The `find` function in MATLAB is used to locate the indices of non-zero elements in an array, enabling efficient data retrieval and manipulation.
Here's a code snippet demonstrating its usage:
A = [0 3 0 5; 2 0 0 0; 1 0 4 0];
[row, col] = find(A); % Finds the row and column indices of non-zero elements
Understanding the `find` Command
What is the `find` Command?
The `find` command in MATLAB is a versatile function used to locate indices of non-zero and specific values in an array or matrix. It plays a crucial role in data analysis and programming, allowing users to efficiently filter and manipulate data.
Syntax of the `find` Command`
The syntax of the `find` command varies based on the requirements of the user. Understanding the syntax is essential for effective use:
- Basic Usage: `find(X)` returns the indices of non-zero elements in the matrix `X`.
- Limiting Output: `find(X, k)` returns the first `k` indices of non-zero elements.
- Direction Control: `find(X, k, direction)` allows for specifying the order of output, such as 'first' or 'last'.
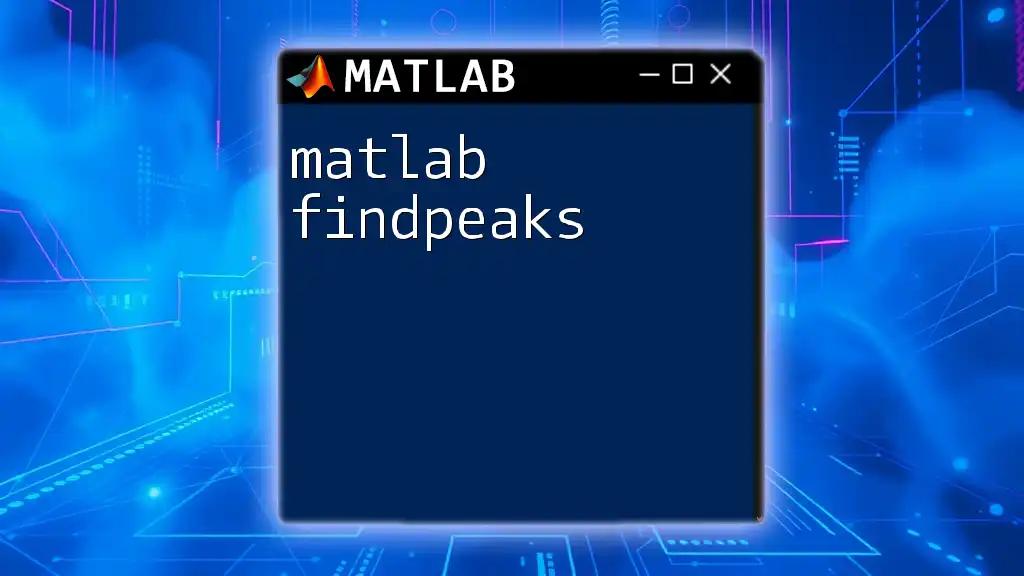
How to Use the `find` Command
Finding Non-Zero Elements
In many applications, identifying non-zero elements is a primary use of the `find` command. The command can sift through data, pinpointing which elements are non-zero.
Example Code Snippet:
A = [0 1 0 2; 0 0 3 4];
idx = find(A);
disp(idx);
Explanation of Output: In this example, the output will display the linear indices of all non-zero elements in matrix `A`. The function flattens the matrix into a single column, hence providing a single dimension as output.
Locating Specific Values in an Array
The `find` command can also be used to locate specific values based on given conditions. This is particularly useful for filtering data sets.
Example Code Snippet:
B = [5, 10, 15, 20];
indices = find(B > 10);
disp(indices);
Explaining the Condition and Output: In this code, `find` identifies which elements in `B` are greater than 10. The output will showcase the indices of those specific elements—15 and 20—which can be useful in subsequent data manipulation tasks.
Multi-Dimensional Arrays
Using the `find` command with matrices (multi-dimensional arrays) enhances its utility, making it possible to extract both row and column indices of non-zero elements.
Example Code Snippet:
C = [1, 0, 3; 4, 0, 6; 7, 0, 9];
[row, col] = find(C);
disp([row, col]);
Expanding on Output for Multi-Dimensional Data: The output will show pairs of indices, representing the row and column positions of non-zero elements in matrix `C`. Understanding how to manipulate these indices is vital for effective data operations.

Advanced Features of the `find` Command
Finding the n Largest/Smallest Elements
A more advanced feature of the `find` command is its ability to locate the top n largest or smallest values within an array. This functionality aids in statistical analysis and can highlight significant data points.
Example Code Snippet:
D = [2, 3, 1, 5, 4];
[~, idx] = maxk(D, 3);
disp(idx);
Interpretation of Results: In this example, `maxk` retrieves the indices of the three largest elements from array `D`. Understanding how to work with this output is crucial for tasks that involve ranking or prioritizing data.
Direction Parameter
The `find` command also includes a parameter for determining the direction of index retrieval, which can dramatically change the output depending on the user's intent.
