The MATLAB function `nnz` counts the number of non-zero elements in an array, helping users quickly assess the sparsity of their data.
A = [1, 0, 0; 0, 3, 4; 5, 0, 0]; % Example matrix
count = nnz(A); % Counts the non-zero elements in matrix A
disp(count); % Displays the count
Understanding the MATLAB `nnz` Function
Introduction to `nnz`
Definition of nnz
The `nnz` function in MATLAB stands for "number of non-zero elements." It is a pivotal tool when working with matrices, especially in operations involving data analysis, machine learning, and optimization tasks where sparse data is a common challenge.
Use cases
Understanding how many non-zero elements are present in a matrix can significantly influence how you manipulate that data. Counting non-zero elements is fundamental when working with sparse matrices, which often arise in mathematical modeling and computational algorithms. This function is also useful in data cleansing and preprocessing, ensuring that only relevant or significant data is utilized for analysis.
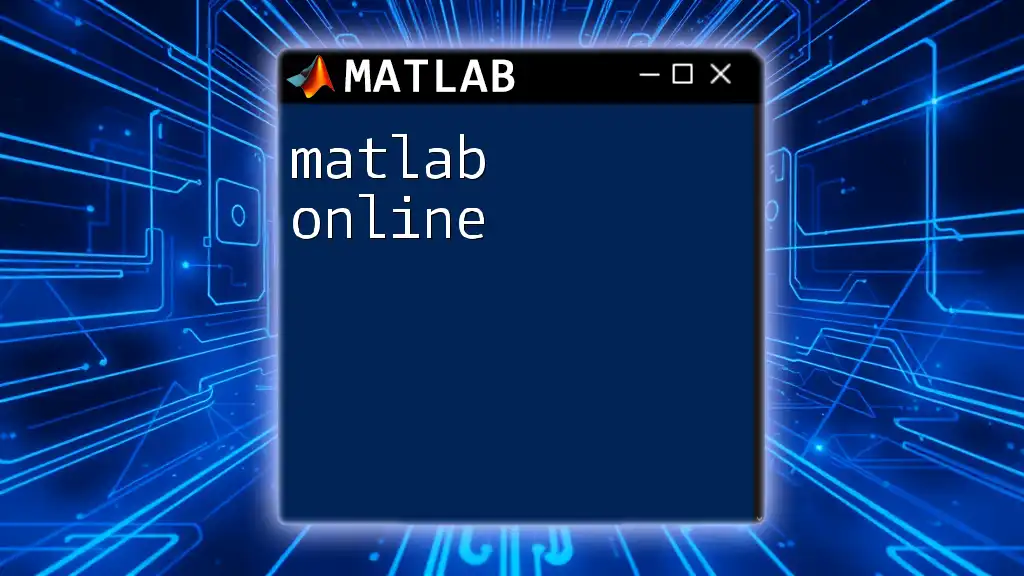
Basic Syntax of `nnz`
Function Syntax and Parameters
The basic syntax of the `nnz` function is quite straightforward:
N = nnz(A)
In this context, `A` represents the input matrix, and `N` is the output variable that stores the count of non-zero elements in the matrix.
Example of Basic Usage
Consider a simple matrix defined as follows:
A = [1 0 0; 0 2 0; 3 0 0];
nonZeroCount = nnz(A);
disp(nonZeroCount); % Output: 3
In this example, the code starts by creating a 3x3 matrix `A`. The `nnz` function then counts the non-zero elements, which are `1`, `2`, and `3`. The result, `3`, is displayed, confirming that there are three non-zero values in the matrix.
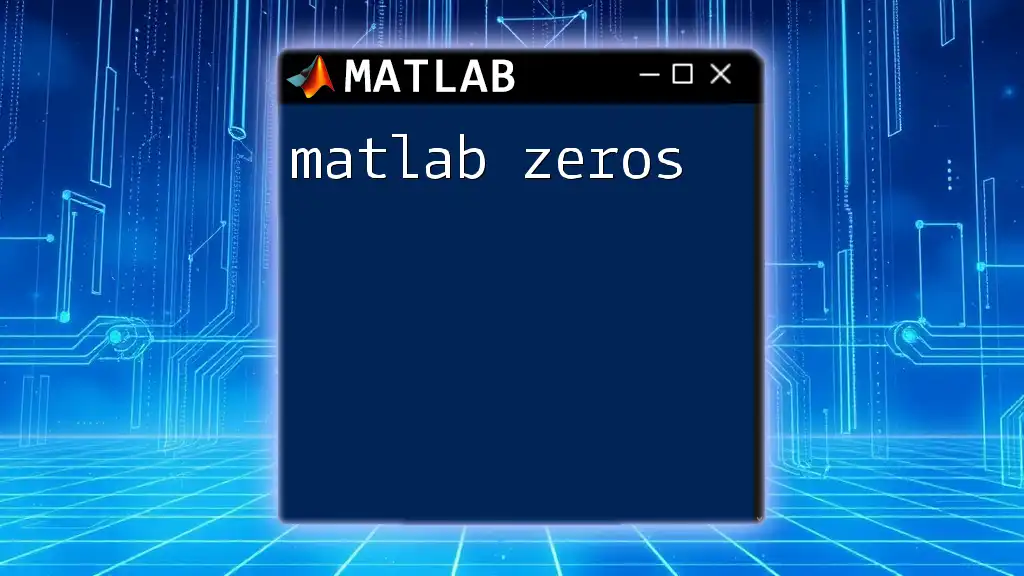
Advanced Usage of `nnz`
Counting Non-Zero Elements in Specific Dimensions
Dimension specification
The `nnz` function can be further refined to count non-zero elements across specific dimensions of a matrix. This adds a layer of flexibility to your data analysis.
