In MATLAB, you can customize the intervals of the x-axis and y-axis on a plot using the `xticks` and `yticks` functions to specify the desired tick marks. Here's a code snippet demonstrating this:
% Sample data
x = 0:0.1:10;
y = sin(x);
% Create the plot
plot(x, y);
% Set x-axis and y-axis intervals
xticks(0:1:10);
yticks(-1:0.5:1);
Understanding Plot Axes in MATLAB
What are Plot Axes?
Plot axes serve as the framework for visualizing data in MATLAB. The X-axis typically represents the independent variable, while the Y-axis represents the dependent variable. Understanding how these axes function is crucial for properly interpreting data trends, correlations, and patterns.
Default Settings vs. Custom Settings
When creating plots, MATLAB automatically determines default axis intervals based on the data provided. While this can be convenient, relying solely on default settings may lead to misinterpretations. Customizing these settings allows users to focus on specific data ranges, display relevant features clearly, and enhance overall readability.

Setting Up Your MATLAB Environment
Installing MATLAB
The first step in your journey with MATLAB is to acquire the software. You can obtain it through the official MathWorks website, where various licenses are available for individual, educational, or commercial users. Follow the installation instructions provided to set it up successfully on your system.
Opening a New Script
To start coding, open a new script by navigating to the Home tab and selecting "New Script." This will open a blank page where you can write and execute your MATLAB commands.
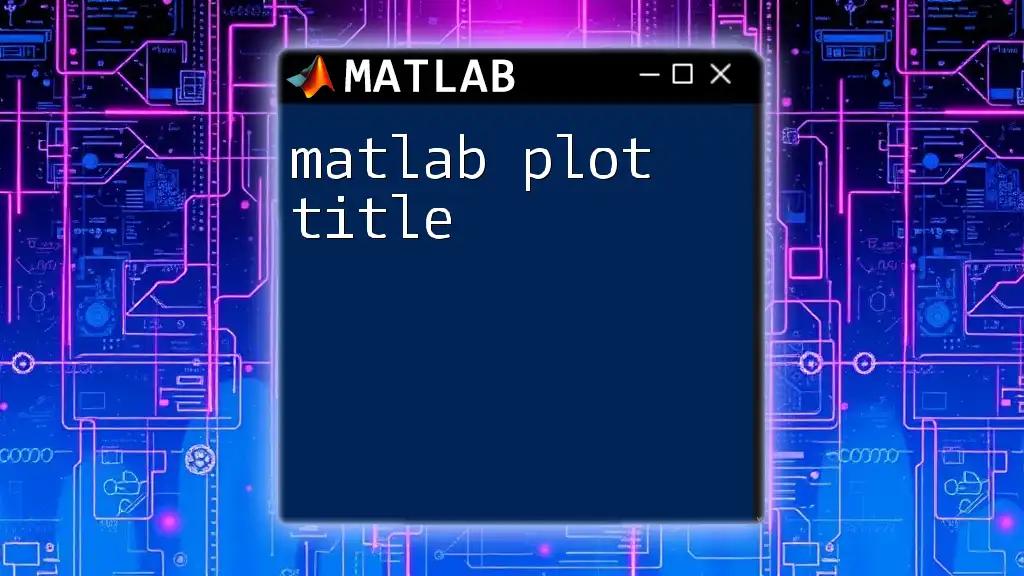
Basic MATLAB Plotting
Creating Simple Plots
A fundamental skill in MATLAB is creating basic plots. Begin by defining your data:
x = 0:0.1:10; % X data
y = sin(x); % Y data
plot(x, y);
title('Simple Sine Wave Plot');
xlabel('X-axis');
ylabel('Y-axis');
This script creates a sine wave plot ranging from 0 to 10 on the X-axis. It’s crucial to label your axes appropriately, as this improves the clarity of your plotted data.
Understanding Default Axes
By default, MATLAB sets axis limits based on the range and distribution of the plotted data. While this works for many scenarios, there are cases where the automatic settings do not effectively highlight important parts of your graph.
