In MATLAB, the `min` function is used to find the smallest element in an array or to compare elements across multiple arrays, returning the minimum value or values along a specified dimension.
Here's a code snippet demonstrating its usage:
% Example array
A = [3, 5, 1; 7, 2, 6];
% Finding the minimum value in the array
minValue = min(A(:)); % Overall minimum
minRow = min(A, [], 2); % Minimum value per row
minCol = min(A, [], 1); % Minimum value per column
Understanding Minimum in MATLAB
Definition of Minimum
In the context of MATLAB and numerical analysis, the term minimum refers to the smallest value within a dataset or the lowest point of a mathematical function. This is crucial for various applications, particularly in fields such as optimization, statistics, and data analysis. A local minimum is defined as a point where a function value is lower than its neighboring points, while a global minimum is the absolute lowest value within the entire dataset.
Applications of Minimum
Finding the minimum value is prevalent in multiple scenarios including:
- Optimization problems: In various industries, optimizing resources, costs, or time often involves minimizing a specific function.
- Curve fitting: When fitting a model to data, the goal is often to minimize the difference between observed data points and the predictions of the model.
- Machine learning: Algorithms often seek to minimize error functions to improve model accuracy.

MATLAB Functions for Finding Minimum
`min` Function
MATLAB provides the `min` function, which is one of the simplest tools available for finding minimum values. The basic syntax is:
m = min(A)
This command returns the smallest element from array A. For example, consider the following code snippet:
A = [3, 5, 1, 7];
m = min(A); % Returns 1
This code finds the minimum value of the array, yielding 1.
Finding Minimum in Matrices
In cases involving matrices, MATLAB allows users to find minimum values across dimensions. The syntax for this is:
[m, idx] = min(A, [], 'dim')
Where 'dim' specifies the dimension along which the minimum is calculated. For example:
B = [4, 2, 8; 6, 5, 1];
m_row = min(B, [], 2); % Minimum of each row
m_col = min(B, [], 1); % Minimum of each column
This code calculates the minimum values for each row and each column of matrix B.
Using `fminunc` for Unconstrained Optimization
For more complex functions where you need to find a minimum without constraints, `fminunc` is the go-to function. The general syntax is:
[x, fval] = fminunc(fun, x0)
Here, fun is a function handle for the objective function, and x0 is the initial guess. For example, consider minimizing a simple quadratic function:
fun = @(x) (x-3).^2 + 5; % Function to minimize
x0 = 0; % Initial guess
[x, fval] = fminunc(fun, x0); % Finds the minimum
In this example, fminunc will find the value of x that minimizes the function, and the output fval will provide the minimum function value.
Using `fmincon` for Constrained Optimization
If your optimization problem includes constraints, you would utilize `fmincon`. The syntax for this function is:
[x, fval] = fmincon(fun, x0, A, b)
Where A and b represent linear inequality constraints. The following example illustrates its usage:
fun = @(x) (x(1)-1).^2 + (x(2)-2).^2; % Objective function
x0 = [0,0]; % Initial guess
A = []; b = []; % No linear inequality constraints
options = optimoptions('fmincon', 'Display', 'off');
[x, fval] = fmincon(fun,x0,A,b,[],[],[],[],[], options);
In this snippet, fmincon minimizes the two-dimensional quadratic function subject to given constraints.

Practical Tips for Finding Minimum in MATLAB
Setting Starting Values
Choosing an appropriate starting point for optimization functions like fminunc and fmincon is critical. A poor choice can lead to convergence on a local minimum rather than the global minimum. It is often beneficial to experiment with multiple starting points to get a reliable solution.
Visualizing the Function
Visual representation can facilitate understanding the function's behavior and its minimum. For instance, plotting a quadratic function can visually show its minimum:
x = linspace(-1, 5, 100);
y = (x - 3).^2 + 5;
plot(x, y); % To visualize the quadratic function
hold on;
plot(3, 5, 'ro'); % Plot the minimum point
title('Function Visualization');
xlabel('x-axis');
ylabel('y-axis');
This code snippet produces a graph that indicates the minimum value clearly, reinforcing the learning process.
Sensitivity Analysis
Conducting a sensitivity analysis helps understand how changes in parameters affect the minimum. This can be crucial in optimization problems where parameter shifts might lead to different outcomes. Using MATLAB, sensitivity analysis can be implemented through iterative evaluations of the function while varying the parameters systematically.
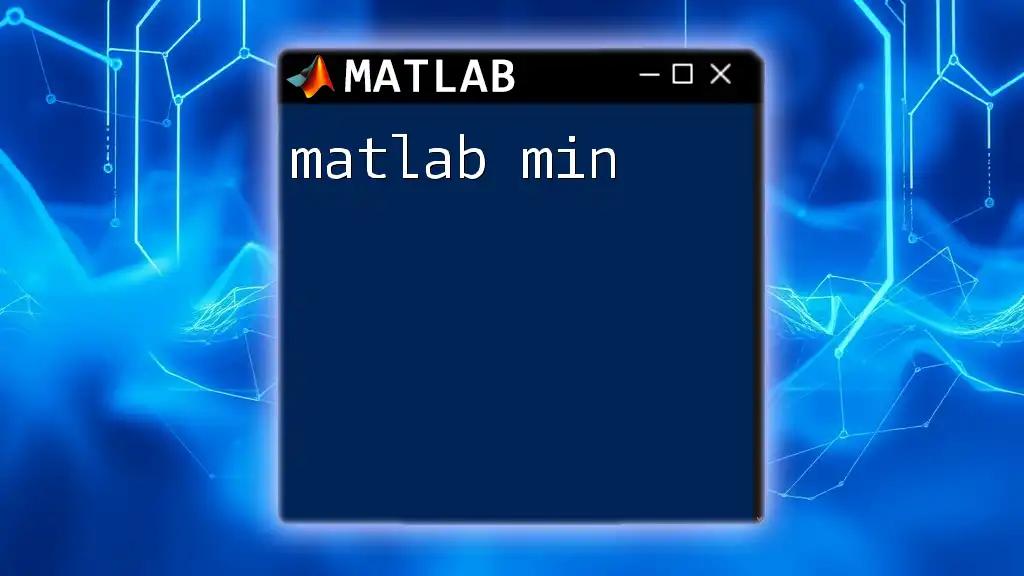
Conclusion
Finding the minimum in MATLAB is a fundamental skill that allows users to solve numerous practical problems efficiently. With functions such as min, fminunc, and fmincon, users can tackle both simple and complex optimization tasks. By practicing these techniques, you can enhance your analytical capabilities and apply them effectively across various fields.

Additional Resources
For further exploration, refer to the MATLAB documentation for the functions discussed. Expanding your knowledge through recommended books or online courses will deepen your understanding of optimization in MATLAB.

Call to Action
Join our courses today to gain hands-on experience and master MATLAB commands effectively, ensuring you can tackle any problem efficiently and elegantly.