The `ksdensity` function in MATLAB estimates the probability density function of a set of data using kernel smoothing, allowing you to visualize the distribution of the data points.
data = randn(100,1); % Generate random data
[f,xi] = ksdensity(data); % Estimate the probability density function
plot(xi,f); % Plot the density estimate
title('Kernel Density Estimate');
xlabel('Data Values');
ylabel('Density');
What is ksdensity?
Kernel Density Estimation (KDE) is a non-parametric way to estimate the probability density function of a random variable. Unlike traditional histogram methods that can be heavily influenced by the choice of bins, KDE provides a smooth curve. This smoothness is crucial for visualizing the underlying distribution without assuming any specific statistical model.
KDE finds applications in various fields, including finance for risk assessment, biology for analyzing animal movement patterns, and environmental science for weather prediction. The MATLAB function `ksdensity` allows users to leverage this powerful statistical tool with just a few lines of code.
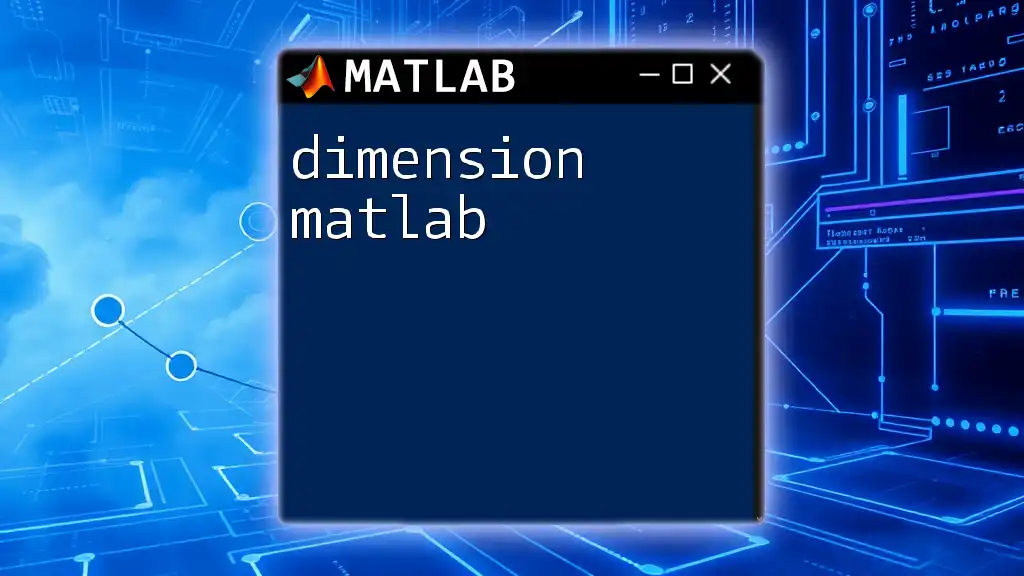
Getting Started with ksdensity in MATLAB
Before diving into the specifics of using the `ksdensity` command, it is essential to ensure you have MATLAB installed on your computer. For new users, MATLAB offers straightforward installation guidelines on their official website.
Once you have MATLAB set up, the next step is to load your data. Depending on your data's format, you can use functions like `readtable` or `load`. For illustration, here’s how to import a dataset containing random numbers that follow a normal distribution:
data = randn(1000, 1); % Generating random normally distributed data
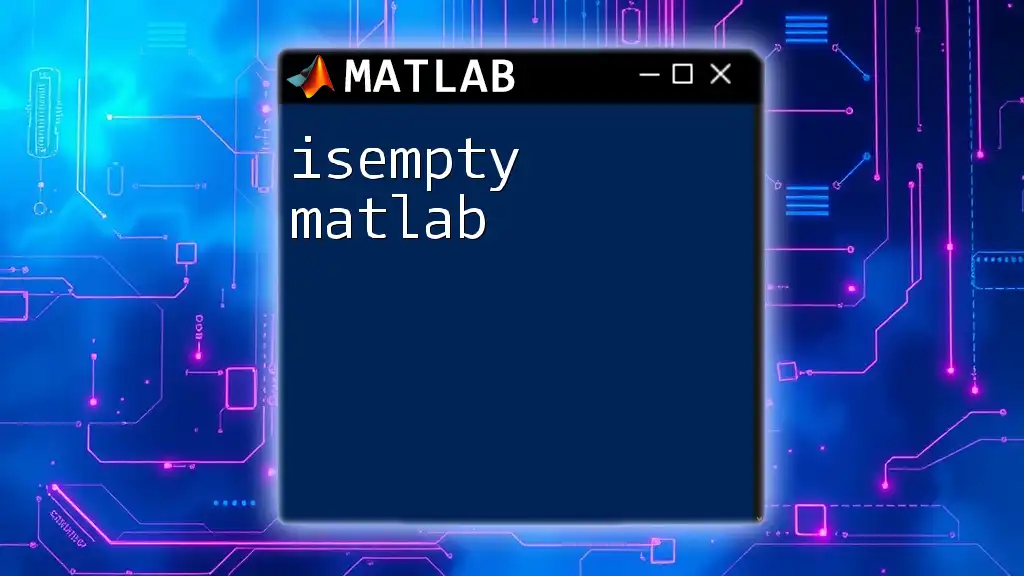
Basic Syntax of ksdensity
The basic syntax for the `ksdensity` function is straightforward:
[f, xi] = ksdensity(data)
- data is the input array containing the points you wish to estimate the density for.
