The `ginput` function in MATLAB allows users to interactively select points from a figure using mouse clicks, returning the coordinates of the selected points.
% Example of using ginput to get three points from a plot
figure; % Create a new figure
plot(rand(10)); % Plot some random data
[x, y] = ginput(3); % Get three points from the user
disp([x, y]); % Display the coordinates of the selected points
What is ginput?
ginput is a powerful function in MATLAB that serves as an interface for obtaining user inputs through mouse clicks on a graphical figure. It allows users to interactively select points in a plot, which can be particularly useful in a variety of applications, such as data collection, graphical analysis, and simple user interface design.
Use Cases of ginput
-
Collecting Data Points from Plots: Researchers and engineers often need to gather specific data points from graphical outputs. The `ginput` function streamlines this process by allowing users to click on the desired points directly on the plots.
-
Interactive Figure Design: Designers can utilize `ginput` to enable interactivity in their visualizations, helping users focus on points of interest.
-
Simple GUI Applications: `ginput` can serve as a foundational element for basic graphical user interfaces, enhancing the user experience by allowing real-time input.
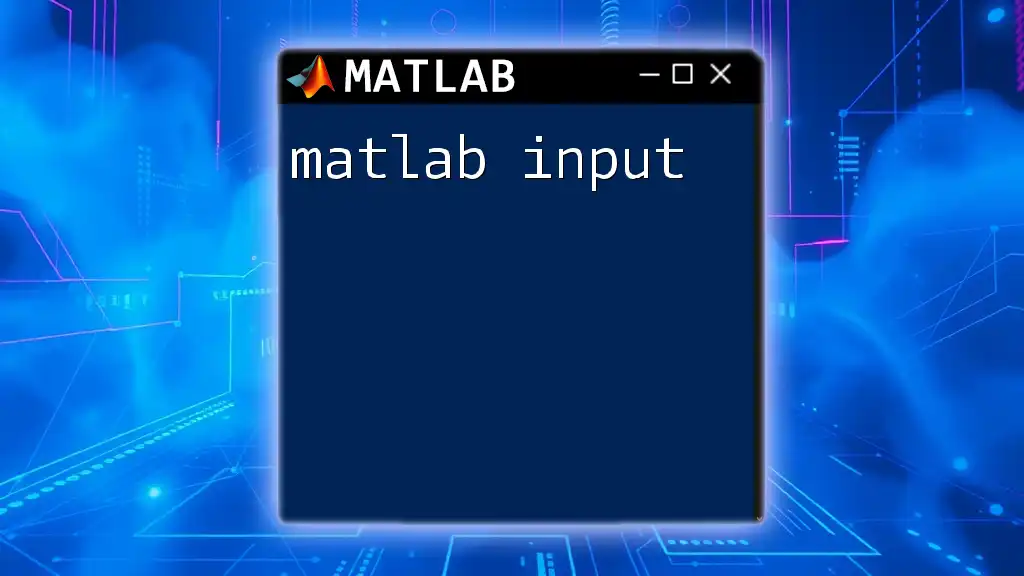
How ginput Works
Basic Functionality
The fundamental syntax of the `ginput` function is:
xy = ginput(n)
In this syntax, `n` represents the number of points you wish to capture. If `n` is omitted, `ginput` will wait for an indefinite number of inputs until the user cancels with the keyboard.
Parameters and Outputs
Parameters Explained
- n: The number of points you want to capture from the user. Setting `n` allows you to limit the number of inputs, which can be useful in controlled experiments or specific applications.
Output of ginput
The `ginput` function returns `xy`, which is an array containing the x and y coordinates of the selected points. Each row corresponds to a point selected by the user, making it easy to work with the data in subsequent analysis or visualization steps.
