`nargin` is a MATLAB function that returns the number of input arguments provided to a function, allowing for flexible handling of different inputs.
Here’s a simple example demonstrating its use:
function myFunction(varargin)
numInputs = nargin;
fprintf('Number of inputs: %d\n', numInputs);
end
Understanding Function Inputs
What are Function Inputs?
Function inputs are the parameters that you pass to a MATLAB function. They are essential for the function to perform its task. There are two primary types of inputs:
- Required inputs must be provided for the function to execute correctly.
- Optional inputs can enhance the function's flexibility and user-friendliness, allowing for default values or varied behavior based on user requirements.
Why Manage Input Arguments?
Managing input arguments is vital in several scenarios. It allows you to:
- Enhance the flexibility of your functions, making your code usable in various contexts without rewriting.
- Avoid runtime errors that occur when the function does not receive the expected number or type of inputs, thus improving the robustness and reliability of your code.
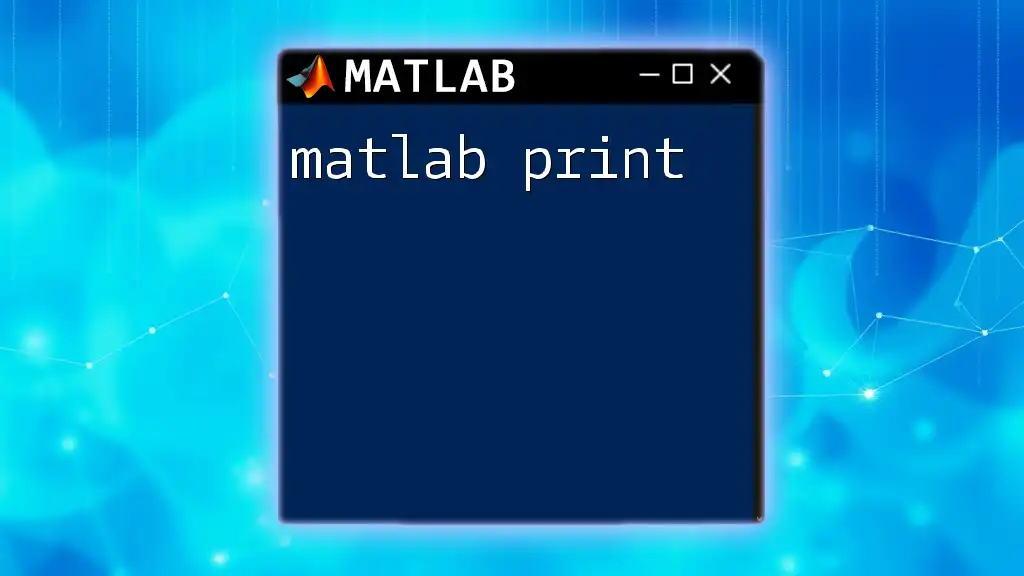
What is nargin?
Definition of nargin
The `nargin` command in MATLAB is a built-in function that tells you how many input arguments a function has received. It’s crucial for functions where the number of inputs can vary based on the user’s requirements.
Syntax of nargin
The syntax for `nargin` is straightforward. You can simply use it within the body of a function to determine how many arguments were provided by the user. You do not need to pass any parameters to `nargin`.
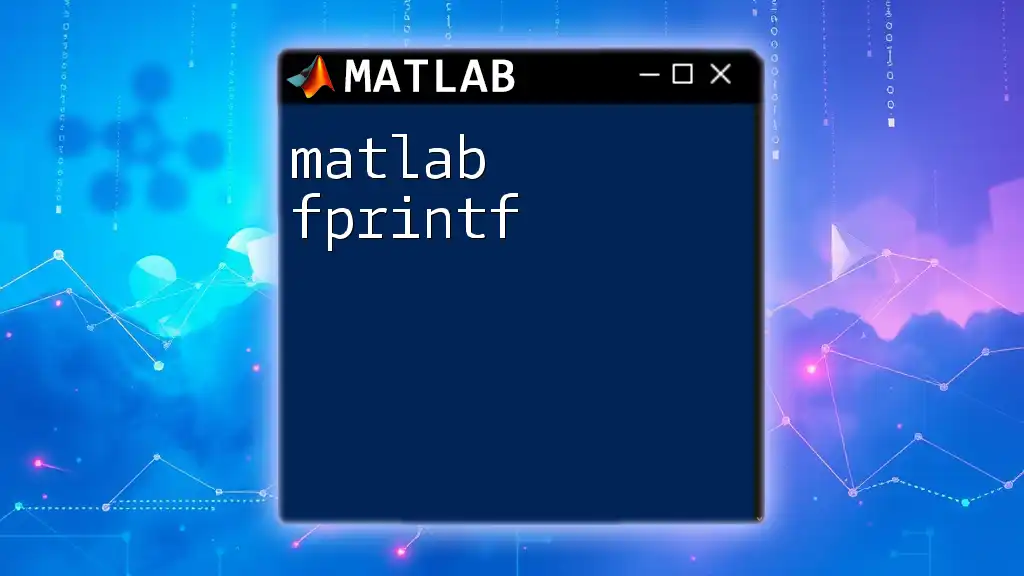
Examples of Normal Usage
Basic Example of nargin
Consider the following function:
function exampleFunction(varargin)
disp(['Number of input arguments: ', num2str(nargin)]);
end
In this example, the function `exampleFunction` accepts a variable number of inputs (thanks to `varargin`, which stands for “variable length input arguments”). When you call this function with different numbers of arguments, `nargin` will display the count, giving you straightforward insight into how many inputs were provided.
