In MATLAB, the `rand` function generates uniformly distributed random numbers, and can be used to create matrices of specified dimensions with random values between 0 and 1. Here's a simple example:
% Generate a 3x3 matrix of random numbers
randomMatrix = rand(3);
Understanding Random Number Generators
What is a Random Number Generator (RNG)?
A Random Number Generator (RNG) is an algorithm designed to produce a sequence of numbers that lack any pattern, simulating the unpredictability associated with random phenomena. In computing, we primarily work with pseudo-random numbers due to their reproducible nature. True random numbers may derive from physical processes, but for most computational purposes, the pseudo-random generation suffices.
Creating Randomness with MATLAB's RNG
MATLAB uses a specific algorithm as its default RNG, which is both efficient and effective for generating random numbers. One critical aspect of RNGs is the seed value. By explicitly setting the seed, researchers and developers can ensure that their experiments or simulations yield consistent results, essential for reproducibility in scientific research.
For example, setting the seed can be done easily:
rng(1); % Sets the seed for reproducibility
This command ensures that every time you run your script with `rng(1)`, MATLAB generates the same sequence of random numbers.
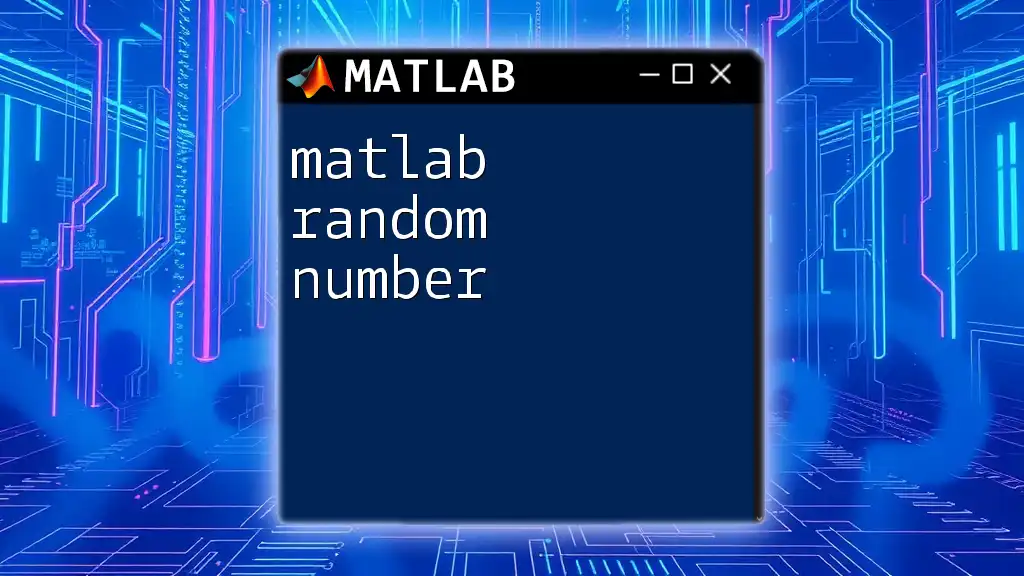
MATLAB Random Number Functions
Simple Random Number Generation
Generating Uniform Random Numbers
MATLAB provides a simple function, `rand()`, which generates uniform random numbers in the interval (0, 1). This is particularly useful when you require a baseline for randomization.
For instance, a simple call to:
random_num = rand();
will yield a single random number between 0 and 1.
Generating Normally Distributed Random Numbers
In many computational applications, you might find the need for numbers drawn from a normal distribution (Gaussian). The `randn()` function accomplishes this, generating numbers with a mean of 0 and a standard deviation of 1.
Here's how you can generate 5 normally distributed random numbers:
normal_random = randn(1, 5); % Generates 5 random numbers
This output is typically useful in simulations and when modeling natural phenomena, which often exhibit normal distribution characteristics.
Custom Range Random Numbers
Uniform Distribution in a Specific Range
In many cases, the default range of (0, 1) is not sufficient. You can easily customize the generation of uniform random numbers within a specific range using the formula:
random_range = a + (b-a) * rand(1, 10); % Generates 10 numbers between a and b
This example effectively scales and shifts the uniform distribution to fit your desired limits of a and b.
Normal Distribution with Specified Mean and Standard Deviation
If you want to draw samples from a normal distribution with a specific mean (mu) and standard deviation (sigma), you can use:
mu = 5; % Mean
sigma = 2; % Standard deviation
normal_custom = mu + sigma * randn(1, 10); % Generates 10 numbers
This is particularly useful for simulations that require normal disturbances with specific characteristics.
