The `num2str` function in MATLAB converts numeric values into their string representation, making it easier to concatenate or display numbers as text.
% Example of using num2str to convert a number to a string
number = 123.456;
str = num2str(number); % str is now '123.456'
Understanding `num2str`
What is `num2str`?
`num2str` is a MATLAB function used to convert numerical data into its string representation. This function is particularly beneficial when you want to display numeric results in a more readable format or when you need to concatenate numeric values with other strings.
Syntax of `num2str`
The basic syntax for the function is straightforward:
str = num2str(x)
Here, `x` can be any numeric value or array, and `str` will be the resulting string representation of `x`. The function also has optional parameters that allow you to customize the conversion further, helping you meet specific formatting requirements.
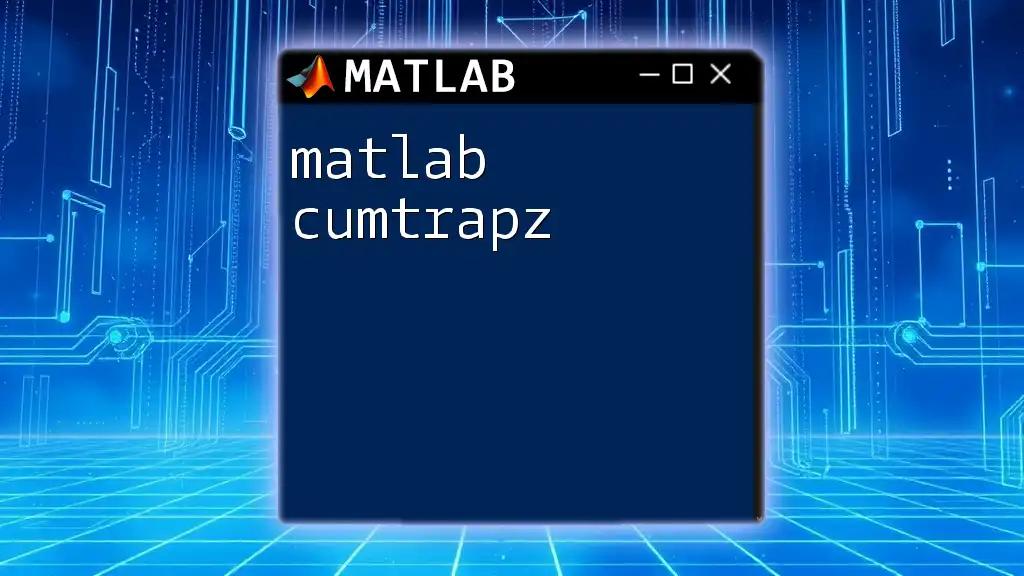
Why Use `num2str`?
Converting Numbers to Readable Strings
When working with numeric data in MATLAB, it’s essential to present results in a way that's easily interpretable. For instance, when generating reports or visualizing data, converting numbers to strings can provide better context. Without this conversion, outputs may be cryptic or difficult to read.
Combining with Other Functions
The versatility of `num2str` shines when it is combined with other functions. For example:
value = 3.14;
disp(['The value of pi is approximately: ' num2str(value)]);
In this example, `num2str` converts the numeric value of pi into a string, allowing it to be seamlessly integrated into the output string provided by the `disp` function. This practice is critical in creating informative printouts or user messages.
