In MATLAB, the `input` function allows users to prompt for user input from the command line, enabling dynamic data collection during program execution.
Here's an example:
userValue = input('Please enter a number: ');
MATLAB Input Basics
What is Input in MATLAB?
In the context of programming, input refers to the data that a user provides to the program for further processing. In MATLAB, understanding input mechanisms enhances your ability to interact dynamically with the program and allows for data to be manipulated efficiently.
Types of Input
MATLAB supports various input types:
- Numerical Input: Used for entering numerical values.
- Textual Input: Can capture characters or strings.
- Logical Input: Boolean values representing true or false.
Basic Input Functions
`input` Function
The `input` function in MATLAB is the primary means for obtaining user input. Its syntax is intuitive, allowing users to prompt for data effectively.
Here's an example of how to use the `input` function:
userInput = input('Please enter a number: ')
In this example, when the command is executed, the user is prompted to enter a number, which is then stored in the variable `userInput`.
Usage Scenarios: The `input` function is particularly beneficial in cases where a user needs to provide dynamic data, such as during simulations or interactive applications.
Example: Calculating Area
Let’s consider a practical example where we prompt the user for the radius of a circle and calculate its area:
radius = input('Enter the radius of the circle: ');
area = pi * radius^2;
fprintf('The area is: %.2f\n', area);
In this snippet, the user is prompted for the radius, and the program calculates and displays the area of the circle.
`disp` Function
The `disp` function is another essential command that is used for displaying output without requiring specific format options.
For example:
disp('Hello, welcome to MATLAB input guide.');
This command simply prints the greeting message to the console, making it an excellent tool for providing feedback or information to users.
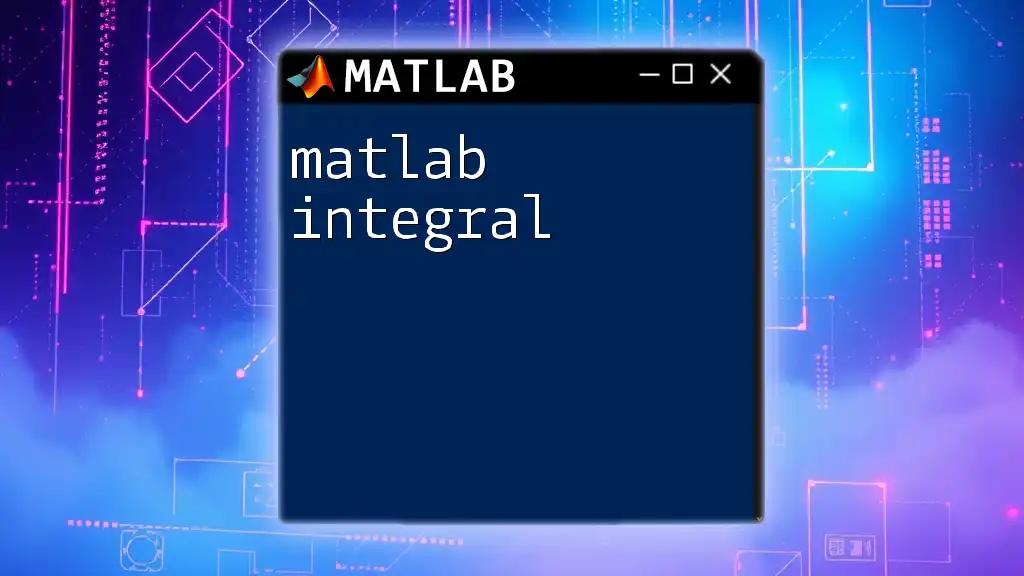
Advanced Input Techniques
Validating Input
Input Validation Techniques
Input validation is crucial for ensuring that the data entered by users meets specific criteria, preventing errors and unexpected behavior in your programs.
Common validation methods include:
- Using `isnumeric`: Determines if the input is a numeric value.
- Using `ischar`: Checks if the input is a string.
- Using `islogical`: Validates boolean values.
Example of Validation: Here’s how to validate that the user has entered a numeric value:
userNumber = input('Enter a number: ');
if isnumeric(userNumber)
disp('Thank you for entering a valid number!');
else
disp('Error! Not a valid number.');
end
In this example, if the user enters a non-numeric value, the program responds appropriately, demonstrating good practice in input handling.
Working with Strings
Using `input` for String Data
When capturing strings, the `input` function can process character arrays by appending the `'s'` argument. This tells MATLAB to interpret the input as a string.
Example: Capturing a Name
name = input('Enter your name: ', 's');
fprintf('Hello, %s!\n', name);
In this code, the user’s name is captured as a string, and a greeting is displayed, highlighting how MATLAB can interact meaningfully with textual input.
Multi-Input Handling
Accepting Multiple Inputs
In situations where multiple pieces of input are required concurrently, MATLAB allows capturing them efficiently.
Example of Multiple Inputs:
[x, y] = deal(input('Enter x: '), input('Enter y: '));
fprintf('You entered x = %d and y = %d\n', x, y);
This example demonstrates how to gather two separate inputs and store them in variables `x` and `y` using the `deal` function, streamlining the input process.