Usage in Handling Optional Inputs
Using `nargin`, we can manage optional inputs effectively. Take a look at this example:
function myFunction(a, b, c)
if nargin < 3
c = 10; % Setting a default value for c
end
disp(['Inputs are: ', num2str(a), ', ', num2str(b), ', ', num2str(c)]);
end
In the function `myFunction`, we check if a third argument `c` was provided. If not, we set `c` to a default value of 10. This check allows the function to execute smoothly even if users forget to provide a value for `c`, showcasing the importance of managing inputs effectively.
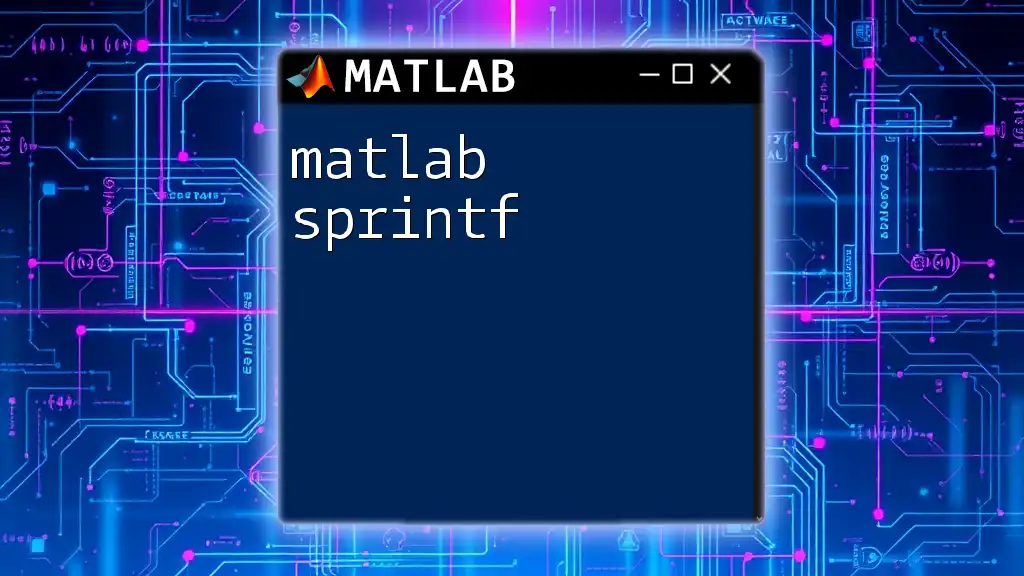
Combating Errors and Handling Missing Inputs
Error Handling Using nargin
Given that unexpected inputs can lead to errors or incorrect outputs, it's essential to implement robust error handling. By using `nargin`, you can prevent runtime errors caused by insufficient arguments.
Example: Basic Error Check
Consider the following example, which incorporates a basic error check:
function safeFunction(a, b)
if nargin < 2
error('Not enough input arguments.');
end
% Function logic here
end
In this example, the function `safeFunction` checks whether at least two arguments are passed. If not, it throws an error, guiding the user to provide the necessary inputs.
Advanced Input Validation
For more advanced situations, you can combine `nargin` with other functions, such as `nargout`, which tells you how many output arguments the function can return. This combination allows for sophisticated input validation where the number of available outputs might affect how inputs are processed.
