The MATLAB `min` function returns the smallest element in an array or the smallest value among corresponding elements of two arrays.
% Example of using the min function
A = [3, 7, 2, 9, 4];
minValue = min(A); % Returns 2
Introduction to the `min` Function
The `min` function in MATLAB is a powerful tool for identifying the smallest values in arrays and matrices. Its primary function allows users to quickly assess numerical data, making it invaluable for tasks involving data analysis, optimization, and statistics. Understanding how to effectively use the `matlab min function` not only enhances programming efficiency but also aids in extracting critical insights from datasets.
Use Cases of the `min` Function
The applications of the `min` function are vast and can be seen in various fields. Here are some examples:
- Engineering: Engineers often need to identify the minimum stress or strain in materials to ensure safety and compliance.
- Data Science: In data preprocessing, finding the minimum of a dataset can help in normalizing the data, crucial for training machine learning algorithms.
- Finance: Financial analysts use the `min` function to determine the lowest price point of stocks over a specified period.
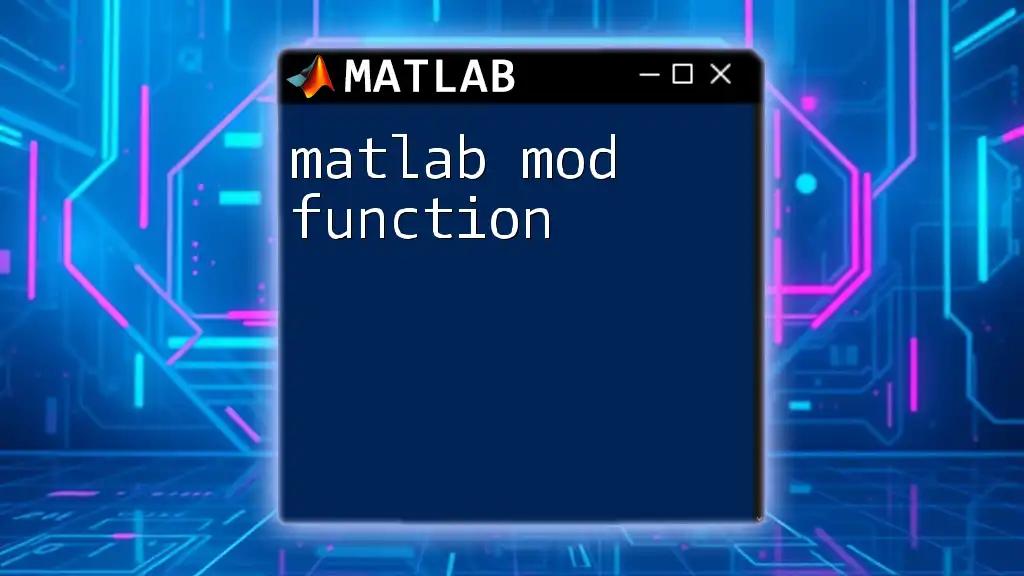
Syntax of the `min` Function
Basic Syntax for One-Dimensional Arrays
The simplest syntax for the `min` function is:
min(A)
In this case, `A` represents a one-dimensional array, and the output will be the minimum value within that array. For instance, if you have:
A = [3, 5, 1, 9, 2];
min_value = min(A); % The result will be 1
Syntax for Two-Dimensional Arrays
When dealing with matrices, the syntax expands slightly. Here’s how to find the minimum values along different dimensions:
min(A, [], dim)
The `dim` parameter specifies the dimension for which you want to find the minimum:
- 1 for columns (minimum of each column)
- 2 for rows (minimum of each row)
For example:
B = [4, 2, 7; 1, 5, 3; 8, 6, 0];
min_column = min(B); % Minimum in each column
% Result is [1 2 0]
min_row = min(B, [], 2); % Minimum in each row
% Result is [2; 1; 0]
Additional Options
The `min` function can also take additional parameters to further refine its output. For example, if you want to find the minimum value while specifying a certain condition, that can be achieved through logical indexing.