- f will contain the density estimates.
- xi represents the points at which the density is estimated.
To see this in action, consider the following example that generates a kernel density estimate from normally distributed data:
data = randn(1, 1000); % Generate random data
[f, xi] = ksdensity(data); % Estimate the density
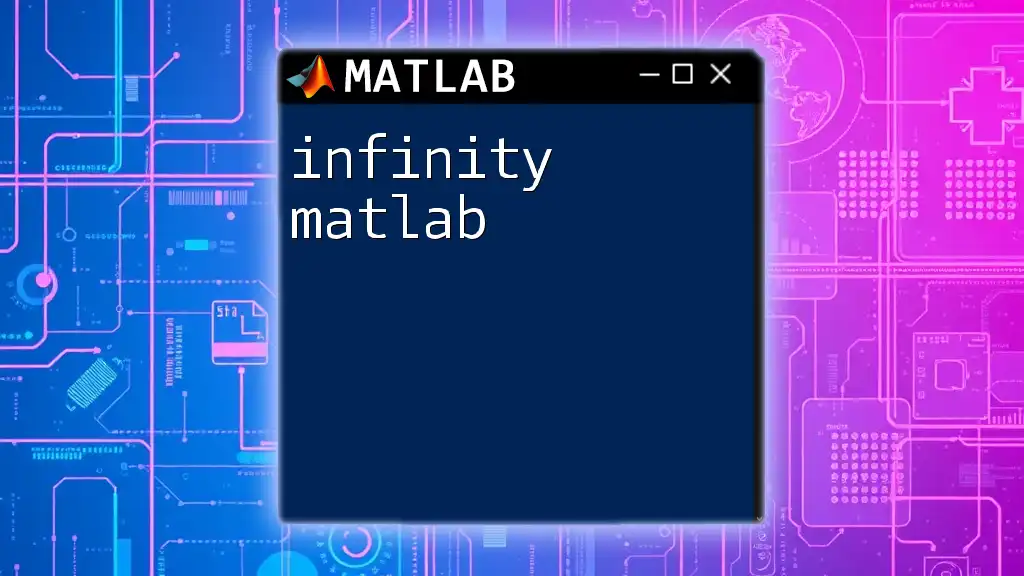
Visualizing Data Using ksdensity
Plotting KDE
Creating a basic plot for your kernel density estimate is simple and efficient in MATLAB. The clinical visualization of density functions helps identify data trends and outliers.
Here’s how you can create a basic KDE plot:
figure;
plot(xi, f);
title('Kernel Density Estimate');
xlabel('Data values');
ylabel('Density');
Enhancing the Plot
You can customize the appearance of your KDE plot by adjusting colors, line styles, and other figure properties. Good visualization practices improve readability and aesthetics.
