The `rms` function in MATLAB calculates the root mean square of a set of values, providing a measure of the magnitude of a varying quantity.
Here's a code snippet demonstrating its use:
data = [3, 4, 5, 6]; % Example data
result = rms(data); % Calculate the root mean square
disp(result); % Display the result
Understanding RMS
What is RMS?
The Root Mean Square (RMS) is a statistical measure used to calculate the magnitude of a varying quantity. Mathematically, it is defined as the square root of the average of the squares of a set of values. The formula for one-dimensional data can be represented as follows:
\[ RMS = \sqrt{\frac{1}{N} \sum_{i=1}^{N} x^2_i} \]
where \(N\) is the number of values in the dataset. The RMS calculation is crucial in many fields such as engineering and statistics, as it provides an effective way to assess the average power or magnitude of a signal.
Applications of RMS
The use of RMS spans across various domains including:
- Industrial Applications: In robotics and automation, RMS is used to analyze the motion of components and ensure smooth operations.
- Electrical Engineering: RMS is critical for analyzing AC signals. It allows engineers to determine the effective voltage/current of these signals, enabling accurate power calculations.
- Sound Engineering: In audio processing, RMS helps in manipulating the dynamic range of sound waves, ensuring consistent audio quality across different playback systems.
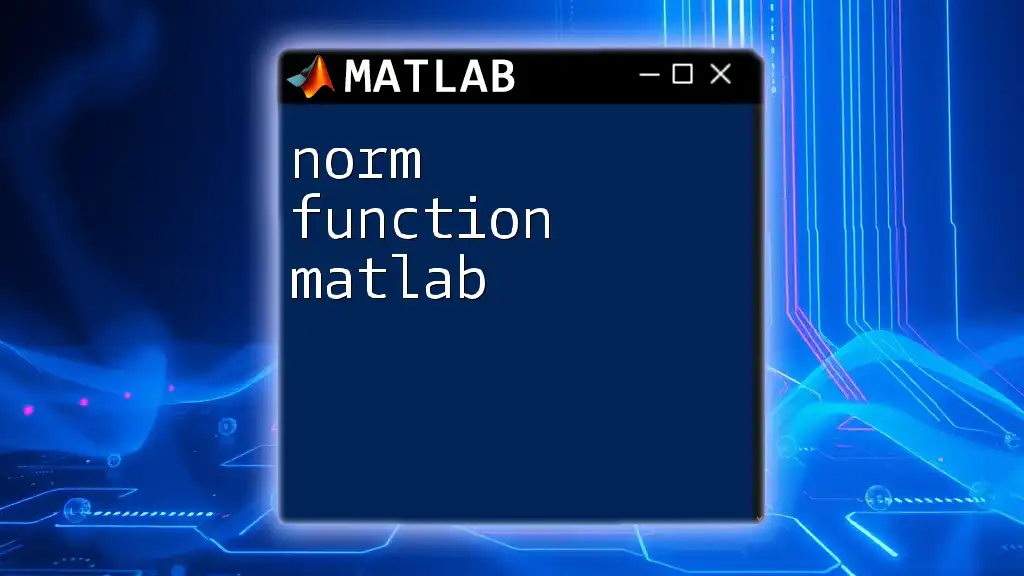
Overview of MATLAB
Why Use MATLAB for RMS?
MATLAB is a powerful programming environment widely used for mathematical computations and data analysis. It offers a plethora of built-in functions that facilitate complex calculations with simplicity and efficiency. Utilizing MATLAB for RMS computations allows users to benefit from its optimized performance and connectivity with other MATLAB toolboxes, enhancing overall data analysis capabilities.
