Indexing in MATLAB allows you to access and manipulate specific elements or subsets of arrays and matrices using indices.
% Example of indexing in a 2D matrix
A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % Define a 3x3 matrix
element = A(2, 3); % Access the element in the 2nd row, 3rd column
row_vector = A(1, :); % Extract the entire 1st row
column_vector = A(:, 2); % Extract the entire 2nd column
Understanding MATLAB Arrays
What are Arrays?
Arrays form the backbone of data handling in MATLAB, representing collections of values in a structured manner. They can be one-dimensional (like a simple list), two-dimensional (like a matrix), or even multi-dimensional. Creating an array is straightforward and often done via square brackets.
For example, you can create a 3x3 matrix with:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9];
In this example, matrix `A` contains three rows and three columns, where each element can be individually accessed or manipulated using various indexing techniques.
Why Indexing is Important
Indexing takes array handling to the next level, allowing you to interact with specific elements, rows, or entire segments of data. This capability is essential for various tasks, including:
- Data Analysis: Accessing and analyzing pertinent information.
- Visualization: Extracting data for graphs and plots.
- Algorithm Development: Feeding specific inputs into functions and algorithms.
By grasping how to effectively index matrices and arrays, you can significantly enhance your MATLAB proficiency.
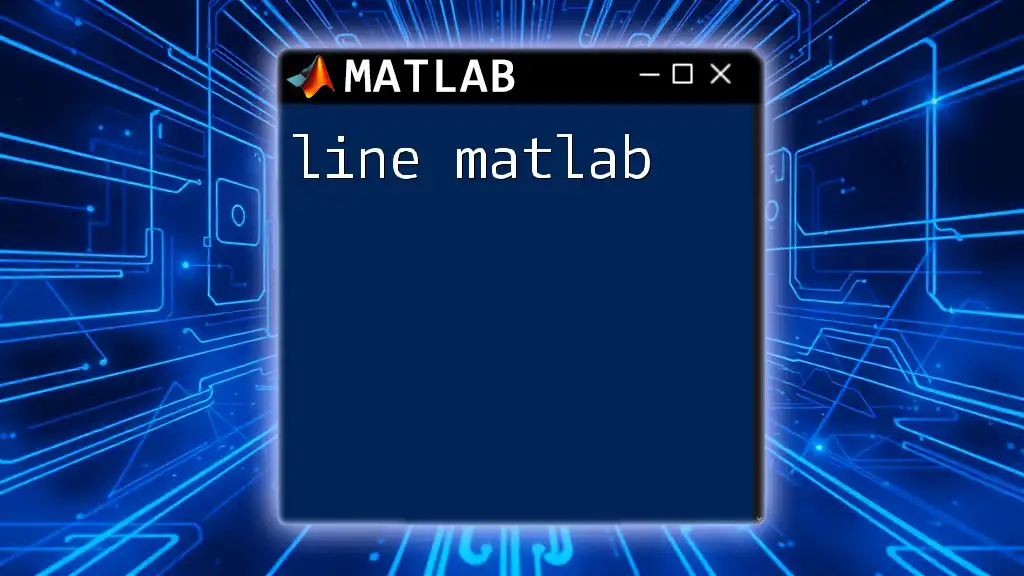
Types of Indexing in MATLAB
Linear Indexing
MATLAB allows you to access array elements using a linear index, which refers to elements in a single column-wise vector. This method is especially useful for one-dimensional arrays but can also be applied to multi-dimensional arrays.
For instance, if you have the matrix `A` previously defined, you can access the element located at the fifth position using:
value = A(5); % Accessing the element in the 5th position
This retrieves the number `5`, as elements are stored in column-major order. Linear indexing is a powerful tool for manipulating data without concern for multi-dimensional structure.
Logical Indexing
How Logical Indexing Works
Logical indexing is a unique way to extract data based on conditions. You create a logical array (containing `true` or `false` values) corresponding to the size of your original array.
