Space vector modulation (SVM) in MATLAB is a technique used to control voltage source inverters by optimizing the output waveform quality and minimizing harmonic distortion.
Here’s a simple example of SVM implementation in MATLAB:
% Space Vector Modulation (SVM) Example
V_dc = 100; % DC link voltage
theta = 0:0.01:2*pi; % Angle range
% Calculate the space vectors
V_a = V_dc * cos(theta);
V_b = V_dc * cos(theta - 2*pi/3);
V_c = V_dc * cos(theta + 2*pi/3);
% 3-phase output voltage
V_phase = [V_a; V_b; V_c];
% Plot the results
figure;
plot(theta, V_phase);
title('Space Vector Modulation');
xlabel('Angle (radians)');
ylabel('Phase Voltage (V)');
legend('V_a', 'V_b', 'V_c');
grid on;
What is Space Vector Modulation?
Space Vector Modulation (SVM) is a sophisticated technique used in the control of AC drives. It modulates the output voltage of an inverter, allowing for smoother and more efficient motor control. SVM is particularly beneficial when compared to traditional modulation methods, such as Pulse Width Modulation (PWM), due to its ability to utilize the inverter's DC link voltage more efficiently, thus enhancing system performance.
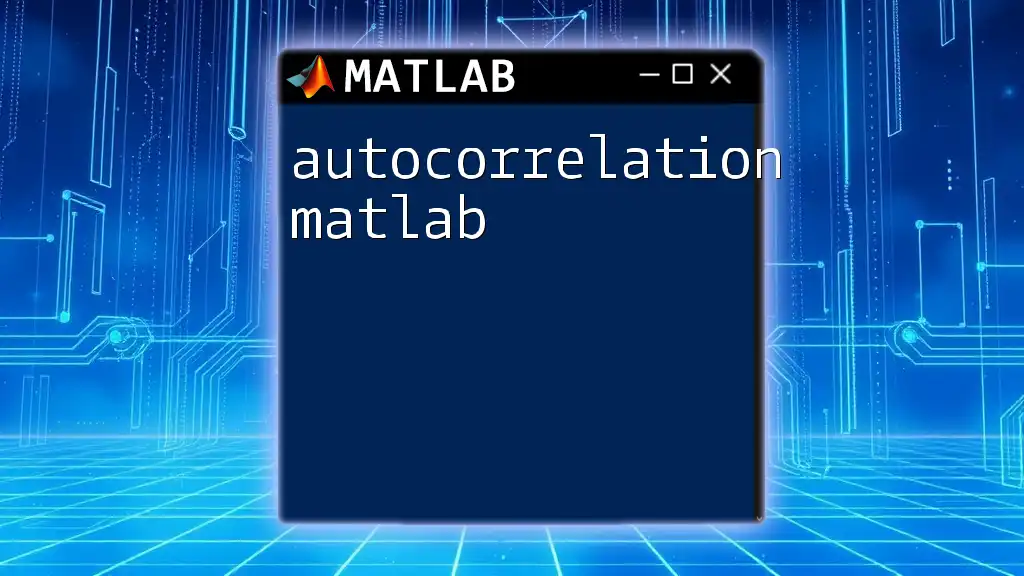
Applications of Space Vector Modulation
SVM is widely implemented across various industries where the control of electric motors and energy systems is crucial. Its key applications include:
- Electric Motors: In controlling speed and torque for industrial drives.
- Power Inverters: Used in renewable energy systems such as solar and wind for energy conversion.
- Transportation: Implemented in electric and hybrid vehicles to optimize performance and efficiency.
The benefits of SVM include improved power quality, reduced harmonic distortion, and efficient use of DC link voltage.
