The `xline` function in MATLAB is used to draw vertical lines on a plot at specified x-values, enhancing visual representation of data points or regions.
Here's a code snippet to illustrate its usage:
x = 0:0.1:10;
y = sin(x);
plot(x, y);
xline(5, 'r--', 'x = 5'); % Draws a red dashed vertical line at x = 5
What is `xline`?
`xline` is a powerful function in MATLAB that allows users to draw vertical lines on graphs at specified x-axis locations. This essential tool serves the purpose of marking specific values or thresholds in a plot, making it easier for viewers to interpret data visually. Vertical lines can highlight important events, signal boundaries, or indicate specific trends, thus enriching the overall graphical presentation.
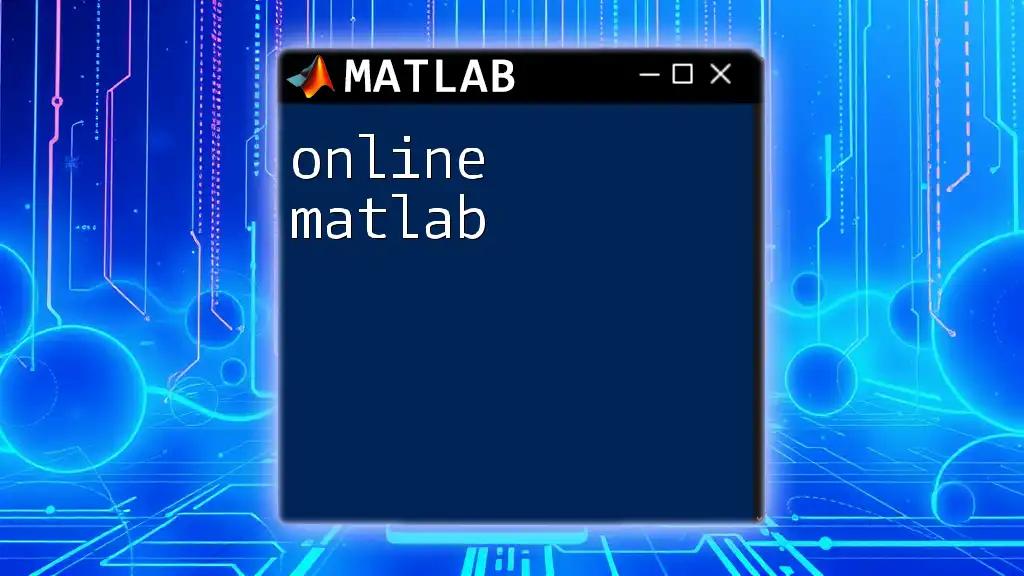
Benefits of Using `xline`
Utilizing `xline` comes with several advantages:
-
Ease of Use: Adding vertical lines is straightforward and requires minimal code, which is especially beneficial for quick data visualization tasks.
-
Customization Options: MATLAB provides various customization parameters for `xline`, allowing you to adjust colors, line styles, and widths, enabling you to tailor your plots to your specific needs.
-
Clarity in Visualization: By incorporating vertical lines, you enhance the understanding of plots, making complex data more accessible.
-
Versatile Use Cases: Whether you're analyzing financial data, scientific measurements, or time series data, `xline` can be a beneficial addition to your graphical toolbox.
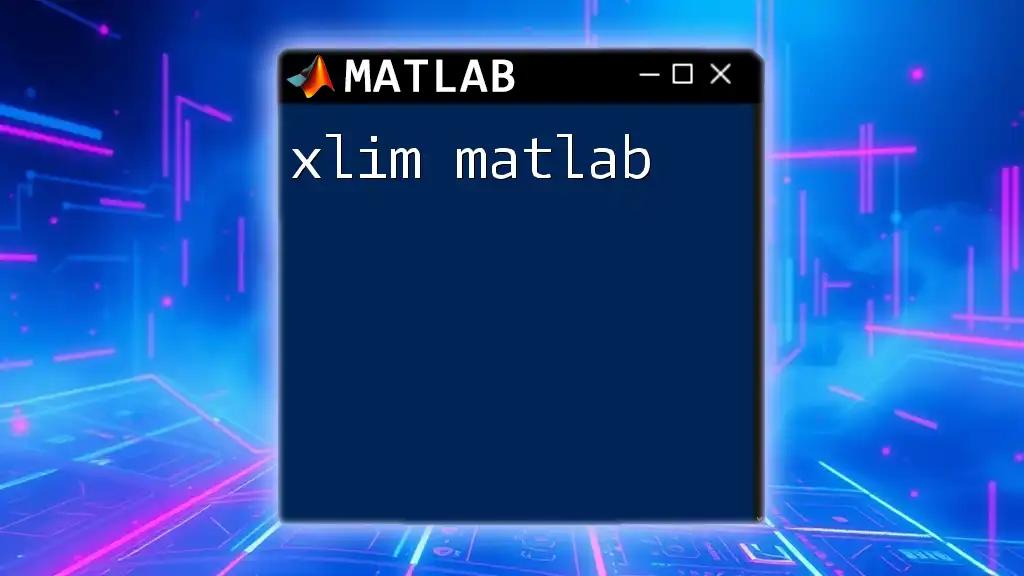
Syntax of `xline`
The basic syntax to implement `xline` is:
xline(x)
Here, `x` represents the value on the x-axis where you want the line to be drawn. This simplicity allows for efficient plotting.