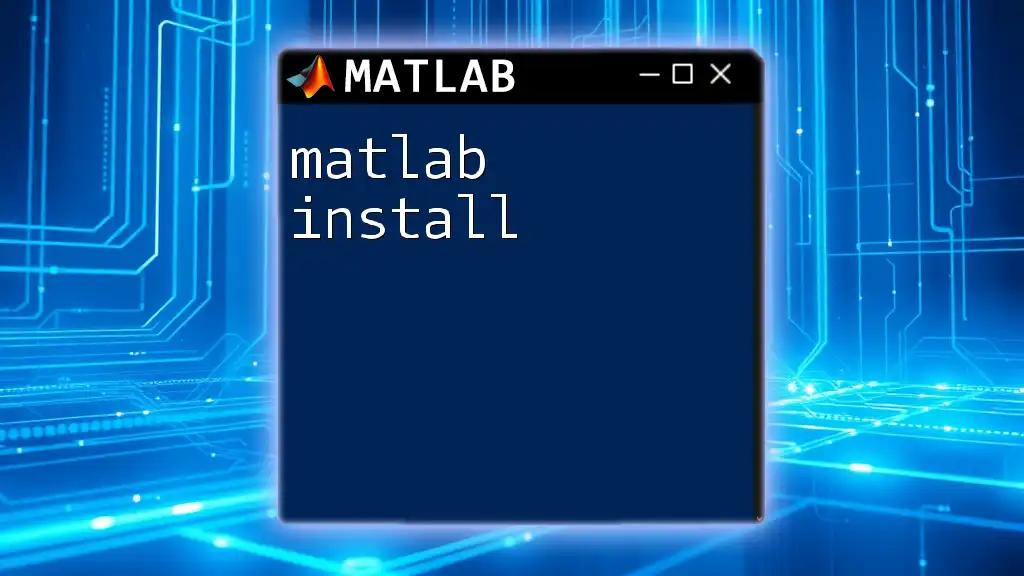
Storing Inputs
Creating Variables from Input
Dynamic variable creation allows for flexibility in coding. In MATLAB, you can use user input to create variables with user-defined names, enhancing the interactive nature of the program.
Example of Variable Creation:
varName = input('Enter variable name as a string: ', 's');
eval([varName ' = input(''Enter value for ' varName ': '');']);
Here, the user’s input determines both the name and value of the variable, which can add an advanced layer to your coding skills.
Using Cell Arrays for Inputs
For handling a variable number of inputs, cell arrays are extremely useful as they allow you to store different types of data conveniently.
Example of Cell Array Inputs:
inputs = cell(1,3);
for i = 1:3
inputs{i} = input(['Enter input ' num2str(i) ': ']);
end
disp(inputs);
This code snippet collects three user inputs and stores them in a cell array, demonstrating how MATLAB can manage structured data effectively.
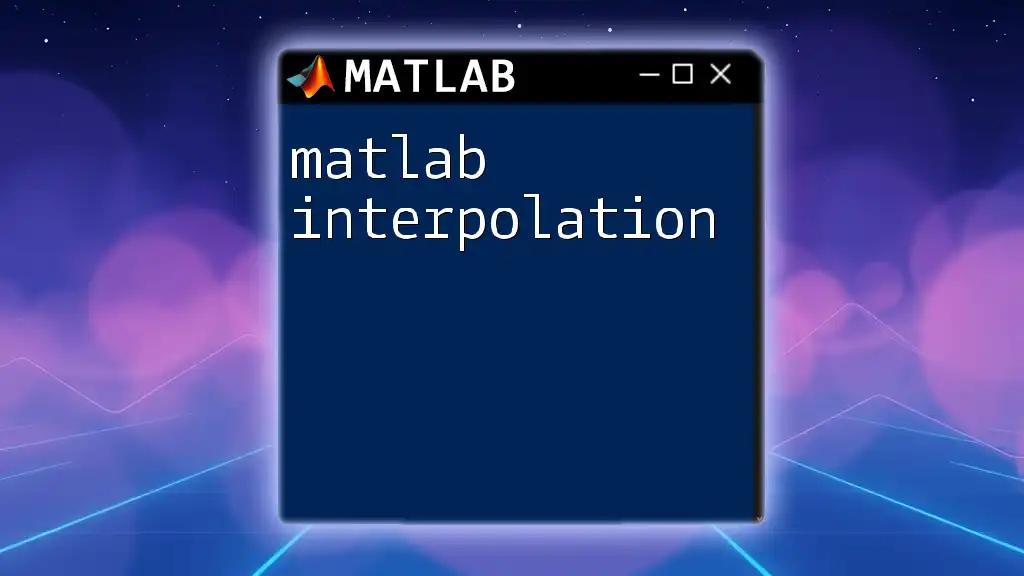
Troubleshooting Common Input Issues
Common Errors and Solutions
Input Mismatch Errors
When a user provides an input that does not match the expected type, it can result in errors. For instance, attempting to perform arithmetic on a string will yield an error message.
