MATLAB can be run on Linux systems, allowing users to leverage the powerful features of both platforms; for instance, to display a simple plot, you can use the following command:
x = 0:0.1:10; % Create a vector from 0 to 10 with an increment of 0.1
y = sin(x); % Calculate the sine of each element in the vector
plot(x, y); % Plot the sine wave
xlabel('x-axis'); % Label for the x-axis
ylabel('sin(x)'); % Label for the y-axis
title('Sine Wave on Linux with MATLAB'); % Title of the plot
Getting Started with MATLAB on Linux
System Requirements
Before diving into using MATLAB on Linux, it's essential to ensure your system meets the requirements. MATLAB is compatible with several distributions of Linux, such as Ubuntu, CentOS, and Fedora. Here are the key specifications:
- Operating System Specifications: Check the MATLAB release notes for the supported Linux versions that work best with the software.
- Hardware Requirements: Ensure your setup meets or exceeds the minimum requirements. Generally, the requirements include:
- RAM: Minimum of 4 GB (8 GB recommended)
- CPU: A dual-core processor or better
- Disk Space: At least 20 GB of available disk space
Installation of MATLAB on Linux
Downloading MATLAB
To install MATLAB on your Linux system, start by downloading the installer from the official MATLAB website. You will need to create a MathWorks account if you don’t already have one.
Installation Process
There are two primary ways to install MATLAB on Linux: through the command line or the graphical user interface (GUI).
- Command-Line Installation: Use terminal commands for a streamlined installation, which is particularly helpful for headless systems.
- Open your terminal and navigate to the directory where the installer is located.
- Run the command to start the installation:
sudo ./install
- GUI Installation: If you prefer a visual approach, run the installer this way simply:
./install
License Configuration
After installation, you must configure your MATLAB license. This often involves activating your license key during the installation process or entering it afterward through the MATLAB interface.
Setting Up Environment Variables
Understanding and managing environment variables is vital for a smooth MATLAB experience on Linux.
Understanding Environment Variables
MATLAB uses environment variables to determine paths and configurations required for execution.
Setting MATLAB Path
To run MATLAB from any terminal session, you need to add its binary location to your system PATH. This can be done by adding the following line to your `.bashrc` or `.bash_profile` file:
export PATH=$PATH:/usr/local/MATLAB/R2023a/bin
After editing the file, apply the changes by running:
source ~/.bashrc
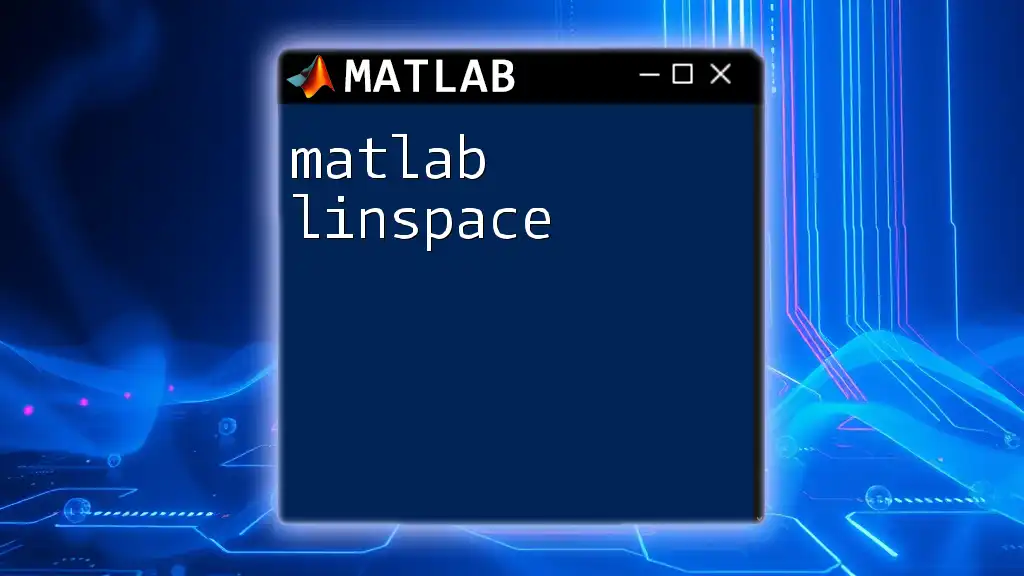
Navigating MATLAB Interface on Linux
MATLAB GUI
The MATLAB GUI on Linux is similar to that of other operating systems, providing tools for quick data analysis, visualization, and development. It consists of:
- Command Window: Directly inputs commands.