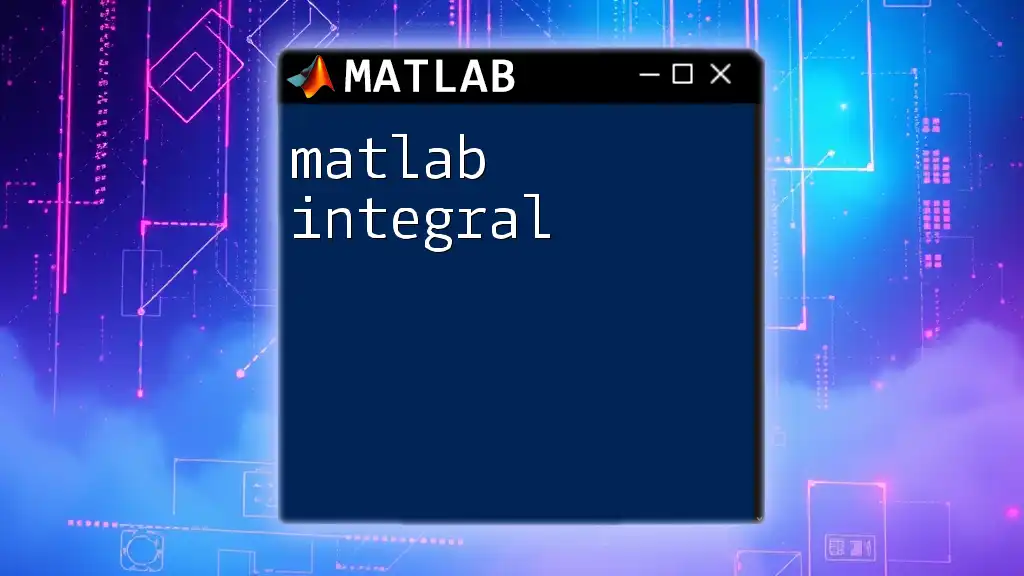
Setting Up for ginput
Creating a Simple Plot
Before you can use `ginput`, you need to set up a figure to capture the inputs. Here’s a code snippet to create a basic plot:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
title('Click on the plot to select points');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
Each line in the code plays a significant role:
- `x` and `y` define the data: This creates a range of x-values and their corresponding sine values.
- The `plot` function generates a visual representation of that data.
- The `title`, `xlabel`, and `ylabel` functions add context to the figure, clarifying what the audience should be looking for.
- The `grid on` command enhances visibility, making it easier for users to pinpoint their selections.
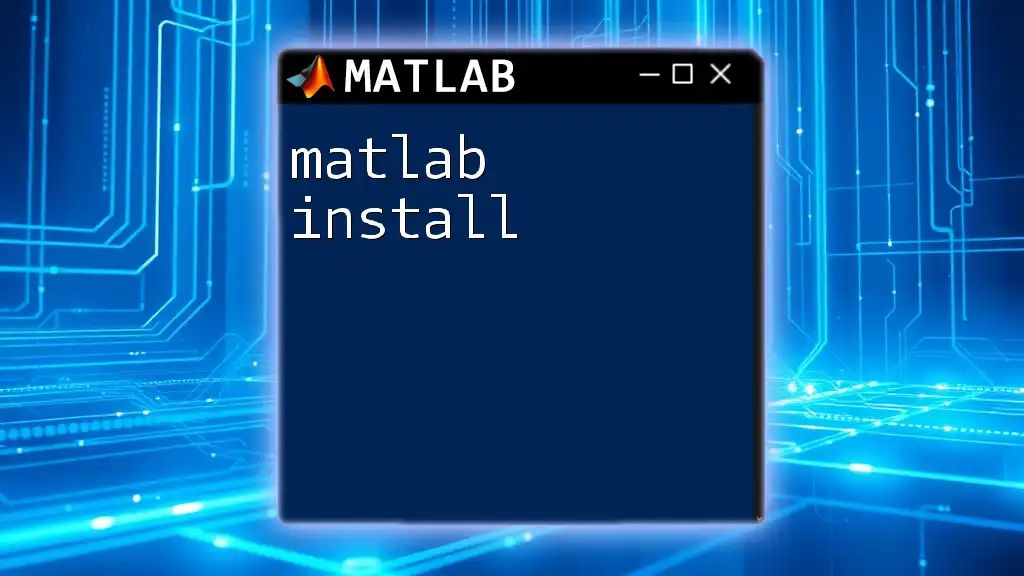
Using ginput in Practice
Capturing Points with ginput
Once your plot is ready, you can implement `ginput` to capture specific points. The following example captures two points clicked by the user:
[x_coords, y_coords] = ginput(2);
disp('You selected the following points:');
disp([x_coords, y_coords]);
- Here, the program will wait for the user to click exactly two points on the plot.
