The "skalarprodukt" (or dot product) in MATLAB computes the scalar product of two vectors, resulting in a single numerical value that represents the magnitude of their similarity.
Here is an example of the MATLAB command to calculate the dot product:
A = [1, 2, 3]; % First vector
B = [4, 5, 6]; % Second vector
skalarprodukt = dot(A, B); % Calculate the dot product
Understanding the Concept of Skalarprodukt
What is Skalarprodukt?
Skalarprodukt, or the dot product, is a fundamental operation in linear algebra that involves the multiplication of two vectors. This operation results in a single scalar value, making it an essential concept in various fields such as physics, engineering, and computer science.
Properties of Skalarprodukt
The dot product has several noteworthy properties:
-
Commutative Property: The order of the vectors does not affect the outcome. That is, \( A \cdot B = B \cdot A \).
-
Distributive Property: The dot product distributes over vector addition. Hence, \( A \cdot (B + C) = A \cdot B + A \cdot C \).
-
Associative Property with Scalar Multiplication: If a scalar \( k \) is multiplied by a vector \( A \), the dot product with another vector \( B \) can be expressed as \( kA \cdot B = k(A \cdot B) \).
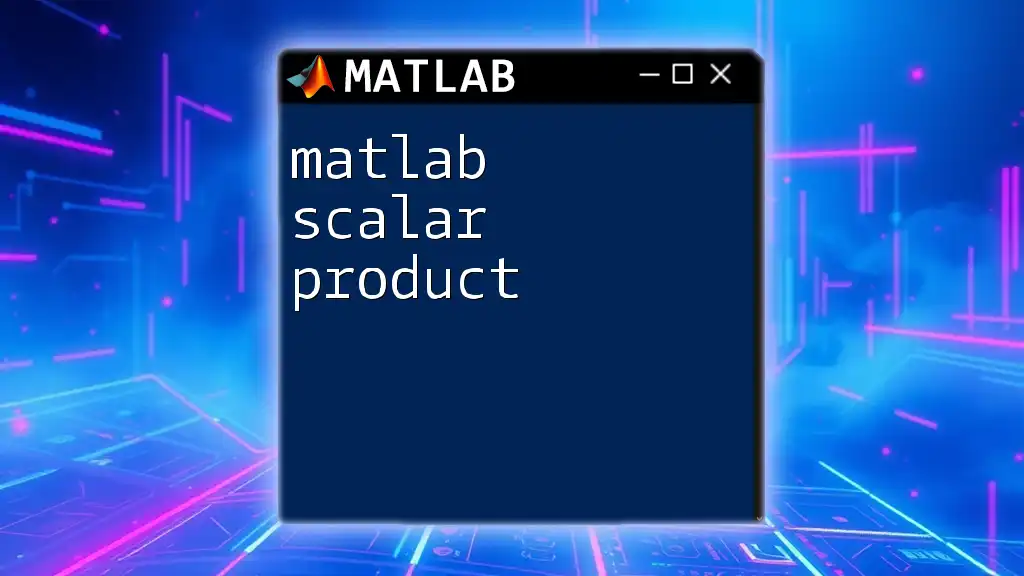
MATLAB Basics
Getting Started with MATLAB
Before diving into the intricacies of MATLAB skalarprodukt, it’s essential to familiarize yourself with the basic interface of MATLAB. The MATLAB workspace consists of several key components, including the command window, the workspace viewer, and the editor.
Basic MATLAB Commands
MATLAB operates primarily on vectors and matrices. You can create them using simple commands:
A = [1, 2, 3]; % A row vector
B = [4; 5; 6]; % A column vector
You can also perform basic operations, such as addition, subtraction, and element-wise multiplication.