- Workspace: Displays variables in the current session.
- Editor: Used to create, edit and debug scripts.
Command Window and Editor
Using Command Window
The Command Window acts as a terminal where you can enter MATLAB commands. The syntax is straightforward. Here are a few basic examples:
x = 5;
y = x^2;
disp(y)
This code snippet assigns `5` to `x`, squares it, and then displays the result.
MATLAB Editor
The MATLAB Editor is a powerful tool for writing more complex scripts. To create a new script, click on the "New Script" icon and write your code. Here’s a basic example you might find in your script:
function myFunction(input)
output = input^2;
disp(['The square is: ', num2str(output)])
end
Save it as `myFunction.m` to execute it later.
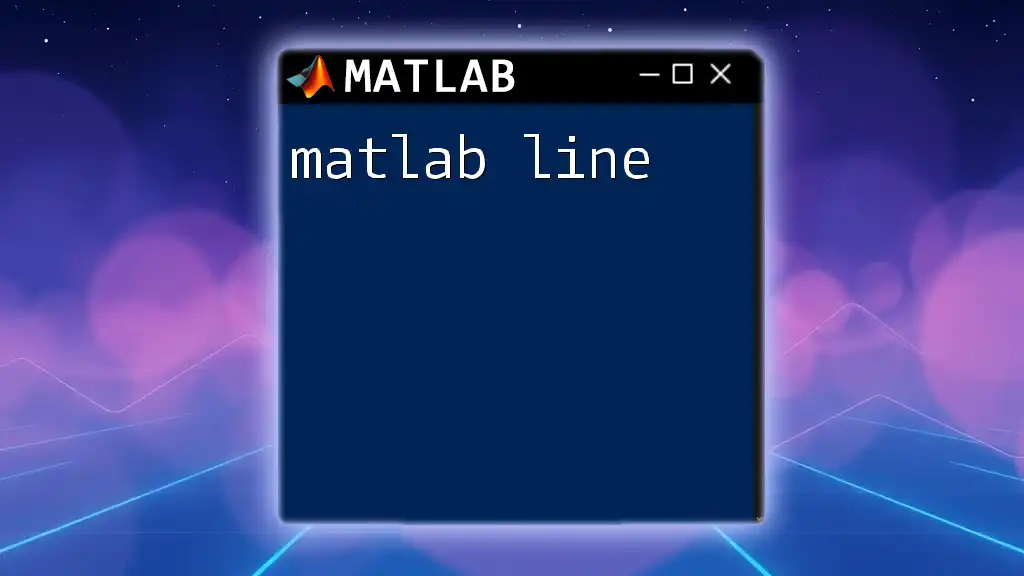
Running MATLAB Scripts on Linux
Creating and Executing Scripts
Creating a script in MATLAB is simple. Use the Editor, write your code, and save it with a `.m` extension. To execute it, simply type the script name (excluding the `.m` extension) in the Command Window:
myFunction(4)
This will display the square of 4 as defined in the function.
Command-Line Usage of MATLAB
You can also invoke MATLAB from the terminal, especially useful for automated tasks or scripts:
Starting MATLAB from Terminal
To start MATLAB from the terminal, simply type:
matlab
Running a Script via Terminal
To run a specific script directly from the terminal without opening the GUI, use the `-batch` flag:
matlab -batch "myFunction(4)"
This command will execute the `myFunction` with an input of `4` and then exit MATLAB.
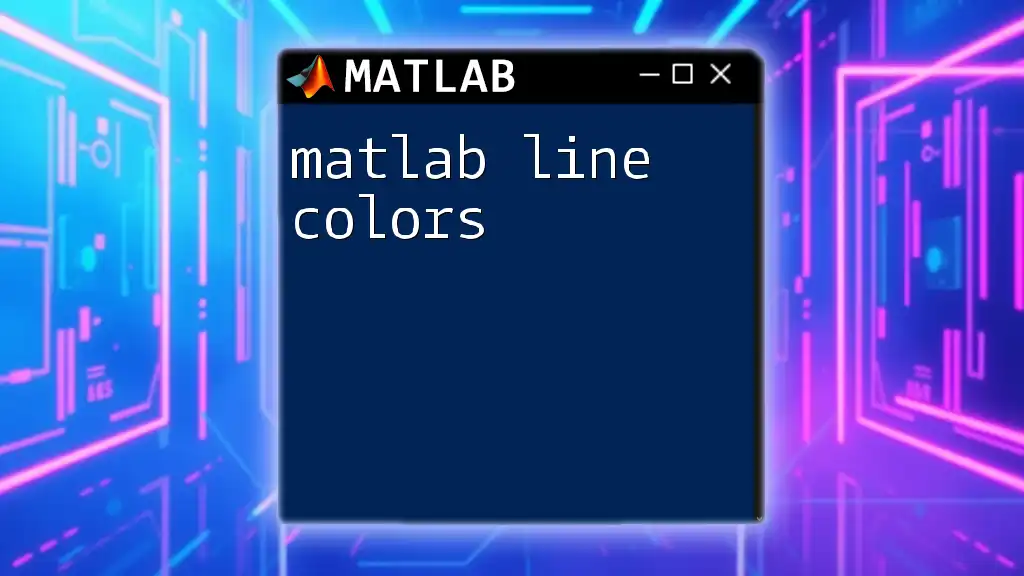
MATLAB Toolboxes and Add-ons on Linux
Installing Toolboxes
MATLAB's functionality can be further augmented through various toolboxes available for Linux users. To manage toolboxes, navigate through the Add-On Explorer in the MATLAB environment.