Below is an enhanced version of the earlier plot:
figure;
plot(xi, f, 'r--', 'LineWidth', 2); % Red dashed line
grid on; % Adding grid lines for better visibility
title('Enhanced Kernel Density Estimate', 'FontWeight', 'bold');
xlabel('Data values', 'FontSize', 12);
ylabel('Density', 'FontSize', 12);
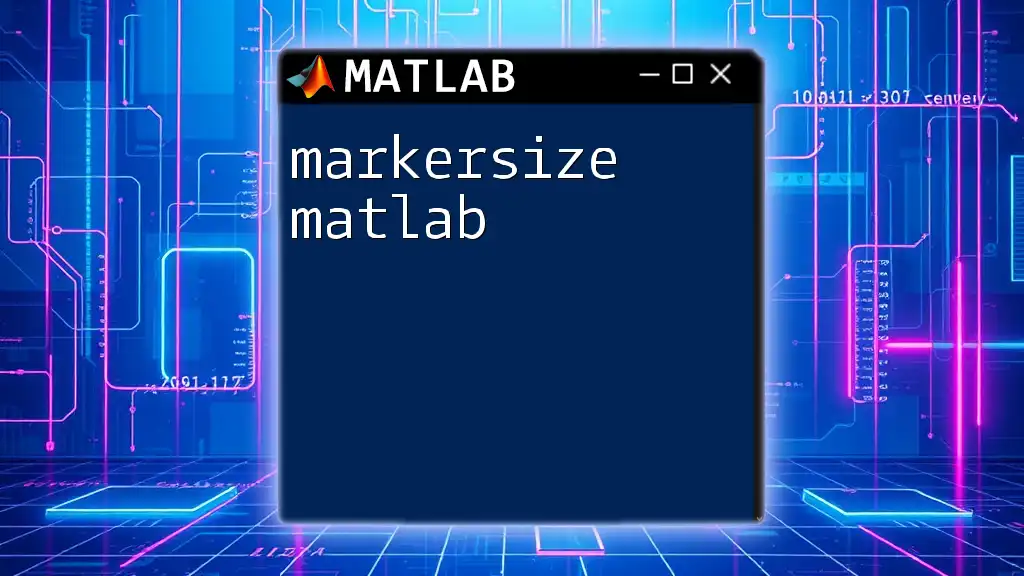
Advanced Features of ksdensity
Adjusting Bandwidth
The bandwidth parameter is pivotal in kernel density estimation. It determines the width of the kernel, influencing the amount of smoothing applied to the data. A smaller bandwidth may capture more detail but can introduce noise, while a larger bandwidth smooths out the data but can obscure important features.
You can specify a custom bandwidth using the 'Bandwidth' parameter in `ksdensity`:
[f, xi] = ksdensity(data, 'Bandwidth', 0.5); % Adjusting bandwidth to 0.5
Experimenting with various bandwidth values can help you find the optimal smoothness for your specific dataset.
Handling Multivariate Data
KDE is not limited to univariate data; it can also accommodate multidimensional datasets. This feature is particularly useful for examining relationships between variables.
To perform a kernel density estimation with bivariate data (two variables), consider the following example, which uses normally distributed data:
data = [randn(100,2); randn(100,2)+5]; % Combine two distributions
[f, xi] = ksdensity(data); % Estimate the density
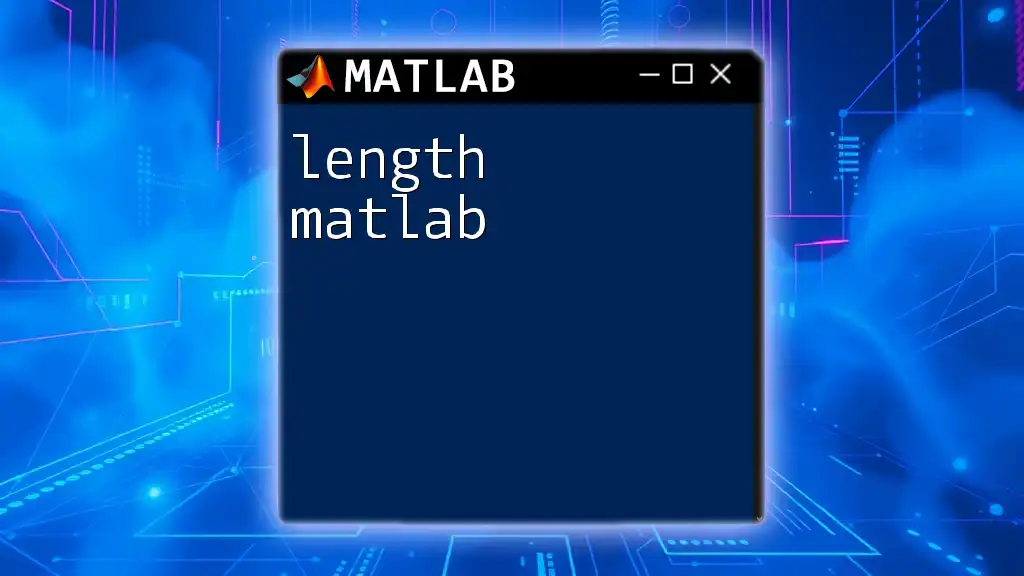
Applications of ksdensity
Case Studies
KDE can be a valuable asset in real-world scenarios. Here are two compelling examples:
-
Analyzing Income Distribution: By applying `ksdensity` to income data, researchers can identify income inequality trends effectively, providing insights to policymakers.
-
Environmental Data Smoothing: In meteorology, KDE can interpret rainfall patterns more clearly. By examining historical rainfall data through `ksdensity`, meteorologists can assess variations and probabilities of extreme weather events.
