In MATLAB, `NaN` (Not a Number) is a placeholder used to represent undefined or unrepresentable numerical values, such as the result of 0/0.
Here’s a basic example of how to create and check for `NaN` values:
% Creating a NaN value
nanValue = NaN;
% Checking if the value is NaN
isNan = isnan(nanValue); % This will return true
Understanding NaN in MATLAB
Definition of NaN
In MATLAB, NaN stands for Not a Number. It represents an undefined or unrepresentable value in numerical calculations. This term is prevalent in data analysis, particularly when dealing with datasets that contain missing or invalid entries. Understanding NaN is crucial, as it affects the results of mathematical operations and statistical computations.
How MATLAB Handles NaN
MATLAB follows the IEEE 754 standard for floating-point computations. According to this standard, NaN is used to denote values that cannot be represented as real numbers. It's essential to differentiate between NaN and other special values:
- Inf: Represents positive infinity.
- -Inf: Represents negative infinity.
These distinctions are vital for maintaining the integrity of calculations and analyses involving real-world data.
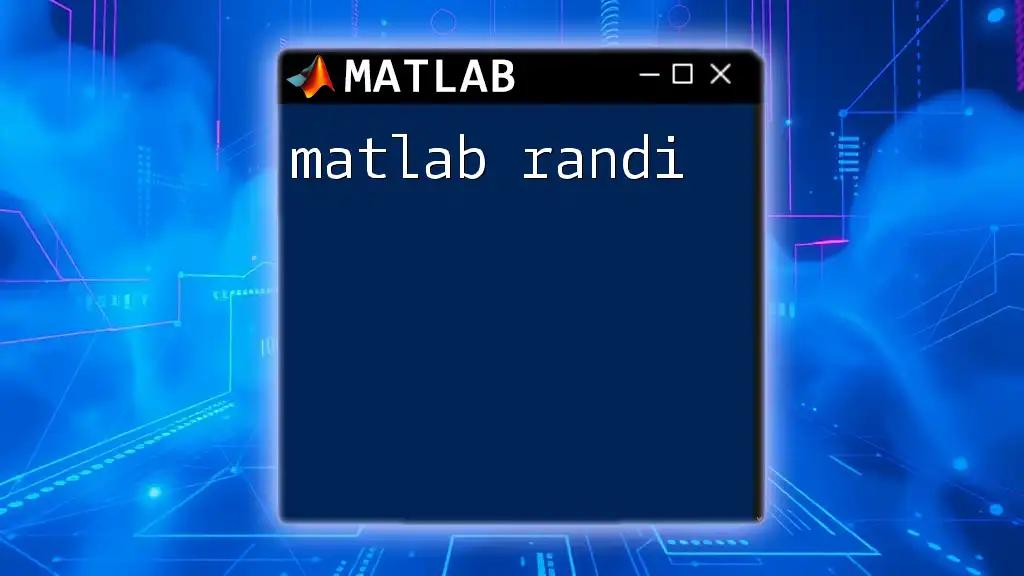
Creating NaN in MATLAB
Explicitly Assigning NaN
You can create a NaN value simply by using the `NaN` keyword in MATLAB. This makes it easy to initialize variables that you intend to assign with a non-existent or unavailable numeric value.
myNaN = NaN;
In this example, `myNaN` is assigned the value NaN, denoting that it has not been calculated or is missing.
Generating NaN in Arrays
NaN values can also be included in MATLAB arrays. This is useful for initializing datasets that may later contain missing values.
A = [1, 2, NaN, 4];
Here, `A` is an array that includes a NaN element. Understanding how to represent NaN in data structures is essential for effective data handling.
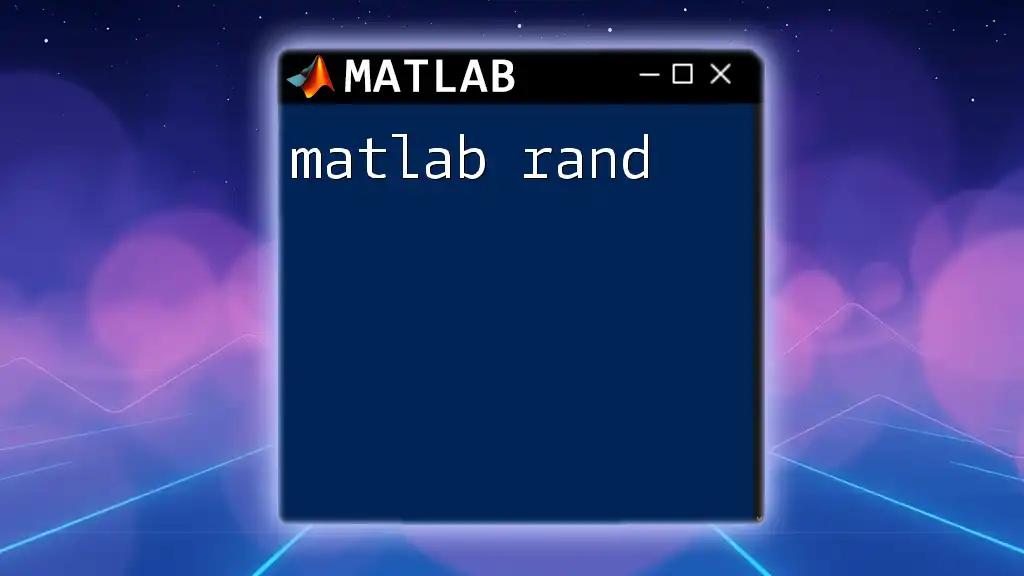
Detecting NaN in MATLAB
Using the `isnan` Function
The `isnan` function is a built-in MATLAB function designed to identify NaN values within arrays. This function returns a logical array, where each element indicates whether the corresponding element in the original array is NaN.
