"MATLAB programming with MathWorks enables users to perform mathematical computations efficiently using a high-level language that supports matrix operations and data visualization."
Here’s a simple code snippet that demonstrates creating a matrix and calculating its determinant:
A = [1, 2; 3, 4]; % Define a 2x2 matrix
detA = det(A); % Calculate the determinant of matrix A
disp(detA); % Display the result
Introduction to MATLAB Programming
What is MATLAB?
MATLAB, short for Matrix Laboratory, is a high-performance programming language and interactive environment primarily used for numerical computing, data analysis, algorithm development, and visualization. With its vast array of built-in functions and toolboxes, MATLAB serves as an essential tool for engineers, scientists, and mathematicians who need to manipulate matrices, visualize data in multiple dimensions, and solve complex mathematical problems.
Overview of MathWorks
MathWorks, the official developer of MATLAB, is renowned for its innovation in computational software. Founded in 1984, MathWorks has consistently enhanced MATLAB and its companion products, catering to evolving needs in academia and industry. The comprehensive suite of tools provided by MathWorks ensures users can perform advanced calculations efficiently, streamlining workflows in data analysis.
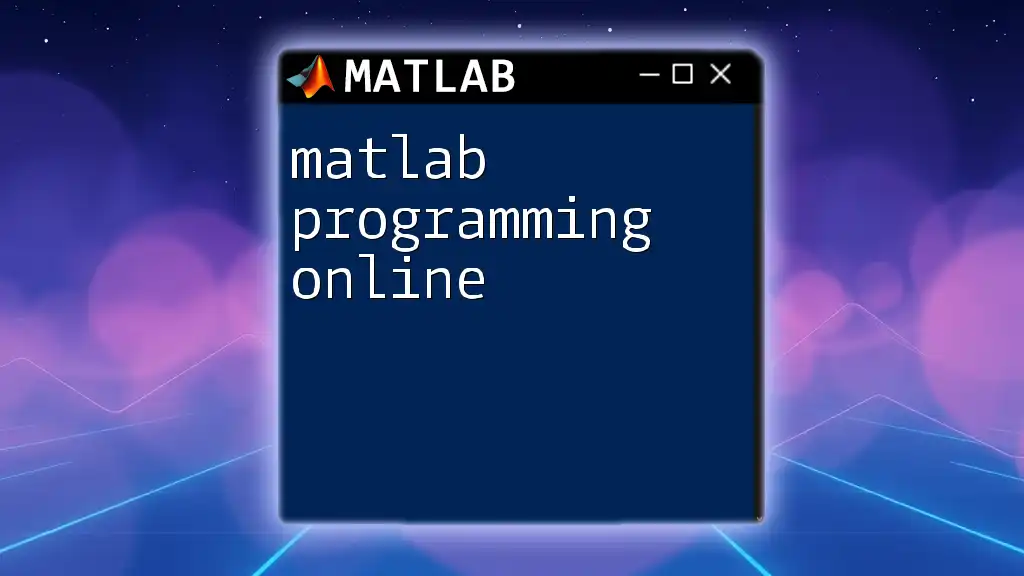
Setting Up MATLAB
Installing MATLAB
To get started with MATLAB programming, you first need to install the software. Depending on your operating system, you should follow the appropriate installation procedures. Visit the MathWorks website for the latest installation instructions and system requirements. Once installed, ensure you activate your license and familiarize yourself with available toolboxes that will best meet your programming needs.
Navigating the MATLAB Environment
Upon opening MATLAB, you'll encounter a user-friendly interface comprising several key components: the Command Window for executing commands; the Workspace, which displays variables; and the Editor, where you can write and debug scripts. Understanding how to navigate these components is crucial for effective programming. Customize your interface according to your workflow preferences to enhance productivity.
