The `linspace` function in MATLAB generates a linearly spaced vector of a specified number of points between two given values.
Here is a code snippet demonstrating its use:
% Generate 5 linearly spaced points between 1 and 10
vector = linspace(1, 10, 5)
Understanding linspace Function
What is linspace?
The MATLAB linspace function is a powerful tool used to generate linearly spaced vectors. It creates a vector of evenly spaced numbers between a specified starting and ending value. This functionality is particularly useful in numerical analysis, data visualization, and simulation scenarios where precise control over sample points is required.
Syntax of linspace
The syntax of the linspace function is straightforward:
linspace(a, b, n)
- Start value (a): This is the initial value of the sequence.
- End value (b): This is the final value of the sequence.
- Number of points (n): This optional parameter specifies how many points should be generated between a and b. If n is omitted, linspace defaults to generating 100 points.
The generated output is a row vector containing n equally spaced points. Understanding the input parameters and their implications is crucial for effectively using linspace in various applications.
About Output
The resultant output of linspace is a vector of values starting from a and ending at b, inclusive. If n points are requested, each point will be the same distance apart, calculated as \((b - a) / (n - 1)\). This predictability makes linspace an excellent choice for creating datasets for testing functions or plotting graphs.
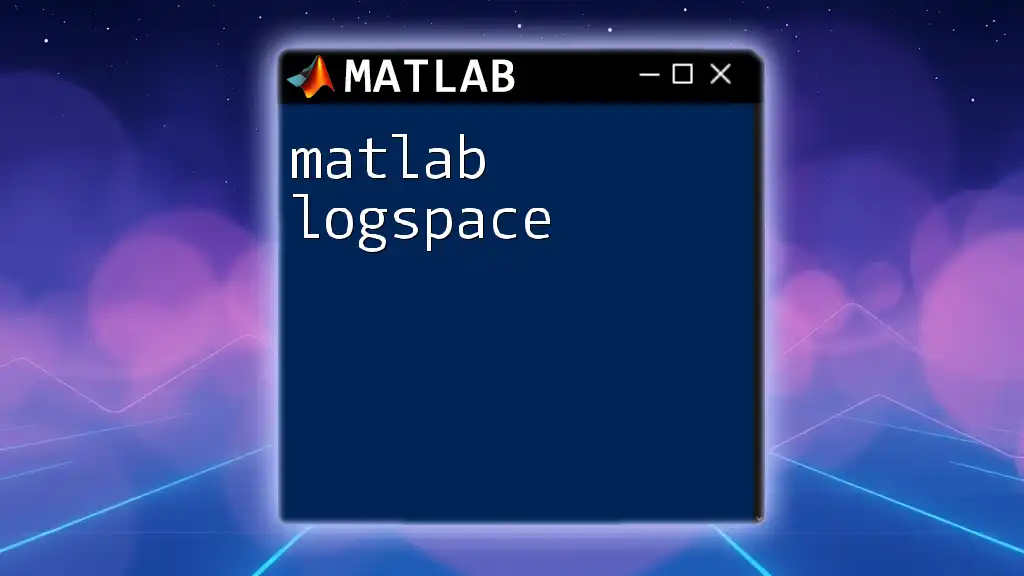
Practical Applications of linspace
Use in Plotting
One of the most common applications of MATLAB linspace is in plotting functions. By generating a set of points, you can create smooth curves or lines on a graph. For instance, consider plotting a sine function:
x = linspace(0, 2*pi, 100);
y = sin(x);
plot(x, y);
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
In this example, the linspace function generates 100 points ranging from 0 to 2π. This results in a smooth sine wave when plotted, illustrating the power of linspace in the visualization of mathematical functions.
Simulation and Modeling
In various simulation scenarios, linspace can help simplify parameter sweeps or scenarios. For instance, if you want to simulate a system's behavior over a range of stiffness coefficients, the following example demonstrates how you can utilize linspace:
k_values = linspace(0.1, 1, 10);
for k = k_values
% Simulate model for each k value
end
Here, linspace generates 10 values between 0.1 and 1. The loop then allows each value of k to be used in simulations iteratively, which is a common practice when exploring monotonic relationships in models.