A = [1, 2, NaN, 4];
nanFlags = isnan(A);
In this snippet, `nanFlags` will contain the values `[false, false, true, false]`, allowing you to pinpoint where the NaN values are located.
Logical Indexing with NaN
MATLAB allows for logical indexing to filter out NaN values from datasets effectively. Using the `isnan` function in combination with logical operators proves beneficial for data cleaning.
validData = A(~isnan(A));
In this example, `validData` will contain only the numeric values from `A`, effectively removing the NaN value. This step is crucial in preparing your data for analysis.

Handling NaN in Data Analysis
Removing NaN Values from Data
When analyzing data, it's often important to consider how NaN values affect your results. Removing NaN values ensures that the analyses performed on your datasets are meaningful and that the conclusions drawn are not influenced by missing data.
B = A(~isnan(A));
In this case, `B` will now hold an array of values stripped of NaN entries. This is a common practice when preparing datasets for statistical analysis.
Summarizing Data with NaN
Several MATLAB functions, such as `sum` and `mean`, directly interact with NaN values. By default, if a NaN value is present, these functions will return NaN as the result, which may lead to misleading conclusions.
totalSum = sum(A); % returns NaN
meanValue = nanmean(A); % requires Statistics Toolbox
The first line will return NaN, while the second line demonstrates how to calculate the mean while ignoring NaN values. Using nan prefixed functions (e.g., `nanmean`, `nansum`) is crucial for proper data summarization when NaN values exist.
Replacing NaN with Other Values
Instead of removing NaN values, there are instances where it may be beneficial to replace them with a defined value, such as zero or the mean of the dataset. This substitution can help maintain dataset size and usability.
A(isnan(A)) = 0; % replacing NaNs with zeros
While replacing NaNs is a valid approach, it's important to recognize the implications. For instance, setting missing values to zero could distort the data's original meaning. Careful evaluation is required to determine the best approach for your specific analysis.
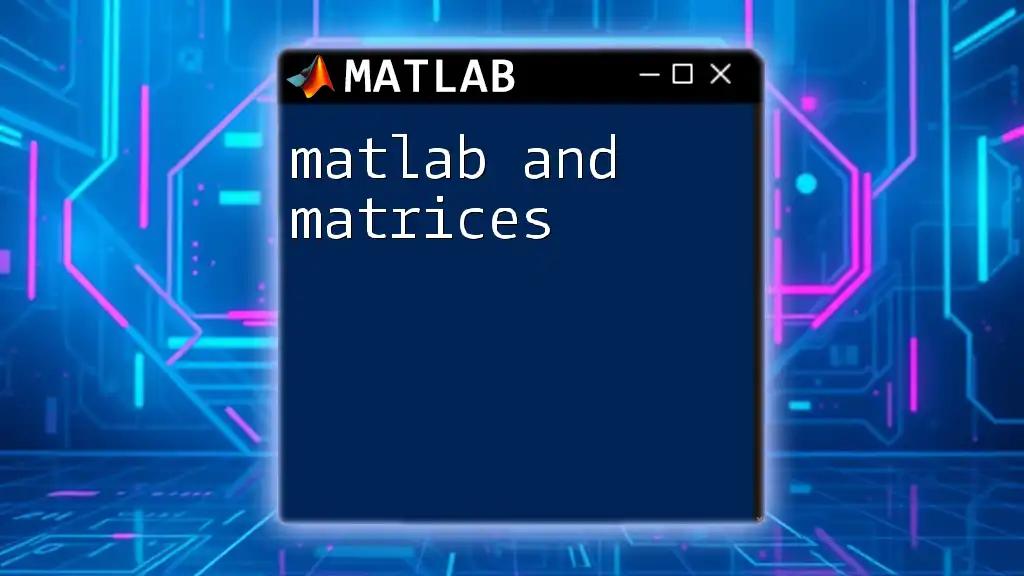
Best Practices for Working with NaN
Data Cleaning Procedures
Effective data cleaning processes are essential when working with NaN values. Start by identifying and handling NaN entries early in your analysis workflow. Documenting how you handle NaNs ensures transparency and reproducibility in your research or projects.
Project Considerations
When collaborating on projects involving datasets with NaN values, communication is key. Always inform your collaborators about the presence of NaN values and how you plan to handle them. This will help in fostering a shared understanding and reducing the risk of misinterpretation of results.

Conclusion
In summary, understanding MATLAB NaN is crucial for anyone involved in data analysis. By mastering the creation, detection, and handling of NaN values, you can enhance the integrity and reliability of your analyses. Recognizing the impact of NaN on computations allows for more informed decision-making in your data-driven projects.