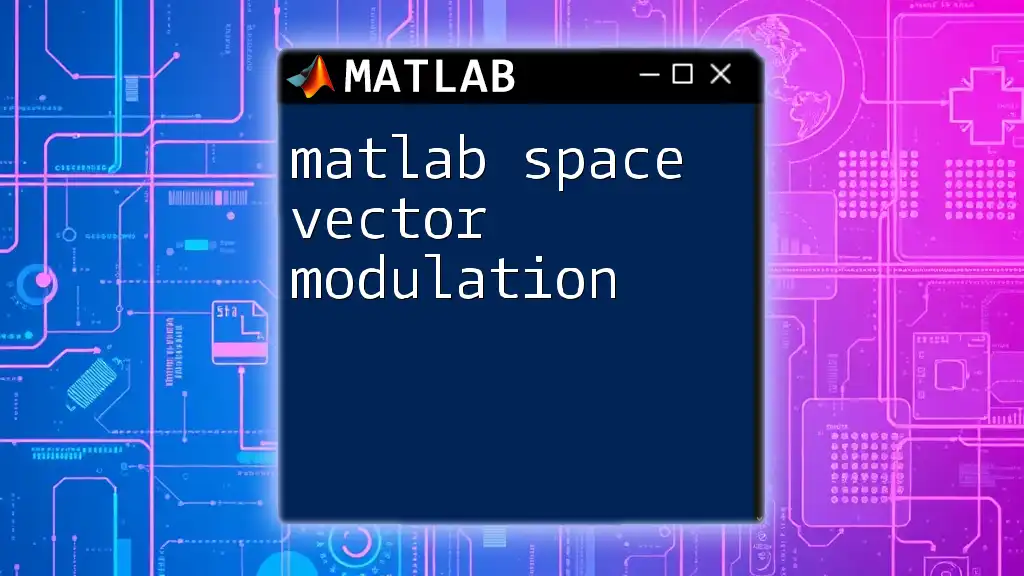
Understanding the Concepts of Space Vector Modulation
Basic Principles of SVM
Space Vector Modulation operates on the principle that a three-phase system can be represented as a single rotating vector in a two-dimensional space. This vector simplifies the analysis and control of motor drive systems. The output voltage is represented as a combination of eight distinct voltage vectors—six active vectors corresponding to the motor's phases and two zero vectors that stabilize the voltage for modulation.
The Space Vector Diagram
Explanation of the Space Vector Diagram
The Space Vector Diagram is a graphical representation used to visualize the relationship between various voltage vectors in a three-phase system. It is typically illustrated as a hexagon, where each vertex corresponds to an active vector, and connections between points represent the possible transitions between these states as they rotate in the clockwise direction.
Significance of the Diagram
This diagram is essential for understanding how voltage vectors combine to produce a desired output. By analyzing the rotational dynamics and phase relationships, engineers can effectively modulate the inverter's output to meet specific operational requirements.
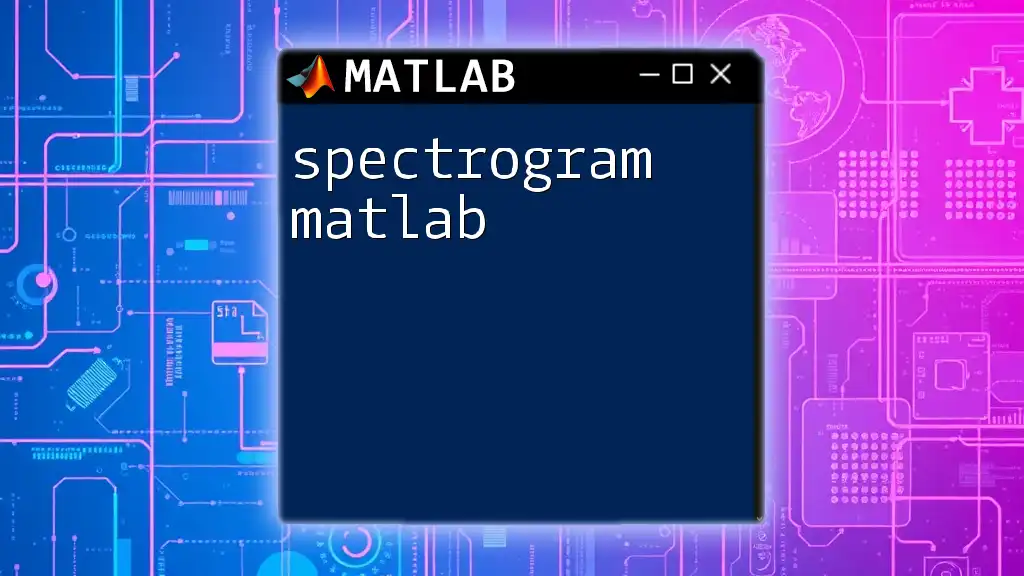
MATLAB Basics for SVM
Overview of MATLAB Environment
MATLAB provides a robust environment for simulation and control system design. Students and practitioners can leverage its numerous features, including rich visualization capabilities and extensive mathematical functions, making it an excellent tool for learning space vector modulation MATLAB.
MATLAB Functions and Toolboxes for SVM
To effectively implement SVM, familiarity with relevant MATLAB toolboxes is essential. Key toolboxes include:
- Simulink: Ideal for designing and simulating dynamic systems.
