The `fminunc` function in MATLAB is used to find the minimum of an unconstrained multivariable function, optimizing the parameters via gradient-based algorithms.
Here's a code snippet demonstrating its usage:
% Define the objective function
objFun = @(x) (x(1)^2 + x(2)^2);
% Initial guess
x0 = [1, 2];
% Call fminunc to find the minimum
options = optimoptions('fminunc','Display','iter');
[x, fval] = fminunc(objFun, x0, options);
Understanding fminunc
What is fminunc?
`fminunc` is a MATLAB function used for unconstrained optimization. It finds the minimum of an objective function of several variables where the function is smooth, meaning it has continuous first derivatives. The primary appeal of fminunc lies in its ability to handle non-linear and complex functions effectively.
It's essential to understand the difference between constrained and unconstrained optimization. Constrained optimization deals with problems where certain constraints (like limits on variable values) need to be satisfied, whereas unconstrained optimization focuses purely on minimizing a function without any restrictions.
Key Features of fminunc
- Gradient-Based Optimization: `fminunc` utilizes gradient information to find the minimum. While this helps in faster convergence, it assumes that the function is differentiable.
- Handles Non-linear Objective Functions: The function is adept at handling complicated functions, making it suitable for various applications, from engineering to finance.
- Smooth Function Requirement: Since `fminunc` relies on gradients, it’s best utilized with functions that are smooth, ensuring that the derivatives are available and continuous.
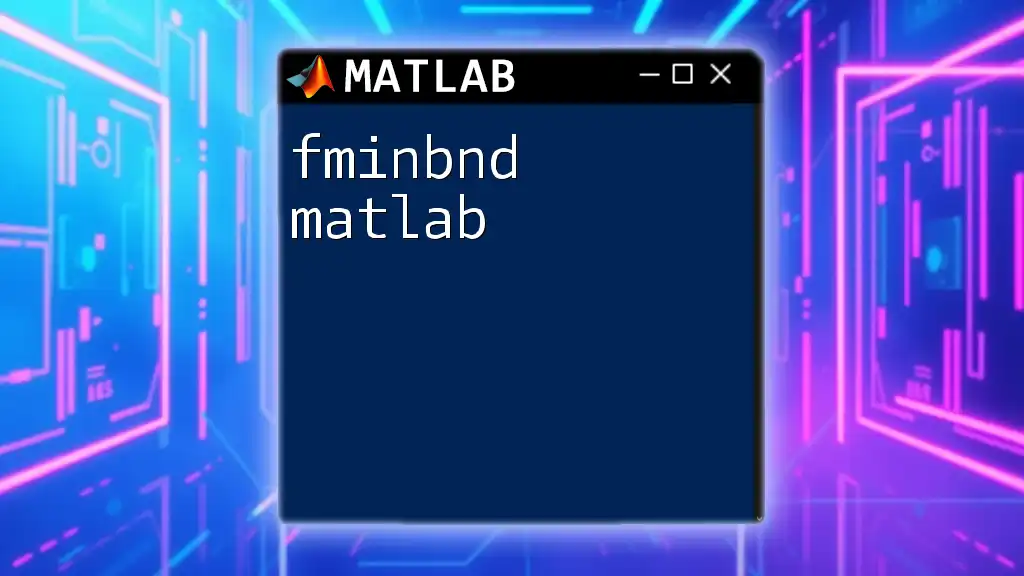
Syntax of fminunc
Basic Syntax
The basic syntax for using `fminunc` in MATLAB is as follows:
[x,fval] = fminunc(fun,x0)
Where:
- `fun` is a handle to the objective function you wish to minimize.
- `x0` is the initial guess for the variables that will be optimized.
- `x` will contain the optimized variable values.
- `fval` will give the value of the objective function at the minimum.
Optional Parameters
`fminunc` allows for additional customization through optional parameters. You can create an options structure using the `optimoptions` function. The call to `fminunc` will then include this options structure.