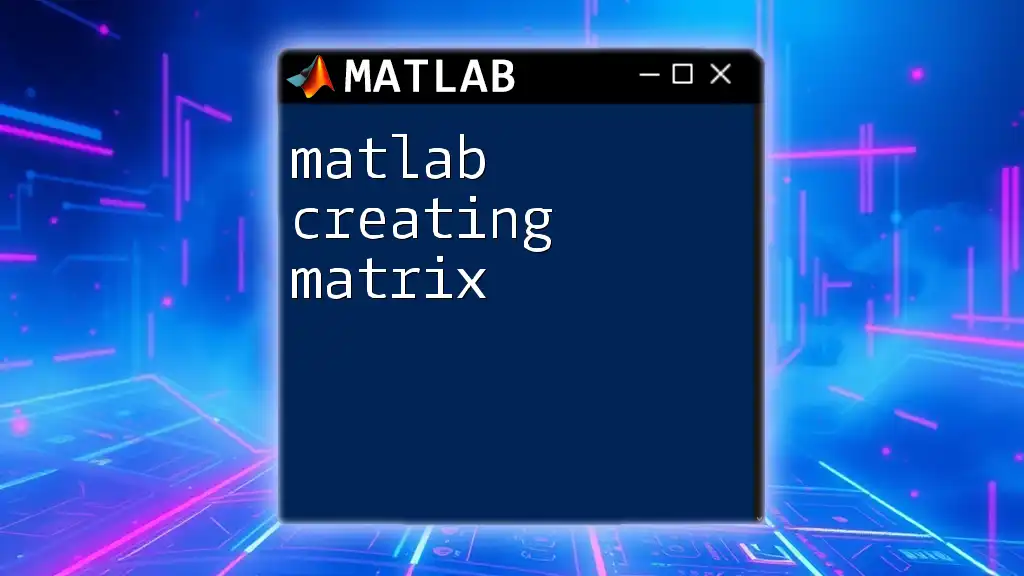
Basic MATLAB Commands
Understanding the Command Window
The Command Window allows users to interact directly with MATLAB. Here, you can type commands and see immediate results. To illustrate this, consider the following example that initializes a variable and displays its value:
x = 5;
disp(x);
In this instance, the variable `x` is assigned the value `5`, which is then displayed in the Command Window.
Using MATLAB as a Calculator
MATLAB’s power extends to its ability to perform various arithmetic operations seamlessly. You can use basic operators such as `+`, `-`, `*`, and `/`. When working with matrices and arrays, understanding how MATLAB handles these data structures is essential. For example, matrix addition can be executed as follows:
A = [1, 2; 3, 4];
B = [5, 6; 7, 8];
C = A + B; % Matrix addition
This creates two matrices, `A` and `B`, and sums them to produce a new matrix `C`.
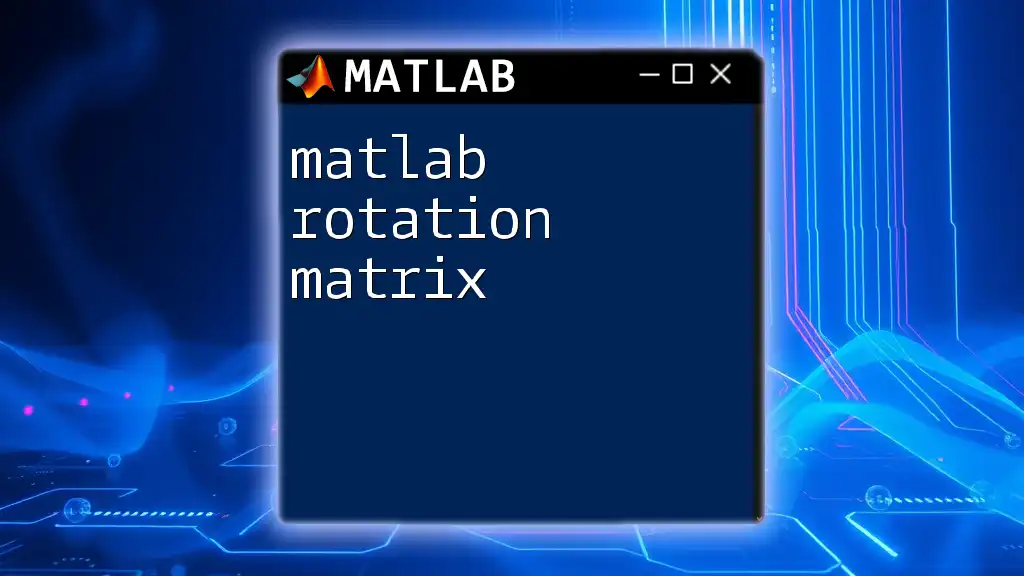
Variables and Data Types in MATLAB
Defining Variables
In MATLAB, defining variables is straightforward. Simply assign a value to a variable name, and MATLAB takes care of the rest. Variable names must begin with a letter and can be followed by letters, numbers, or underscores.
