In MATLAB, the `sum` function is used to calculate the sum of array elements along a specified dimension, with the default being the first non-singleton dimension.
% Example of summing elements in a vector
vector = [1, 2, 3, 4];
total = sum(vector); % total will be 10
Introduction to the `sum` Function
The `sum` function in MATLAB is a powerful tool for performing summation operations on arrays and matrices. Summation is a fundamental operation in numerical computing, and understanding how to effectively use the `sum` function can significantly enhance your data analysis capabilities in MATLAB.

Syntax and Usage of `sum`
Basic Syntax
The basic structure of the `sum` function is straightforward:
result = sum(A)
In this syntax, `A` is the input array or matrix whose elements you wish to sum, and `result` will store the outcome of the summation.
Parameters of the `sum` Function
The `sum` function has a couple of parameters:
- Input Arguments:
- `A`: The array or matrix from which to calculate the sum.
- `dim` (optional): Specifies the dimension along which the summation is performed. By default, if not specified, MATLAB sums along the first dimension whose size does not equal one.
Output of the `sum` Function
The output of the `sum` function varies depending on the dimensions and types of the input. If the input is a vector, the output will be a scalar. For matrices, the output can either be a row vector (summing across columns) or a column vector (summing across rows).

Summation Across Different Dimensions
Summing Across Rows and Columns
You can use the `dim` argument in the `sum` function to specify which dimension to sum. Here’s how to do it:
rowSum = sum(A, 1); % Sum across columns
colSum = sum(A, 2); % Sum across rows
This allows you to tailor your summation to your specific needs.
Example Code Snippets with Explanations
Consider the following matrix:
A = [1, 2;
3, 4;
5, 6];
When summing across columns:
rowSum = sum(A, 1) % Output: [9, 12]
Here, `rowSum` gives you the sum of each column. Conversely, summing across rows:
colSum = sum(A, 2) % Output: [3; 7; 11]
In this case, `colSum` provides the total for each row.

Working with Different Data Types
Summing Vectors
When it comes to vectors, the summation is quite direct. For instance:
v = [1, 2, 3, 4];
total = sum(v); % Output: 10
This computes the total of all elements in the vector `v`.
Summing Matrices
For multi-dimensional arrays, the `sum` function gracefully handles matrices. MATLAB's versatility shines as it allows summation over specified dimensions.
Summing Complex Numbers
MATLAB natively supports complex numbers, and the `sum` function can effectively handle them without any special considerations.
c = [1+2i, 3+4i];
complexSum = sum(c); % Output: 4 + 6i
The output showcases how the real and imaginary parts are summed separately.
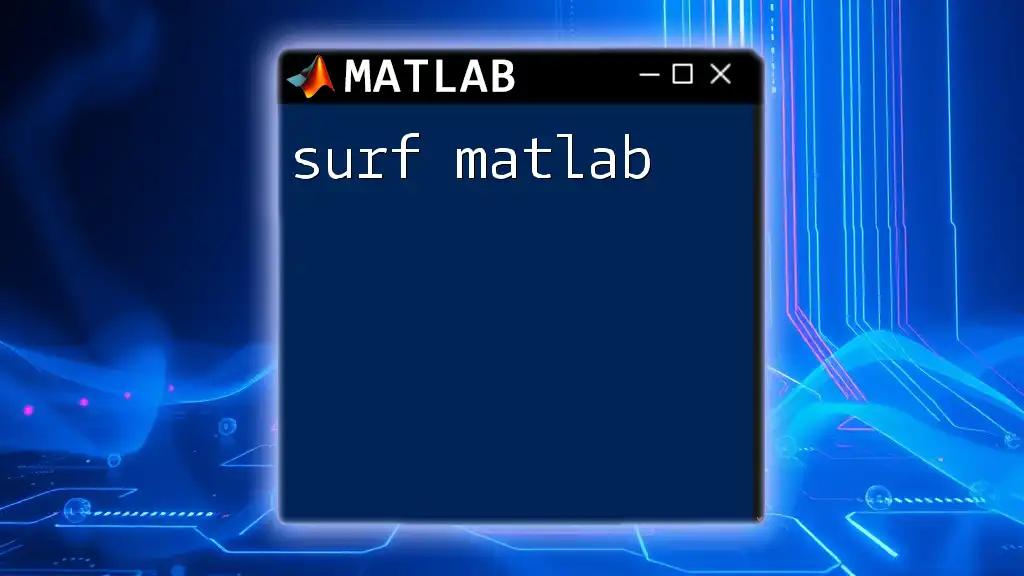
Sum with Conditions
Using Logical Indexing
You can also perform conditional summation using logical indexing—a powerful feature in MATLAB. This enables you to sum only those elements that meet certain criteria:
A = [1, 2, 3, 4, 5];
sumOfEvens = sum(A(mod(A, 2) == 0); % Output: 6
In this case, `sumOfEvens` sums only the even numbers present in the array.