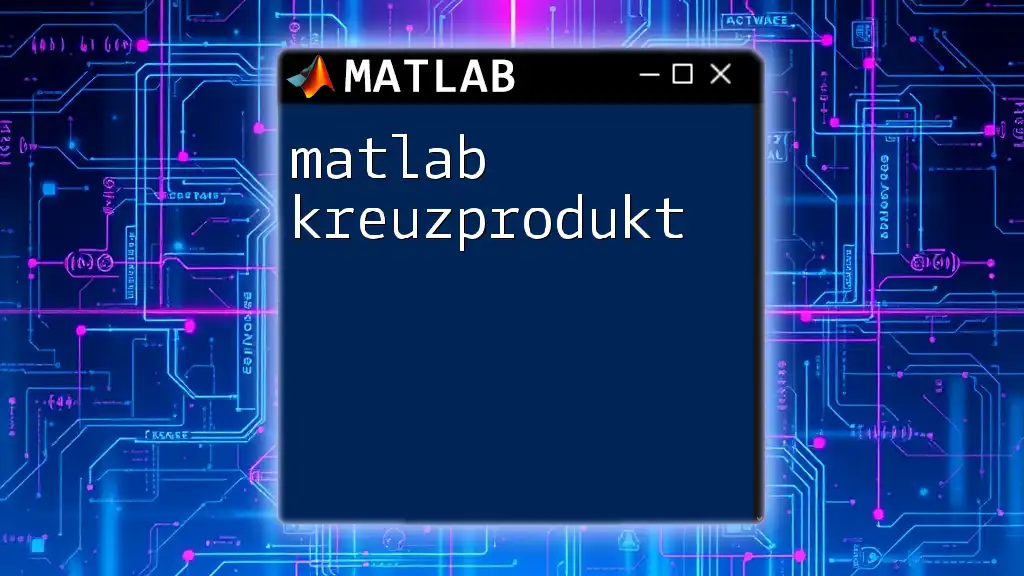
Implementing Skalarprodukt in MATLAB
The Dot Product Function
MATLAB provides a built-in function called `dot()` specifically for calculating the dot product of two vectors. The syntax for using this function is straightforward:
C = dot(A, B);
Example of Using `dot()`
Consider the vectors \( A \) and \( B \):
A = [1, 2, 3];
B = [4, 5, 6];
C = dot(A, B);
In this example, \( C \) will equal 32, which is computed as \( 1 \times 4 + 2 \times 5 + 3 \times 6 \).
Manual Calculation of Skalarprodukt
Although the `dot()` function is convenient, understanding how to compute the dot product manually is critical for learning. One way to implement it is by using a loop:
A = [1, 2, 3];
B = [4, 5, 6];
C = 0;
for i = 1:length(A)
C = C + A(i) * B(i);
end
In this code, we initialize \( C \) to zero and accumulate the products of corresponding elements in vectors \( A \) and \( B \).
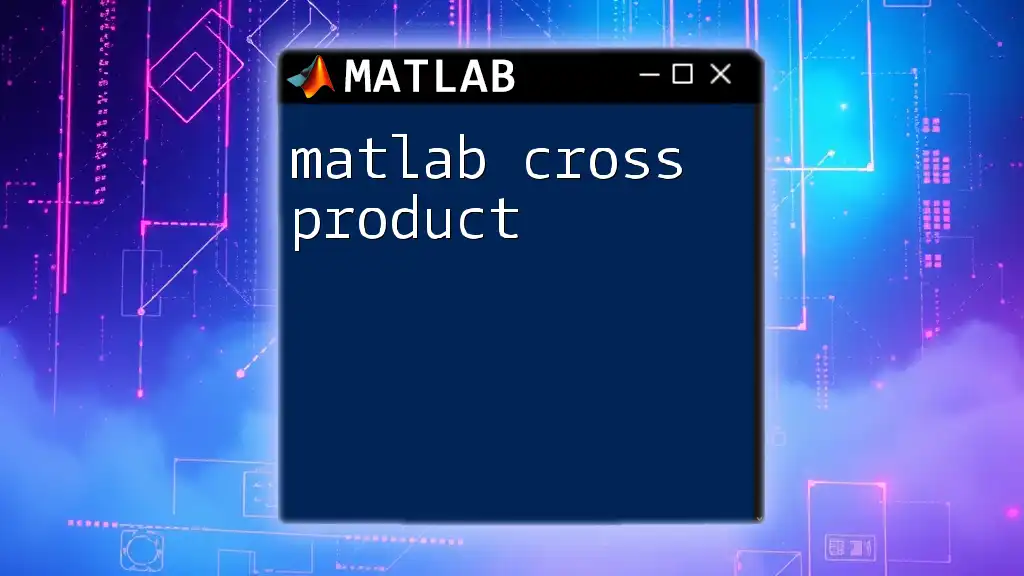
Practical Examples and Applications
Example 1: Vector Projection
Vector projection is an important concept where the Skalarprodukt plays a crucial role. The projection of vector \( A \) onto vector \( B \) can be calculated as follows:
A = [1, 2];
B = [4, 6];
projection = (dot(A,B) / dot(B,B)) * B;
This formula computes how much of \( A \) lies in the direction of \( B \).
Example 2: Cosine Similarity
Cosine similarity is widely used in machine learning and data analysis applications. It measures the cosine of the angle between two non-zero vectors, effectively understanding their orientation. Here’s how to implement it:
A = [1, 2, 3];
B = [4, 5, 6];
similarity = dot(A,B) / (norm(A) * norm(B));
In this code snippet, `norm()` computes the magnitude of the vectors, allowing us to calculate the cosine similarity effectively.