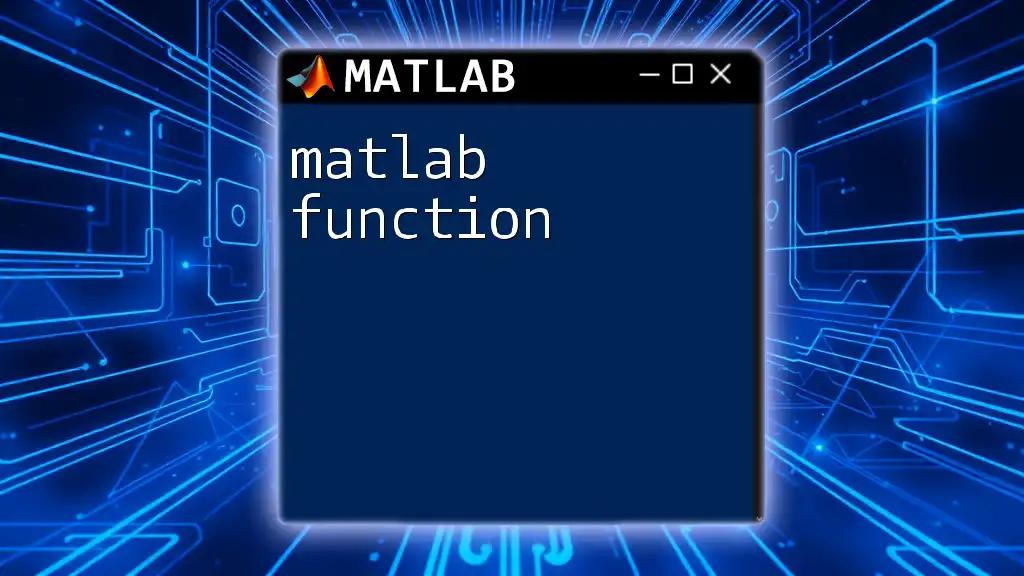
Using the `min` Function: Step-by-Step Examples
Finding the Minimum of a Vector
Let's start with a one-dimensional example where we have a vector and want to extract its minimum value:
A = [8, 3, 7, 1, 5];
min_value = min(A); % This will return 1
In this scenario, the `min` function examines each value in the array and returns the smallest one, which is 1.
Finding the Minimum of a Matrix
Now let's explore how this applies to a matrix:
B = [4, 2, 7; 1, 5, 3; 8, 6, 0];
min_column = min(B); % Minimum in each column
min_row = min(B, [], 2); % Minimum in each row
The output from `min(B)` will yield `[1 2 0]`, which means that 1 is the minimum in the first column, 2 in the second, and 0 in the third. Conversely, using `min(B, [], 2)` provides `[2; 1; 0]`, indicating the minimum values across each row.
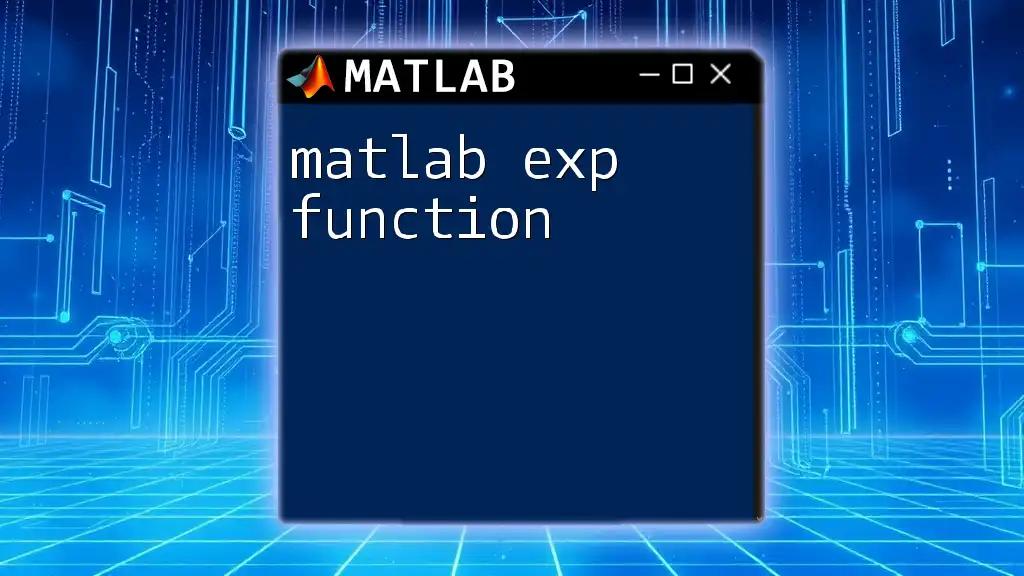
Advanced Uses of the `min` Function
Finding Minimum Values with Conditions
Real-world data is often messy. The `min` function can be combined with conditional statements to streamline data processing. For instance, if we want to find the minimum value in an array that meets a specific criterion, we can do the following:
C = [-3, -1, 5, 4, -2];
min_positive = min(C(C > 0)); % This will return 4
In the above code, the condition `C > 0` filters the values to include only positive numbers, helping us easily find the minimum of that subset.
Using `min` in Complex Data Structures
For more intricate data types, such as cell arrays or structures, applying the `min` function requires careful manipulation. For example, consider a cell array containing numeric arrays:
cellArray = {3, 5, 1; 2, 8, 7};
min_value_cell = cellfun(@min, cellArray);
Using `cellfun` applies the `min` function to each cell, returning the minimum values from each cell in a new array.
