Space Vector Modulation (SVM) is an advanced technique used in inverter control that optimizes motor drive performance by selecting the best switch states to represent a reference voltage vector in a three-phase system.
Here's a simple MATLAB code snippet demonstrating the basic concept of space vector modulation:
% Space Vector Modulation (SVM) Example
V_dc = 1; % DC Voltage
theta = 0:pi/6:2*pi; % Angle range for a full cycle
V_s1 = V_dc * [cos(theta); sin(theta)]; % Generating the space vectors
% Plotting the space vectors
figure;
plot(V_s1(1,:), V_s1(2,:), 'o-');
title('Space Vector Modulation');
xlabel('Voltage along a-axis');
ylabel('Voltage along b-axis');
axis equal;
grid on;
Understanding the Basics of Space Vector Modulation
What is Space Vector Modulation?
Space Vector Modulation (SVM) is an advanced technique used in power electronics for the control of three-phase voltage source inverters. Unlike traditional Pulse Width Modulation (PWM), which typically generates multiple voltage levels, SVM allows for more efficient utilization of the voltage space, thereby improving overall performance. The key advantage of SVM lies in its ability to minimize harmonic distortion and maximize output voltage, providing a smoother and more efficient operation for motor drives and other applications.
Key Concepts and Terminology
To gain a better understanding of SVM, it is essential to familiarize yourself with a few foundational concepts:
- α-β-frame: This is a reference frame used to represent the three-phase voltages in a two-dimensional plane. Understanding this frame is crucial, as it simplifies the analysis and implementation of SVM.
- Voltage Vectors: In SVM, the inverter's output can be represented as discrete voltage vectors in the α-β plane. Each vector corresponds to a specific combination of switching states in the inverter.
- Reference Voltage Vector: This is the desired output voltage of the inverter expressed as a vector. The objective of SVM is to manipulate various switching states to get as close as possible to this reference vector.
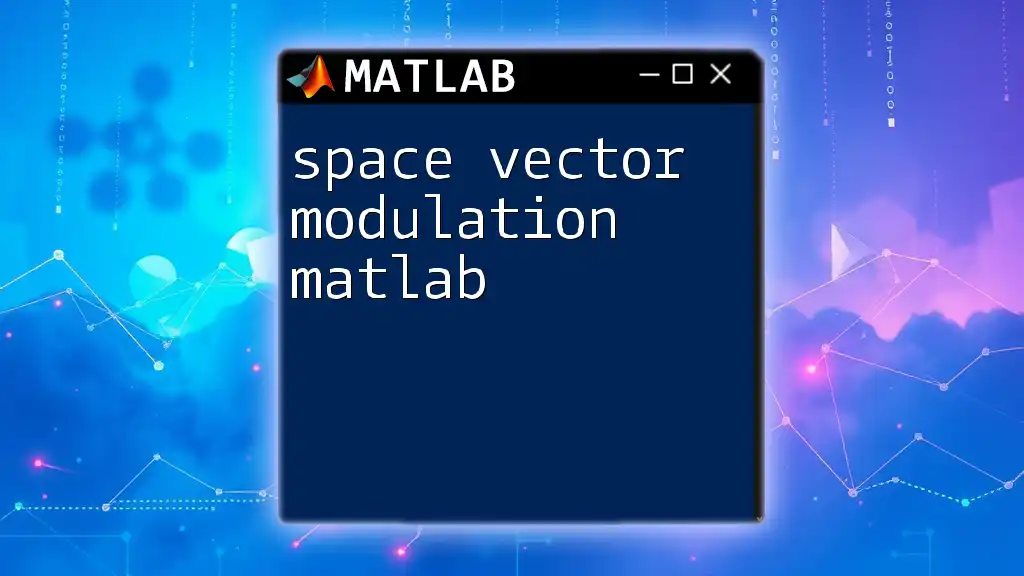
Mathematical Foundations of SVM
Mathematical Representation of Space Vectors
Space vectors can be mathematically represented using complex numbers. In this context, voltages in the α-β-frame are expressed as:
\[ V_{αβ} = V_α + jV_β \]
Where \(j\) represents the imaginary unit. This representation is essential when calculating the angles and magnitudes of the voltage vectors required for modulation.
