In MATLAB, the `max` function is used to find the largest element in an array or matrix, and it can also return the indices of the maximum values.
Here’s a code snippet demonstrating how to use the `max` function:
% Example of finding the maximum value and its index in an array
A = [3, 5, 1, 7, 2];
[maxValue, index] = max(A);
disp(['Maximum Value: ', num2str(maxValue), ', Index: ', num2str(index)]);
Understanding the Maximum Function in MATLAB
The `max` Function
The `max` function in MATLAB is designed to determine the maximum value in an array. It plays a crucial role in data analysis, optimization, and mathematical modeling.
Basic Syntax of the `max` Function
The general form of the `max` function is straightforward:
maxValue = max(A)
This command computes the maximum value in the array `A`.
Example:
% Example of using max with a vector
A = [3, 5, 2, 8, 6];
maxValue = max(A); % maxValue will be 8
In this scenario, the maximum value found within the vector `A` is 8.
Types of Input for the `max` Function
Vector Inputs
When `max` is applied to a vector, it returns the single maximum value. This operation is quite intuitive and efficient in MATLAB, allowing users to quickly identify the largest element in a one-dimensional array.
Example:
A = [1, 2, 3, 4];
fprintf('The maximum value is: %d\n', max(A)); % Output: 4
Here, the maximum value is 4.
Matrix Inputs
When dealing with matrices, MATLAB's `max` function behaves differently; it evaluates the maximum along each column by default.
Example:
B = [1, 2, 3; 4, 5, 6];
rowMax = max(B); % Returns maximum for each column
This will yield a vector containing the maximum values for each column:
- Column 1: 4
- Column 2: 5
- Column 3: 6
To obtain the maximum values across each row, use the second argument to specify the dimension:
colMax = max(B, [], 1); % Maximum for each column
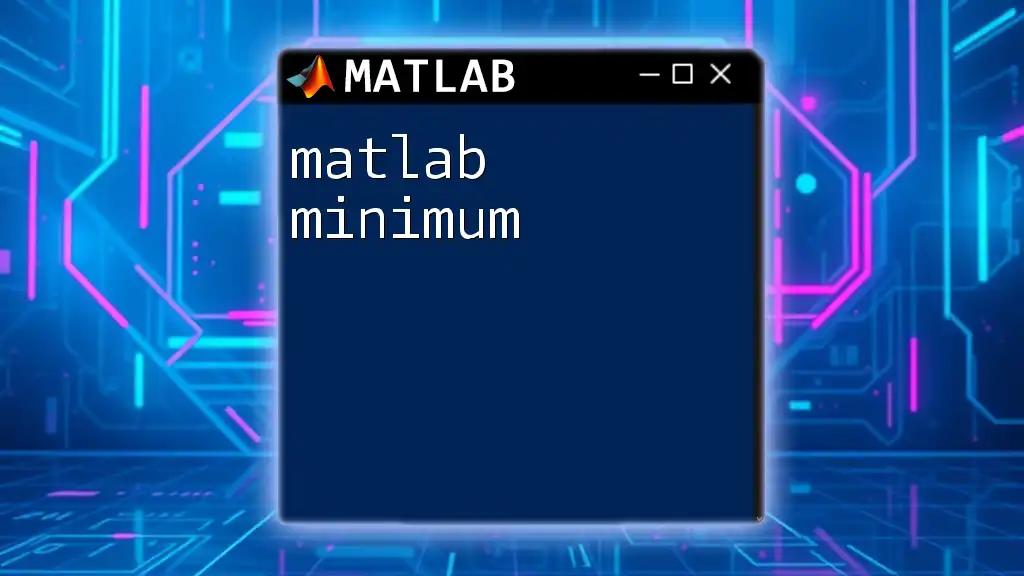
Advanced Usage of the `max` Function
Returning Indices of Maximum Values
Often, it’s helpful to know not just the maximum value but also where it resides in the array. For this, MATLAB provides a second output argument in the `max` function.
Example:
[maxValue, index] = max(A);
fprintf('Max value: %d at index %d\n', maxValue, index);
In this instance, if `A = [3, 5, 2, 8, 6]`, the output will show "Max value: 8 at index 4".
Applying `max` to Multi-Dimensional Arrays
MATLAB can also handle multi-dimensional arrays effectively. The `max` function can maximize values over specified dimensions, allowing users to maintain control over their calculations.
Example:
C = rand(3, 4); % Creates a 3x4 matrix of random numbers
maxRow = max(C, [], 1); % Max across each column
maxCol = max(C, [], 2); % Max across each row
In this case, users can easily fetch the maximum values column-wise and row-wise by setting the appropriate dimension argument.

Practical Applications of Finding Maximums in MATLAB
Optimizing Functions
The `max` function can be integrated into optimization problems where finding maximum values of functions is critical. MATLAB provides powerful optimization functions such as `fminunc` and `fmincon` to help achieve this.
Example:
fun = @(x) -1*(x(1)-2)^2 - (x(2)-3)^2; % Define the function to maximize
x0 = [0, 0]; % Initial guess
options = optimoptions('fminunc', 'Display', 'off');
[xMax, fval] = fminunc(fun, x0, options);
In this function maximization scenario, a negative of the quadratic function is constructed to find its peak, leading to optimized outputs in variable `xMax`.