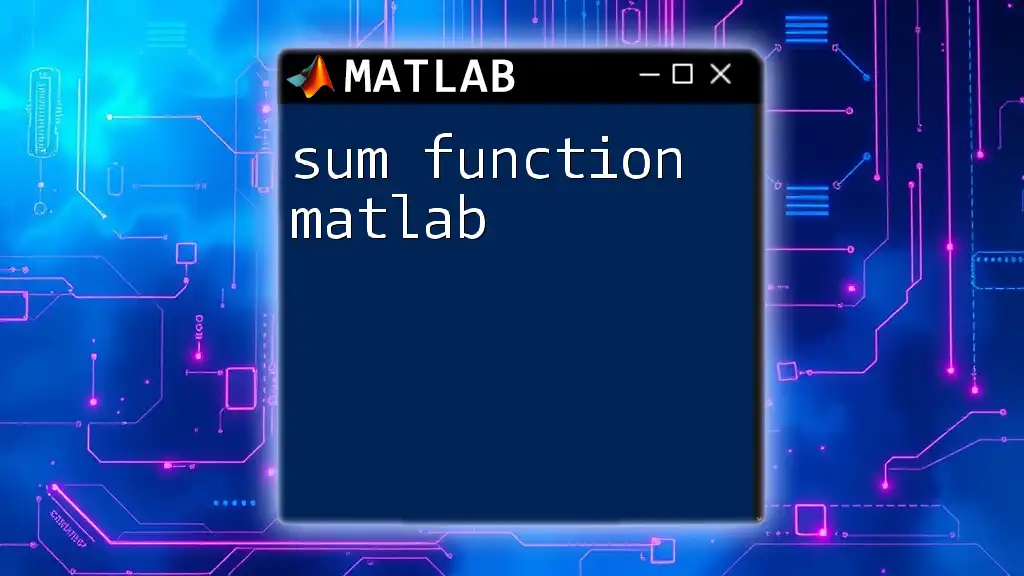
The RMS Function in MATLAB
Syntax of the rms Function
The rms function in MATLAB is utilized to compute the root mean square of an array. The general syntax of the function is:
rms(X)
Here, `X` can be a vector, matrix, or multidimensional array, representing the dataset for which you want to compute the RMS.
Input Parameters
The `rms` function can handle various types of input:
- Vectors: A one-dimensional array of values for which RMS is calculated directly.
- Matrices: In the case of matrices, RMS can be computed for either rows or columns based on the dimension specified.
- Multidimensional Arrays: The function can also handle multi-dimensional arrays, performing the calculation along specified dimensions.
Basic Example of the rms Function
Here’s a simple example demonstrating the use of the rms function:
data = [1, 2, 3, 4, 5];
result = rms(data);
disp(result);
In this example, we compute the RMS of the vector containing integers from 1 to 5. The output is \(3.16\), which indicates the effective magnitude of the dataset.
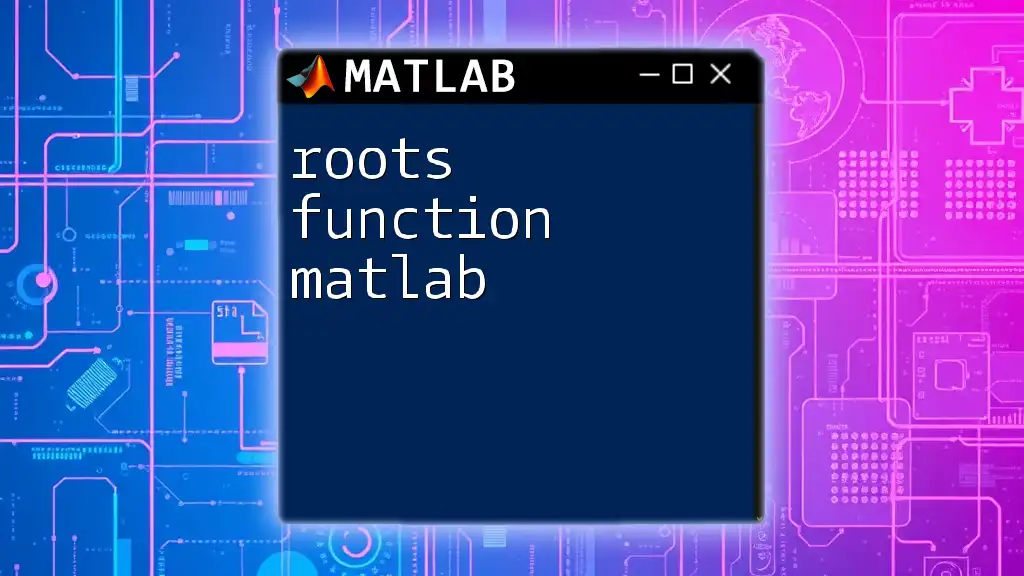
Detailed Example Applications
Example 1: RMS in Signal Processing
RMS is particularly important in signal processing, where it helps analyze audio signals. Let’s say we want to evaluate a sine wave signal. The MATLAB code for this scenario is as follows:
t = 0:0.001:1; % time vector
signal = sin(2 * pi * 10 * t); % generate a 10 Hz sine wave
rms_value = rms(signal);