Types of Toolboxes Available for Linux
Some popular toolboxes suitable for diverse applications include:
- Statistics and Machine Learning Toolbox: Great for statistical analysis and predictive modeling.
- Image Processing Toolbox: Excellent for processing and analyzing images.
- Control System Toolbox: Perfect for designing and analyzing control systems.
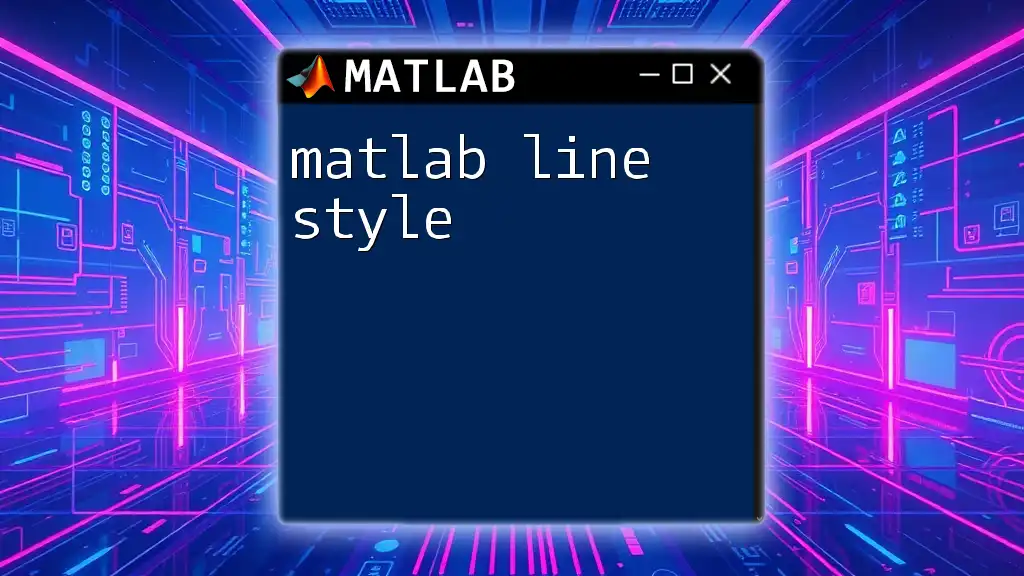
Common Issues with MATLAB on Linux
Troubleshooting Installation Problems
While installing MATLAB on Linux, users often encounter issues. Here are some common ones and their solutions:
-
Dependencies Not Met: You may need to install additional packages. Use your package manager to do so. For Ubuntu, you can find missing dependencies using:
sudo apt-get install <missing-package>
-
Installation Errors: If you encounter errors during the installation, checking the installation log can provide insights on how to resolve them.
Performance Optimization Tips
To enhance the performance of MATLAB on Linux, consider the following tips:
- File Management: Organizing your MATLAB files into projects or folders can drastically improve efficiency.
- Handling Large Data: Utilize MATLAB's built-in functions such as `matfile` for efficient loading of large datasets. This prevents large data from overwhelming your system memory.
