The `numel` function in MATLAB returns the number of elements in an array, regardless of its dimensions.
A = [1, 2, 3; 4, 5, 6];
elementCount = numel(A);
disp(elementCount); % Output: 6
What is `numel`?
`numel` is a fundamental function in MATLAB that returns the number of elements in an array, cell, or structure. Understanding how to utilize this function effectively is crucial for anyone working with data in MATLAB, as it aids in dynamic programming, data analysis, and memory management.
Understanding the Basics of `numel`
How `numel` Works
The `numel` function counts all the elements present in the array, regardless of its dimensionality. For instance, when provided with a two-dimensional array, `numel` computes the total number of elements by multiplying the number of rows by the number of columns.
Handling Different Data Types
Numeric Arrays
When dealing with numeric arrays, `numel` is straightforward. For example:
A = [1, 2, 3; 4, 5, 6];
n = numel(A);
In this code, `numel(A)` returns 6, because there are six elements in the array. This versatility applies to both row and column vectors:
B = [1, 2, 3];
n = numel(B);
Here, `numel(B)` also returns 3, accurately reflecting the number of elements.
Cell Arrays
`numel` is particularly valuable when working with cell arrays that may contain different types of data. Consider the following example:
C = {1, 2, 'text', rand(2,2)};
n = numel(C);
In this case, `numel(C)` will yield 4 since there are four cells in the array, regardless of their contents. This makes `numel` very useful for handling heterogeneous data.
Structures
Structures are another essential data type in MATLAB. With structures, `numel` counts the number of fields rather than values. For example:
S.name = 'John';
S.age = 30;
n = numel(S);
Here, using `numel(S)` will return 1, indicating that the structure `S` contains one main structure. To count the fields, you would typically use `fieldnames` instead.
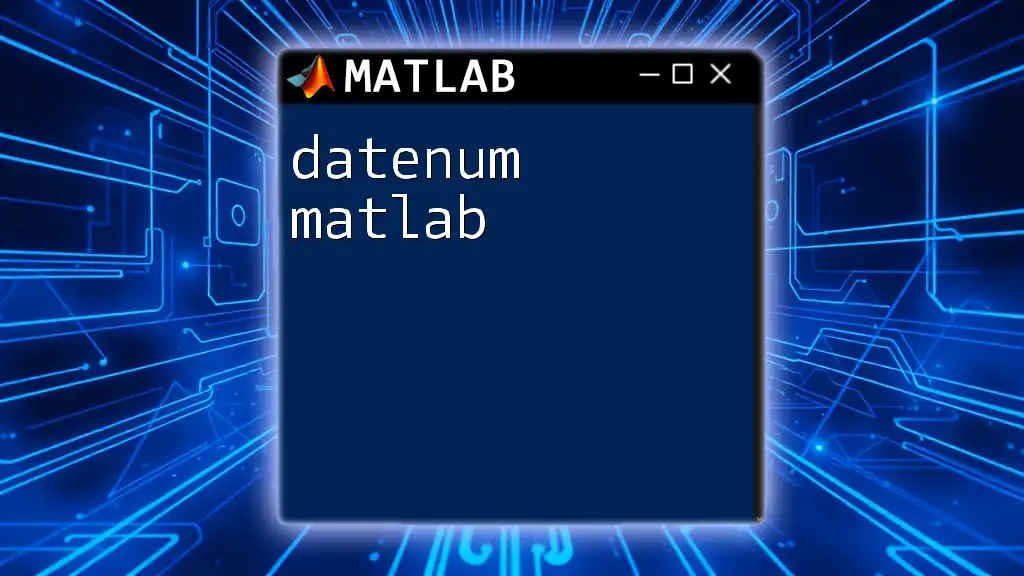
Practical Applications of `numel`
Data Analysis
One common application of `numel` is to assist in data analysis. For instance, analyzing image data dimensions is often crucial in image processing tasks. By using:
img = imread('image.png');
imgElements = numel(img);
You can uncover how many pixel values exist in the image, which is vital for processing large datasets efficiently.