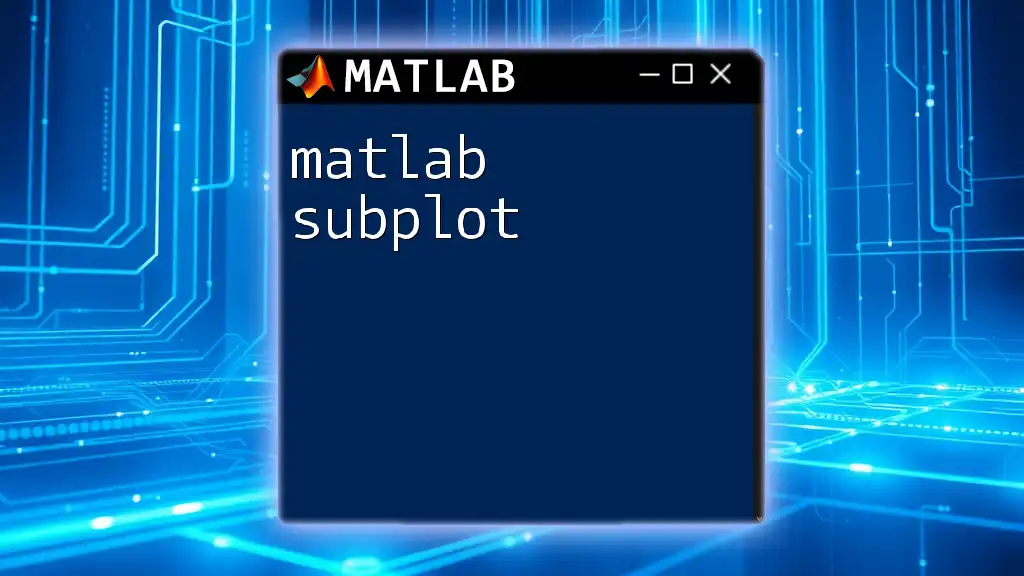
Error Handling and Debugging
Common Errors in Skalarprodukt Calculations
When working with MATLAB skalarprodukt, it's essential to be aware of common pitfalls:
-
Dimension Mismatch: Ensure both vectors are of the same length. For instance, \( A \) cannot be a 3x1 matrix while \( B \) is a 2x1 matrix.
-
Type Mismatch: Remember that MATLAB treats matrices and vectors differently; always ensure you’re not mixing these types unexpectedly.
Debugging Tips
To avoid errors, make use of debugging practices:
- Utilize `size(A)` and `class(A)` to check the dimensions and data types of your vectors before performing operations.
- Implement error checking using conditional statements to confirm your inputs meet expected requirements.
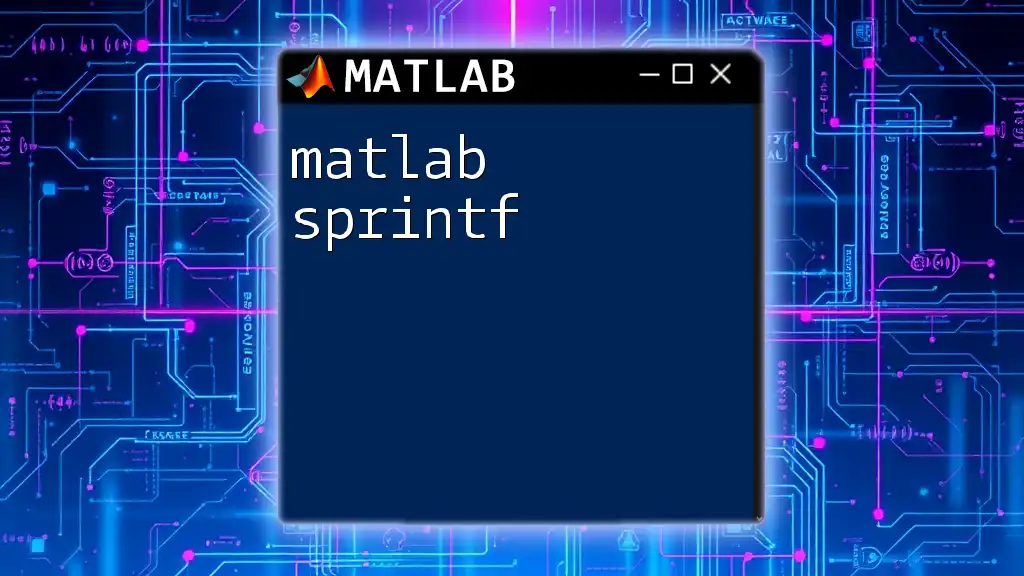
Advanced Techniques
Using Skalarprodukt with Complex Numbers
MATLAB allows you to calculate the Skalarprodukt even with complex numbers. Here’s how:
A = [1+2i, 2+3i];
B = [4+5i, 5+6i];
C = dot(A, B);
In this case, the `dot()` function will handle the complex multiplication appropriately, resulting in a complex scalar.