- Control System Toolbox: Provides functions for system modeling and analysis.
- Signal Processing Toolbox: Combines functions that are useful for generating and processing signals.
Understanding functions such as `plot`, `svpwm`, and `rot90` will be beneficial in constructing and analyzing your SVM models.
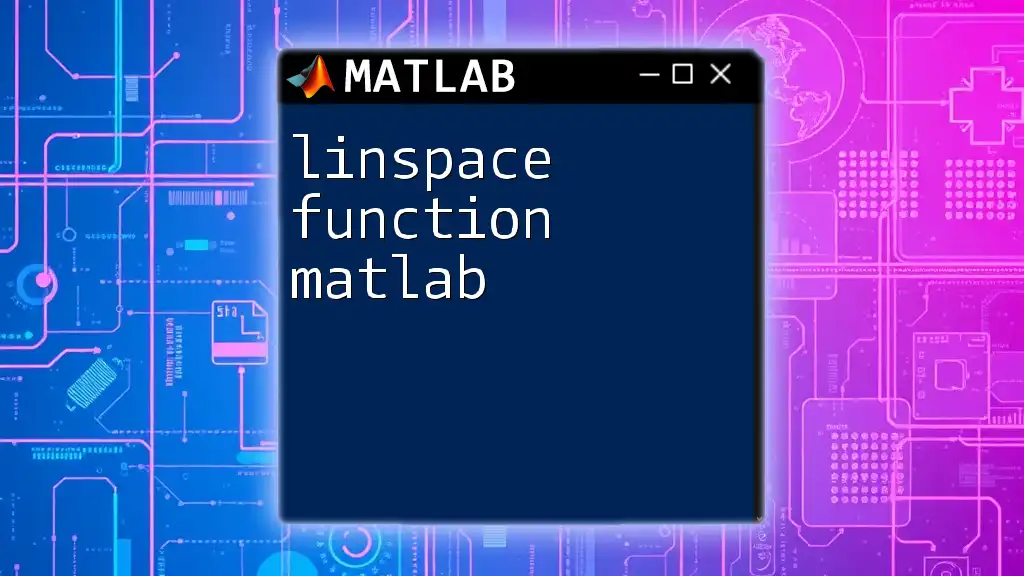
Implementing Space Vector Modulation in MATLAB
Step-by-Step Guide to SVM Implementation
Defining Parameters
Defining the operational parameters is the first step in implementing SVM through MATLAB. Key parameters include the DC link voltage, switching frequency, and desired output frequency. The following code snippet demonstrates how to set these parameters:
V_dc = 400; % DC link voltage
fs = 10000; % Switching frequency
f = 50; % Desired output frequency
Generating the Reference Signal
Next, we need to create sine wave reference signals, which will serve as the basis for the modulation process. The following example generates a sine wave function to represent the desired output voltage:
t = 0:1/fs:1; % Time vector
V_ref = V_dc * sin(2*pi*f*t); % Reference voltage
Constructing Space Vectors
Identifying Active and Zero Vectors
In SVM, it is crucial to distinguish between active and zero vectors. Active vectors correspond to the voltages applied to the motor phases, while zero vectors stabilize the output. Understanding this distinction allows for more precise control over the motor.