Best Practices to Avoid Errors
Incorporating safety checks and fallback mechanisms helps prevent errors from crashing your program. Always anticipate user mistakes and implement checks accordingly.
Example of Error Handling
A robust way to handle potential input errors is to use try-catch blocks:
try
userInput = input('Enter a number: ');
if ~isnumeric(userInput)
error('Invalid input! Please enter a number.');
end
catch ME
disp(ME.message);
end
This example captures errors gracefully and provides feedback to the user, improving the overall user experience.
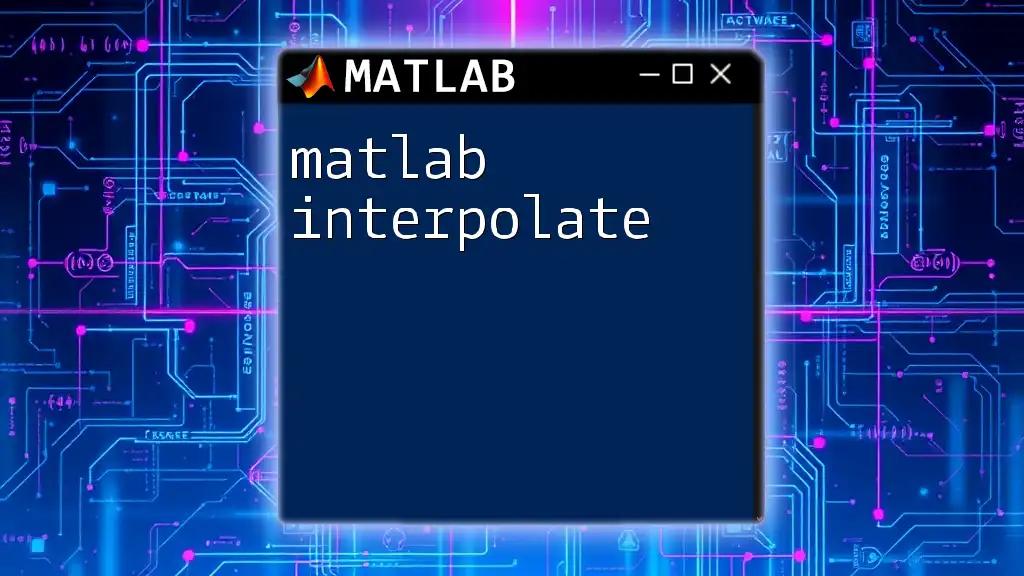
Conclusion
Understanding how to utilize MATLAB input effectively is critical for creating interactive and dynamic programs. The concepts explored in this guide, from basic input functions to advanced validation techniques, illustrate the importance of managing user input appropriately. By practicing these concepts, you will enhance your MATLAB skills and develop a deeper comprehension of how to engage with your users more effectively.
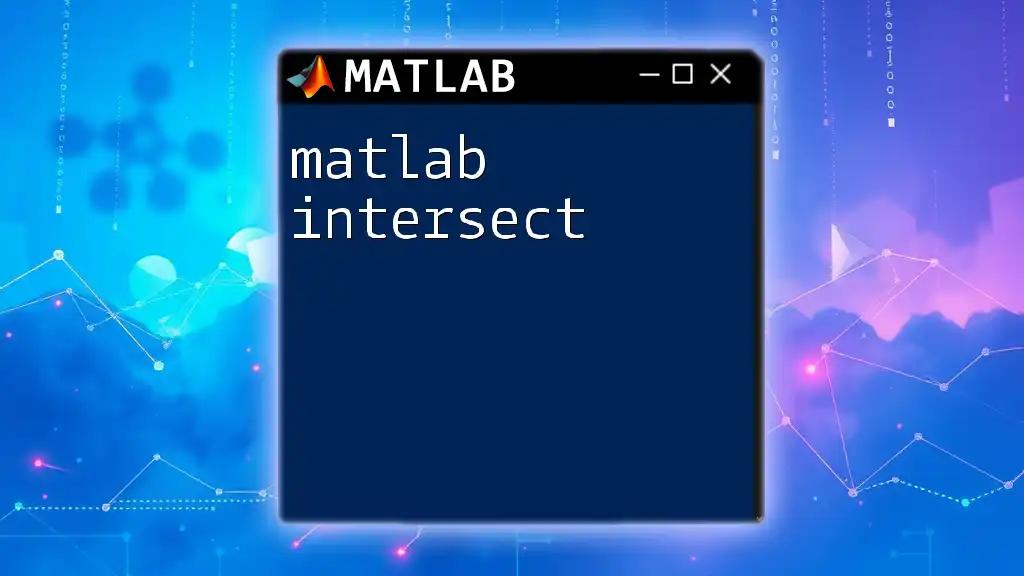
Additional Resources
To further expand your knowledge, explore the official MATLAB documentation and engage with online courses that can provide a structured learning path. Online forums and community resources also offer a wealth of information and support for MATLAB learners.