The `range` function in MATLAB computes the difference between the maximum and minimum values of a dataset, providing a measure of spread.
Here’s how you can use it:
data = [2, 5, 9, 3, 7];
range_value = range(data);
disp(range_value);
Understanding Range in MATLAB
Definition of Range
In the context of MATLAB, the term range refers to the difference between the maximum and minimum values of a dataset. Mathematically, the range can be expressed as:
\[ \text{Range} = \text{Maximum Value} - \text{Minimum Value} \]
This concept is crucial in various analyses, as it provides insight into the spread of data points. Understanding the range helps in assessing the variability of a dataset, aiding decision-making and statistical modeling.
The Range Function in MATLAB
MATLAB provides a built-in function to calculate the range of a dataset quickly. The syntax for the `range` function is straightforward:
r = range(X)
In this syntax, `X` is the input dataset, which can be a vector, matrix, or multidimensional array. The output `r` will be a scalar representing the range of the input values.
Why Use the Range Function?
Using the built-in range function in MATLAB offers significant advantages over manual calculations, such as:
- Efficiency: The function is optimized for performance, allowing it to compute the range rapidly even for large datasets.
- Readability: Utilizing built-in functions makes your code cleaner and easier for others to understand, enhancing collaboration and maintenance.
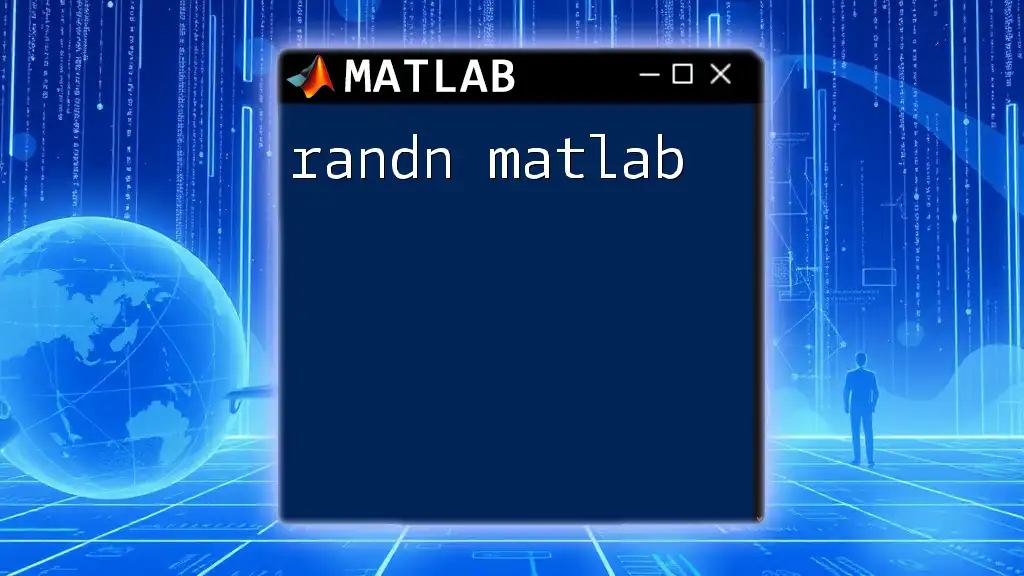
Calculating Range in MATLAB
Basic Usage of the Range Function
To illustrate the use of the `range` function in MATLAB, consider the following simple example:
data = [5, 10, 15, 20, 25];
r = range(data);
disp(['The range of the data is: ', num2str(r)]);
In this example, the dataset `data` contains five values. By invoking the `range` function, we efficiently find that the range is 20. The output confirms this:
The range of the data is: 20
This example demonstrates how MATLAB can simplify the process of calculating data attributes.
Range in Multidimensional Arrays
MATLAB allows the calculation of range across both rows and columns in a matrix. The syntax indicates which dimension you want to consider—`1` for columns and `2` for rows.
matrix = [1, 4, 9; 4, 5, 6; 2, 8, 3];
rowRange = range(matrix, 2); % Range across rows
colRange = range(matrix, 1); % Range across columns
disp(['Row range: ', num2str(rowRange')]);
disp(['Column range: ', num2str(colRange)]);
The output of this code will show the range for each row and column:
Row range: [8 4 6]
Column range: [3 4 6]
This feature is particularly useful in data analysis, allowing users to quickly quantify variations within multidimensional datasets.