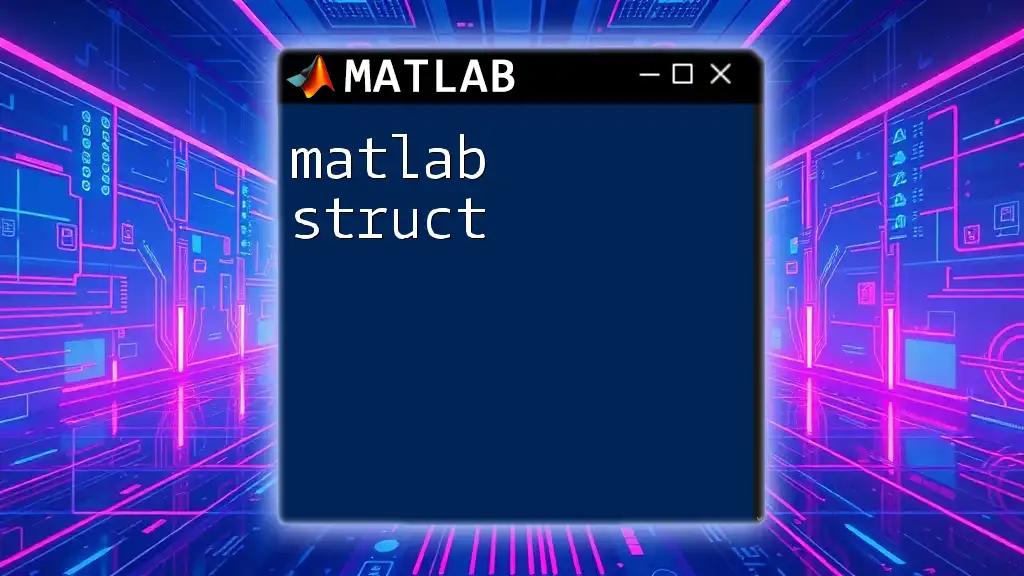
Key Features of `num2str`
Specifying Format
One of the standout features of `num2str` is its ability to specify formatting options. You can control how many decimal places to include or switch to scientific notation as needed.
For example:
str1 = num2str(3.14159, '%.2f'); % Fixed-point notation
str2 = num2str(3.14159, '%.4e'); % Scientific notation
In the first line, `%.2f` generates a string formatted to two decimal places, while in the second, `%.4e` outputs the string in scientific notation with four decimal places.
Handling Arrays
`num2str` doesn’t just work with single numbers—it can also convert whole arrays into strings.
For example, consider a 1D array:
arr = [1.2, 3.4, 5.6];
str_arr = num2str(arr);
This will create a space-separated string of numbers. With a 2D array, `num2str` maintains a readable format as well:
matrix = [1.2, 3.4; 5.6, 7.8];
str_matrix = num2str(matrix);
The result will be neatly formatted as a matrix string representation, making it suitable for display or further processing.
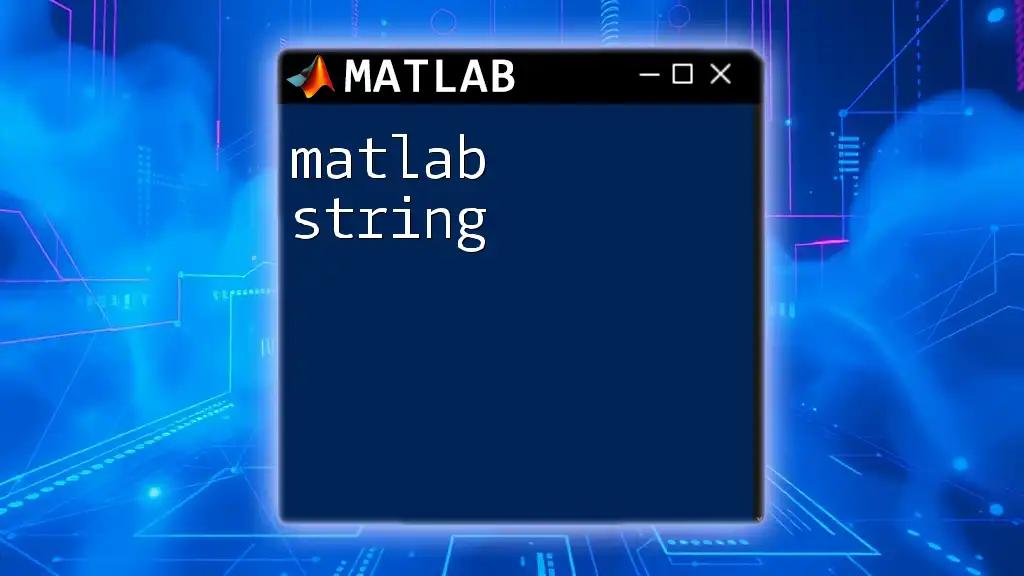
Advanced Usage of `num2str`
Customizing Delimiters
Sometimes, you may want to have a specific delimiter rather than the default space. `num2str` enables you to customize how elements are separated.