- The user's selections are stored in `x_coords` and `y_coords`, which are arrays representing the x and y coordinates of the points clicked.
- The `disp` function outputs the selected coordinates in the Command Window, ensuring users can double-check their selections.
Visualizing User Selections
After collecting the points, it’s helpful to visualize them on the original plot to confirm accuracy. You can modify the plot with the following code:
hold on;
plot(x_coords, y_coords, 'ro', 'MarkerSize', 10); % Red circles at selected points
hold off;
Using `hold on` allows you to overlay the selected points without losing the original plot. The `plot` function is called again to display the selected coordinates as red circles, with an increased marker size for visibility. Using `hold off` afterward ensures that any future plotting commands will not overlay new data on the existing figure.
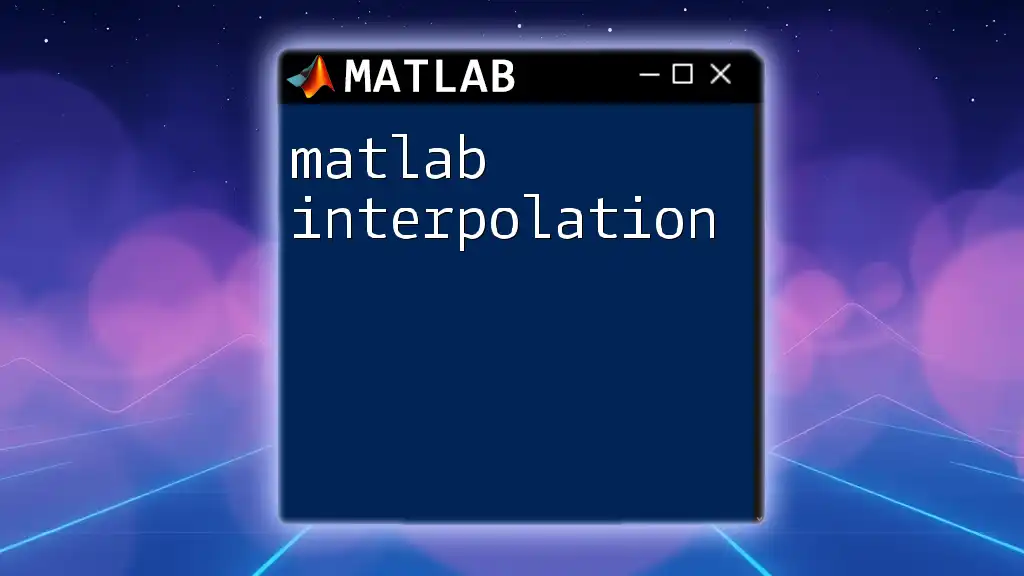
Advanced Use of ginput
Continuous Input Capture
In some situations, it may be preferable to allow for continuous user input until they decide to stop. You can use `ginput` without specifying the number of clicks like this:
disp('Select points (Press Enter to stop):');
[x_coords, y_coords] = ginput; % No limitation on number of inputs
This code snippet releases the user from the constraints of a fixed point count, creating intuitive data gathering while users navigate through the plot freely.
Customizing ginput Behavior
An advanced feature of `ginput` is the ability to handle user input more elegantly. Users can press the Enter key to signal they're done selecting points, allowing for a flexible interaction. Familiarizing yourself with how to manage keyboard shortcuts can enhance user experience significantly; consider displaying instructions directly on the plot for clarity.