Example Code Snippet:
E = [10, 20, 30, 40];
[idx, val] = find(E > 15, 2, 'last');
disp(idx);
disp(val);
Explaining 'first' vs. 'last': In this case, if 'last' is utilized, it indicates that the function will return the last two occurrences of elements that meet the specified condition (greater than 15). Understanding these subtleties enables users to wield the command more effectively.

Practical Applications of `find`
Data Cleaning and Filtering
The `find` command is invaluable in data cleaning tasks. It can help isolate NaN values or zeros, allowing users to effectively prepare their data for analysis.
Example Code Snippet:
F = [NaN, 1; 2, NaN; 3, 4];
validIdx = find(~isnan(F));
disp(validIdx);
In this snippet, the command finds all indices that do not contain NaN values, making it easy to focus on valid entries in an analysis.
Condition-Based Analysis
Using `find` in condition-based analysis allows for thorough investigations into data attributes and relationships.
Example Code Snippet:
G = [7, 1, 4; 2, 6, 3];
conditionCheck = find(G > 5);
disp(conditionCheck);
This code identifies which elements exceed a specified threshold (in this case, 5), streamlining processes that depend on condition checks and enabling faster data-driven decision-making.
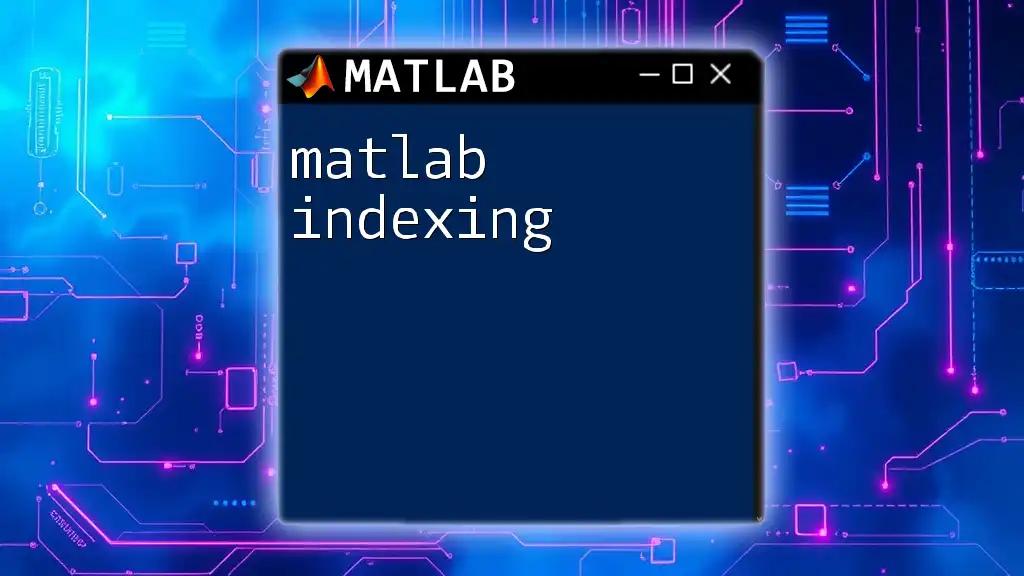
Best Practices for Using `find`
Avoiding Common Pitfalls
When using the `find` command, users often overlook the dimensions of their data or misuse the command features. It is important to be aware of these potential errors to ensure accurate results.
Performance Considerations
For large datasets, employing `find` efficiently can greatly affect performance. Utilizing logical indexing or vectorized solutions when feasible can enhance execution speed.
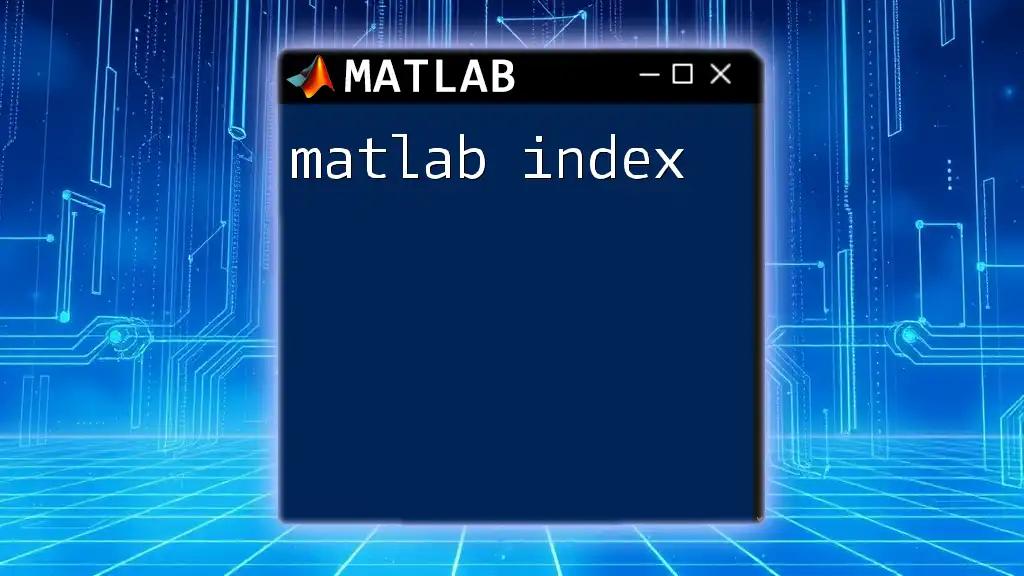
Conclusion
The `find` command in MATLAB is an incredibly powerful tool in the programmer’s toolkit, enabling detailed data manipulation and condition checking. By mastering this command, users can significantly enhance their data analysis skills and streamline their workflow.