Example:
options = optimoptions('fminunc','Algorithm','quasi-newton');
[x,fval,exitflag] = fminunc(fun,x0,options)
Commonly Used Options
- `Display`: Controls how much output the function provides during execution. You can set it to 'iter' for iterative output or 'final' for just the final results.
- `MaxIter`: This option allows you to specify the maximum number of iterations the optimization process should run.
- `TolFun`: Tolerance on the function value; once the function value is below this threshold, the optimization stops.
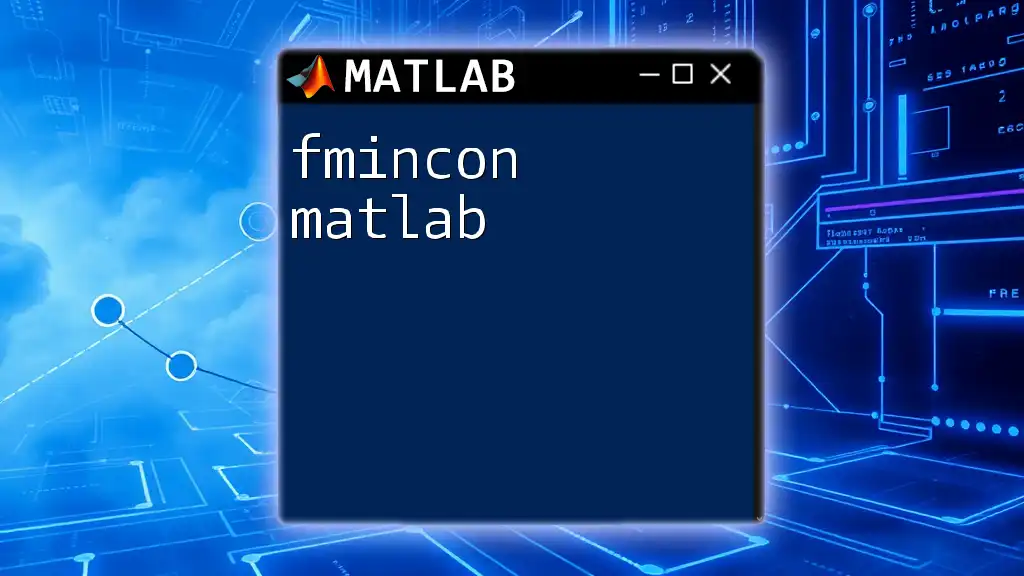
Setting Up Your Objective Function
Creating a Function Handle
A function handle in MATLAB is a MATLAB data type that represents a function. You can create an anonymous function directly in your script or use a separate function file.
Example of creating an anonymous function:
fun = @(x) (x(1)-2)^2 + (x(2)-3)^2; % Example function
Example Objective Functions
To illustrate various types of objective functions:
- Quadratic Functions: A simple quadratic function demonstrates how `fminunc` works.
- Non-linear Functions: Functions like `sin(x)` or `exp(x)`, which oscillate or grow rapidly, showcase the flexibility of `fminunc`.
- Multi-variable Functions: Functions with several variables can also be minimized, facilitating more complex optimization needs.