fprintf('RMS value of the signal: %.2f\n', rms_value);
In this example, we generate a sine wave signal at 10 Hz and compute its RMS value, which provides insight into the signal's magnitude. The RMS value will help determine how loud the signal is perceived in audio applications.
Example 2: RMS of a Matrix
When dealing with datasets represented as matrices, it's often necessary to calculate the RMS value for each column or row. Here’s an example demonstrating how to compute the RMS for each column of a matrix:
data_matrix = [1 2; 3 4; 5 6];
rms_columns = rms(data_matrix, 1); % Calculate RMS along the rows
disp(rms_columns);
This code calculates the RMS for each column in the `data_matrix`. The output displays a row vector containing the RMS values of each column, showcasing how the data behaves across different dimensions.
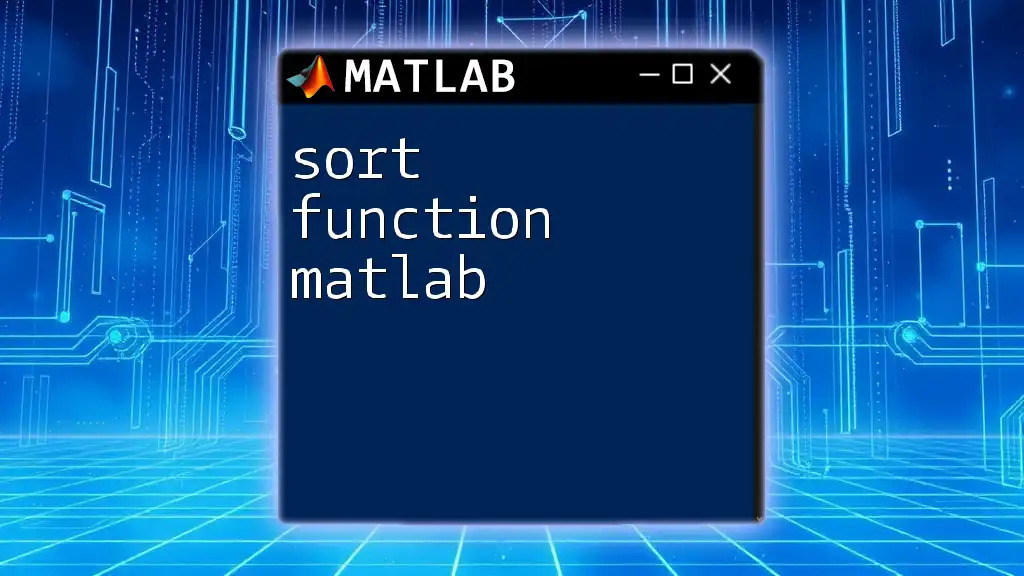
Advanced Usage of RMS Function
Specifying Dimensions in the rms Function
The RMS function allows users to specify which dimension of a matrix or multi-dimensional array to operate along using the syntax:
rms(X, dim)
For instance, consider the following example:
data = rand(3, 3); % generate a 3x3 random matrix
rms_rows = rms(data, 2); % RMS along rows
rms_cols = rms(data, 1); % RMS along columns
Here, we generate a 3x3 random matrix and compute the RMS for rows and columns separately. Understanding how to manipulate dimensions is crucial for effective data analysis and allows tailored computations for specific datasets.