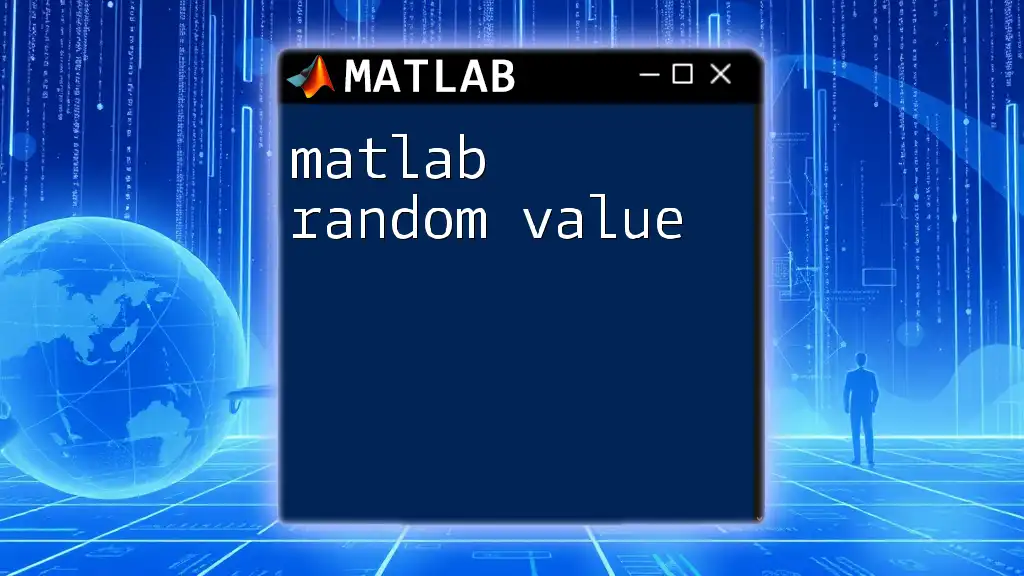
Random Sampling Techniques in MATLAB
Random Sampling from Arrays
When you have a dataset and you need to sample from it, MATLAB’s `randsample()` function becomes invaluable. It allows you to randomly select a number of elements from an array while providing the option for replacement.
Here’s how to create a random sample from the array of numbers 1 to 100:
data = 1:100;
sample = randsample(data, 10); % Sample 10 elements
Sampling With Replacement and Without Replacement
When sampling without replacement, every selected element is removed from the pool for subsequent selections, ensuring unique samples. Conversely, sampling with replacement allows the same element to be chosen multiple times. Understanding these concepts is essential for proper data analysis.
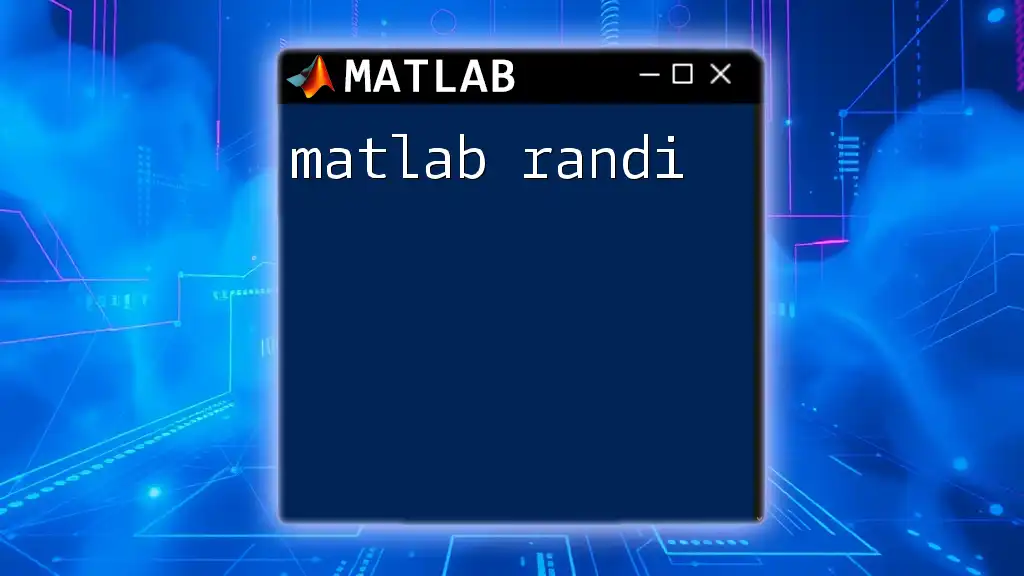
Operations with Random Numbers
Statistical Analysis of Random Numbers
Once you have generated random data, analyzing it statistically is a crucial step. For example, you can calculate the mean, median, and variance easily using MATLAB’s built-in functions.