Data Types in MATLAB
Understanding data types is fundamental for effective MATLAB programming. Common data types include:
- Double: The default numeric type in MATLAB, representing floating-point numbers.
- Char: Used for character arrays or strings.
- Cell: A flexible type that allows storage of different data types.
- Struct: A data type that groups related data using named fields.
Familiarity with these data types ensures you can choose the right structure for your data, optimizing performance and memory usage.
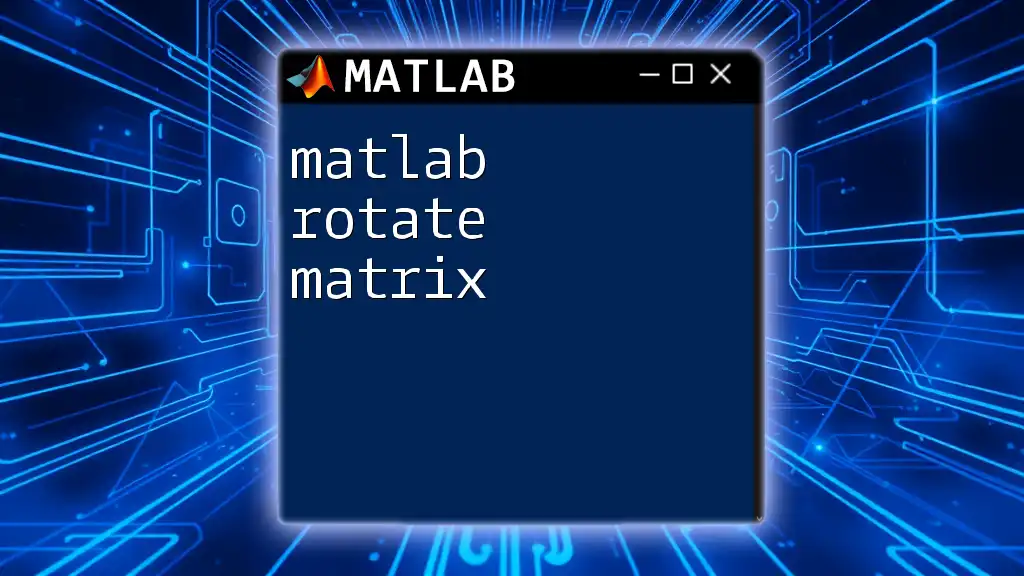
Control Structures in MATLAB
Conditional Statements
MATLAB allows for conditional execution of code using `if`, `else`, and `switch` constructs. Here's a simple example of how these statements can control the flow of a program:
if x > 10
disp('x is greater than 10');
else
disp('x is less than or equal to 10');
end
This checks the value of `x` and displays a message based on its value.
Loops in MATLAB
To execute sections of code multiple times, you can leverage loops. For instance, `for` loops are great for fixed iterations:
for i = 1:5
disp(i);
end
This loop outputs the numbers 1 through 5 to the Command Window.