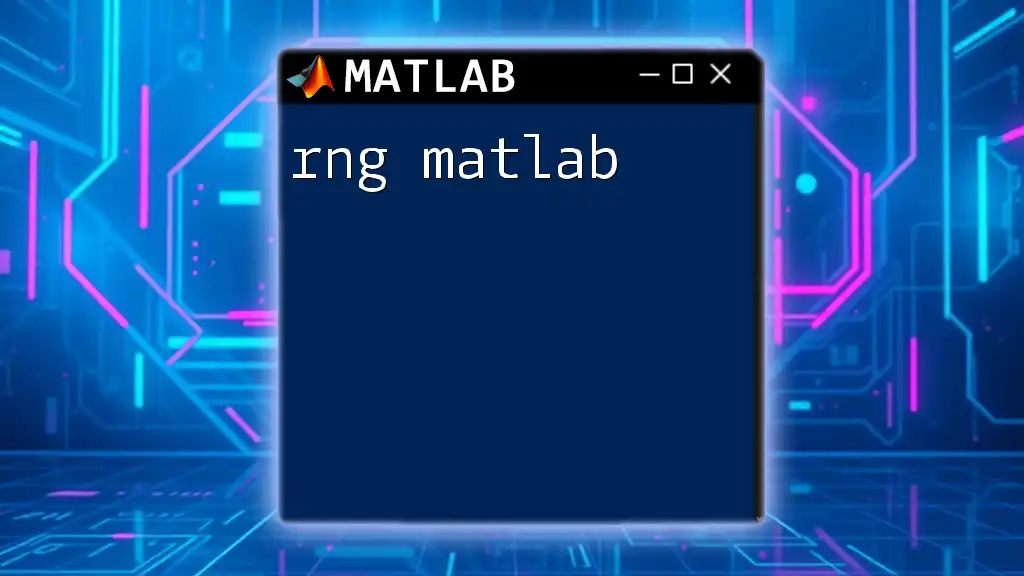
Custom Range Function
Creating Your Own Function
While the built-in `range` function is powerful, there may be instances where a custom function is necessary. These situations could arise when dealing with datasets that require special handling or specific criteria.
Example Code Snippet for Custom Function
To create a custom range function, it can be defined as follows:
function r = customRange(data)
r = max(data) - min(data);
end
In this function, we calculate the range by subtracting the minimum value from the maximum value. This script is an illustration of how you can make the calculation explicit and customizable, thereby allowing for further modifications as per your analysis needs.
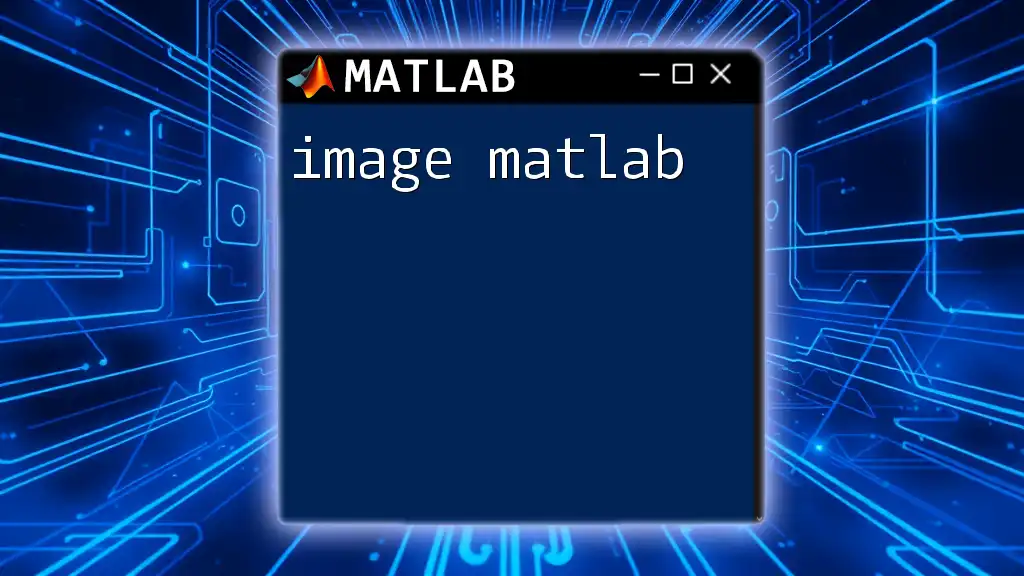
Visualizing Data Range
Plotting Data with Range Indicators
Visualization is a crucial aspect of data analysis, and incorporating range information can greatly enhance the interpretability of your data. MATLAB provides powerful plotting functions that allow you to visualize the range effectively.