Consider a set of 100 random numbers:
data = randn(1, 100); % Generate 100 random numbers
mean_value = mean(data);
median_value = median(data);
variance_value = var(data);
The above code will give you insights into your random data’s distribution, which is often a key aspect of understanding behavior in simulations.
Visualizing Random Data
Visualizations such as histograms and boxplots can convey significant insights. Using MATLAB, you can visualize the distribution of random numbers quickly and effectively:
histogram(data); % Creates a histogram of the data
boxplot(data); % Creates a boxplot of the data
These plots allow you to detect patterns, outliers, and the overall distribution shape of your random data.
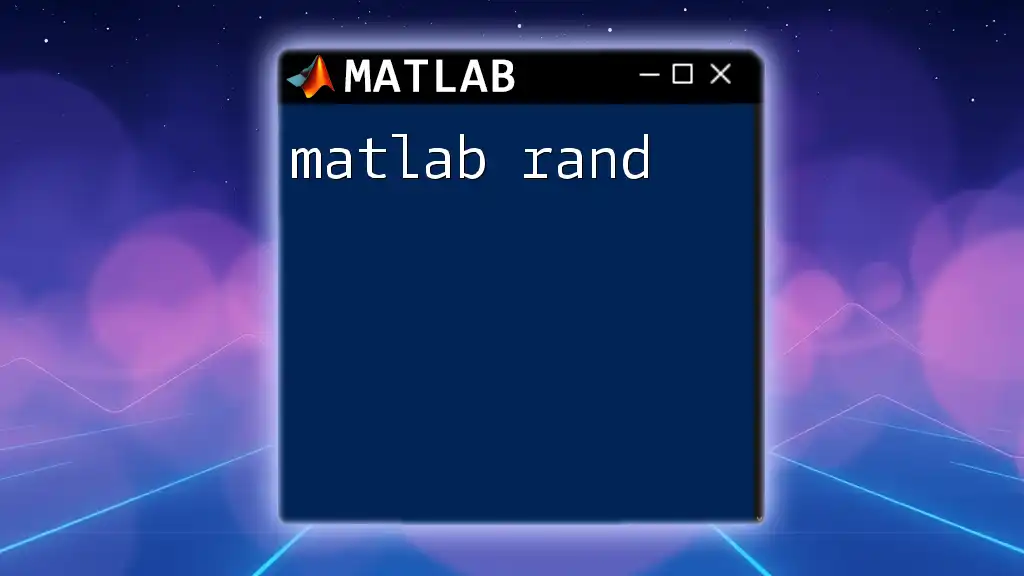
Advanced Random Functions
Random Number Streams
MATLAB enables the creation of random number streams for specific purposes. Streams allow for separate sequences of random numbers, which can be useful for different simulations or processes happening concurrently. You can create a random stream using:
stream = RandStream('mt19937ar', 'Seed', 1);
random_stream = rand(stream, 1, 10); % Generates 10 random numbers from the stream
This ensures that you have complete control over the random number generation processes without interference.
Using `randperm()` for Random Permutations
In addition to random sampling, you may need to generate a randomized order of elements. The `randperm()` function provides this functionality. It generates a random permutation of integers.
Here’s an example:
permuted_array = randperm(20); % Random permutation of numbers 1-20
This is particularly useful in experimental designs and random assignment procedures.