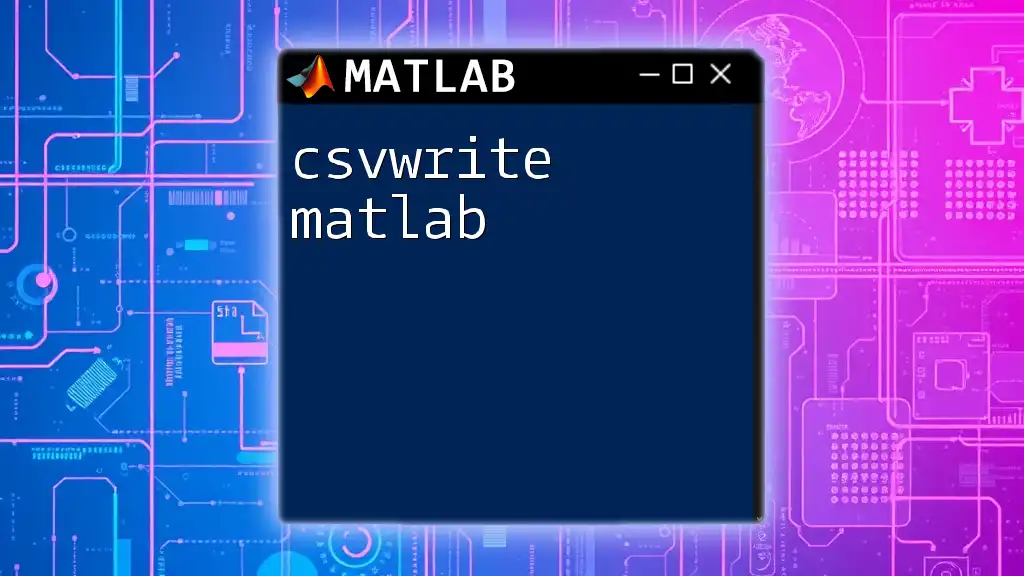
Troubleshooting Common Issues
When utilizing `ksdensity`, it's important to be aware of some common pitfalls:
- Mismatched Data Dimensions: Ensure that your input dataset does not have mismatched sizes for dimensionality specifications.
- Appropriate Parameter Selection: Selecting a suitable bandwidth is crucial. If the plot appears too jagged or overly smooth, revisit the bandwidth parameter.
New users may encounter errors or warnings. Understanding these messages and experimenting with the parameters will enrich your learning experience and enhance your familiarity with MATLAB functions.
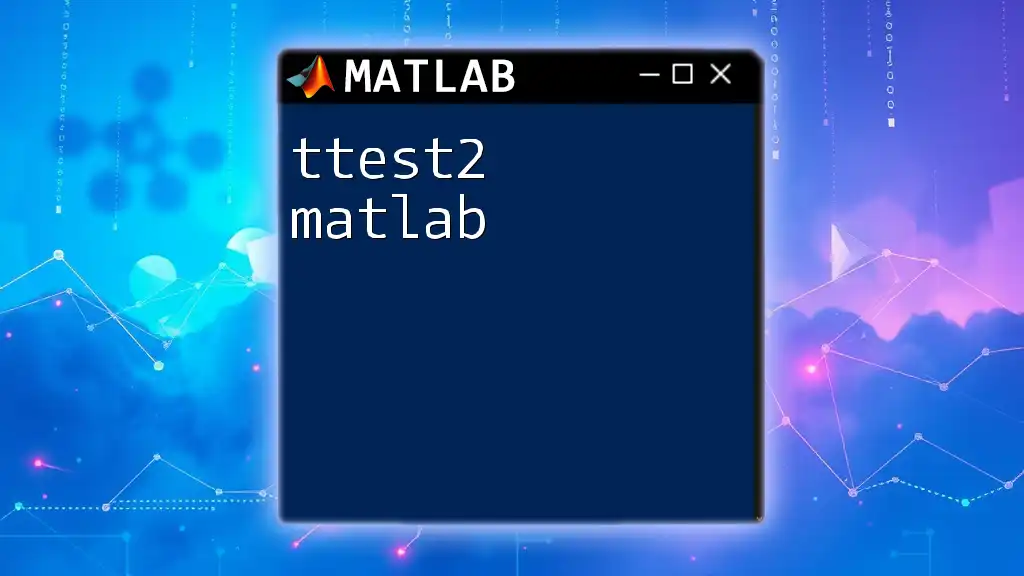
Conclusion
The `ksdensity` function in MATLAB is an invaluable tool for smooth and effective kernel density estimation. Through detailed examples and explanations, you can now leverage this function to enhance your data visualization skills and statistical analysis capabilities.