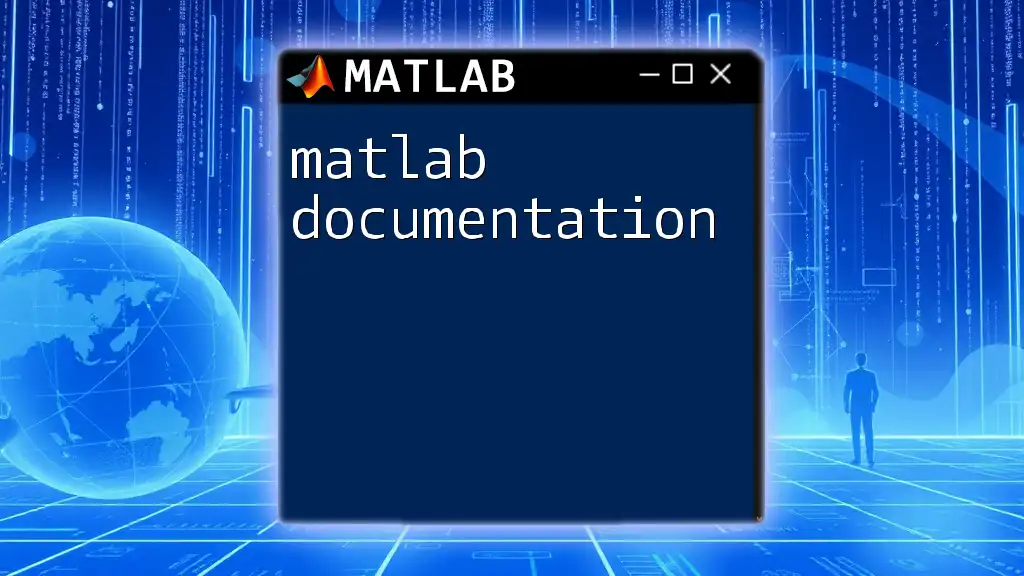
Functions and Scripts
Creating Functions
Functions in MATLAB enable you to encapsulate code that can be reused throughout your program. To define a function, use the `function` keyword, specify the output, input arguments, and the body of the function. For example:
function result = add(a, b)
result = a + b;
end
This function takes two arguments, `a` and `b`, and returns their sum.
Writing Scripts
Scripts are essentially collections of MATLAB commands stored in a `.m` file. Unlike functions, scripts do not accept input arguments. They are useful for automating tasks and executing sequences of commands. A simple script could include commands to load data, process it, and visualize results.
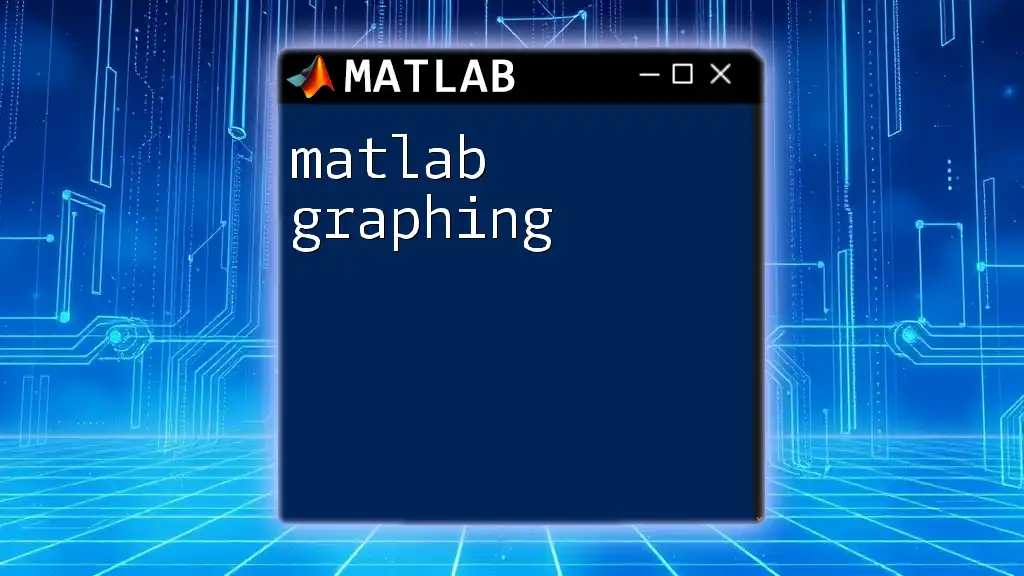
Data Visualization in MATLAB
Plotting Basics
MATLAB excels in data visualization, allowing you to create a variety of plots. The `plot` function creates 2D line plots with ease:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Sine Wave');
xlabel('X axis');
ylabel('Y axis');
This code generates a sine wave and labels the axes appropriately.