Equations Governing SVM
Several key equations govern the principles of SVM. One significant metric in this area is the modulation index (M), defined as the ratio of the desired output voltage to the maximum possible output voltage from the inverter. Mathematically, it can be expressed as:
\[ M = \frac{V_{ref}}{V_{max}} \]
Where:
- \(V_{ref}\) is the reference voltage vector's magnitude.
- \(V_{max}\) is the maximum achievable voltage vector from the inverter, typically equal to \(\frac{V_{dc}}{2}\).
Understanding and manipulating these equations forms the basis of implementing effective space vector modulation strategies.
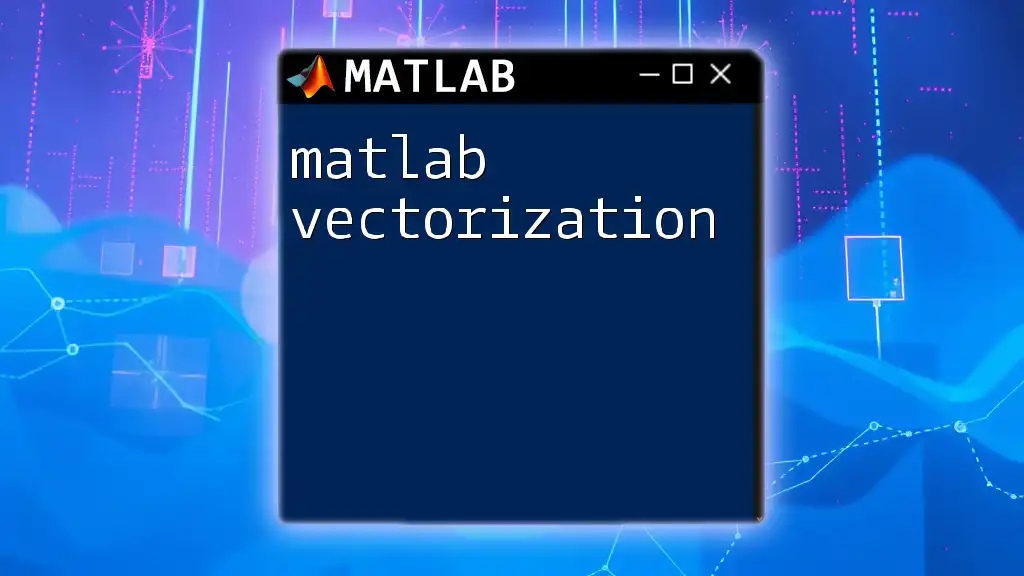
Implementation of SVM in MATLAB
Setting Up Your MATLAB Environment
Before diving into coding, ensure that you have MATLAB installed with the necessary toolboxes, such as Simulink and the Control System Toolbox. These will provide the functions and capabilities required to implement SVM efficiently.
Writing Your First SVM Algorithm in MATLAB
Step 1: Defining Parameters
Start by defining the essential parameters for your SVM implementation. Here’s a basic example of how you can set these parameters in MATLAB:
Ts = 1e-4; % Sampling time in seconds
V_dc = 100; % DC bus voltage
M = 0.8; % Modulation index
These parameters will play a pivotal role in your calculations and control logic.
Step 2: Generating the Space Vectors
Next, generate the space vectors based on the defined parameters. This can be done using the angle representation in the α-β frame:
theta = 0:pi/3:2*pi; % Angle for generating space vectors
V_space = V_dc * [cos(theta); sin(theta)];
This code effectively computes the space vectors for the entire 360-degree range in the two-dimensional plane.
Step 3: Implementing the SVM Algorithm
The core of the SVM process is the algorithm responsible for generating the switching states based on the reference vector. Below is a simplified example of how you can implement this in MATLAB:
function [u] = SVM(V_ref)
% Function to implement Space Vector Modulation
% Generate switching states based on V_ref
% Normalize the reference vector
V_ref = V_ref / norm(V_ref);
...
% Logic for determining time duration of each sector
...
end
This function outlines the basic structure, where you can fill in the logic to determine how to switch the inverter states based on the given reference vector.