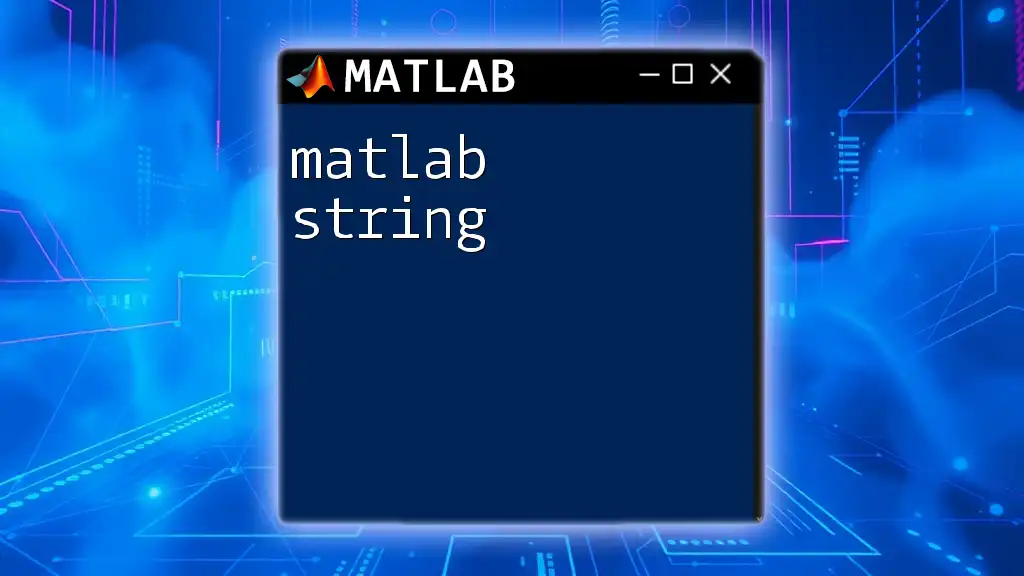
Best Practices for Using nargin
When to Use nargin
Using `nargin` is recommended in scenarios where:
- Your functions accept a varying number of inputs.
- You want to provide default values for optional parameters.
- You aim to improve the robustness and usability of your code by handling various user inputs gracefully.
Common Mistakes to Avoid
When working with `nargin`, be cautious of the following pitfalls:
- Neglecting to handle insufficient inputs can result in unexpected behavior and hard-to-debug errors.
- Overusing `nargin` in simple functions may lead to unnecessary complexity, especially if all inputs are mandatory or if default values are not needed.
- Not testing functions with varying input scenarios, which could unveil hidden issues or unexpected behavior.
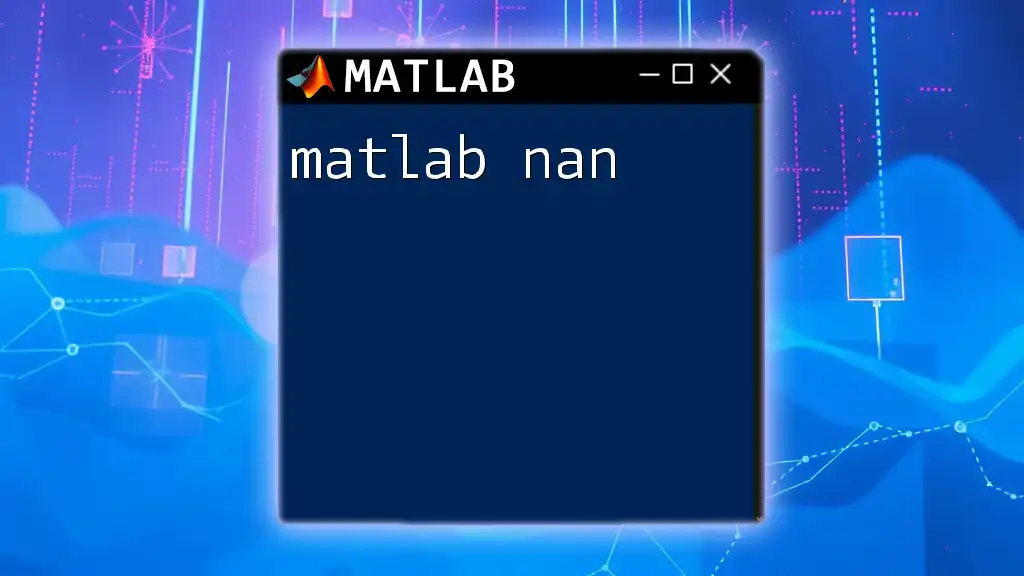
Conclusion
Understanding `nargin` is essential for effective MATLAB programming. It promotes better management of function inputs, leading to more flexible and robust code. By practicing the examples provided and integrating `nargin` into your coding practices, you'll enhance your skill set and improve the usability of your functions.