Advanced Plotting Techniques
You can further customize your plots by adding titles, legends, and annotations. Additionally, MATLAB supports 3D visualizations using functions such as `surf` and `mesh`, providing insights into complex datasets.
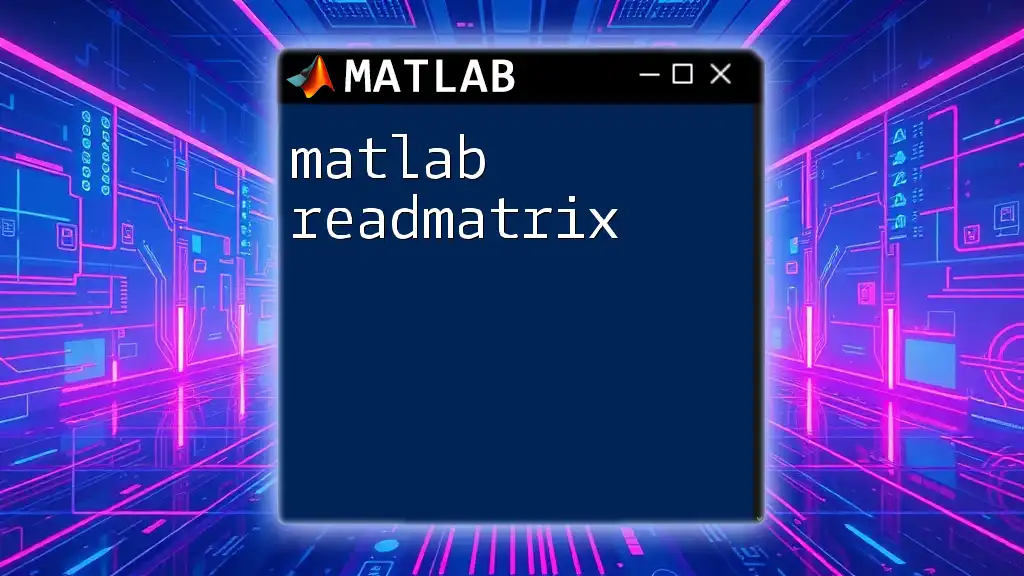
Importing and Exporting Data
Reading Data into MATLAB
MATLAB provides robust methods for importing various data formats. For instance, to read data from a CSV file, use the following command:
data = readtable('data.csv');
This command loads the data into a table, making it easy to manipulate and analyze.
Exporting Data from MATLAB
Once you have processed your data, exporting results is just as straightforward. To save your data back into a CSV file, you can use:
writetable(data, 'output.csv');
This allows you to share results or use them in other applications.
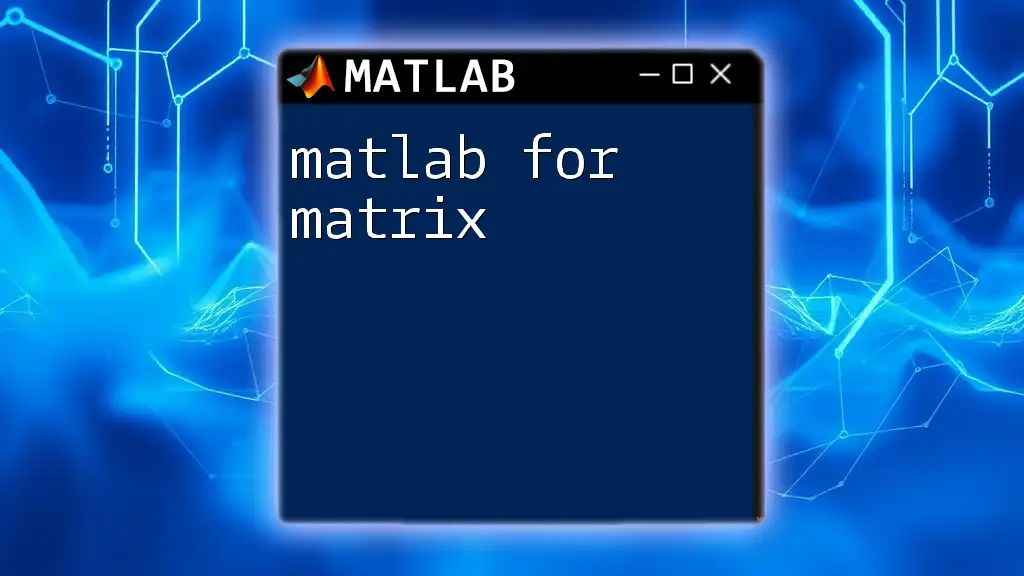
Troubleshooting and Debugging
Common Errors in MATLAB
As you program in MATLAB, understanding common pitfalls such as syntax errors or variable mismanagement is essential. Familiarize yourself with error messages MATLAB provides, as these often contain valuable clues for troubleshooting.