Real-World Scenarios
Finding maximum values has real-world applications, especially in fields like finance. For example, analysts might want to study the highest stock price over a specific period. Using `max`, analysts can efficiently analyze large datasets and derive strategies based on peak performance.

Best Practices in Using `max` in MATLAB
Efficiency Considerations
- Use Built-in Functions: Whenever possible, leverage built-in MATLAB functions like `max`, as they are optimized for performance.
- Preallocate Arrays: Minimize memory overhead by preallocating arrays before using `max` in loops or iterations.
Common Pitfalls and How to Avoid Them
- Mismatched Dimensions: When using `max`, ensure the dimensions are correctly handled to avoid unexpected results.
- Data Types: Be aware of the data types; for instance, `max` may behave differently on strings compared to numerical arrays.
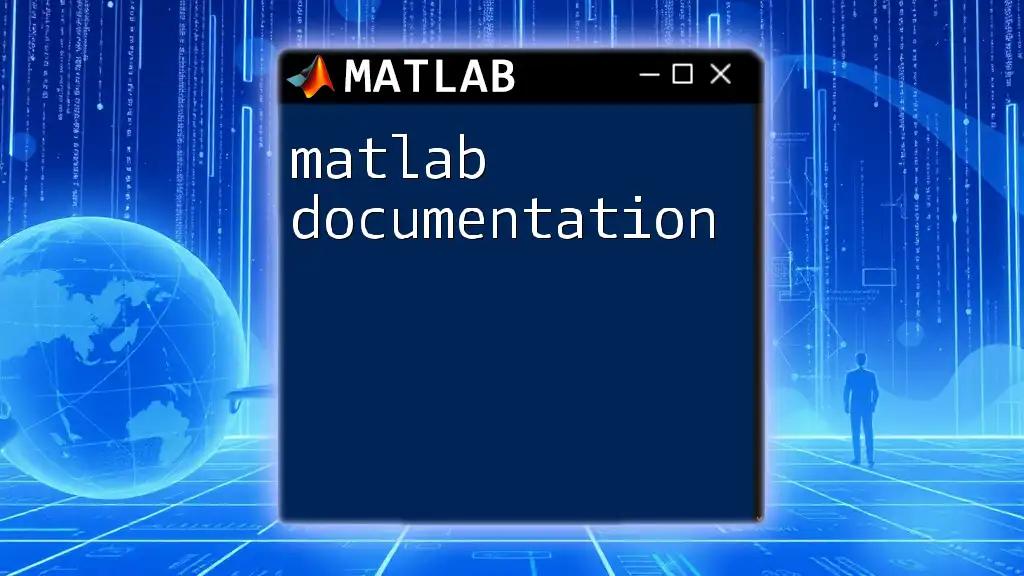
Conclusion
The `max` function in MATLAB is a cornerstone for data analysis, optimization, and mathematical operations. Understanding its applications, syntax, and nuances enables users to leverage its full potential in their computations. Whether you're optimizing a function or analyzing financial data, mastering the various forms of the matlab maximum function is invaluable for efficient programming.
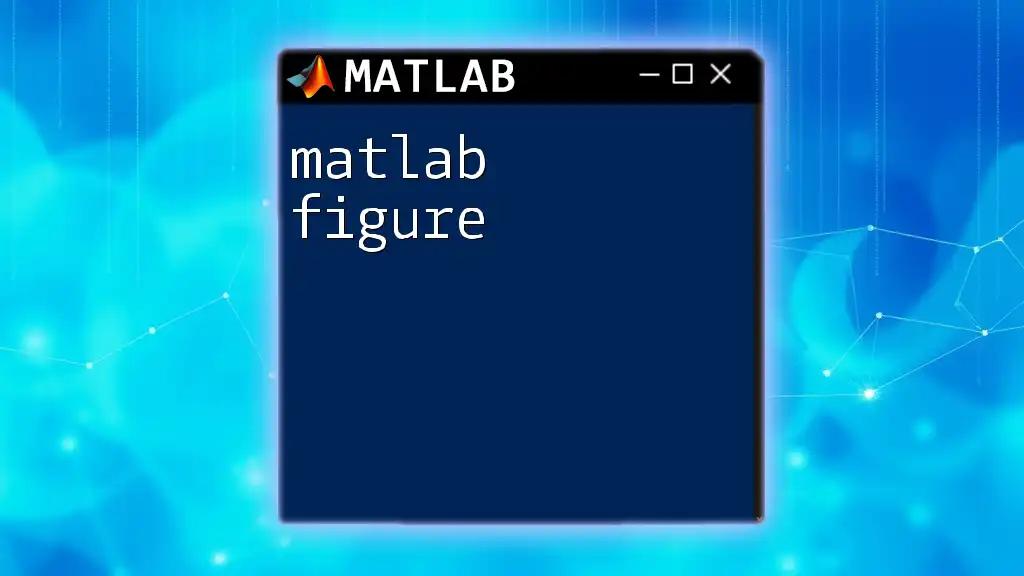
Frequently Asked Questions
What is the difference between `max` and `min` in MATLAB?
The `max` function retrieves the maximum value in an array, while the `min` function finds the minimum value with a similar syntax structure.
Can the `max` function handle complex numbers?
Yes, the `max` function can handle complex numbers. When applied, it evaluates the maximum based on the magnitude of the complex numbers.
How to handle errors when using the `max` function?
Common errors often stem from dimension mismatch or incompatible data types. Ensure your input arrays are appropriately sized and typed for smooth execution.