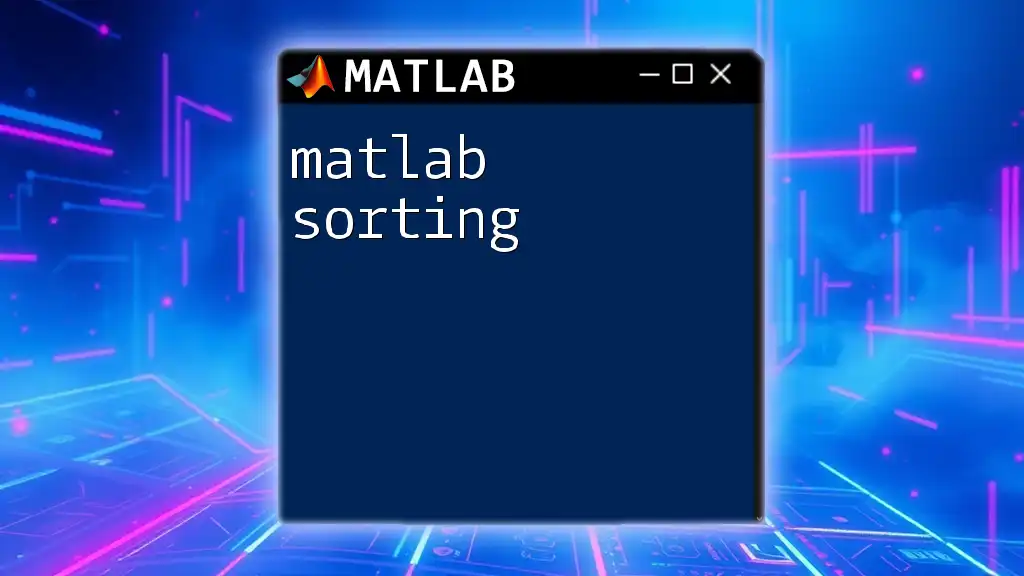
Additional Resources
For further reading and advanced topics in MATLAB, it’s highly recommended to explore textbooks on MATLAB programming and consult the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/nargin.html) for in-depth specifics about all built-in functions.
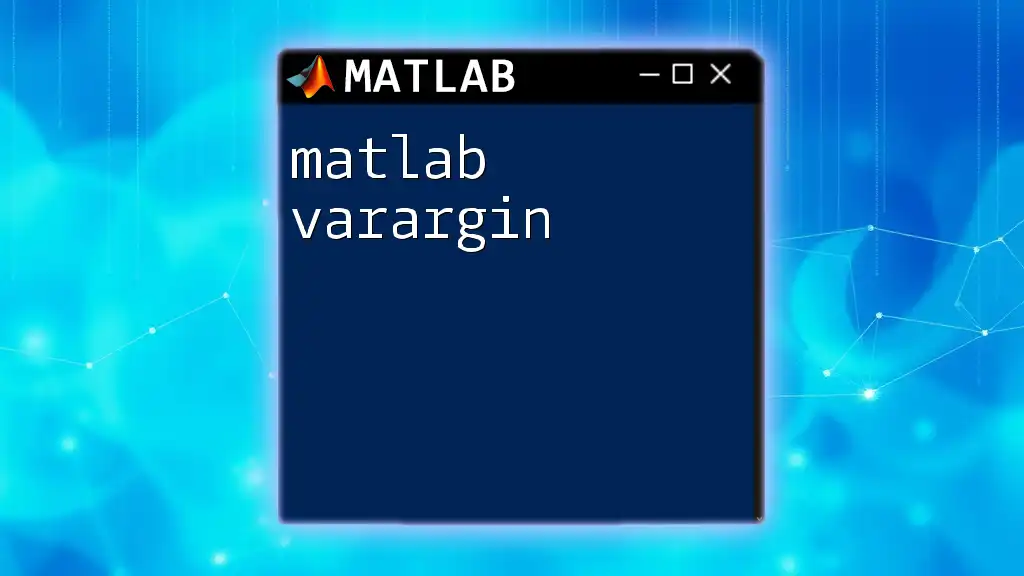
Call to Action
Now that you've learned about the power of `nargin`, try implementing it in your functions! Experiment with various input combinations and see how it can enhance your code. If you have any questions or want to share your experiences, please feel free to reach out!