Using Debugging Tools
MATLAB includes debugging tools to assist in diagnosing and fixing issues. You can set breakpoints in your code to halt execution at specific lines and inspect variables, helping you understand where things may have gone wrong.
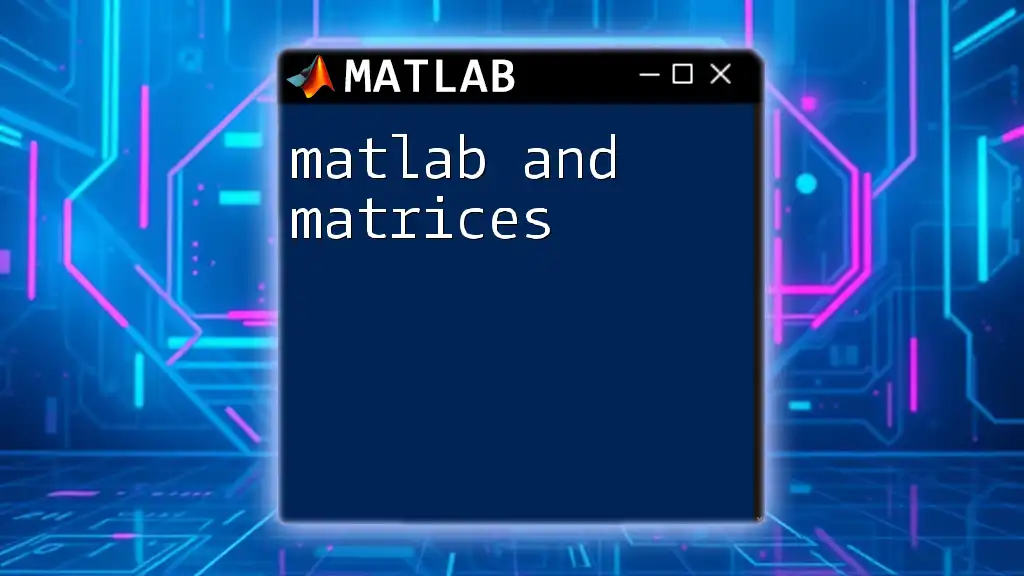
Resources for Learning MATLAB
Official MathWorks Documentation
The official documentation from MathWorks is a treasure trove of information, covering everything from basic functions to advanced topics. Regularly consulting the documentation can provide insights and answer specific questions that arise during programming.
Online Courses and Tutorials
Beyond the documentation, numerous online courses and tutorials exist, catering to various skill levels. Forums and community discussions can also provide support and knowledge-sharing opportunities, enhancing your learning experience.