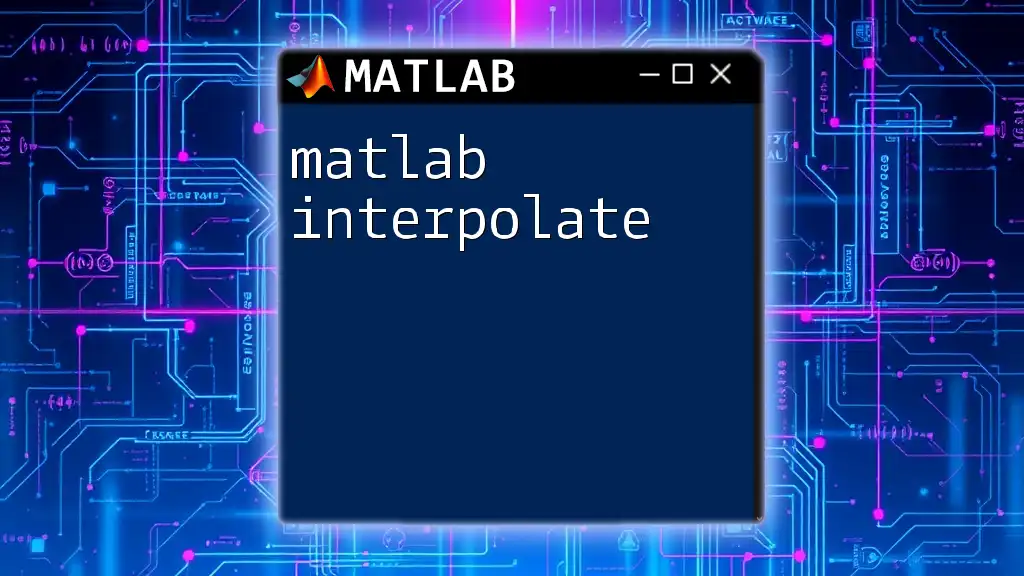
Tips for Effective Use of ginput
To maximize the efficacy of the `ginput` function:
-
Make Plots User-Friendly: Ensure that titles and labels are clear and informative. Giving users clear instructions on how to select points can make a significant difference.
-
Enhance Visibility: Use grid lines and contrasting colors to help users identify where they are clicking.
-
Avoid Common Pitfalls: Ensure the figure remains open during use; if the figure is closed, `ginput` will not be able to capture any more points.
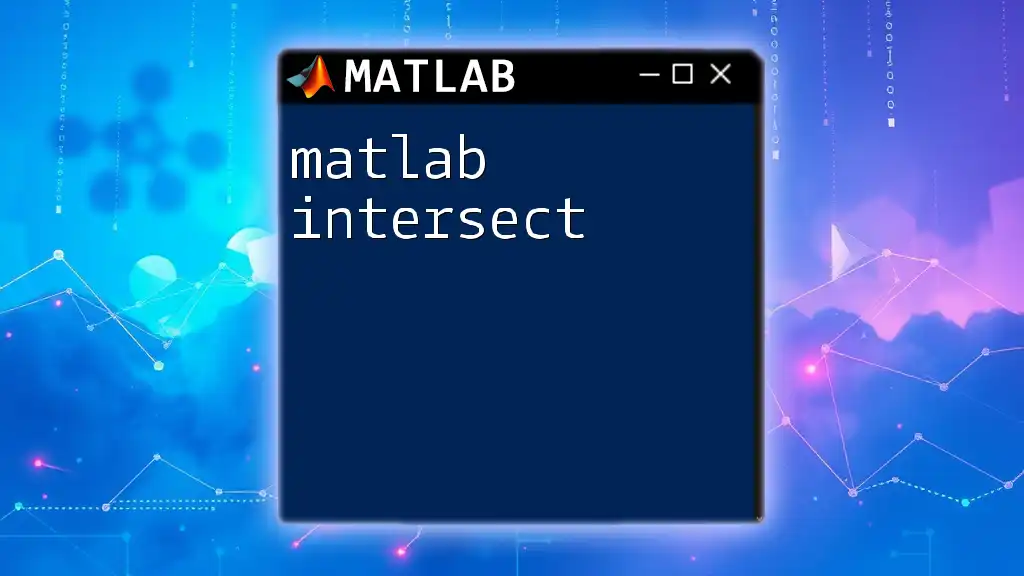
Troubleshooting Common Issues
There are several common issues that users may encounter when working with `ginput`:
-
Figure Closing Prematurely: If the figure window is closed accidentally, `ginput` will throw an error. Make sure to instruct users not to close the window until they are done interacting.