For example:
arr = [1.2, 3.4, 5.6];
str_custom = num2str(arr, '%.2f', 'delimiter', ', ');
This command produces a string where the numbers are separated by a comma and a space, enhancing readability for viewers who might find the default format cluttered.
Converting Complex Numbers
The `num2str` function is also adept at converting complex numbers into string representation. For instance:
cplx = 3 + 4i;
str_cplx = num2str(cplx);
This will result in the string output that clearly represents the complex number, aiding in applications where complex arithmetic is performed.
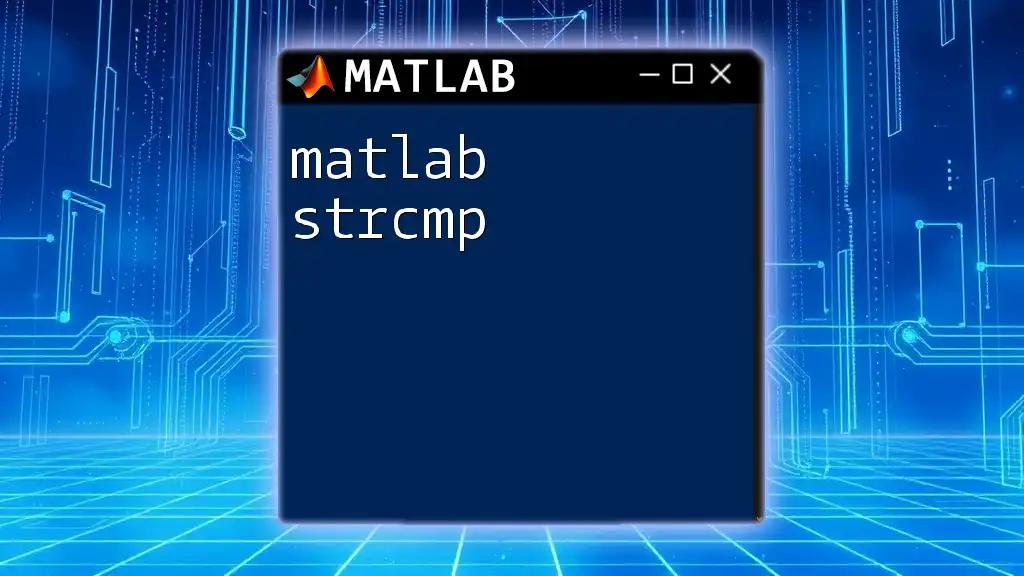
Performance Considerations
Efficiency in Large Datasets
When working with large datasets, the efficiency of `num2str` can be a concern. The function handles conversions effectively, but excessive use—especially in loops—can lead to performance bottlenecks. It is advisable to optimize string conversion tasks by minimizing the number of calls to `num2str`.
Alternative Methods
In situations where performance becomes an issue, or more specific formatting is required, consider other MATLAB functions like `sprintf` or `string`. Each offers unique advantages:
str_sprintf = sprintf('Value: %.2f', 3.14);
`sprintf` allows for formatted output and can handle multiple values efficiently, making it more suitable in cases of extensive formatting needs.