Example Code Snippet for Visualization
Here's how to plot data along with indicators for the minimum and maximum values:
x = 1:10;
y = [2, 3, 5, 6, 8, 5, 6, 7, 10, 9];
rangeValue = range(y);
plot(x, y, '-o'); hold on;
yline(min(y), 'r--', 'Min Value');
yline(max(y), 'b--', 'Max Value');
title(['Data Range Visualization: ', num2str(rangeValue)]);
xlabel('X-axis');
ylabel('Y-axis');
When this code is executed, a plot will display the series of data points, along with horizontal dashed lines indicating the minimum (in red) and maximum (in blue) values. This visual representation not only highlights the range but also allows viewers to grasp the dataset’s distribution at a glance.
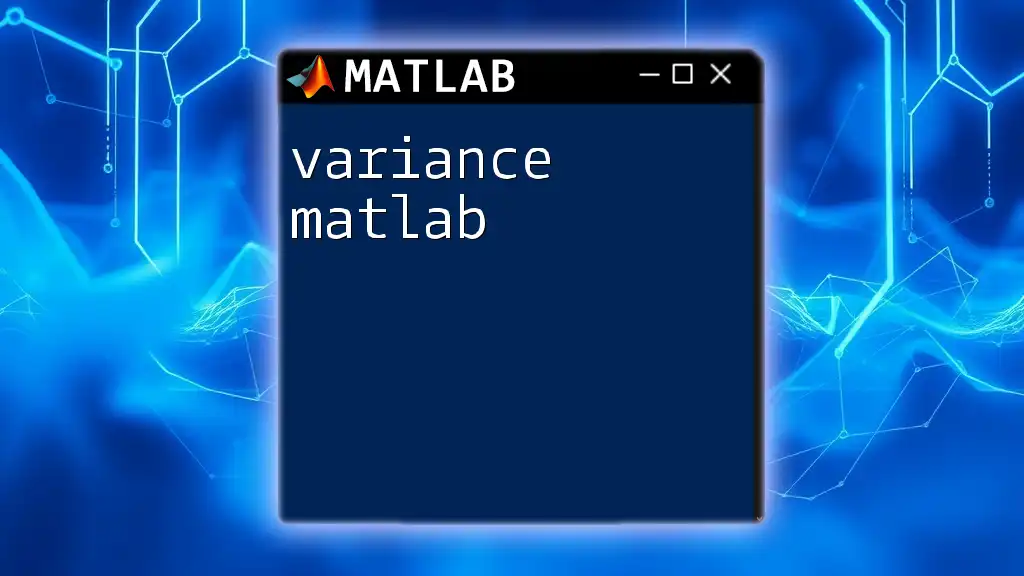
Common Errors and Troubleshooting
Frequently Encountered Issues
While using the `range` function, users may encounter certain common errors, such as:
- Data Type Issues: Ensure the input to the `range` function is numeric. Passing strings or complex data types can result in errors.
- NaN Values Handling: If your dataset includes Not-a-Number (NaN) values, the function may produce unexpected results. Consider preprocessing your data to handle these values effectively.
To avoid issues, ensure your data is clean and formatted correctly before performing range calculations.