Handling Complex Numbers
MATLAB's RMS function can also handle complex numbers effectively. Here’s how you can compute the RMS for a complex dataset:
complex_data = [1 + 2i, 2 + 3i; 3 + 4i, 4 + 5i];
rms_complex = rms(complex_data);
disp(rms_complex);
In this code, we calculate the RMS value of a complex number matrix. The RMS value gives meaningful insights into both the magnitude and the phase characteristics of the signals represented by the complex numbers.
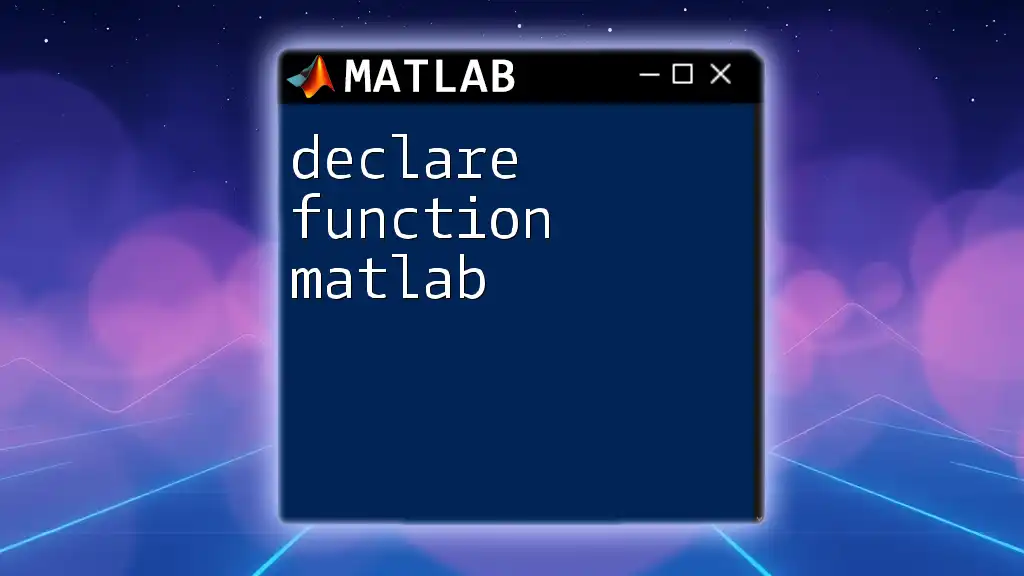
Common Issues and Troubleshooting
Common Errors with the rms Function
Users may encounter several common errors when using the RMS function, such as:
- Dimension Mismatch: Ensure that the dimensions specified for matrix inputs align correctly, or MATLAB will throw an error.
- Input Type Errors: The function expects numeric arrays. Passing in non-numeric types will result in errors.
To avoid these issues, always validate your dataset and ensure the inputs meet the function's requirements.
Performance Tips
When working with large datasets, consider the following tips to enhance performance:
- Utilize vectorization rather than loops when calculating RMS for large arrays.
- Minimize the use of temporary variables within loops to save memory and computational power.