Optimization in Code
Knowing the number of elements in an array can also lead to performance improvements. By using `numel` within loops, you can work with data dynamically, effectively tailoring your code. For example:
for i = 1:numel(A)
% Process each element of A
end
This flexibility allows your code to adapt to the size of the data dynamically, thus enhancing its robustness and efficiency.
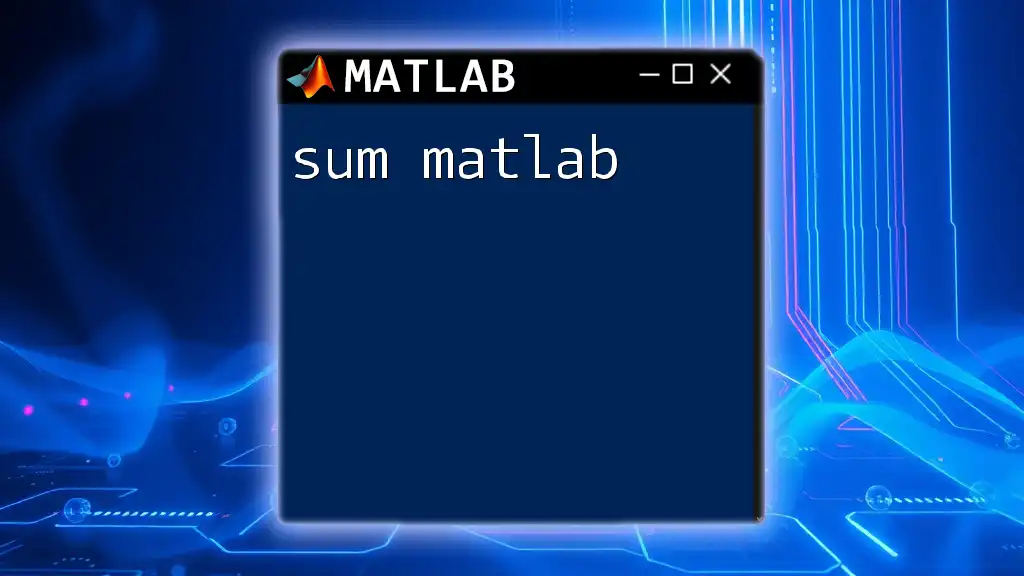
Common Errors and Troubleshooting
Empty Arrays
One common error arises when using `numel` with empty arrays. For instance, if you type:
E = [];
n = numel(E);
The function will return 0, which is expected. Understanding this behavior is vital to avoiding confusion when working with dynamic data that may be empty.
Complex Data Structures
When dealing with deeply nested cell arrays or structures, you may encounter challenges. If you input a complex structure without navigating first to the depth, it can lead to misleading results or confusion. Always ensure you know the structure’s layout before using `numel` on it.
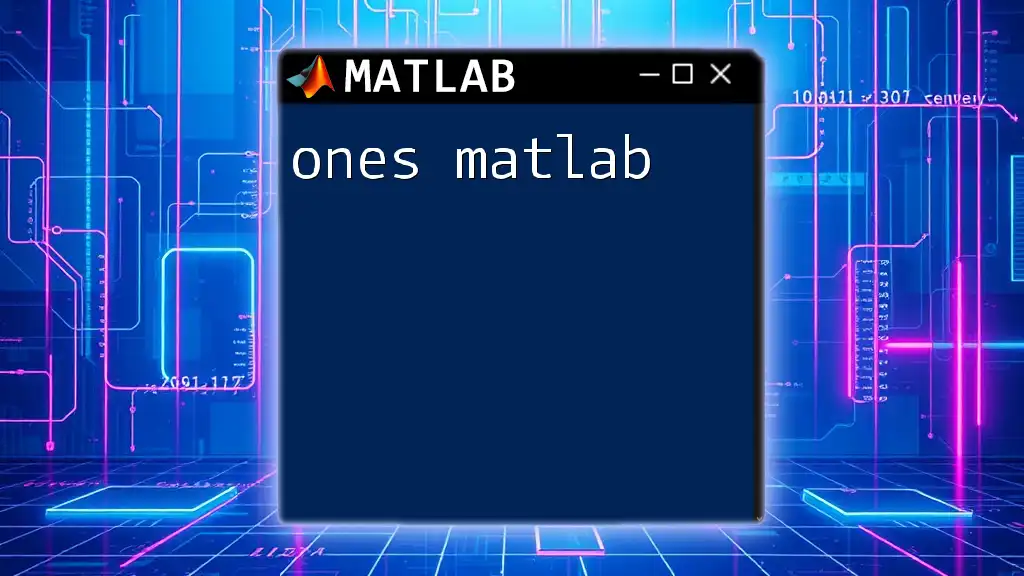
Comparing `numel` with Other Functions
Using `length` and `size`
In MATLAB, while `numel` provides a count of all elements, other functions such as `length` and `size` offer different insights:
- `length`: This function returns the largest dimension of an array. While it’s useful, it might not accurately depict the total number of elements in multi-dimensional arrays.