In addition, `xline` includes several optional parameters that allow for customization, enhancing the visual impact of your plots.
For example:
xline(x, 'Label', 'My Line');
In this syntax, 'Label' provides a textual identifier for the line.
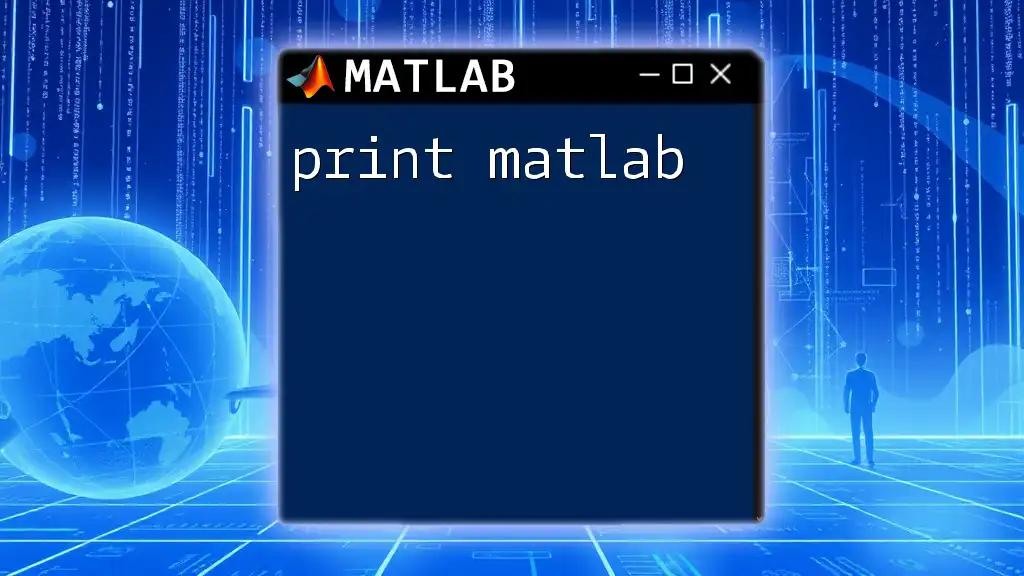
Customizing `xline`
Line Properties
Color
To change the color of your vertical line, you simply add the `'Color'` parameter:
xline(x, 'color', 'r'); % Red line
This customization ensures that the vertical line stands out against the plot background, enhancing visibility.
Line Style
You can modify the line style by choosing from options like solid, dashed, or dotted. For a dashed line:
xline(x, '--'); % Dashed line
This flexibility allows you to choose the line style that best fits your data representation goals.
Line Width
To make your lines thicker or thinner, adjust the `LineWidth` parameter:
xline(x, 'LineWidth', 2); % Thicker line
A thicker line can emphasize key values of interest, while a normal width may suffice for less critical markers.
Text Labels
Adding descriptive labels to vertical lines significantly aids in interpreting the plot. You can include a label with your line as follows:
xline(x, 'Label', 'My Line');
Applying clear labels is crucial in data analysis, as it helps convey meaning and context.
Multiple Lines
You can also draw multiple vertical lines in a single plot, which is helpful for marking several key values:
xline([x1 x2], 'Label', 'Two Lines');
This feature allows for sophisticated visual analysis by showing more than one significant point on a single graph.