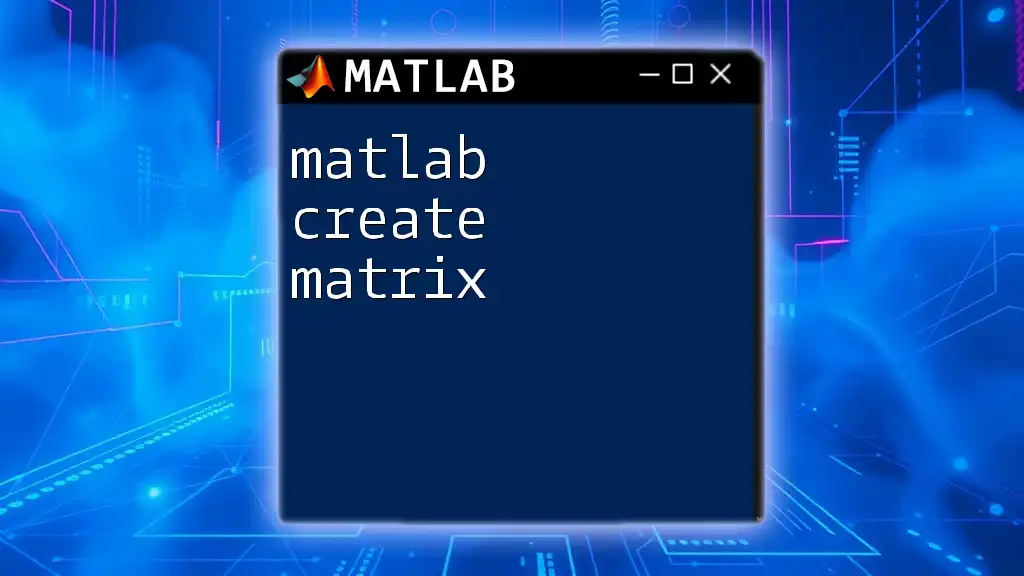
Conclusion
This guide offers a solid foundation in MATLAB programming and the capabilities provided by MathWorks. It is essential for you to experiment with the examples provided and apply the concepts to real-world scenarios, consolidating your understanding of this powerful tool.
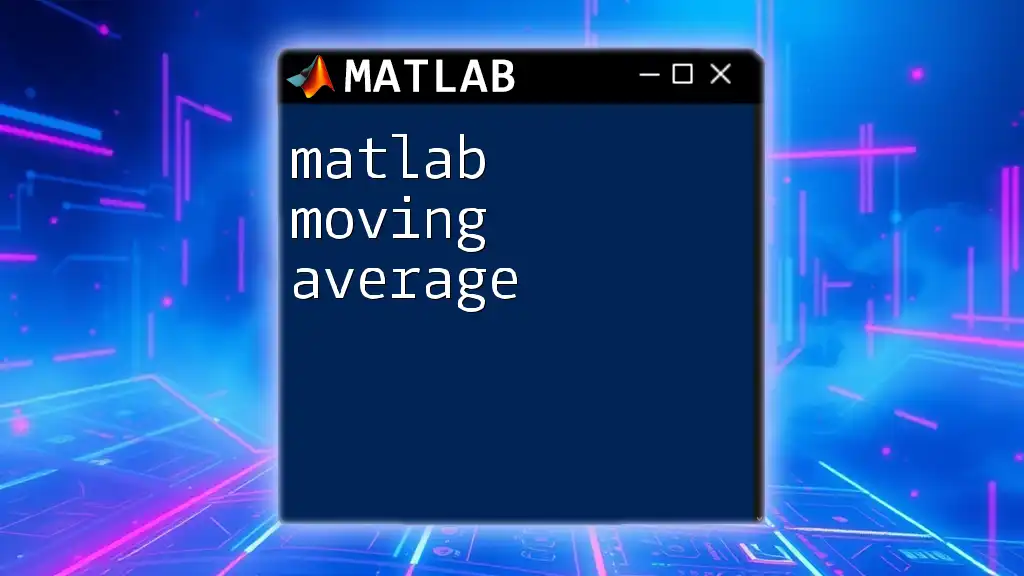
Call to Action
Start your journey with MATLAB today! Enroll in our comprehensive MATLAB course and gain the skills needed to excel in programming with MathWorks. Explore additional resources for further learning, and join the vibrant community of MATLAB users ready to share knowledge and help each other grow.