len = length(A);
- `size`: This function provides the size of each dimension of an array. For example, apply `size(A)` and you’ll get a two-element vector indicating the number of rows and columns.
dims = size(A);
In scenarios where you specifically need to know the total count of elements, `numel` is often the most appropriate choice.
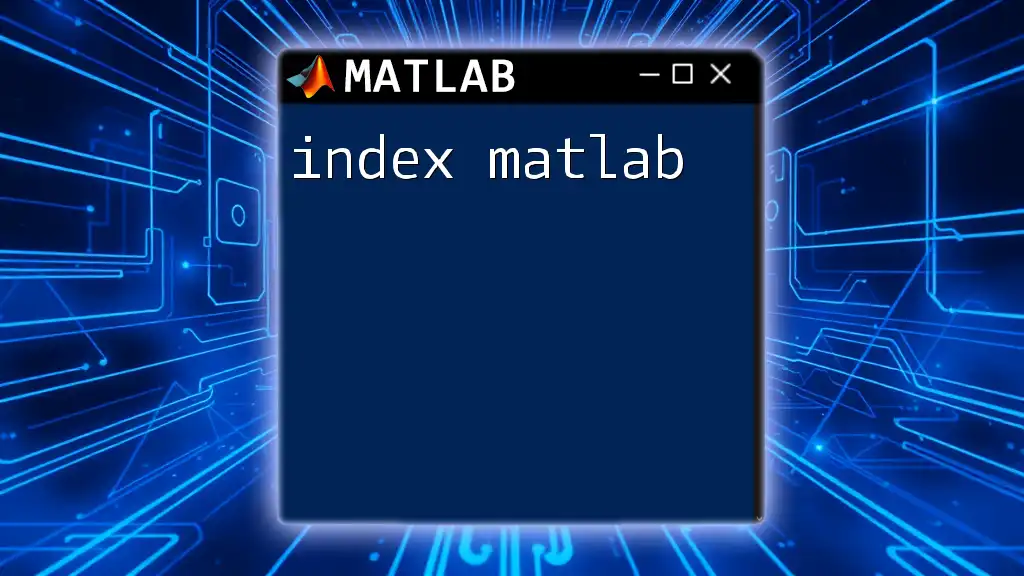
Conclusion
In summary, mastering the `numel` function in MATLAB is instrumental for any data-oriented application. Its efficiency in counting elements across various data types can enhance your data manipulation strategies significantly. Becoming proficient in using `numel` will empower you to write cleaner, more adaptive code and ultimately lead you to more accurate data analysis results.