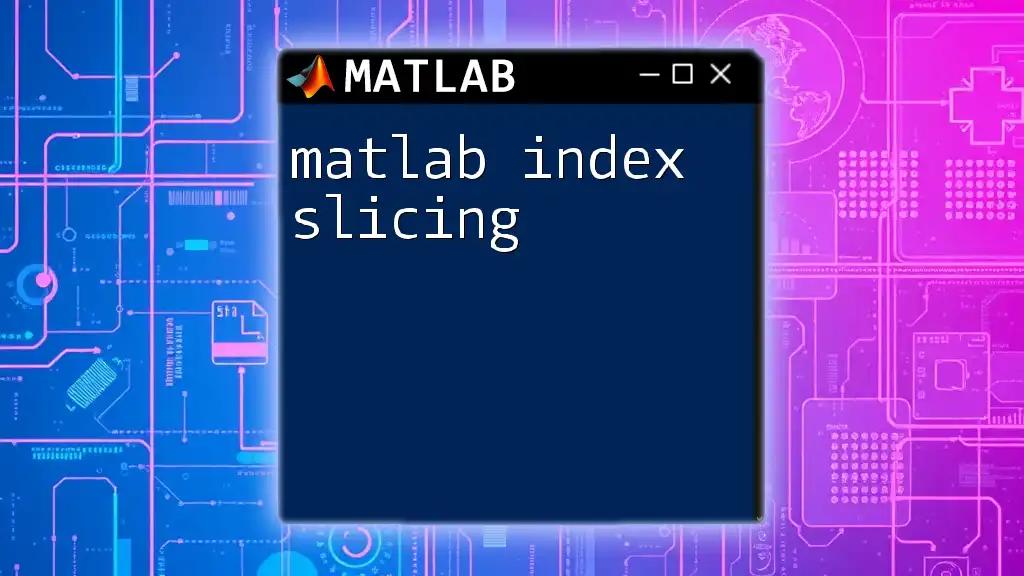
Additional Resources
For more in-depth understanding and capabilities of the `find` command, users are encouraged to explore the official MATLAB documentation and supplementary readings on MATLAB functions and data analysis techniques.
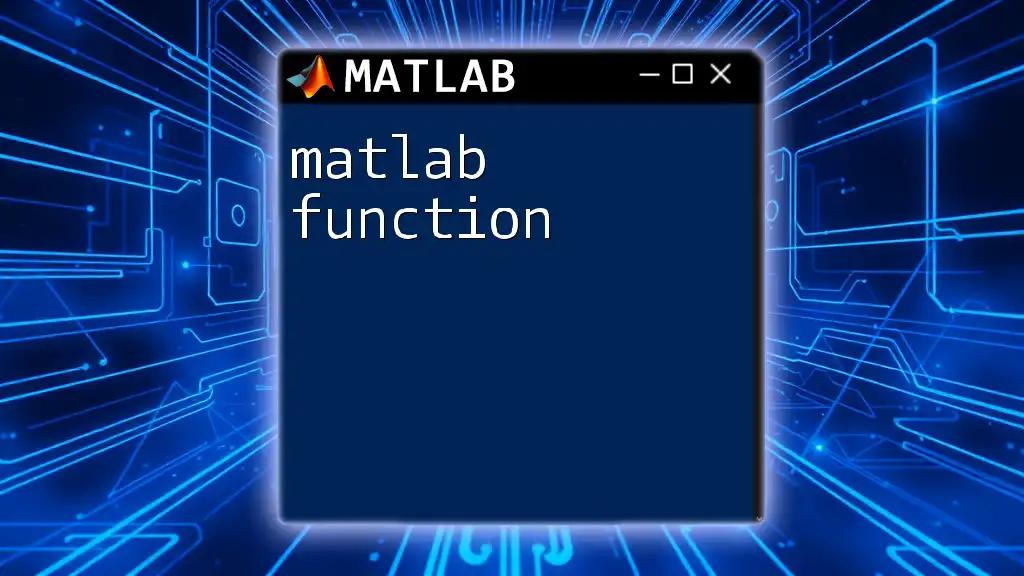
Call to Action
Dive into your MATLAB projects today and start experimenting with the `find` command. Discover how it can revolutionize your data handling experience! For more structured learning, check out our upcoming courses and workshops focused on efficient MATLAB usage.