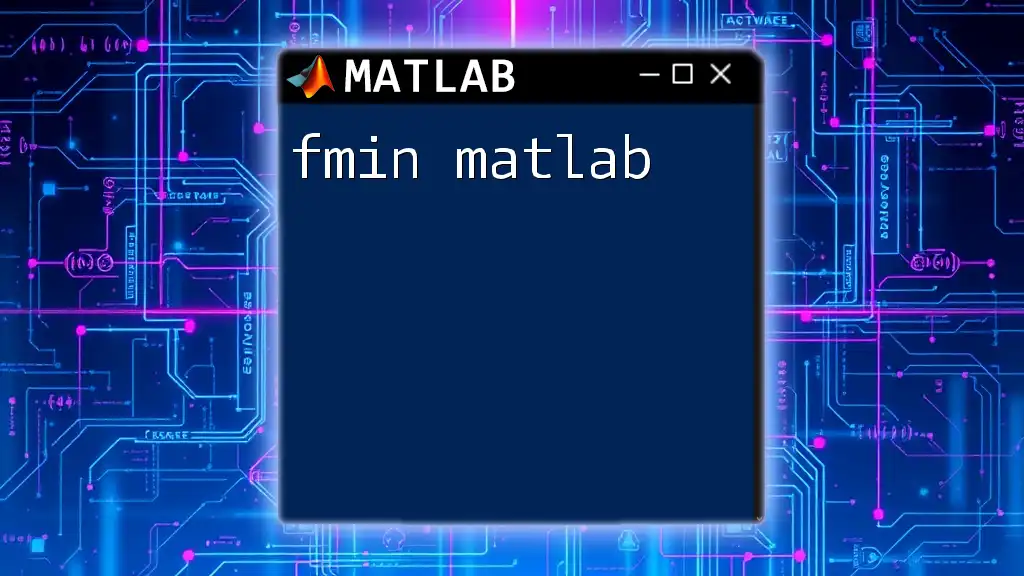
Running fminunc: A Step-by-Step Example
Problem Statement
For instance, you want to minimize the function: \( f(x, y) = (x-2)^2 + (y-3)^2 \)
Step 1: Define the Objective Function
You can define an objective function either as an anonymous function or in a separate file. Below is the implementation:
function f = myObjectiveFunction(x)
f = (x(1)-2)^2 + (x(2)-3)^2;
end
Step 2: Set Initial Guess
Choosing an appropriate initial guess can dramatically affect convergence. For this example:
x0 = [0,0]; % Initial guess
Step 3: Call fminunc
Now, you can execute the optimization routine:
[x,fval] = fminunc(@myObjectiveFunction,x0);
Step 4: Interpret the Results
The output will show:
- `x`: the optimal values of the variables, indicating where the function reaches its minimum.
- `fval`: the minimum value of the objective function at those optimal variables.
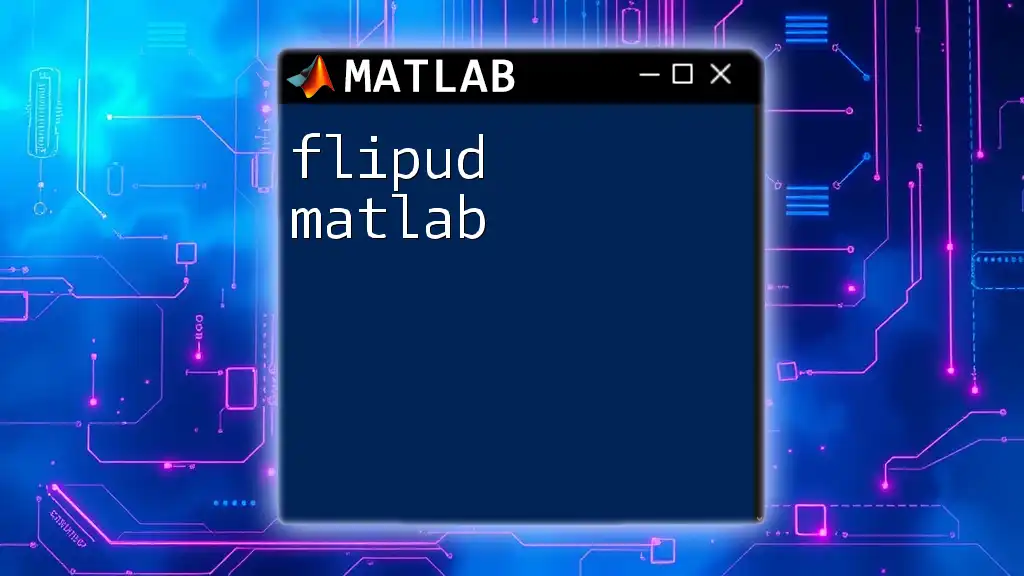
Troubleshooting Common Issues
Divergence or No Convergence
If `fminunc` does not converge or diverges, it may indicate issues with the function's gradients or that the function itself is ill-conditioned. A common solution would be reviewing the smoothness of the objective function.