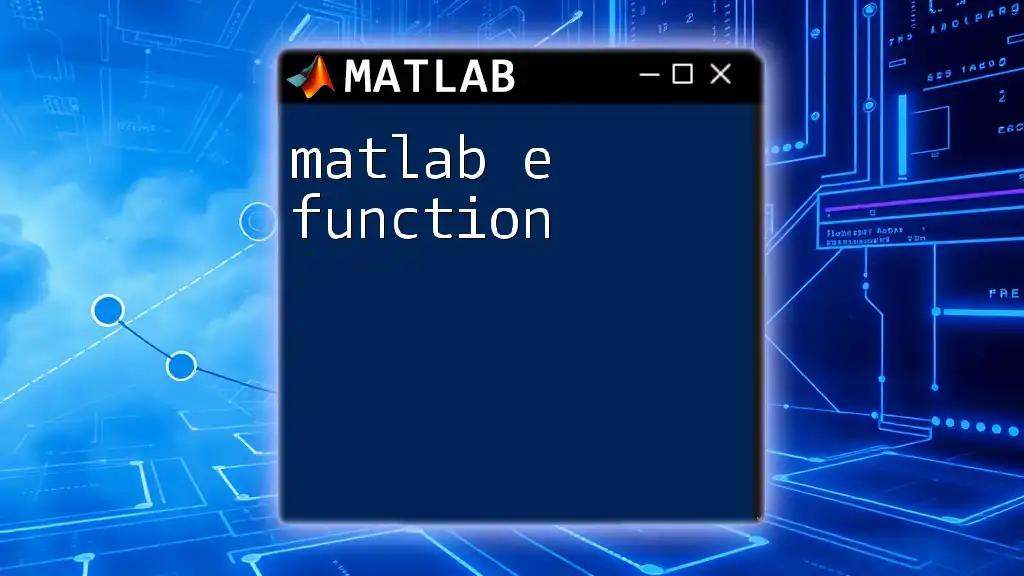
Common Mistakes and Troubleshooting
Common Errors with the `min` Function
Users often encounter errors when the dimensions of input arrays do not match their expectations. For instance, trying to find the minimum along a dimension not present in the array leads to misleading outputs. Always verify dimensions before applying the `min` function.
Performance Considerations
When dealing with large datasets, using the `min` function can sometimes lead to computational inefficiencies. To optimize usage:
- Consider pre-filtering data.
- Use logical indexing carefully to minimize unnecessary computations.

Working with Different Data Types
Using `min` with Different Numeric Types
MATLAB's `min` function supports various numeric types, including integers and doubles. This versatility allows users to seamlessly analyze a range of data formats without worrying about type compatibility.
`min` Function with Non-Numeric Arrays
Interestingly, the `min` function can even work with string arrays or categorical data:
strArray = ["apple", "orange", "banana"];
min_str = min(strArray); % This returns 'apple'
This approach highlights the function's flexibility in handling non-numeric datasets.
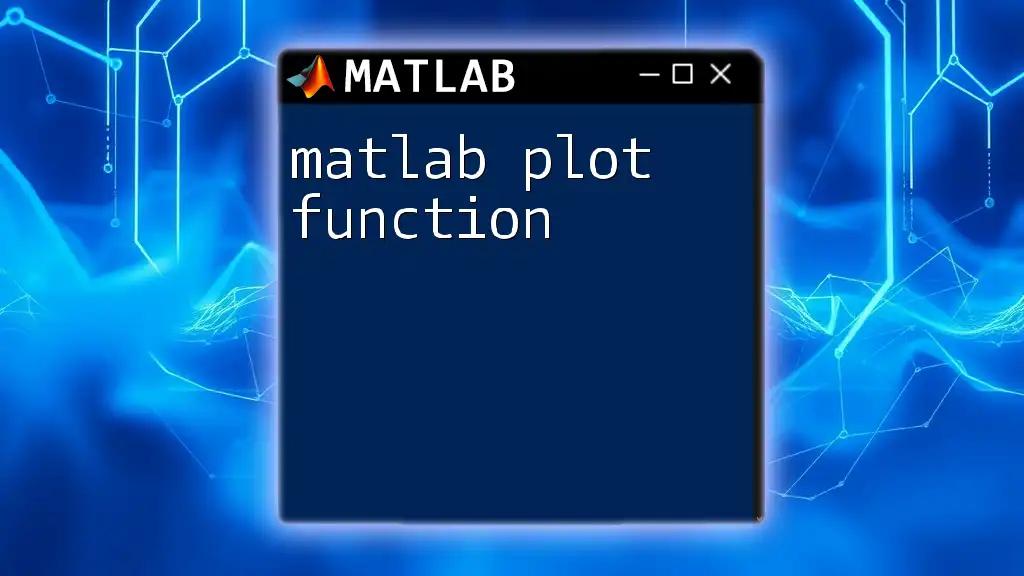
Conclusion
In summary, the `matlab min function` serves as a foundational tool for any MATLAB user, simplifying the process of finding minimum values in datasets. Whether you're working with one-dimensional arrays, matrices, or more complex data structures, mastering the `min` function enhances your data analysis capabilities.
As you continue your MATLAB journey, consider exploring further resources, such as the official MATLAB documentation, to deepen your understanding. Practice using the `min` function across various datasets and share your experiences with the community—the insights gained from collaborative learning are invaluable!