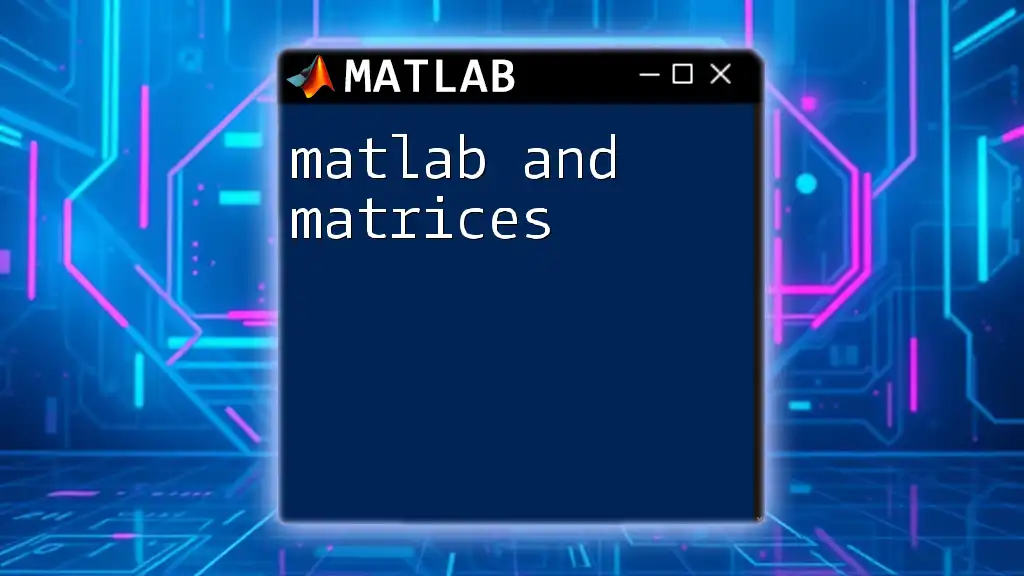
Common Errors and Best Practices
Storage and Data Types
When working with large datasets, consider how to store and manipulate data effectively to enhance performance. Choosing appropriate data types can have significant impacts on memory usage and computation speed.
Reproducibility Issues
One of the more common pitfalls involves reproducibility. It’s essential to remember the use of seeds when generating random numbers. If you don't set the seed consistently, subsequent runs of your algorithm will yield different results, which can complicate debugging and validation processes.
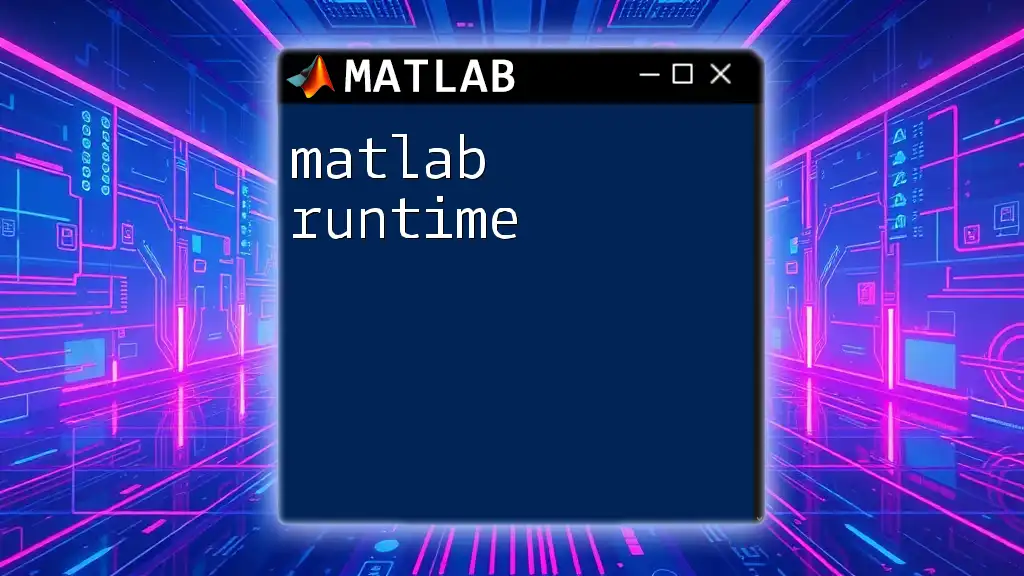
Conclusion
In summary, the MATLAB random functions provide a powerful suite of tools for generating, analyzing, and sampling random numbers. From basic uniform distributions to advanced sampling techniques, MATLAB equips users with everything necessary to harness the concept of randomness effectively in various applications.
By understanding and utilizing these functions, you have the foundation needed to apply randomness in simulations, model uncertainties, and conduct statistical analyses, making it an essential skill in both programming and data science.