Combining linspace with Meshgrid
The meshgrid function in MATLAB enhances the capabilities of linspace by allowing for multidimensional scenarios. By combining both functions, you can create grid coordinates, perfect for plotting surfaces in a 3D space. Here’s how you can easily achieve this:
[x, y] = meshgrid(linspace(-3, 3, 30), linspace(-3, 3, 30));
z = x.^2 + y.^2;
surf(x, y, z);
In this example, linspace generates points in both x and y directions to form a two-dimensional grid. The resulting z values create a 3D surface plot, visually representing a mathematical model. This is particularly effective for demonstrating complex relationships in engineering and physics.
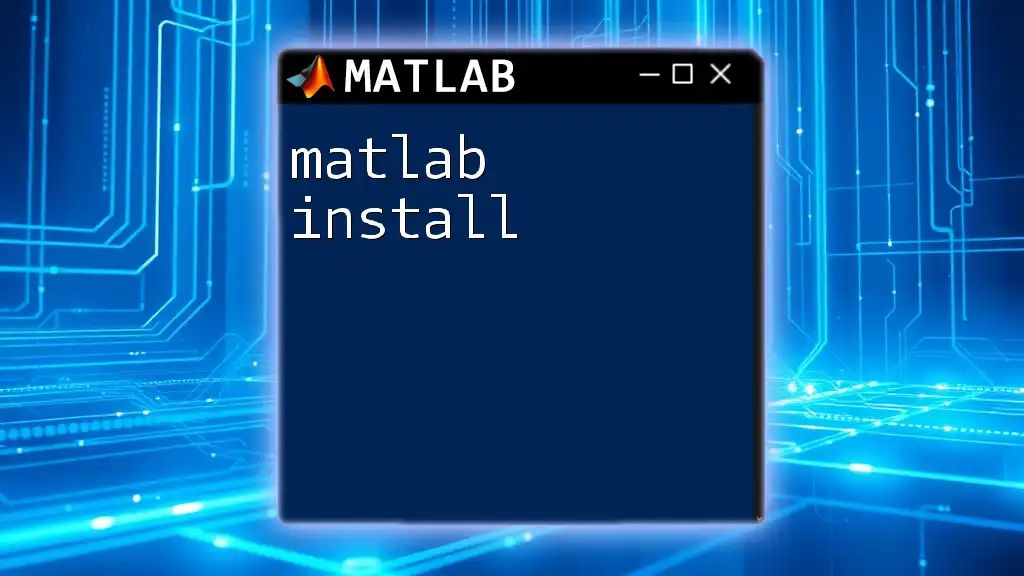
Advanced Usage of linspace
Custom Functions Integration
The versatility of MATLAB linspace allows it to easily integrate with user-defined functions. For example, suppose you want to explore a quadratic function within a specific range. You could implement it as follows:
function y = quadratic(x)
y = x.^2 + 2*x + 1;
end
x_vals = linspace(-10, 10, 50);
y_vals = quadratic(x_vals);
plot(x_vals, y_vals);
This approach illustrates how linspace provides the domain for a custom function, allowing you to visualize the results seamlessly. Such practices can significantly aid in understanding relationships between variables in mathematical modeling.
Performance Considerations
When working with large datasets or functions, the choice of how to generate point vectors can impact performance. Linspace often offers a more efficient method over manual array creation techniques.
For scenarios involving extensive computations, using linspace ensures that points are distributed evenly without the overhead of loops or additional calculations. Thus, it’s worth considering in applications where execution speed and memory optimization are critical.