Example Scenarios
Consider a scenario where you might want to sum student scores that exceed a certain threshold:
scores = [75, 85, 90, 60, 55];
highScores = sum(scores(scores > 70)); % Output: 265
This showcases how conditional summation can be applied to extract meaningful totals from datasets.
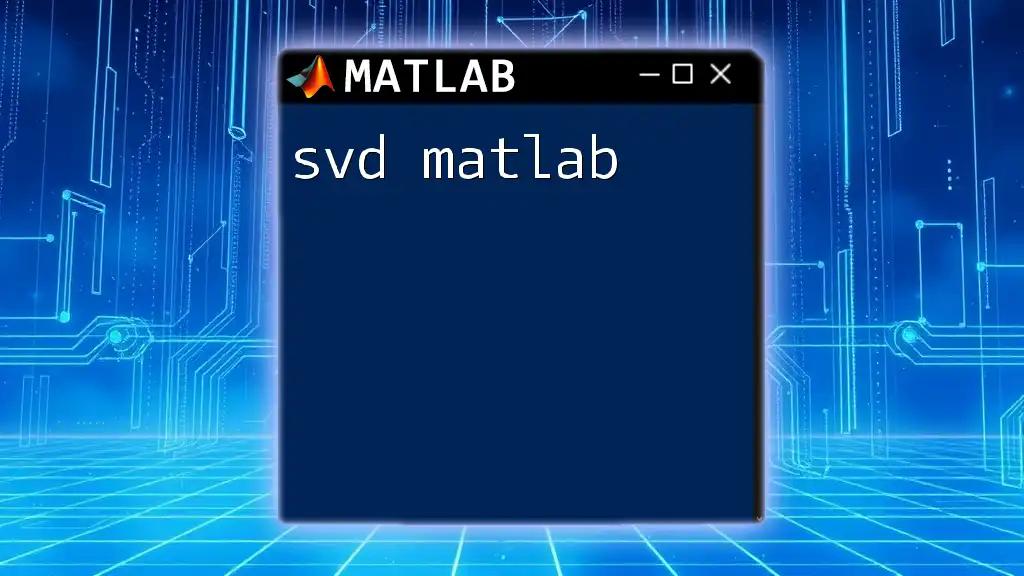
Performance Considerations
Vectorization vs. Loops
An important aspect of using `sum` is the choice between vectorized operations and traditional loops. MATLAB is optimized for vector and matrix operations, meaning using `sum` is generally faster than implementing loops for the same task.
For instance, instead of using:
total = 0;
for i = 1:length(v)
total = total + v(i);
end
Utilizing `sum` is not only syntactically simpler but also computationally more efficient:
total = sum(v);
Profiling Code Performance
To ensure your code runs optimally, MATLAB provides tools like the Profiler. This tool allows you to measure the execution time of your functions and efficiently pinpoint bottlenecks, enabling you to refine your summation tasks.

Practical Applications of the `sum` Function
Data Analysis
The `sum` function is often employed in descriptive statistics, especially when calculating totals which lead to averages:
data = [12, 15, 23, 42];
meanValue = sum(data) / length(data); % Average calculation
Financial Calculations
In finance, summation is crucial for calculating total expenses, revenues, or profits. For example:
expenses = [300, 450, 200];
totalExpenses = sum(expenses); % Output: 950
Simulation Scenarios
In simulation models, such as Monte Carlo methods, the `sum` function can be used extensively to collect and analyze the results of simulations, highlighting its versatility across different domains.
