Singular Value Decomposition (SVD) in MATLAB is a matrix factorization technique that expresses a matrix as the product of three matrices, which can be used for tasks such as dimensionality reduction and data compression.
Here’s a simple code snippet demonstrating SVD decomposition in MATLAB:
% Example of SVD decomposition in MATLAB
A = [1, 2; 3, 4; 5, 6]; % Example matrix
[U, S, V] = svd(A); % Perform SVD
Understanding Singular Value Decomposition (SVD)
What is SVD?
Singular Value Decomposition (SVD) is a powerful mathematical technique in linear algebra that breaks down a matrix into three component matrices. Formally, it expresses any given matrix \( A \) in the form:
\[ A = U \Sigma V^T \]
This representation is fundamental in numerous data processing applications, particularly in fields like statistics, signal processing, and machine learning.
Components of SVD
U Matrix
U is an orthogonal matrix that contains the left singular vectors of the matrix \( A \). These vectors correspond to the columns of the original matrix and are vital in defining the range of \( A \). Each column in \( U \) represents an orthonormal basis for the column space of \( A \), and its dimensions will be \( m \times m \), where \( m \) is the number of rows in \( A \).
Σ (Sigma) Matrix
The Σ matrix is a diagonal matrix containing the singular values of \( A \). These singular values are always non-negative and are arranged in descending order, which gives insight into the inherent structure of the matrix and its rank. The dimensions of Σ will be \( m \times n \), where \( n \) is the number of columns in \( A \).
V Matrix
The V matrix, similar to U, is also an orthogonal matrix but contains the right singular vectors of \( A \). The columns of \( V \) form an orthonormal basis for the row space of \( A \) and will have dimensions of \( n \times n \).

Practical Application of SVD in MATLAB
SVD Command in MATLAB
To utilize SVD in MATLAB, the `svd` function is employed, which is straightforward. The basic syntax for computing the SVD of a matrix \( A \) is as follows:
[U, S, V] = svd(A);
This command decomposes the matrix \( A \) into matrices \( U, \Sigma, \) and \( V \), which can then be used for various analytical purposes.
Example: Performing SVD in MATLAB
Let’s dive into an example to see how SVD can be applied in MATLAB effectively.
-
Creating a Sample Matrix: First, define a sample matrix \( A \):
A = [1, 2; 3, 4; 5, 6];
-
Applying SVD: Now, apply the SVD command:
[U, S, V] = svd(A);
-
Understanding the Outputs: After executing the command, you'll obtain three matrices:
- U: Represents the left singular vectors.
- S: The diagonal matrix containing singular values.
- V: Represents the right singular vectors.
With this breakdown, each part of the decomposition serves distinct roles in analyzing the original matrix \( A \).
Interpreting the Results
Understanding U, Σ, and V Matrices
Upon performing SVD, it's crucial to interpret the results effectively.
-
U Matrix Explanation: The \( U \) matrix highlights the orthonormal basis corresponding to the column space of \( A \). Its structure provides insight into how the original data is spanned in a higher-dimensional space.
-
Σ Matrix Explanation: The diagonal values in the \( S \) matrix indicate the magnitude of the importance each singular vector has in capturing the variance of the data. The higher the singular value, the more significant that particular factor is.
-
V Matrix Explanation: The \( V \) matrix serves as a reflection of the original data across its row space, helping understand relationships and variations between different row vectors in matrix \( A \).