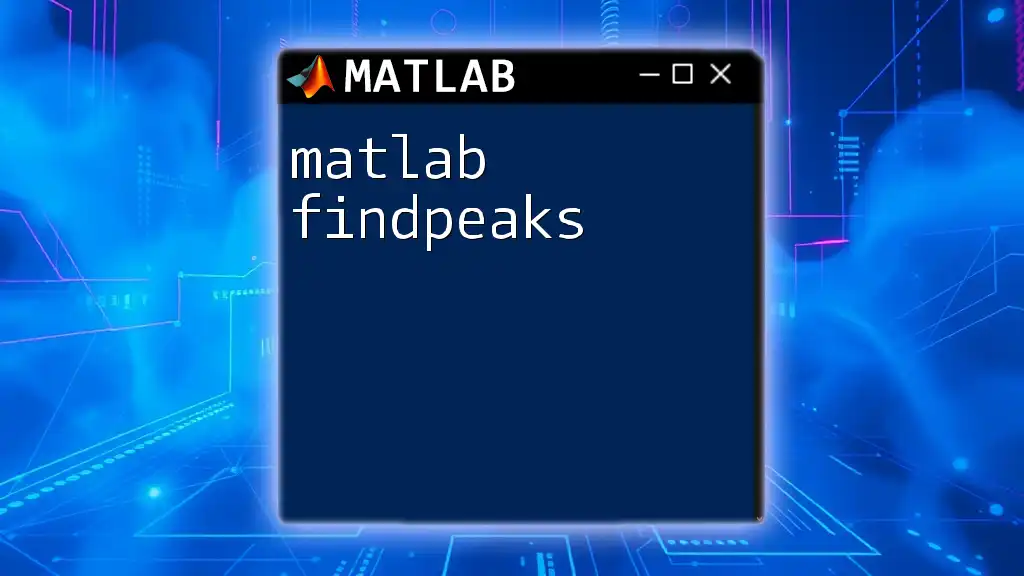
Common Errors and Troubleshooting
Potential Issues with linspace
While linspace is generally straightforward to use, common mistakes include:
- Omitting the number of points: Failing to specify n can lead to unexpected results. It’s crucial to recall that MATLAB defaults to generating 100 points, which may not suit all applications.
- Incorrect input types: Ensure that the start and end values are numeric. Misconfigured input can lead to runtime errors.
Debugging Techniques
If you encounter issues while utilizing linspace, these strategies may help:
- Check the output: Print the output vector to examine its structure and ensure it meets your expectations.
- Validate input types: Use the `class()` function in MATLAB to ensure that input values are numeric.
- Use `size()`: This function can help verify the dimensions of generated vectors during debugging sessions.
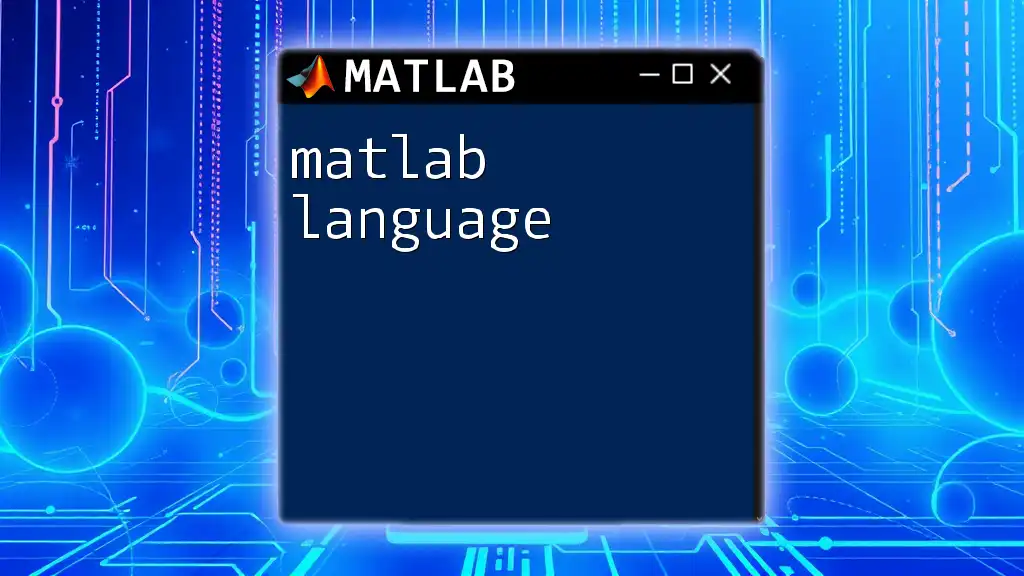
Conclusion
The MATLAB linspace function is an invaluable tool for anyone engaged with numerical computations, modeling, or graphical representations of data. Understanding how to effectively leverage linspace can drastically enhance your efficiency and the quality of your analyses.
From generating smooth plots to simulating complex models, linspace plays a central role in MATLAB operations. Practicing with linspace across different scenarios will not only solidify your grasp of its functionality but also inspire creativity in your data exploration tasks.