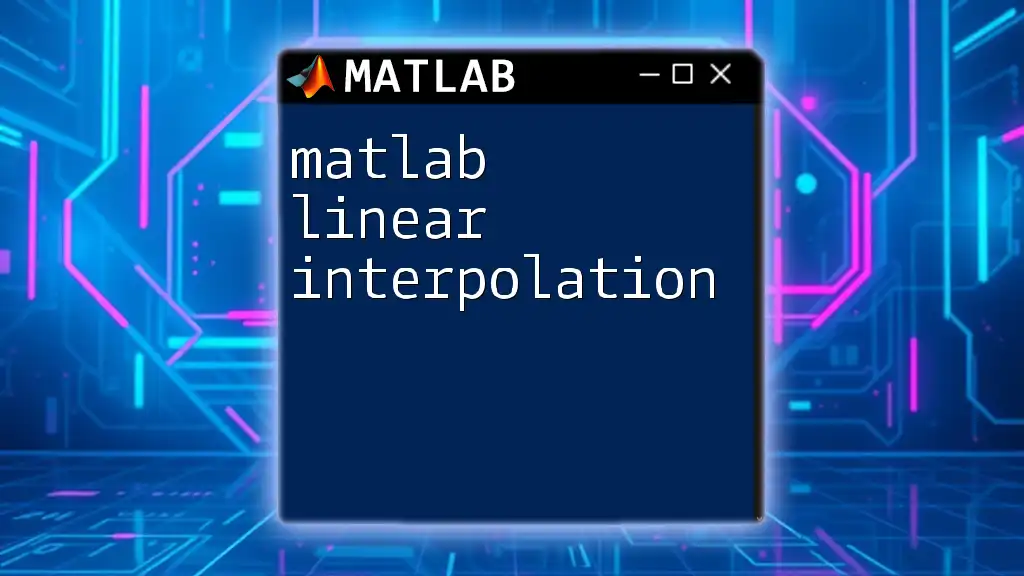
Example Projects using MATLAB on Linux
Simple Data Analysis Project
Here’s a quick example of how to create a simple data analysis project using MATLAB. Begin by generating random data and plotting a histogram:
% Sample data generation
data = rand(100,1);
histogram(data)
title('Sample Histogram')
xlabel('Data Values')
ylabel('Frequency')
This code snippet generates a histogram of 100 random values between 0 and 1.
Visualization Project
MATLAB is renowned for its powerful visualization capabilities. Here’s how you can create a simple sine wave plot:
x = 0:0.1:10; % Generate x values from 0 to 10
y = sin(x); % Compute sine of x
plot(x, y) % Plot the sine wave
title('Sine Wave')
xlabel('X-axis')
ylabel('Y-axis')
This script produces a smooth sine wave, showcasing the beauty of MATLAB’s plotting functions.
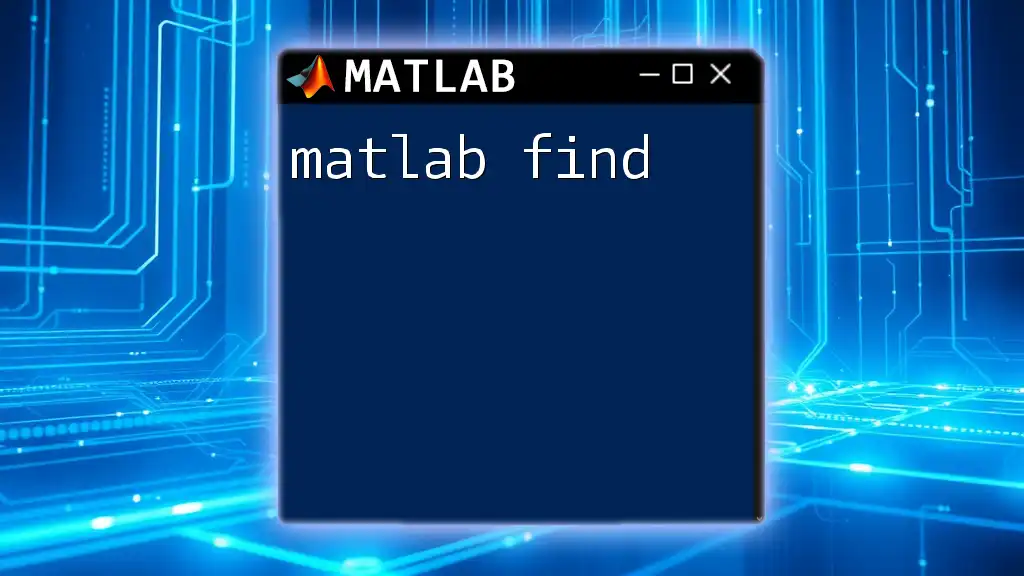
Conclusion
In this guide, we explored a comprehensive approach to using MATLAB on Linux. From installation to troubleshooting, and even executing scripts, you now have the tools and knowledge to leverage MATLAB effectively in a Linux environment. Embrace the rich features of MATLAB to enhance your projects, whether it's data analysis, visualization, or building complex simulations.