Ill-defined Objective Functions
An ill-defined objective function might lead to unexpected behavior in optimization. Ensure that your function is well-constructed, and if possible, provide gradient information using the `GradObj` option.
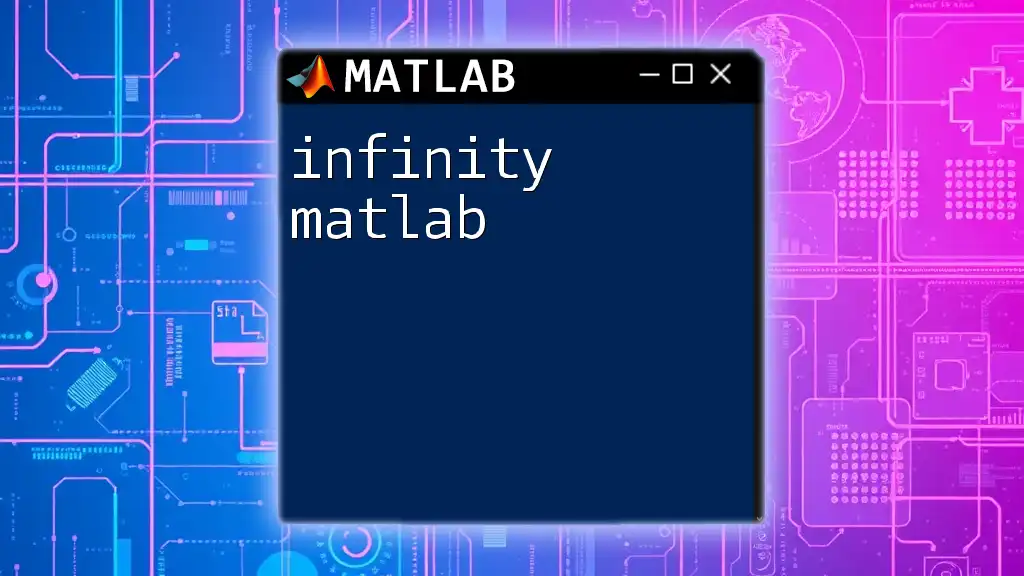
Practical Applications of fminunc
Real-World Use Cases
- Engineering Design Optimization: Engineers can use `fminunc` for optimizing parameters in design processes, resulting in more efficient products.
- Machine Learning Parameter Tuning: In machine learning, `fminunc` can optimize model hyperparameters to improve predictive accuracy.
- Financial Modeling: Financial analysts use it to minimize risk or cost functions when modeling portfolios.
Advanced Use Case Example
An advanced example could involve optimizing a complex logistic regression model, where you minimize the logistic loss function using `fminunc`. This showcases the versatility of the function across varying domains.