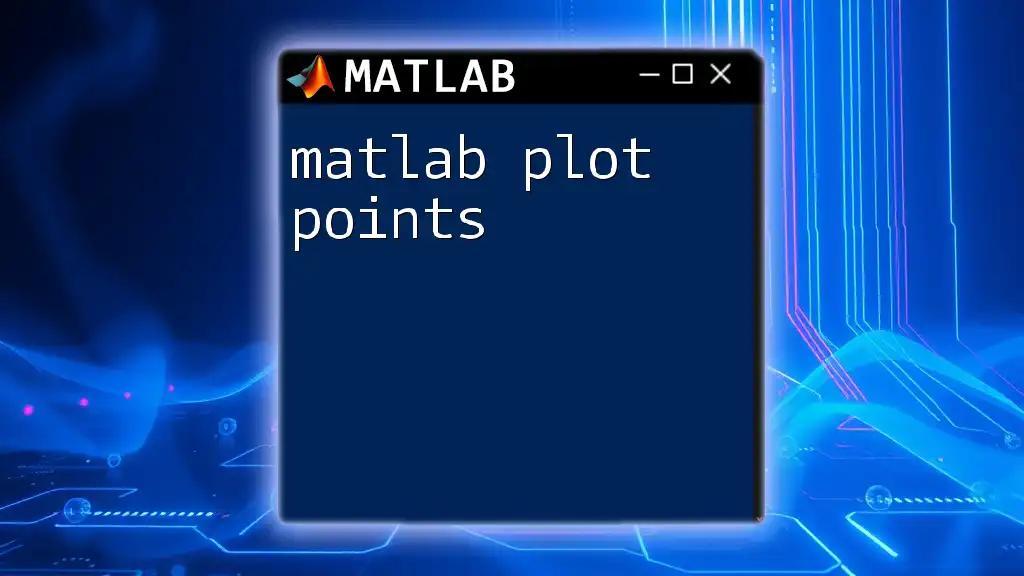
Customizing Axis Intervals
Why Customize Axis Intervals?
Customizing axis intervals enhances data interpretation and allows users to highlight specific data points or ranges. This feature is invaluable when dealing with complex datasets in scientific research or financial analysis, where certain trends or anomalies must be made clear.
Using `xlim` and `ylim`
To manually set the axes limits, you can use the `xlim` and `ylim` functions. For example:
xlim([0 10]); % Set x-axis limits from 0 to 10
ylim([-1 1]); % Set y-axis limits from -1 to 1
This code snippet specifies that the X-axis should only display values from 0 to 10 and the Y-axis from -1 to 1, effectively zooming in on the area of interest.
Using `set` Function
The `set` function provides another method for axis customization. This approach can enhance the clarity of your command:
ax = gca; % Get current axes
set(ax, 'XLim', [0 10], 'YLim', [-1 1]);
This snippet retrieves the current axes object and sets the X and Y limits simultaneously, giving you greater control over how your plot appears.
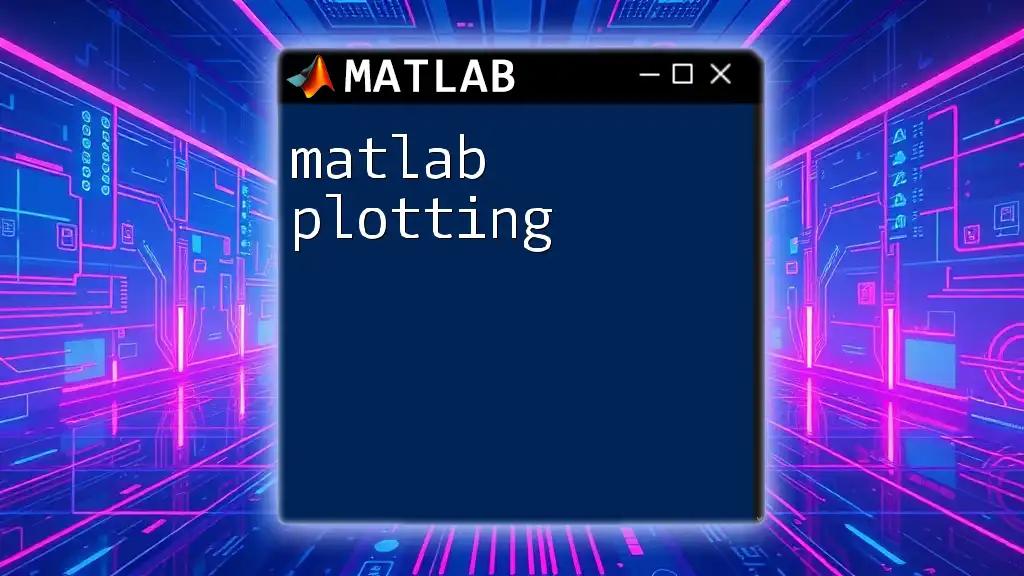
Advanced Customization Techniques
Custom Tick Marks with `xticks` and `yticks`
Customizing tick marks allows for precision in representing data intervals. You can define specific tick marks using the `xticks` and `yticks` functions:
xticks(0:1:10); % Set x-ticks at intervals of 1
yticks(-1:0.5:1); % Set y-ticks at intervals of 0.5
This example establishes a clear and concise interval structure for both axes, facilitating easier data interpretation.
Formatting Tick Labels
Formatting tick labels ensures that the displayed numeric values are easy to read and interpret. For example:
xticklabels({'0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '10'});
This command customizes the X-axis tick labels, enhancing readability and providing a clearer context for the plotted data.