Visualizing Results
Visualizing the output can greatly help in comprehending your SVM results. By plotting the space vector trajectory, you can monitor how closely your output follows the reference voltage. Here’s an example of how to plot these space vectors in MATLAB:
figure;
plot(V_space(1,:), V_space(2,:), 'o-');
xlabel('Vα'); ylabel('Vβ');
title('Space Vector Trajectory');
grid on;
This code generates a plot showing the trajectory of the voltage vectors in the α-β plane, facilitating a better understanding of how the system operates.
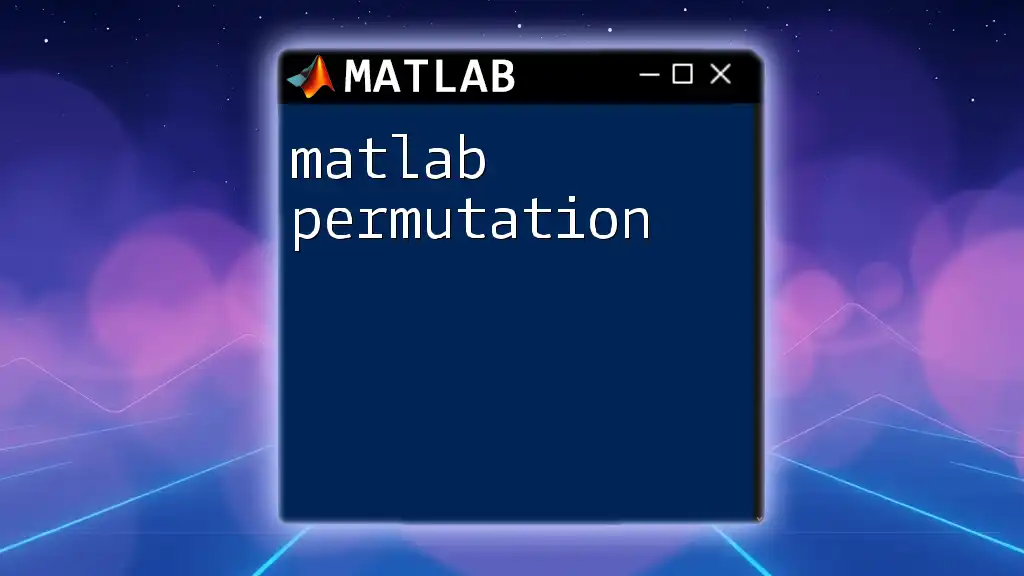
Navigating Real-World Applications of SVM
Applications in Power Electronics
The versatility of SVM makes it a valuable tool in various applications such as:
- Inverters: Efficiently controlling the output voltage in renewable energy systems.
- Motor Drives: Improving the performance of AC motors through precise voltage control.
- Renewable Energy Systems: Enhancing the output of solar inverters for better energy management.
Advantages of Using MATLAB for SVM
Using MATLAB for implementing Space Vector Modulation brings several advantages:
- Rapid Prototyping: MATLAB allows for swift development and iteration of algorithms, enabling quick adjustments based on simulation results.
- Simulation Capabilities: The integration with Simulink provides a graphical interface to simulate and visualize complex systems, making it easier to identify issues before hardware implementation.
- Efficient Debugging: MATLAB’s robust debugging tools simplify the process of tracing errors in algorithms, ensuring reliability in control systems.