For instance:
B = A > 5; % Creates a logical array based on the condition
Here, the logical array `B` indicates which elements of `A` are greater than 5. You can then use this logical array to index into `A` like so:
extractedValues = A(B); % Using logical indexing to retrieve values
This results in an array of all values from `A` that satisfy the condition, which is both efficient and intuitive.
Applications of Logical Indexing
Logical indexing is particularly helpful for filtering data. For example, if you're interested in retrieving values greater than `5`, you can use:
selectedValues = A(A > 5);
This snippet efficiently collects all elements from `A` meeting the specified criterion, which is invaluable in data processing and cleaning.
Subscript Indexing
Working with Row and Column Indices
Subscript indexing allows precise access to elements by specifying both row and column indices. This is particularly useful in two-dimensional arrays, such as matrices.
For example, to retrieve the element at row 2 and column 3 of matrix `A`, you would write:
element = A(2, 3); % Accessing the element at the specified position
Here, the result will be `6`, demonstrating how subscript indexing enables targeted access to matrix data.
Accessing Multiple Elements
You can also use subscript indexing to extract subarrays or slices of your data by specifying ranges. For instance:
rows = A(1:2, 1:2); % Extracting a sub-matrix consisting of the first two rows and columns
This effectively retrieves the top-left `2x2` section of matrix `A`:
1 2
4 5
This flexibility is critical for data manipulation in more complex analyses.
Cell Array Indexing
While standard arrays are essential, cell arrays offer unique capabilities. They allow you to store different types of data in each cell. Accessing data within cell arrays requires curly braces.
Consider the following cell array:
C = {1, 2, 'hello'; 3, 4, 'world'};
To access the string `world`, you would type:
value = C{2, 3}; % Accessing 'world'
This demonstrates how fancy indexing accommodates various data types within a single structure.
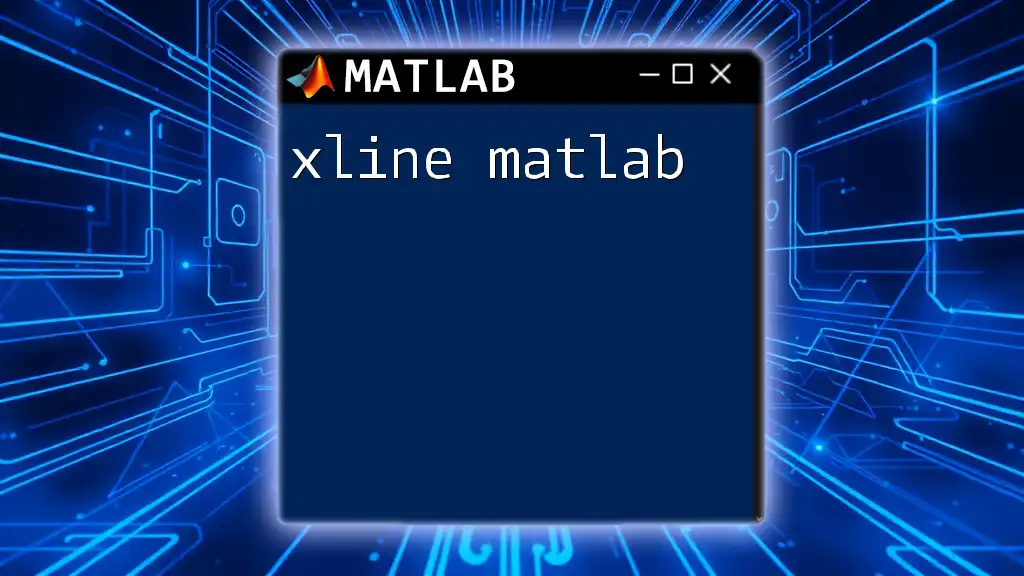
Modifying Elements Using Indexing
Updating Values
Indexing isn't just about reading data; it also enables modifying existing values. For example, you might want to change the value located at row 1, column 1 of matrix `A`. You can achieve this with:
A(1, 1) = 10; % Changing the value at the specified position
After executing this line, the new value of `A(1, 1)` is `10`, reflecting the change in the original array.