Syntax for dimension specification
You can specify the dimension in the following manner:
N = nnz(A, dim)
Here, `dim` indicates the dimension you want to analyze: `1` for counting over rows and `2` for counting over columns.
Examples of Counting by Dimension
Count along rows
If you want to count the number of non-zero elements in each row of a matrix:
B = [1 0 1; 0 2 0; 3 0 0];
nonZeroCountRows = nnz(B, 1);
disp(nonZeroCountRows); % Output: 2 1 1
In this case, the output `[2 1 1]` tells us that the first row has two non-zero elements, the second row has one, and the third row also has one.
Count along columns
To count non-zero elements by columns, you can do the following:
nonZeroCountCols = nnz(B, 2);
disp(nonZeroCountCols); % Output: 1; 1; 2
This output `[1; 1; 2]` indicates that each of the three columns contains one non-zero in the first column, one in the second, and two in the third.
Practical Applications of Dimensional Counting
The ability to count non-zero elements by dimension is especially useful in various scenarios, such as data preprocessing in machine learning where some features may have more active signals than others. Moreover, knowing the dimensional properties can aid in the design of optimization algorithms which operate more effectively with a clear understanding of the underlying data structure.
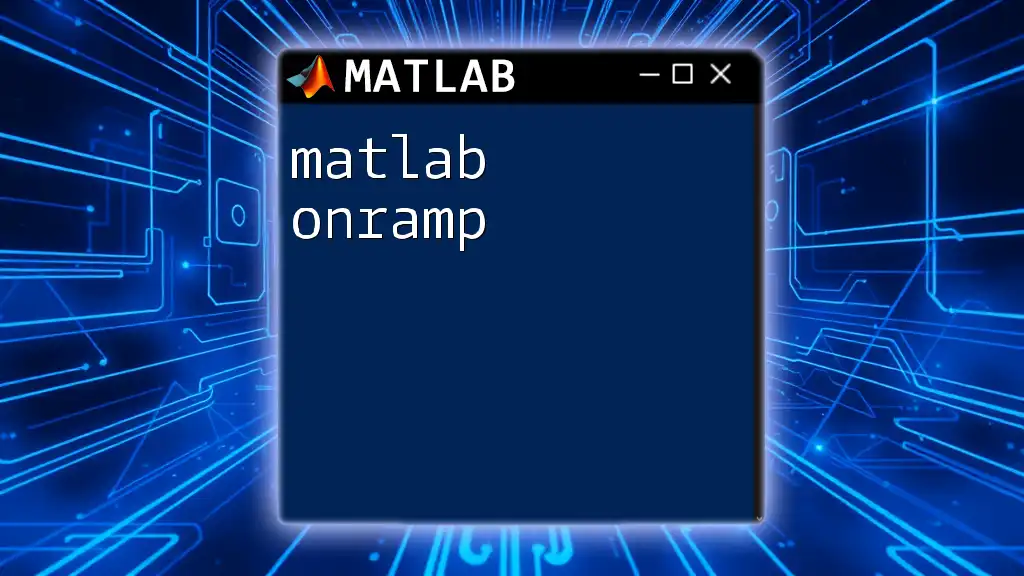
Working with Sparse Matrices
What are Sparse Matrices?
Definition and importance
A sparse matrix is a matrix in which most of the elements are zero. These structures reduce memory usage and improve computational efficiency during operations. They are indispensable in large-scale scientific computations, where storing a full matrix is inefficient and unnecessary.
Using `nnz` with Sparse Matrices
The `nnz` function works seamlessly with sparse matrices. It still counts the number of non-zero elements, but it does so without requiring the entire matrix to be loaded into memory. This makes it especially powerful for handling large datasets.
Example with Sparse Matrix
Here’s how you can use `nnz` with a sparse matrix:
S = sparse([1 2 3], [1 2 1], [1 2 3]);
sparseCount = nnz(S);
disp(sparseCount); % Output: 3
This code snippet shows the creation of a sparse matrix `S`. When we apply the `nnz` function, it efficiently determines that there are three non-zero entries, demonstrating its utility in managing sparse data.
Advantages of Using Sparse Matrices
When dealing with large datasets, leveraging sparse matrices can lead to significant improvements in memory efficiency and speed of calculations. For example, algorithms that rely on matrix calculations can execute faster, thus enhancing overall performance, enabling analyses that would otherwise be constrained by computational limits.