Performance Considerations
When implementing Skalarprodukt calculations, performance can vary significantly based on the method used. Using MATLAB’s built-in `dot()` function is generally faster and more efficient than a manual loop, especially for large vectors. Emphasize vectorization—performing operations on entire arrays rather than individual elements—to improve performance.
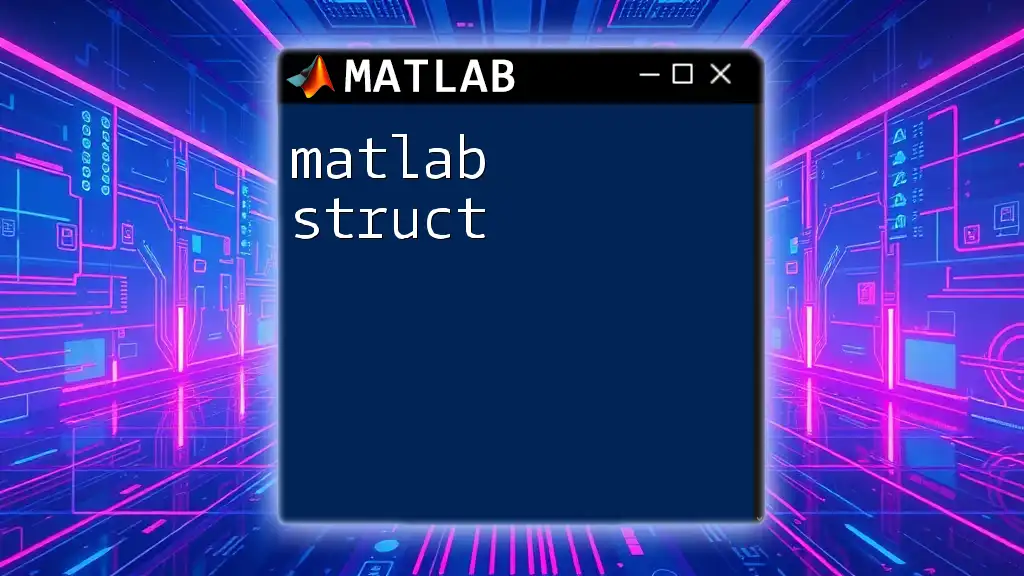
Conclusion
In this comprehensive guide on MATLAB skalarprodukt, we explored the definition, properties, and various methods of calculating the dot product. From using MATLAB’s built-in functions to manual implementations, and even advanced applications such as vector projection and cosine similarity, you have gained a solid foundation in this crucial mathematical operation.
Encouraging further exploration is key. It’s beneficial to practice with additional MATLAB commands and engage with related resources for continued learning. By enhancing your understanding of MATLAB, you empower yourself to tackle more complex computational tasks with ease.
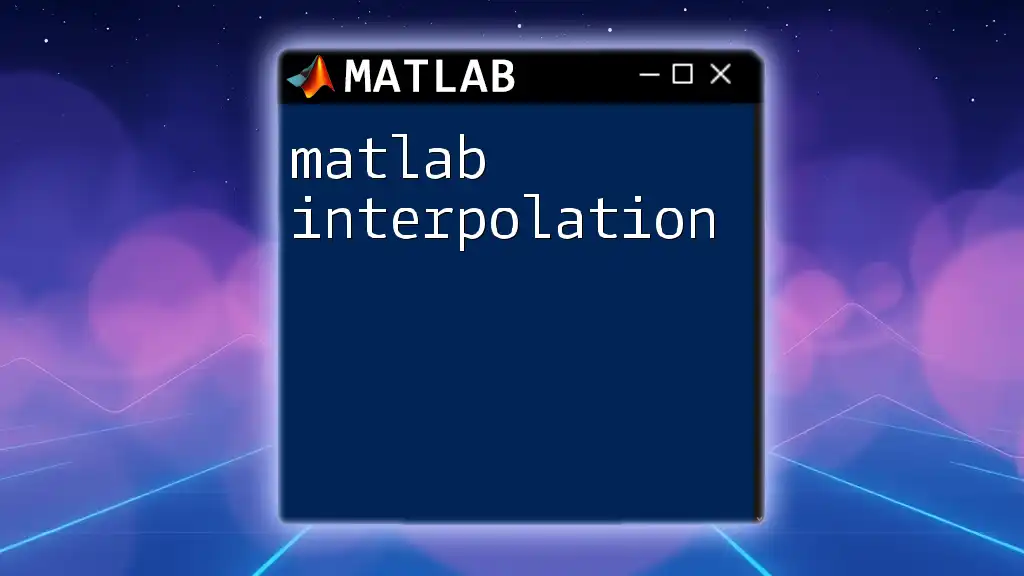
Call to Action
Join our MATLAB community to stay updated with tutorials, tips, and resources that will enhance your programming skills. We encourage you to share your experiences or ask questions related to MATLAB and skalarprodukt. Your feedback is invaluable as we continue to learn together!