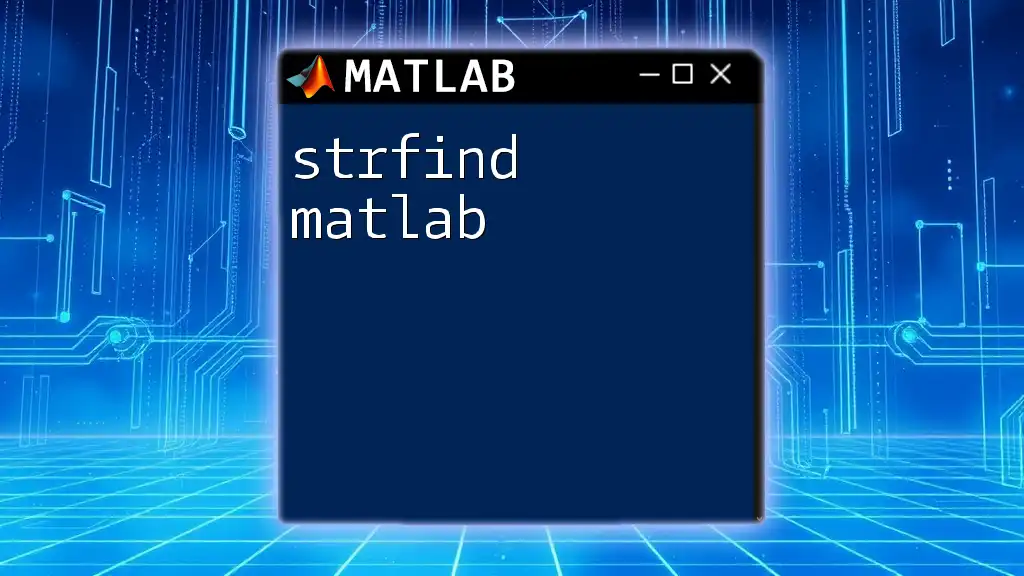
Conclusion
By leveraging the capabilities of `fminunc`, individuals can tackle a diverse range of optimization problems. Whether you are an engineer needing to minimize design parameters or a data scientist tuning a model, fminunc in MATLAB is a powerful tool. Practice with various functions, and don't hesitate to experiment with `optimoptions` to fine-tune your optimization tasks!
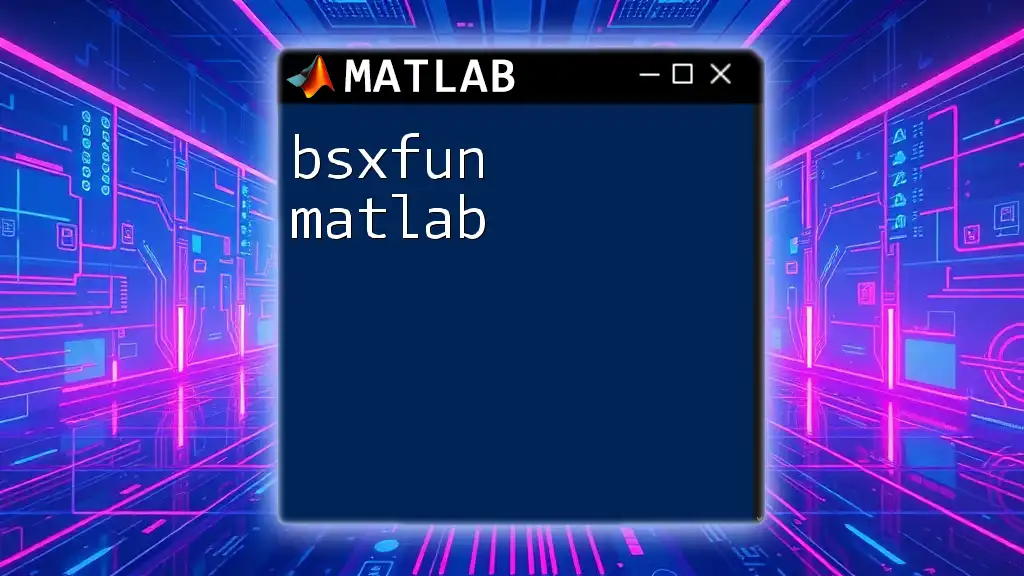
Additional Resources
Further Reading on Optimization in MATLAB
Explore the official MATLAB documentation for comprehensive details and further cases surrounding fminunc and optimization functions.
Community and Support
Engage with the MATLAB community through forums and online tutorials to enhance your understanding and resolve any queries regarding optimization techniques in MATLAB.