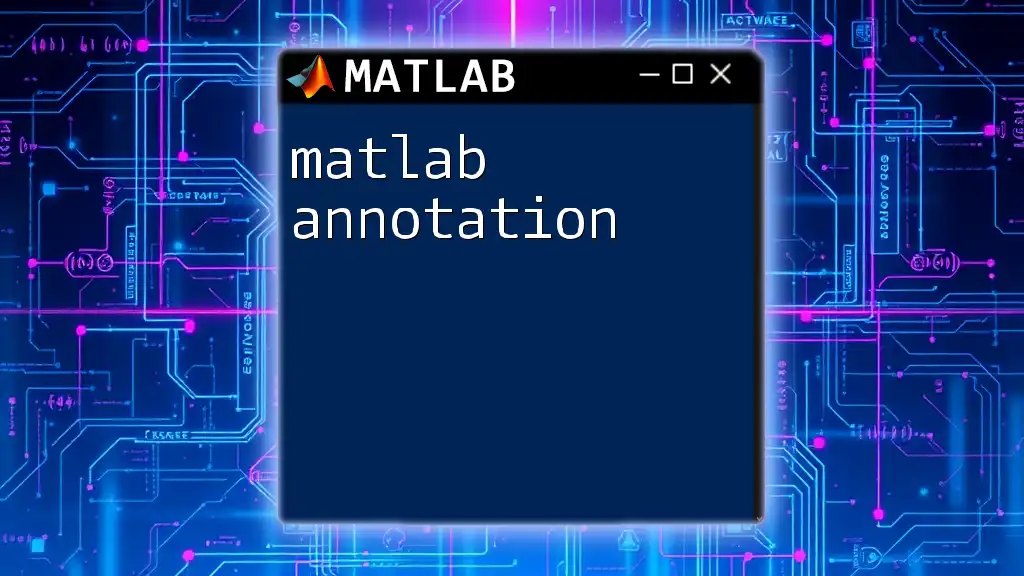
Additional Resources
For further exploration, consider checking out the official MATLAB documentation and recommended online courses that delve deeper into random number generation, statistics, and data analysis techniques.
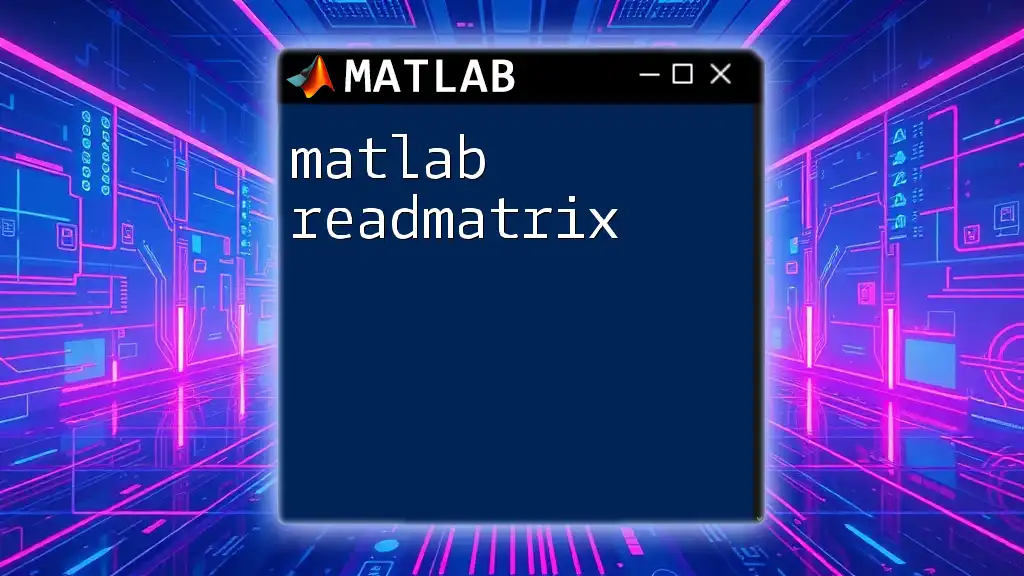
Frequently Asked Questions (FAQ)
What is the difference between `rand()` and `randn()`?
The `rand()` function generates random numbers uniformly distributed in the interval (0, 1), whereas `randn()` produces numbers from a standard normal distribution (mean of 0 and standard deviation of 1).
How can I ensure my random numbers are truly random?
While MATLAB primarily generates pseudo-random numbers, setting a unique seed each time ensures different sequences. For true randomness, consider incorporating hardware randomness sources in external applications.
Can I simulate a random walk in MATLAB?
Yes! You can simulate a random walk using cumulative sums of generated random steps. For instance:
steps = randi([-1, 1], 1, 100); % Generate 100 random steps
random_walk = cumsum(steps); % Cumulative sum for the walk
plot(random_walk); % Plotting the random walk
This script visualizes the path of the random walk generated by sum cumulatively across the selected random steps.