Adding and Deleting Elements
You can also use indexing to manage the size of arrays by adding or deleting elements.
To add a new row to matrix `A`, you can do:
A(end + 1, :) = [10, 11, 12]; % Appending a new row
The `end + 1` selector dynamically appends a new row filled with the specified values.
Conversely, if you wish to delete a specific row, you can use:
A(2, :) = []; % Deleting the second row from the matrix
This efficiently modifies the matrix size and structure.
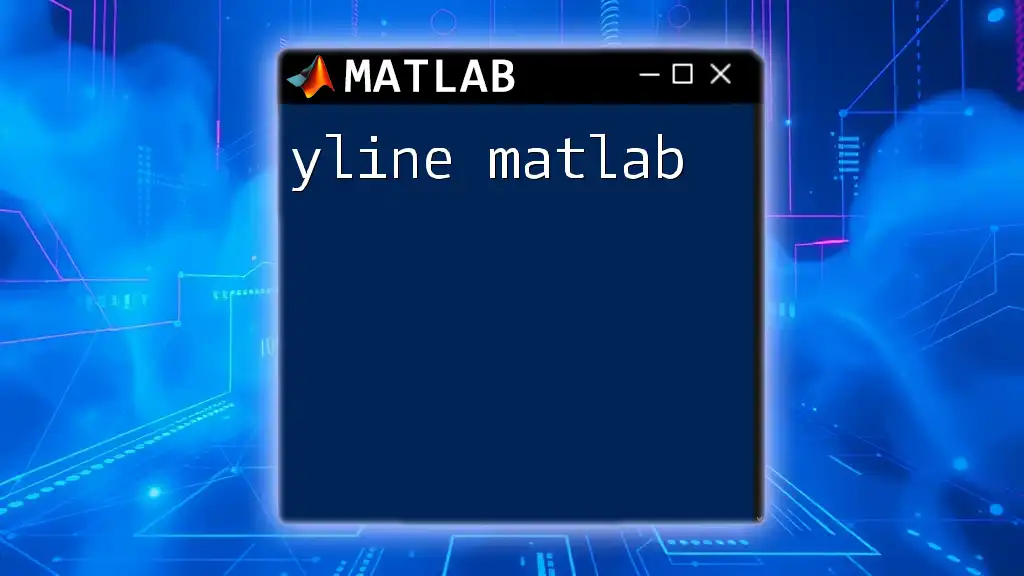
Best Practices for Indexing in MATLAB
Tips for Efficient Indexing
To make the most out of MATLAB indexing, consider implementing the following best practices:
- Preallocation: When working with large datasets, preallocate space for your arrays instead of dynamically resizing them. This enhances performance by minimizing memory fragmentation.
A = zeros(1000, 1); % Preallocate a column vector of size 1000
- Vectorization: Opt for vectorized operations where possible, which can lead to faster execution compared to using loops that rely heavily on indexing.
Common Mistakes to Avoid
While indexing is straightforward, there are pitfalls to be aware of:
-
Index Out of Bounds: Attempting to access elements outside the size of the array will lead to errors. Always check your array dimensions using the `size()` function.
-
Mismatched Dimensions: Ensure that your logical indices match the dimensions of the array they are indexing. Mismatched dimensions can cause errors in execution.