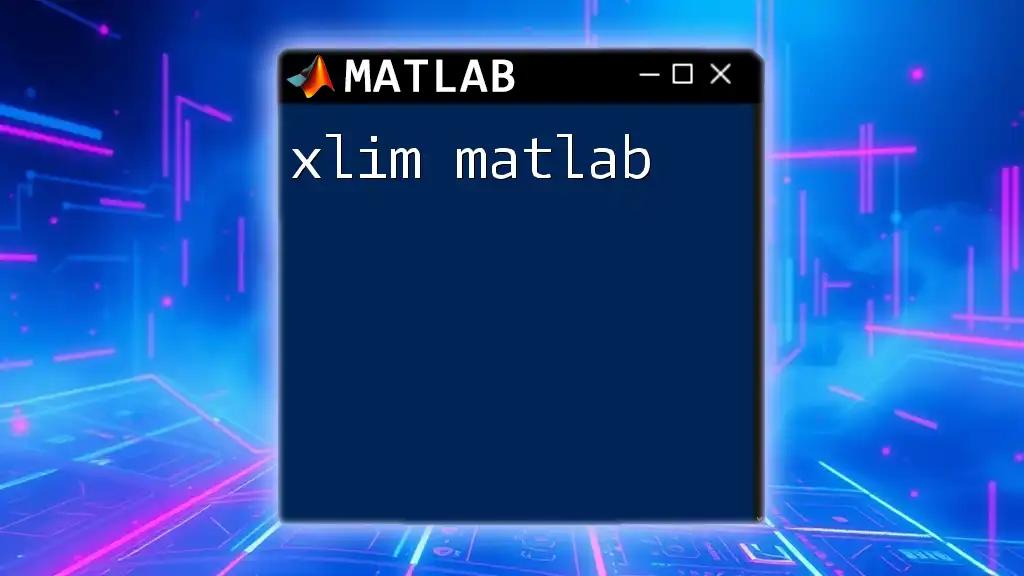
Troubleshooting Common Issues
Common Mistakes and Pitfalls
Beginners often face common pitfalls, such as:
- Mismatched Dimensions: Ensure the dimensions of matrices align when performing operations.
- Not Specifying the Dimension: Forgetting to specify the dimension could lead to unexpected output.
Being aware of these issues helps you avoid them and use `sum` effectively.
Debugging Techniques
When debugging code involving summation, utilize MATLAB’s `disp()` or `fprintf()` function to display intermediate values and spot errors in logic or data handling:
disp(rowSum);
This helps in tracing where the summation might be going wrong.
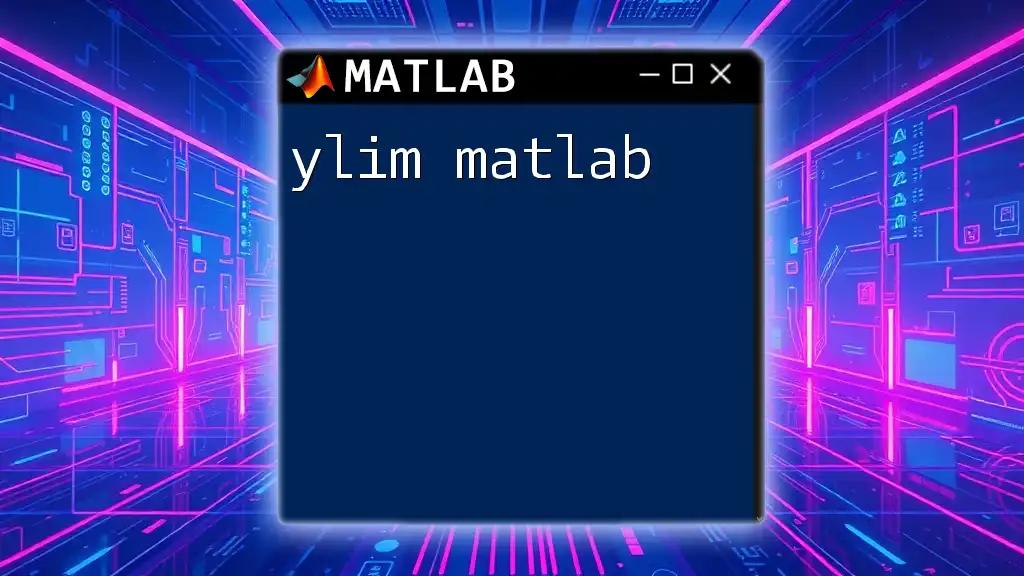
Conclusion
Understanding the `sum` function in MATLAB is vital not only for performing basic arithmetic but also for engaging in complex data analysis. With its versatility and powerful capabilities, mastering this function opens doors for more advanced computations and effective problem-solving. Continue exploring the extensive documentation and resources available to enhance your MATLAB skills further.
Engage in practice exercises that apply what you've learned, and experimenting hands-on is key to truly understanding how to harness the power of summation in MATLAB.