By exploring and experimenting with different datasets and parameters, you can uncover deeper insights and improve your proficiency in statistical analysis using MATLAB.
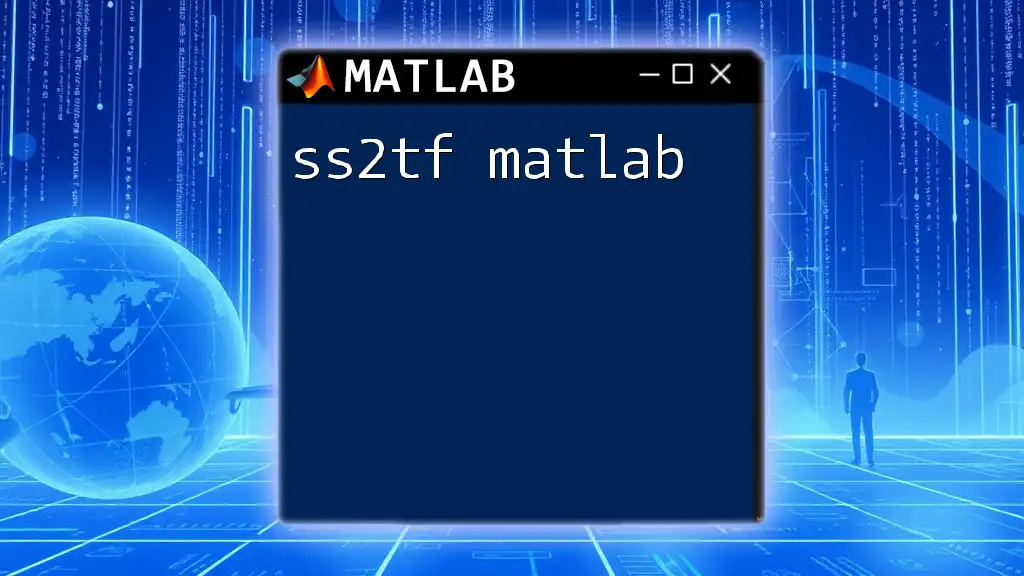
Additional Resources
For further elucidation on advanced topics related to `ksdensity`, you can consult MATLAB’s official documentation and various textbooks. Engaging with communities through forums and social media platforms dedicated to MATLAB can also immensely support your learning journey.
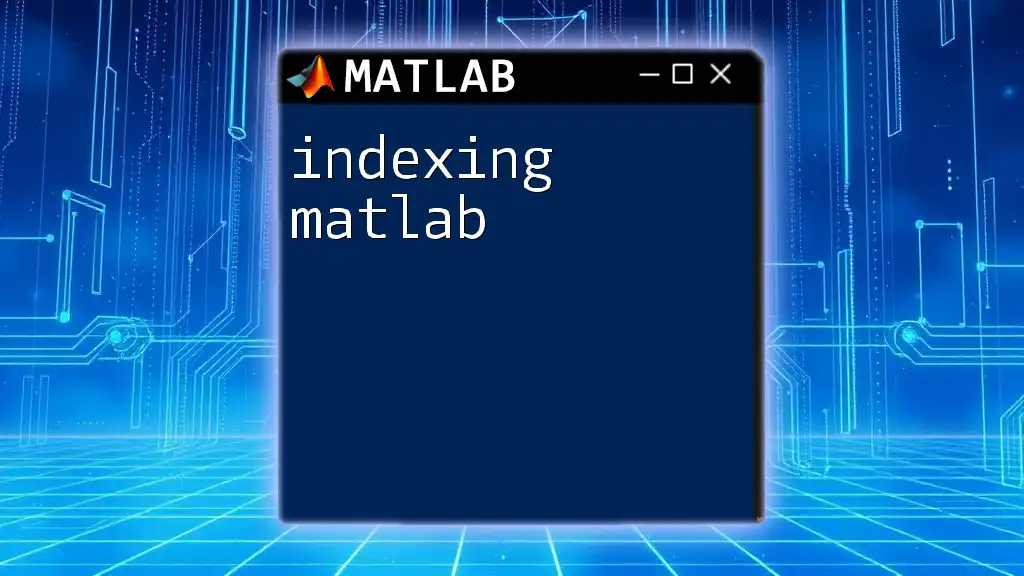
Call to Action
We invite you to explore our courses and workshops on MATLAB to enhance your understanding of data analysis techniques. Free resources for beginner MATLAB users are also available, guiding you through your journey into the world of programming and statistical analysis.