Using `axis` Command
The `axis` command is a quick way to set both axes limits at once, offering a cleaner approach in your script:
axis([0 10 -1 1]); % Set limits for x and y axes simultaneously
This single line of code adjusts the limits for both axes, streamlining your coding process.
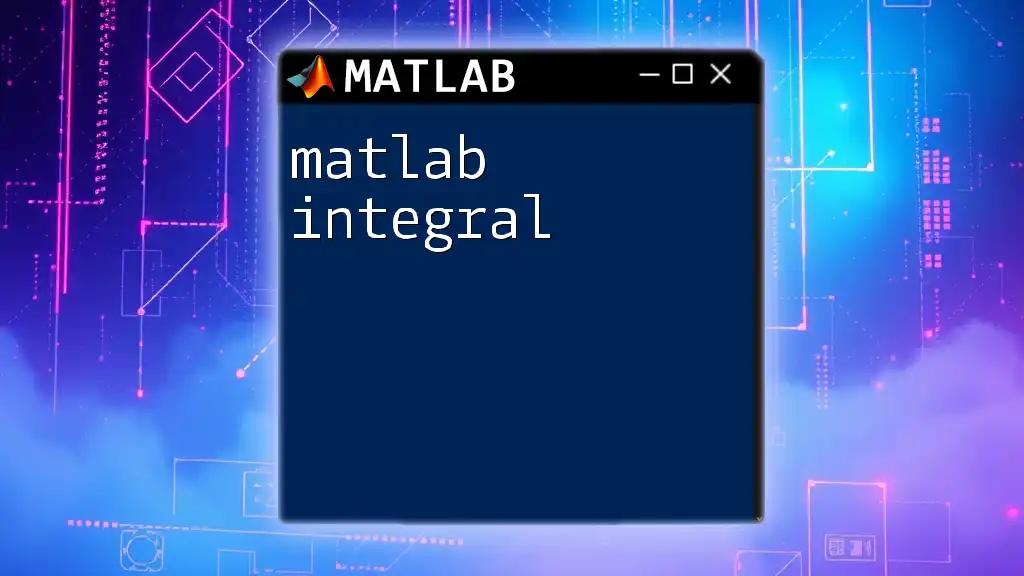
Example Use Cases
Case Study: Visualizing Scientific Data
In scientific applications, customized axis intervals can reveal trends and anomalies in data that default intervals might obscure. For instance, plotting experimental results with specific limits can better illustrate the phenomena being studied.
Case Study: Financial Data Representation
In finance, effective visualization is critical for making informed decisions. Customized axis intervals allow traders to focus on crucial ranges, such as price fluctuations within a narrow band, highlighting opportunities and risks more clearly.
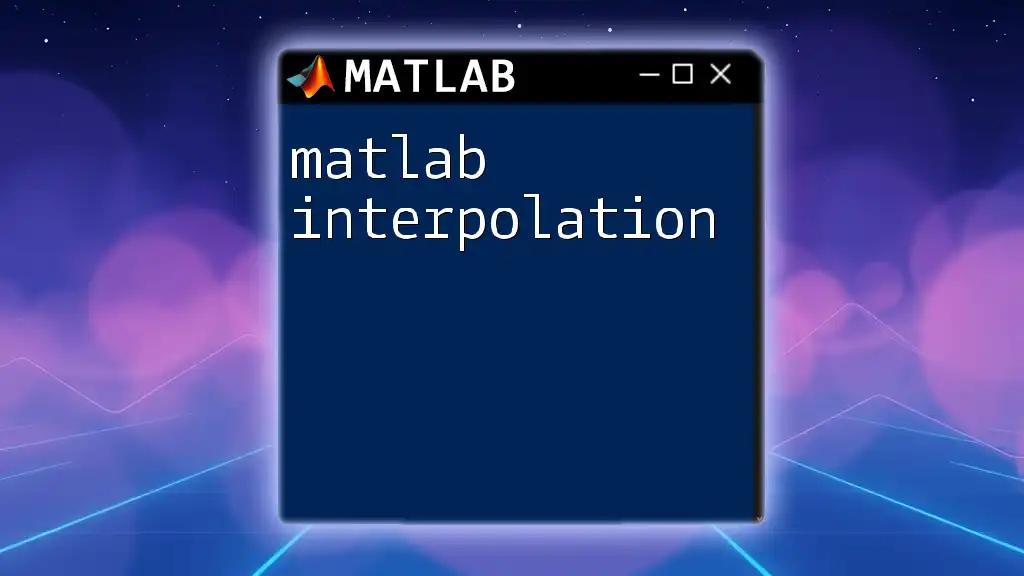
Tips and Best Practices
Keep It Simple
Avoid cluttering plots with excessive details. Strive for simplicity, ensuring that your plots effectively communicate the necessary information without overwhelming the viewer.