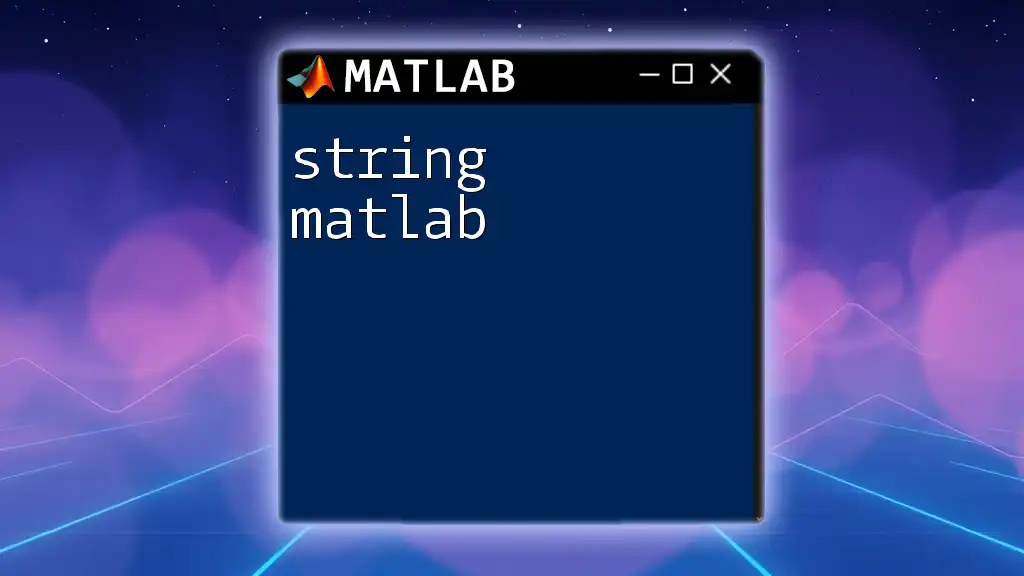
Conclusion
Understanding how to use the `range` function in MATLAB is essential for anyone involved in data analysis. From efficiently computing range values to visualizing them effectively, MATLAB’s capabilities greatly simplify the process. The efficiency, readability, and support for multidimensional datasets make the range function a must-know tool for programmers and analysts alike.
With the foundation laid in this guide, readers are encouraged to practice and explore further applications of this valuable function through hands-on experimentation with various datasets. Embrace the power of MATLAB and harness the insights provided by understanding your data’s range.
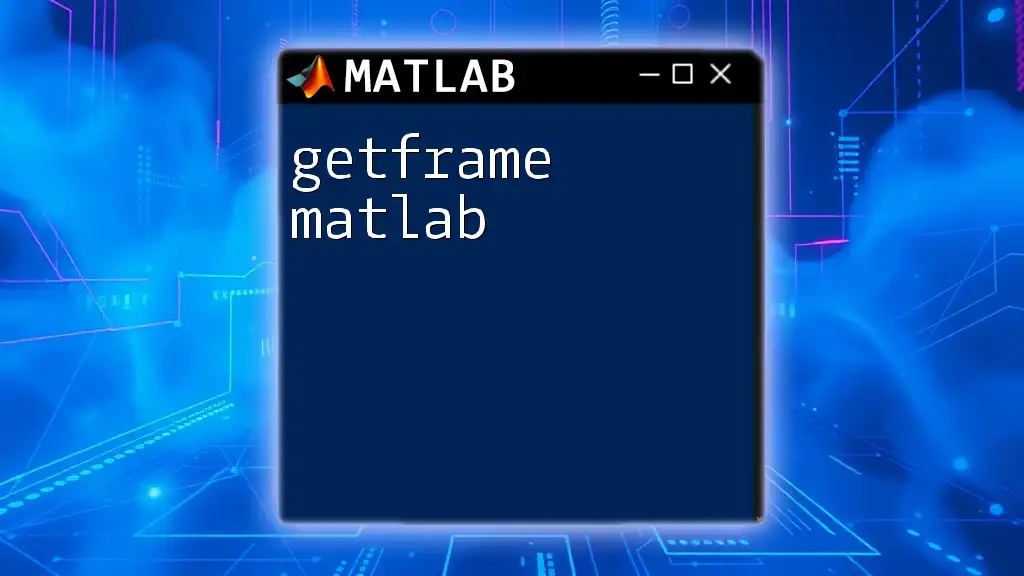
Additional Resources
Recommended Reading
For those eager to dive deeper into MATLAB functionalities, consider exploring books, articles, and tutorials that cover more advanced commands and general MATLAB best practices.
Online Communities and Forums
Joining online forums such as MATLAB Central and Stack Overflow is a great way to connect with other users, seek help, and exchange ideas regarding MATLAB-related queries.
Final Tip
Practice makes perfect! Delve into the code provided in this guide, experiment with different datasets, and don’t hesitate to create your own custom functions to enhance your MATLAB skills further.