Applications of SVD in MATLAB
Dimensionality Reduction
One of the major applications of SVD in MATLAB is Principal Component Analysis (PCA), where we can reduce the dimensionality of large datasets while preserving as much variance as possible. The relationship between PCA and SVD lies in projecting high-dimensional data down to a lower-dimensional space using the largest singular values.
To perform PCA, the steps are as follows:
-
Calculate SVD: As before, we’ll utilize the SVD:
[U, S, V] = svd(A);
-
Transform the Data: To achieve dimensionality reduction, multiply the \( U \) matrix by the reduced singular values:
K = 2; % Reduce the dimensionality to 2 A_reduced = U(:, 1:K) * S(1:K, 1:K);
This method compresses the data while retaining significant information.
Image Compression Techniques
SVD can significantly enhance image processing, particularly in image compression. By utilizing SVD, an image can be represented in a smaller number of singular values, resulting in effective storage and transmission.
-
Image Representation: For instance, if you read an image matrix in MATLAB:
img = imread('example.jpg'); img_matrix = double(img);
-
Apply SVD: You can decompose this image matrix using SVD:
[U, S, V] = svd(img_matrix);
-
Reconstructing the Image: You can then reconstruct the image with reduced singular values, which aids in compression.
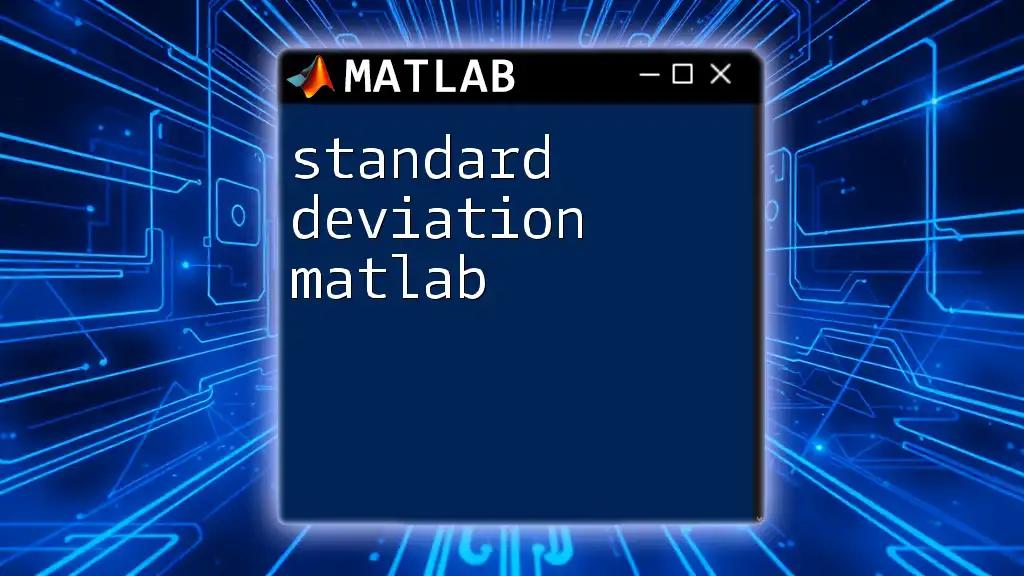
Advanced SVD Techniques
Truncated SVD
Truncated SVD refers to the process of keeping only the top \( K \) singular values while discarding the rest. This is particularly useful for approximations in datasets where data fidelity is not a critical concern.
-
Implementing Truncated SVD:
K = 2; % Number of singular values to retain U_k = U(:, 1:K); S_k = S(1:K, 1:K); A_approx = U_k * S_k * V(:, 1:K)';
This approximation helps in reducing computational load and is often used in generalizing data in machine learning algorithms.
Sparse SVD
When dealing with large datasets, Sparse SVD can be advantageous, allowing for efficiently storing and processing matrices that are primarily composed of zeros. MATLAB provides the `svds` function for this purpose.
-
Using the `svds` Function:
[U, S, V] = svds(A, K);
This command computes the top \( K \) singular values and corresponding singular vectors without computing the full SVD, saving computation time and memory.

Conclusion
Singular Value Decomposition (SVD) is a foundational technique in data analysis that provides critical insights across a multitude of applications, from data compression to dimensionality reduction. By mastering SVD decomposition in MATLAB, you can leverage its powerful capabilities to handle complex datasets. Engage with the concepts, practice with your custom datasets, and discover more applications of SVD to enhance your data science skills and analytical capabilities.
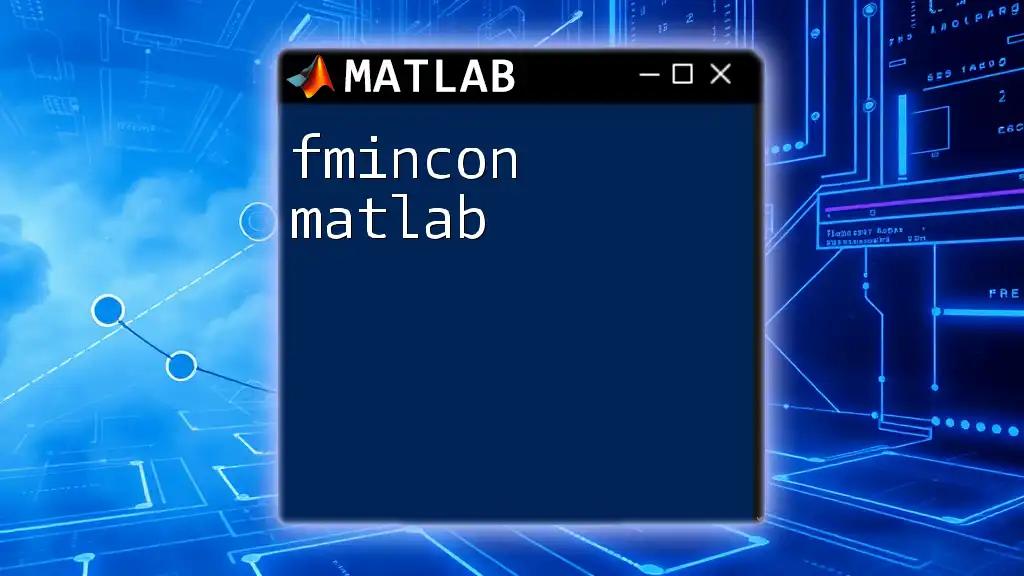
Additional Resources
For further exploration, refer to MATLAB’s official documentation on SVD and consider diving into academic materials focusing on applications of SVD across different domains. Understanding SVD deeply will not only help in MATLAB but also across various fields employing data analysis techniques.