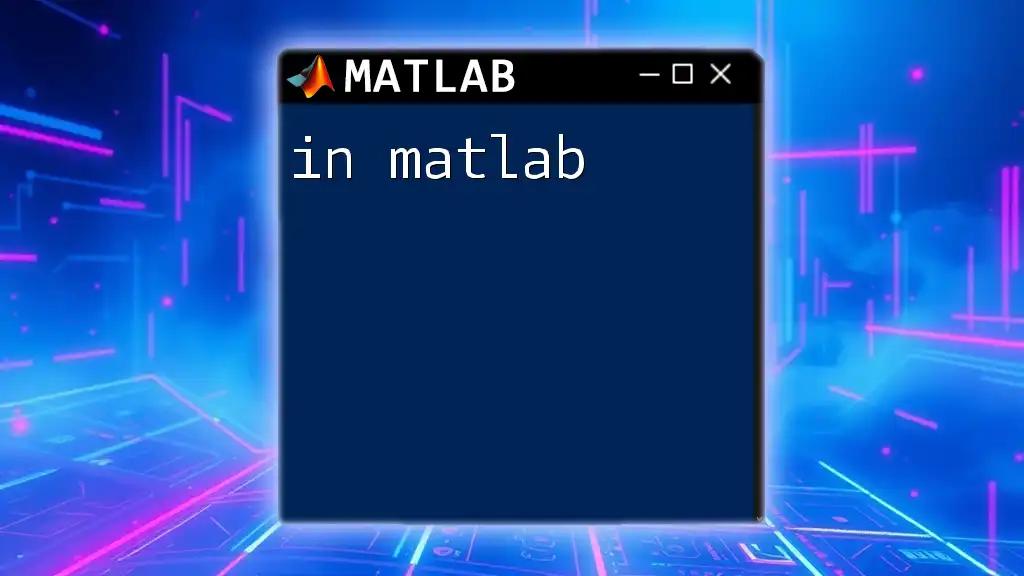
Examples of `xline` in Action
Basic Example
Consider a simple plot where we want to draw a single vertical line at \(x = 3\):
x = 3;
plot(1:10);
xline(x, 'Color', 'g', 'LineWidth', 2);
title('Basic Example of xline');
xlabel('X-axis');
ylabel('Y-axis');
This code will produce a plot with a green vertical line at \(x = 3\). Such simple implementations demonstrate how `xline` can enhance visual clarity.
Advanced Example
In a more advanced scenario, let’s add multiple vertical lines with varied styles and labels:
x = [2, 5, 7];
plot(1:10);
xline(x(1), 'r', 'LineWidth', 2, 'Label', 'Line 1');
xline(x(2), 'g--', 'Label', 'Line 2');
xline(x(3), 'b:', 'LineWidth', 3, 'Label', 'Line 3');
title('Advanced Example of xline');
In this example, three different vertical lines are depicted, each styled uniquely, facilitating an engaging and informative visualization.
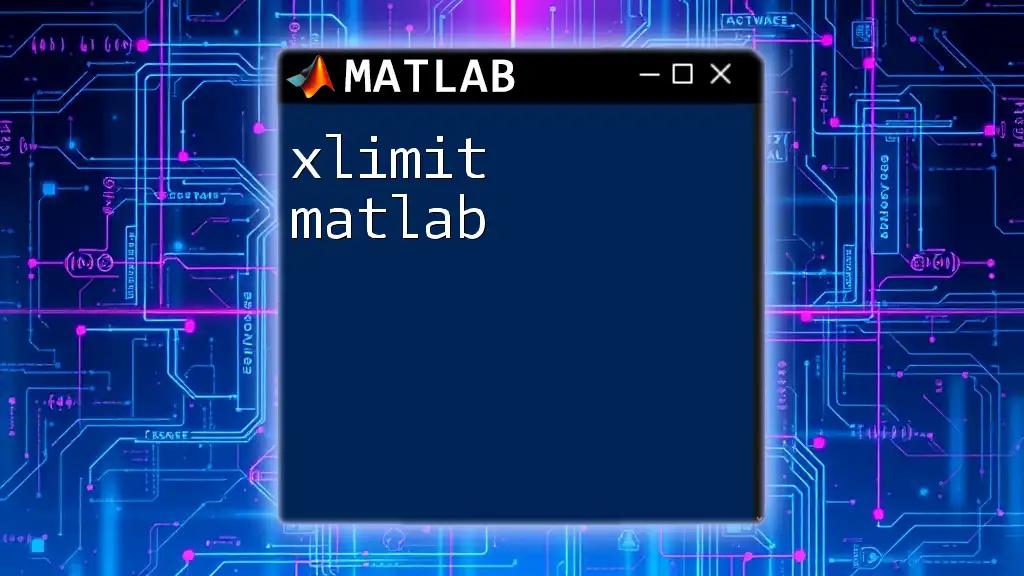
Common Use Cases for `xline`
Understanding where to apply vertical lines can significantly enhance your data visualization efforts. Here are some common use cases:
-
Highlighting Thresholds: Use vertical lines to denote significant results, statistical thresholds, or target values in your data set.
-
Marking Events: When analyzing time series data, vertical lines can indicate key events (e.g., economic policy changes, equipment failures), making those moments stand out.
-
Visualizing Inflection Points: In analyzing trends, vertical lines can mark points where significant changes occur, aiding your audience in grasping inflection points quickly.