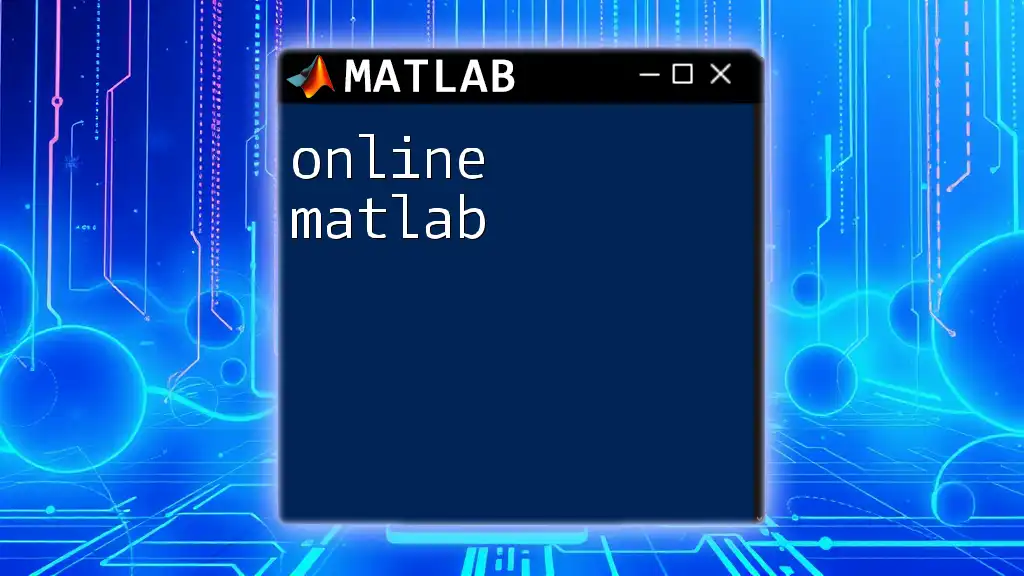
Conclusion
Understanding how to implement effective indexing in MATLAB is vital for any aspiring engineer or data scientist. By mastering linear, logical, and subscript indexing, you can unlock the full potential of MATLAB for your projects. The examples provided in this guide illustrate the various ways to manipulate arrays and facilitate robust data handling—key skills in any computational toolkit.
Continue practicing these techniques to enhance your versatility in MATLAB, and explore more advanced topics to further cement your learning!
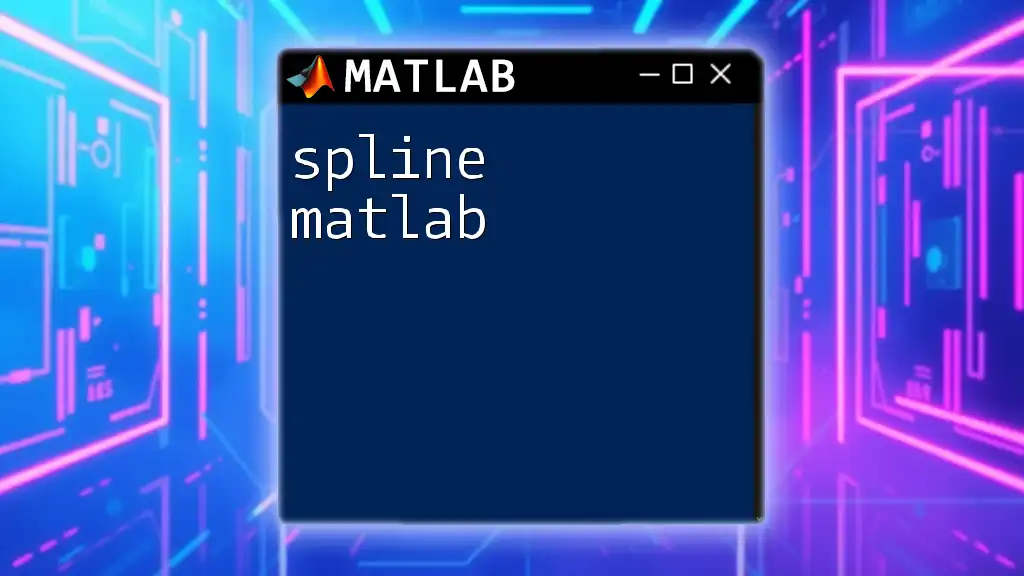
Additional Resources
For those looking to deepen their knowledge, refer to the [official MATLAB documentation](https://www.mathworks.com/help/matlab/indexing.html) and explore additional online courses and tutorials tailored to MATLAB programming.