-
Error Handling: Consider implementing a try-catch block around your `ginput` calls to handle unexpected inputs gracefully:
try
[x_coords, y_coords] = ginput(2);
catch
disp('Operation canceled or an error occurred');
end
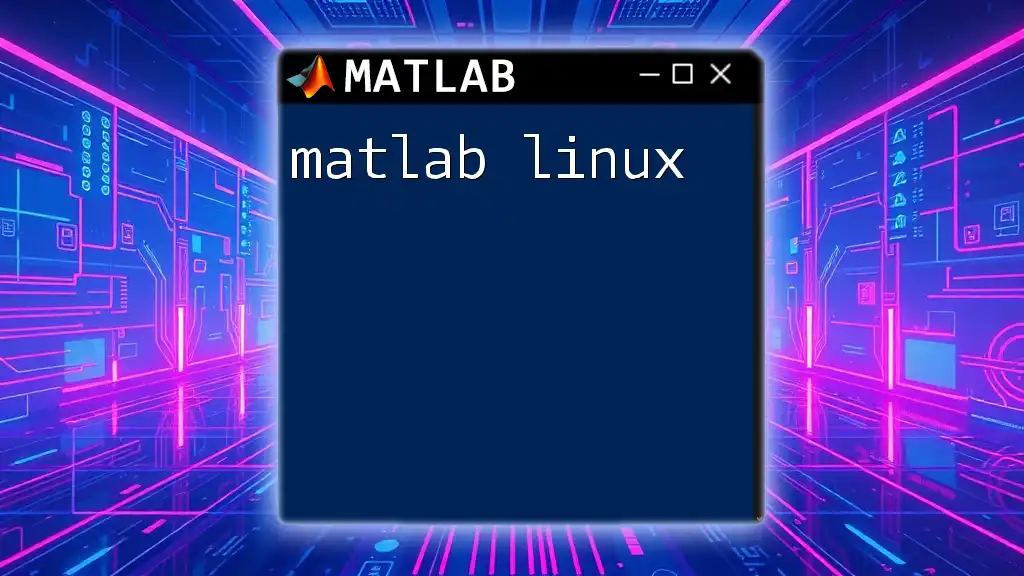
Conclusion
The `ginput` function in MATLAB is an invaluable tool that allows for seamless interaction with graphical data. From simple data collection to enhanced user experience in applications, mastering `ginput` empowers users to engage effectively with their visualizations. Continuous practice and experimentation with `ginput` will lead to greater proficiency and creativity in your MATLAB applications.
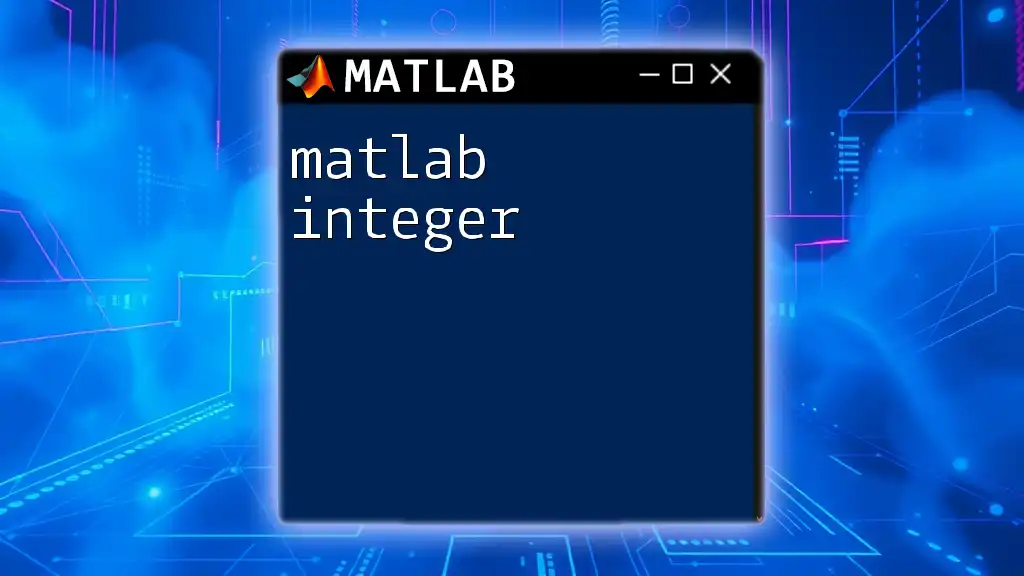
Additional Resources
For further learning, consider exploring the official MATLAB documentation on `ginput`, as well as recommended video tutorials that provide practical demonstrations of the function in action.