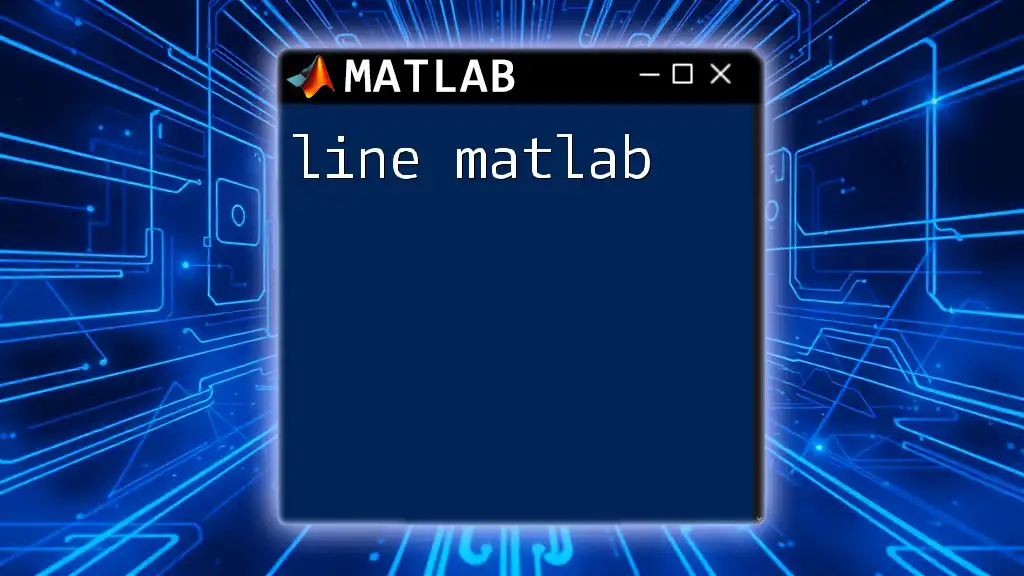
Additional Resources
For further learning, you might want to explore the official MATLAB documentation on `numel`, along with various online courses and forums that specialize in MATLAB programming and data analysis techniques.
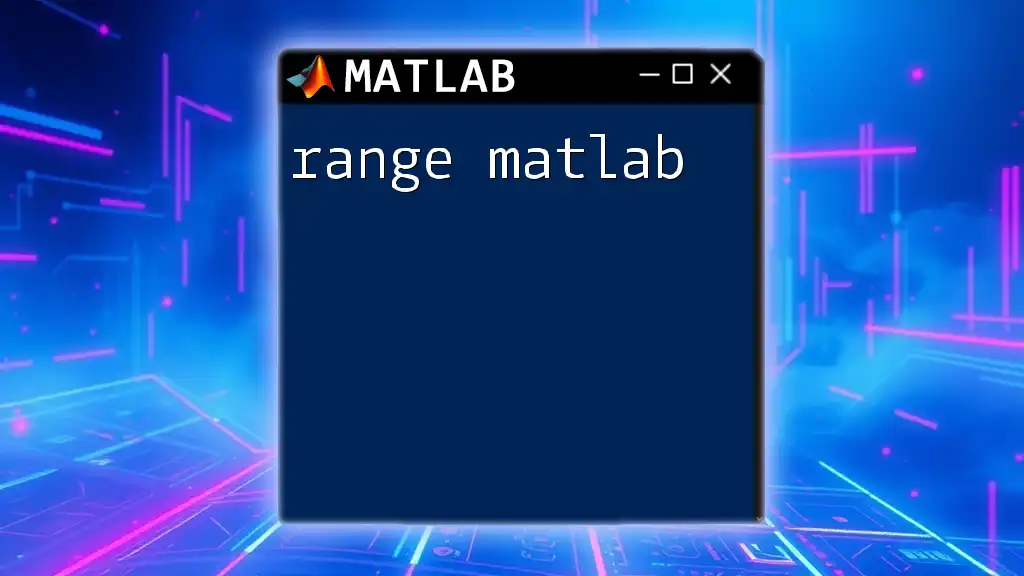
Frequently Asked Questions (FAQs)
What happens if I pass a non-array argument to `numel`? When you pass non-array arguments, `numel` will return 1 for scalar values and produce an appropriate output based on the data type, allowing for seamless integration.
Can I use `numel` with multidimensional arrays? Absolutely! `numel` works effectively with multidimensional arrays to return the total count of elements across all dimensions.
How does `numel` handle NaN values in matrices? `numel` counts all elements, including NaN values, which means that if a matrix contains two NaNs, the `numel` function will still return the total count.