In summary, learning MATLAB programming online offers an accessible and flexible means to acquire valuable programming skills. Whether you aspire to work in engineering, data analysis, or scientific computing, MATLAB is a powerful tool in your arsenal. With the resources, techniques, and practices discussed in this guide, you are well-equipped to begin your MATLAB journey.

Call to Action
Are you ready to dive deeper into the world of MATLAB? Join our dedicated MATLAB programming course today to receive expert guidance and further enhance your programming skills!