Consistency is Key
When presenting multiple plots, maintain consistent axis intervals and formatting. This promotes easier comparison and comprehension across visualizations, essential in reports and presentations.
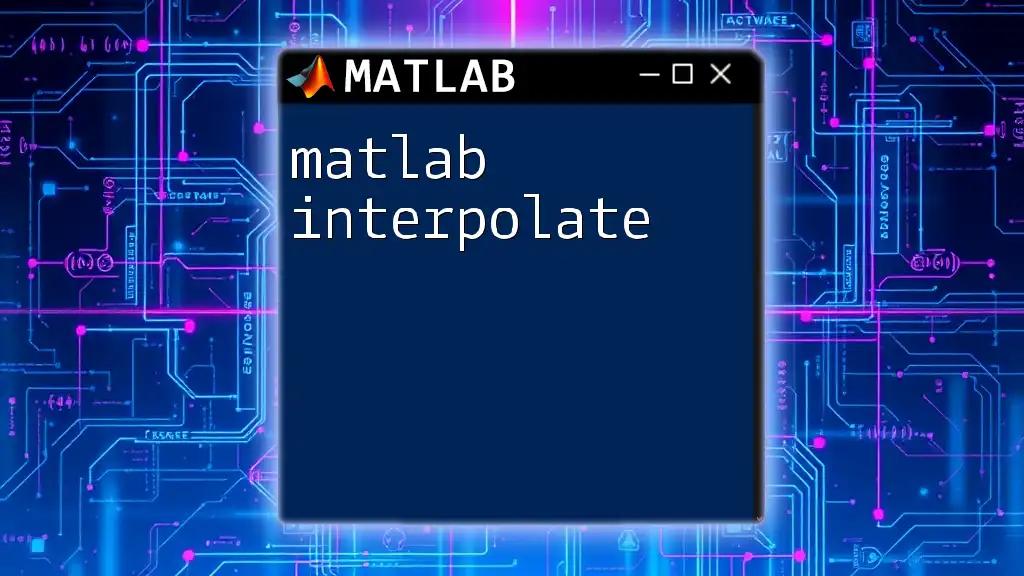
Troubleshooting Common Issues
Axis Limits Not Showing as Expected
If you encounter issues where axis limits do not align with what you set, ensure that you have not included extra commands that may override your settings. Also, check the data range to confirm that it falls within your specified limits.
Overlapping Tick Marks
To avoid overlapping tick marks, consider changing the intervals or formatting options. You can also adjust the font size of the tick labels for greater clarity.
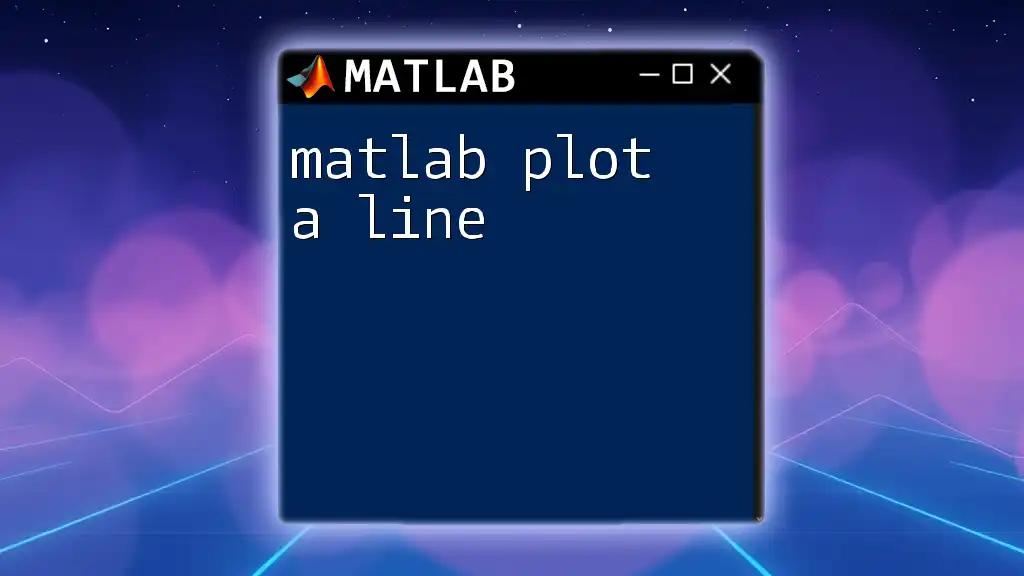
Conclusion
Customizing your MATLAB plot axis interval is a powerful way to enhance data representation. By utilizing functions like `xlim`, `ylim`, `xticks`, and `yticks`, you can unlock profound insights from your data, tailoring visualizations to convey the intended message clearly. With practice and implementation of the techniques discussed, you’ll become proficient in creating clear, effective visual representations of your data.