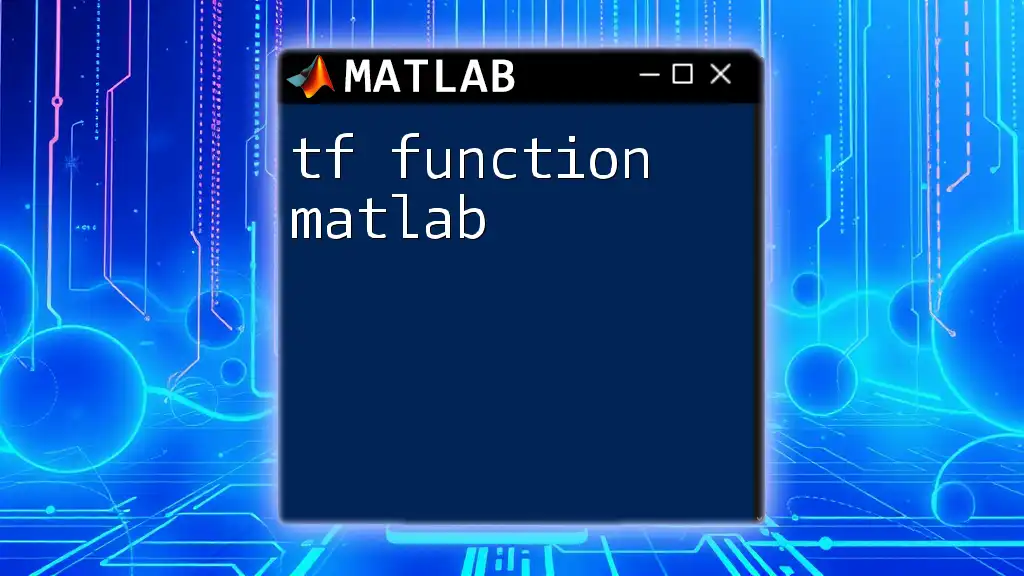
Conclusion
The rms function in MATLAB is a powerful tool for calculating the root mean square of datasets across various applications. By understanding its syntax, functionality, and application contexts, you can leverage this function to gain deeper insights into your data. Don't hesitate to experiment with your datasets and explore the vast capabilities of MATLAB to solve complex problems efficiently.
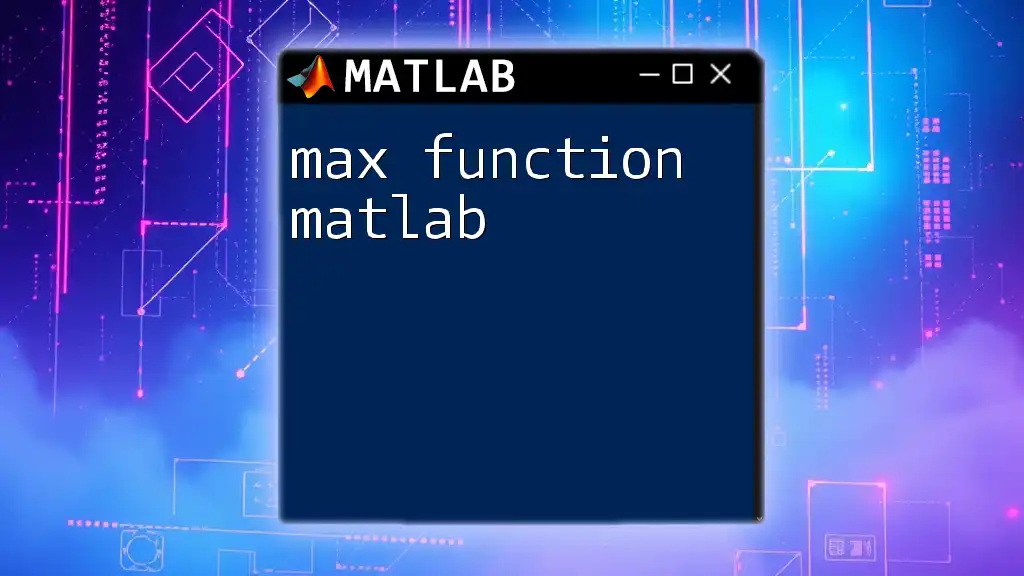
Additional Resources
Recommended Reading
For further exploration, refer to the official MATLAB documentation related to the rms function and its applications.
Community and Support
Engage with MATLAB programming communities and forums to share insights, seek assistance, or explore advanced topics. If you have questions or seek personalized guidance, feel free to reach out for support.