Code Snippet for Constructing Space Vectors
Constructing the active space vectors can be accomplished through the following code snippet:
% Define active vectors
V_a = [V_dc, 0; -V_dc/2, V_dc*sqrt(3)/2; -V_dc/2, -V_dc*sqrt(3)/2];
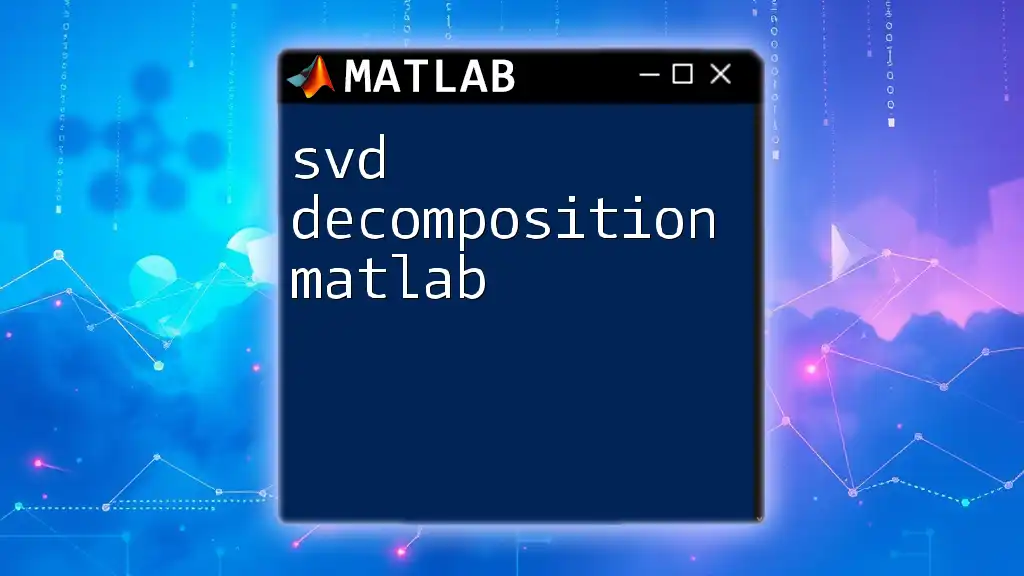
Modulation Techniques in MATLAB
Sinusoidal Pulse Width Modulation (SPWM)
SPWM is one of the most basic forms of modulation. While straightforward, it effectively produces a sine wave output. The following code demonstrates how to generate an SPWM signal in MATLAB:
PWM_signal = sawtooth(2*pi*fs*t, 0.5); % SPWM generation
Space Vector PWM (SVPWM)
Space Vector PWM is an advanced form of modulation that offers improved efficiency and lower harmonic distortion compared to traditional PWM methods. It dynamically selects the closest active and zero vectors to track the reference voltage optimally. Here is an example code implementation:
% SVPWM algorithm implementation example
duty_cycle = svpwm_algorithm(V_ref);
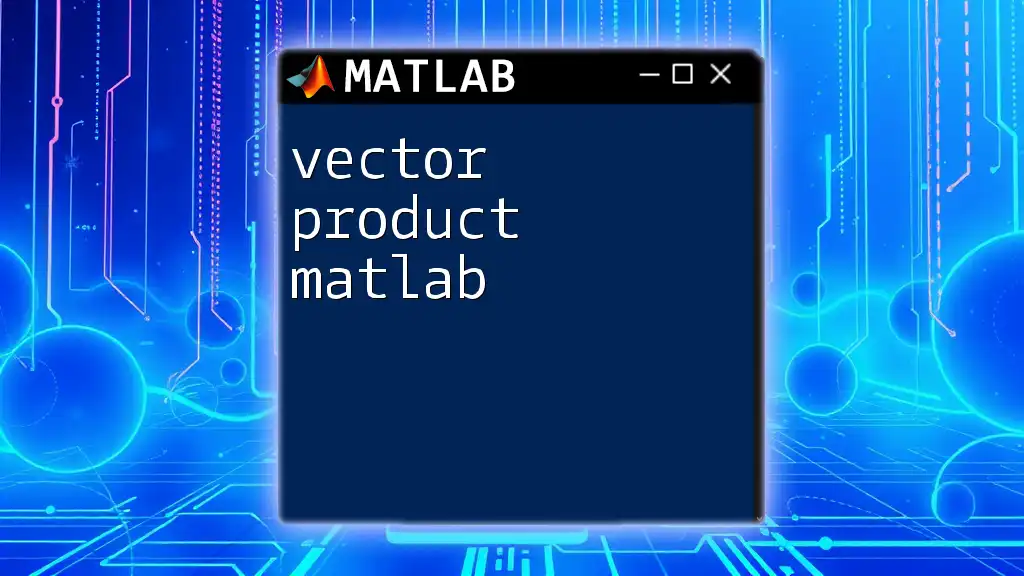
Visualization and Analysis in MATLAB
Plotting Vector Diagrams and Waveforms
Utilizing MATLAB’s plotting capabilities is crucial for analyzing the performance of your SVM model. You can visualize the reference voltage waveform and compare it against the actual output signals. Here’s how to plot the reference waveform:
plot(t, V_ref);
title('Reference Voltage Waveform');