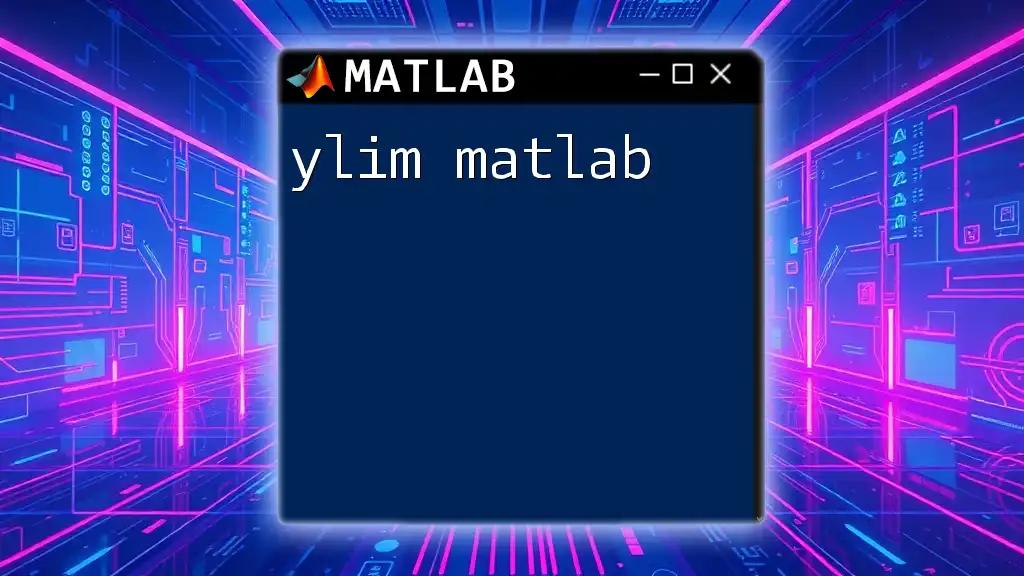
Troubleshooting Common Issues
Despite its usefulness, users may face common challenges:
-
Lines Not Appearing as Expected: Often, missing or misplaced lines can result from incorrect x-values or plotting errors. Double-check the values you’ve specified and ensure they are correctly within the x-axis range.
-
Overlapping Lines: If multiple lines are too close to one another, they may overlap, making interpretation difficult. Adjusting colors, styles, or line widths can help in distinguishing them more clearly.
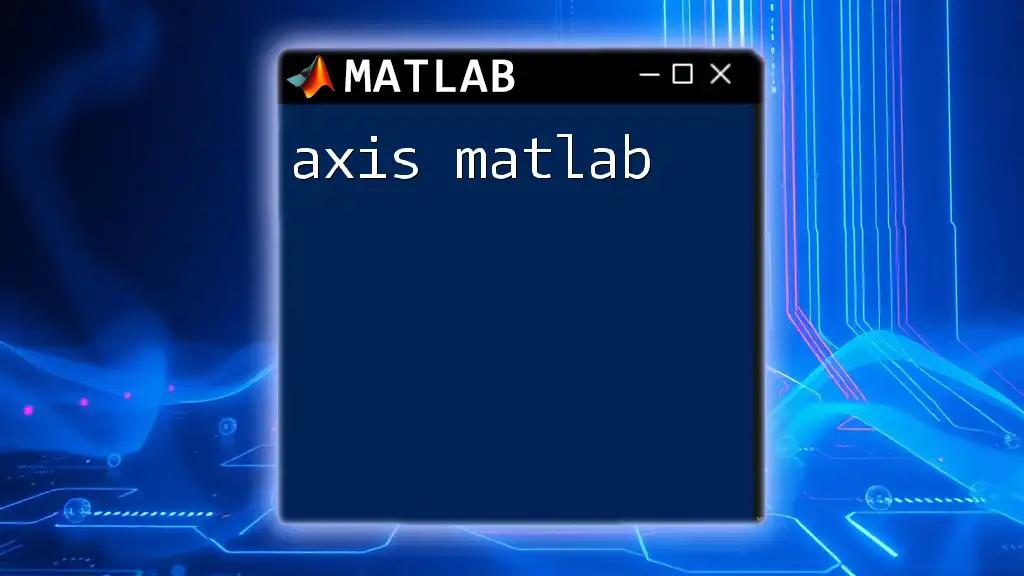
Conclusion
In summary, the `xline` function in MATLAB is a robust tool that simplifies the process of adding vertical lines to plots. With its straightforward syntax and extensive customization options, `xline` helps transform complex datasets into visually understandable insights. Experimenting with these functionalities will enable you to elevate your data visualizations and communicate your findings more effectively.
For those eager to dive deeper into this function, MATLAB's official documentation and community forums can be invaluable resources and are excellent places to seek assistance as you explore the full potential of the `xline matlab` command.