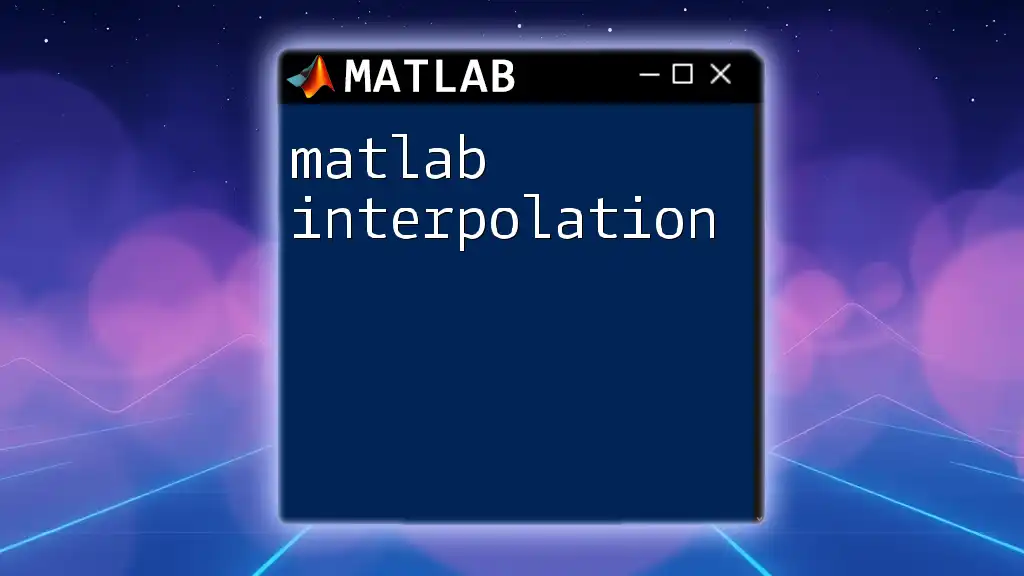
Advanced Topics in Space Vector Modulation
Optimization Techniques for SVM
In the quest for higher efficiency, advanced algorithms such as Field Oriented Control (FOC) and Direct Torque Control (DTC) can be integrated with SVM. These techniques help minimize harmonic distortion and enhance torque control, leading to improved motor performance.
Future Trends in SVM
As the industry continues to evolve, we can expect to see significant advancements in SVM. The integration of artificial intelligence (AI) and machine learning (ML) is likely to play a pivotal role in optimizing SVM strategies, paving the way for smarter, self-regulating systems that can adjust based on real-time conditions.
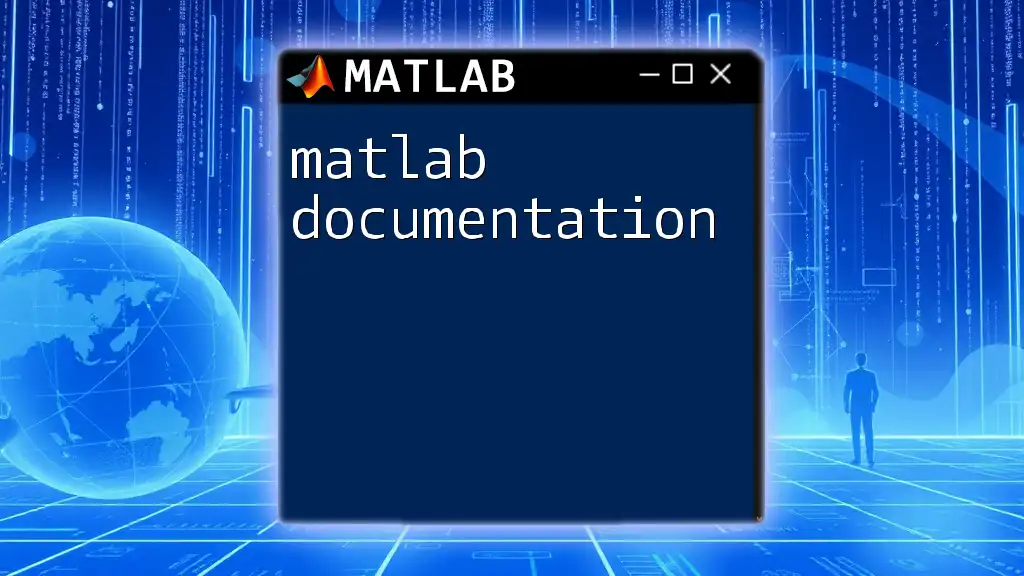
Conclusion
In this article, we covered the essentials of MATLAB Space Vector Modulation, exploring both the theoretical foundations and practical implementations. By mastering SVM, you can leverage MATLAB’s powerful tools to develop efficient, high-performance control systems. As this technology continues to advance, the significance of SVM in various applications will only grow, making it a crucial area of study for aspiring engineers and technologists.