xlabel('Time (s)');
ylabel('Voltage (V)');
grid on;
Performance Metrics
To assess the efficiency of your SVM implementation, it is necessary to calculate performance metrics like efficiency and harmonic distortion. The following code snippet illustrates how to compute efficiency based on input and output power:
efficiency = calculate_efficiency(output, input);
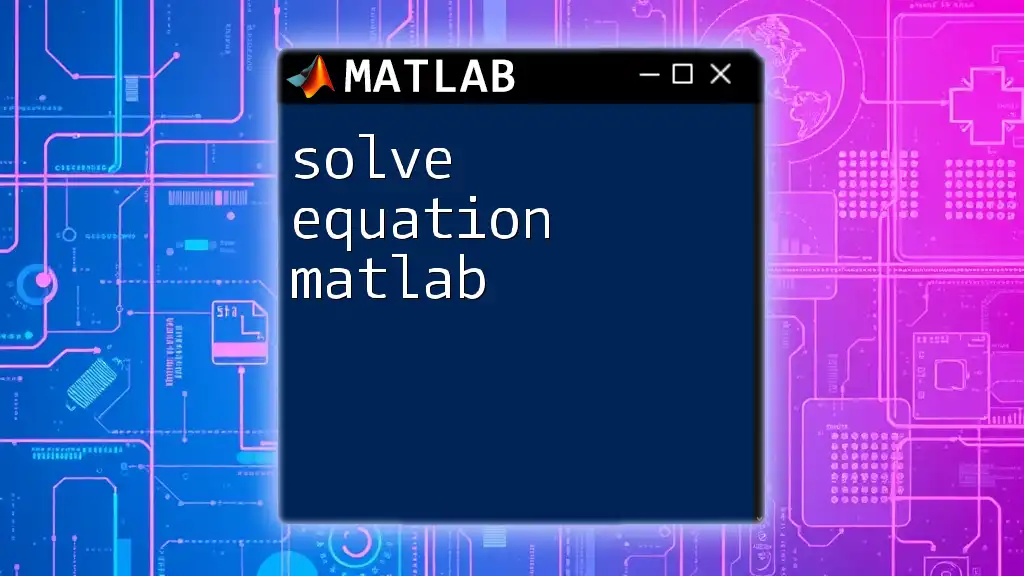
Troubleshooting Common Issues in SVM
Common Challenges
When working with space vector modulation MATLAB, you may encounter challenges such as noise in the output signal or instability in the generated waveform. To address these issues, it's vital to ensure correct parameter definition and vector construction. Additional tips include increasing the sampling rate and utilizing filters to improve signal quality.
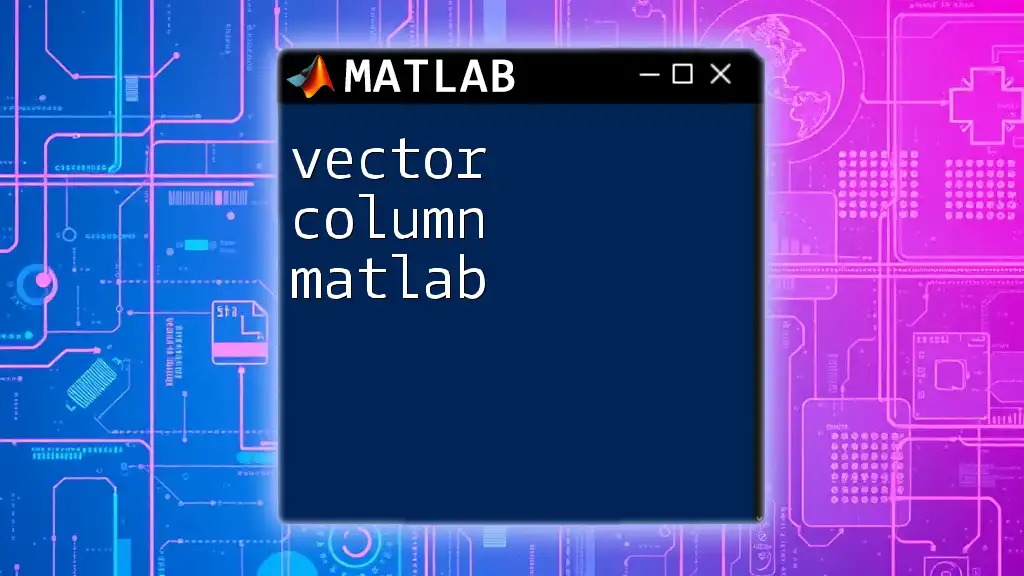
Conclusion
In conclusion, mastering space vector modulation MATLAB is crucial for effectively controlling AC drives and enhancing system performance. By understanding the principles, implementing SVM in MATLAB, and leveraging the available tools and techniques, you can optimize motor control systems significantly. I encourage you to explore and experiment with MATLAB to deepen your knowledge and harness the full potential of SVM in practical applications. With diligent practice and application, you will soon become proficient in this essential technology.