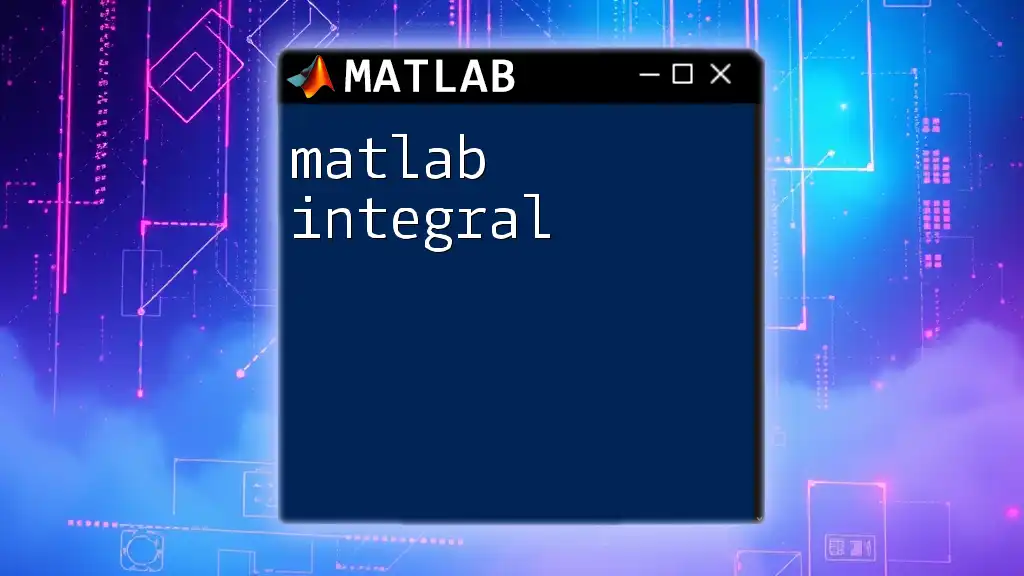
Performance Considerations
Why Non-Zero Counts Matter in Large Datasets
Knowing the number of non-zero elements can drastically improve performance in various applications, especially when working with large datasets where memory and processing power are at a premium. This efficiency is particularly evident in optimization problems, where the computation speed can be directly correlated to the density of non-zero elements.
Profiling MATLAB Code with `nnz`
Using the `nnz` function as part of your profiling can provide insights into your code's efficiency. By incorporating it in MATLAB's profiling tools, developers can ascertain bottlenecks related to data sparsity and adjust their algorithms accordingly to enhance performance.
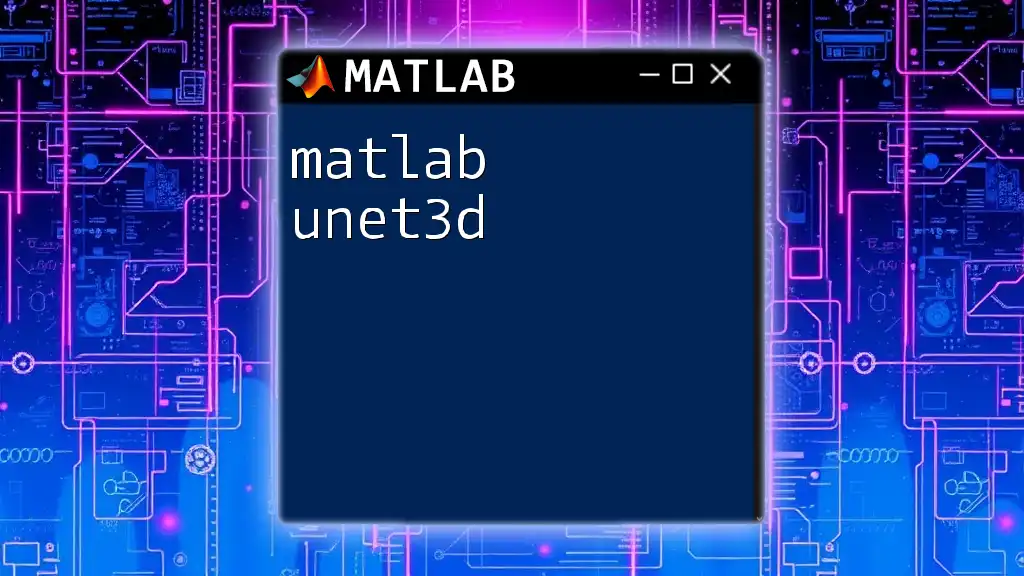
Conclusion
In summary, the MATLAB `nnz` function is an essential tool for counting non-zero elements in matrices. Its straightforward syntax, versatility in handling matrix dimensions, and compatibility with sparse matrices make it invaluable in computational applications. By understanding and utilizing `nnz`, you can enhance your data analysis techniques and optimize the performance of your algorithms. We encourage you to experiment with `nnz` in your projects and explore further into its capabilities for advanced matrix manipulation and analysis.
For more in-depth learning, explore additional MATLAB resources that focus on matrix operations and performance optimization.