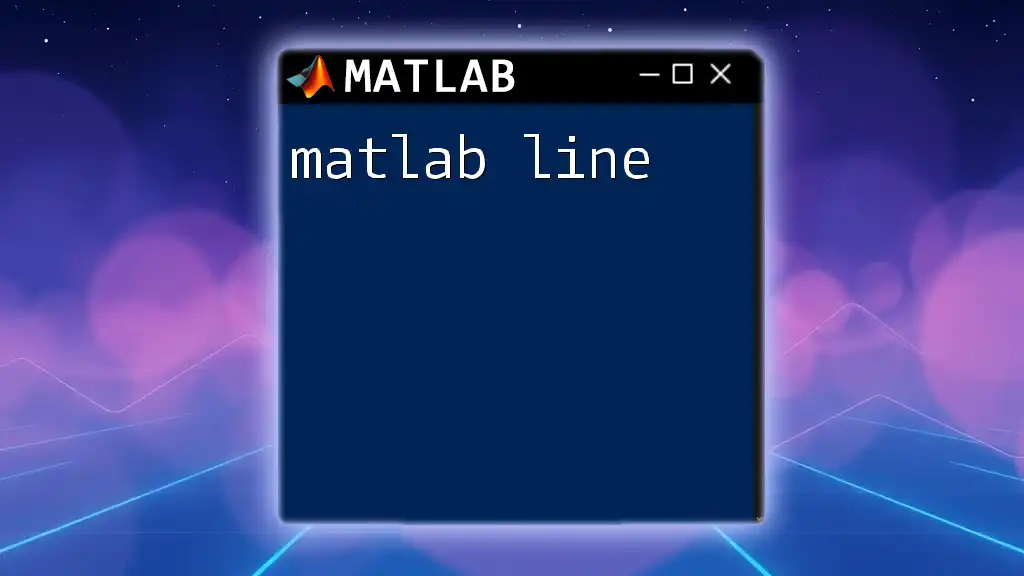
Additional Resources
For further exploration, consider diving into the MATLAB documentation, which provides thorough insights into the linspace function. You can also engage with various tutorials and communities dedicated to MATLAB, where you can deepen your understanding and connect with fellow learners.
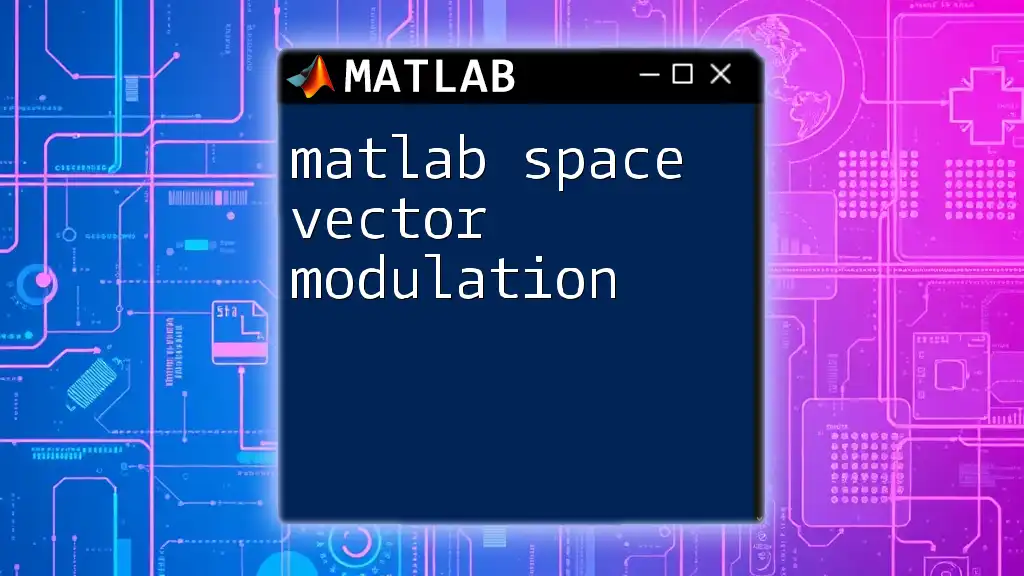
Call to Action
I encourage you to practice using linspace in your projects, whether in plotting or simulations. Additionally, consider joining my company’s upcoming MATLAB workshops to gain insights and methodologies for mastering critical MATLAB commands in an efficient and effective manner.