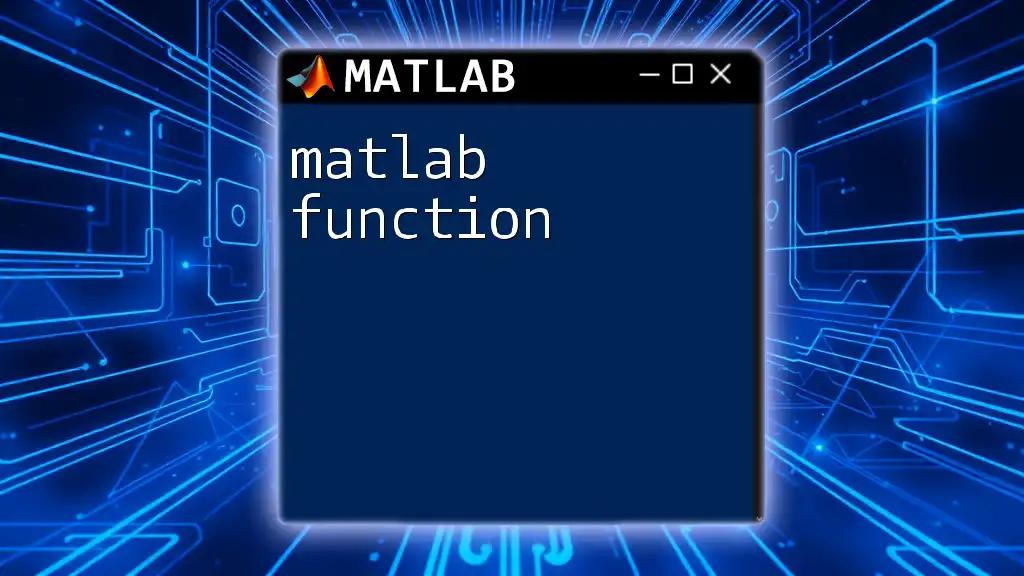
Common Mistakes and Troubleshooting
Common Errors in Usage
While `num2str` is straightforward to use, several common mistakes can hinder its effectiveness:
- Incorrect formatting strings: Ensure that the format specifiers match your data types. Mistakes here can lead to unanticipated results or errors.
- Neglecting complex numbers: Failing to recognize when to use `num2str` for complex numbers may result in displays that are not helpful.
FAQs
- Can `num2str` handle NaN values? Yes, `num2str` will convert NaN to the string 'NaN'.
- What happens to empty arrays? An empty matrix will return an empty string.
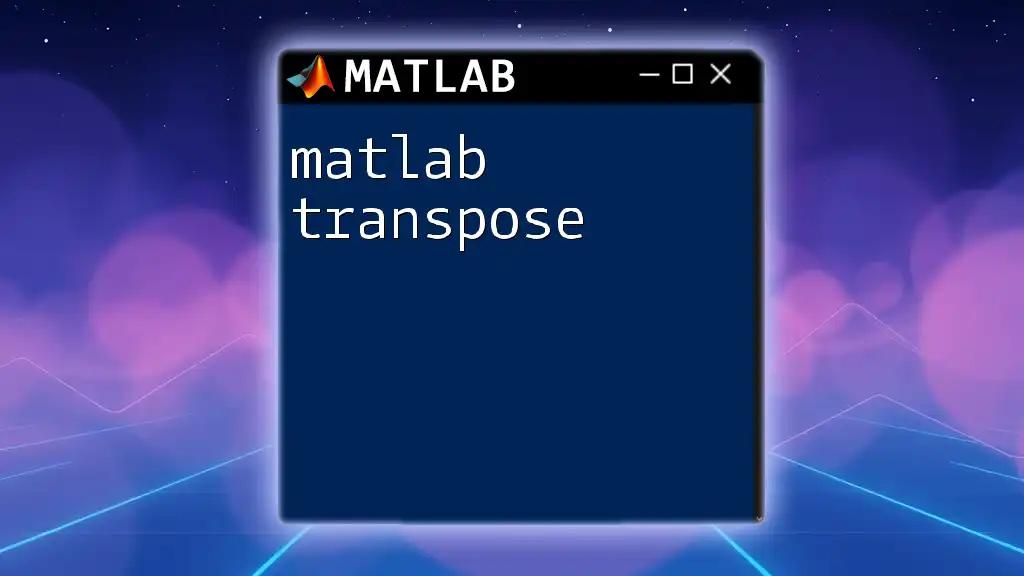
Conclusion
The `matlab num2str` function is an invaluable tool for converting numerical data into more digestible formats. Its ability to handle both basic numeric cases and complex scenarios—like arrays and customized outputs—makes it a critical component of effective MATLAB programming. Experimenting with its various features can drastically enhance your coding efficiency and result in clearer, more informative outputs.