LU decomposition in MATLAB is a method for decomposing a matrix into a lower triangular matrix (L) and an upper triangular matrix (U), which can be used to solve linear equations efficiently.
A = [4, 3; 6, 3]; % Example matrix
[L, U] = lu(A); % Perform LU decomposition
What is LU Decomposition?
LU decomposition refers to the factorization of a matrix \( A \) into two components: a lower triangular matrix \( L \) and an upper triangular matrix \( U \). This means any square matrix \( A \) can be expressed as:
\[ A = LU \]
Understanding LU decomposition is fundamental in linear algebra because it simplifies the process of solving systems of linear equations, inverting matrices, and performing other computational tasks with greater efficiency.
Importance in Numerical Analysis
The elegance of LU decomposition lies in its ability to reduce computational complexity when solving linear systems. Instead of directly approaching the system \( Ax = b \), we can transform it into two simpler equations:
- Solve for \( y \) in \( Ly = b \).
- Solve for \( x \) in \( Ux = y \).
This two-step process greatly enhances numerical stability and efficiency, particularly for large systems.
Conditions for LU Decomposition
Not all matrices are suitable for LU decomposition. To achieve this factorization, the matrix \( A \) must be square and preferably non-singular (having a non-zero determinant). In instances where these conditions aren't met, pivoting may be necessary to reorder the rows and ensure stability while avoiding division by zero.
Partial pivoting is often employed, where we rearrange the rows of \( A \) to place the largest absolute value in the leading position. This ensures greater accuracy in numerical computations.
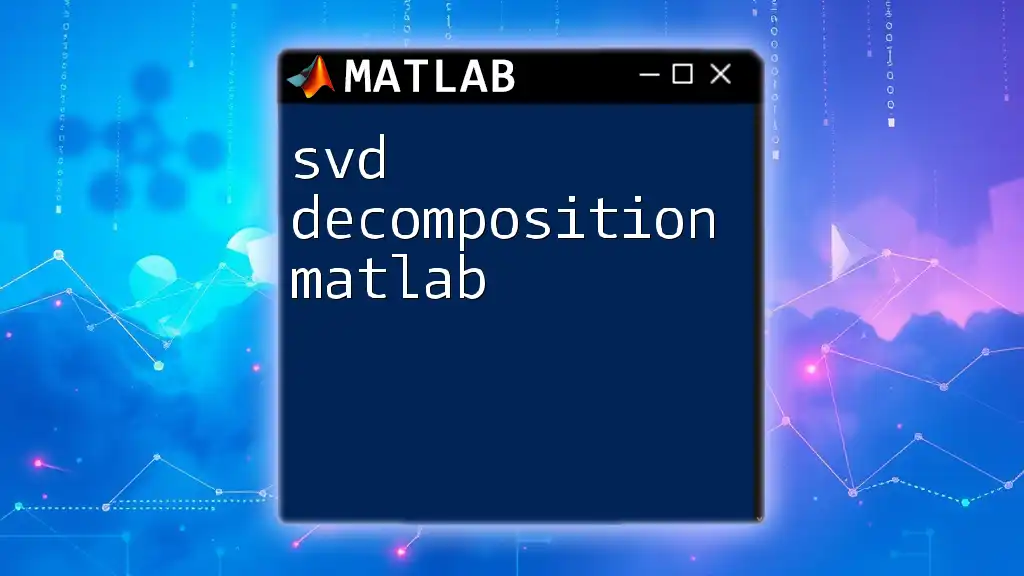
Performing LU Decomposition in MATLAB
MATLAB's Built-in Functions
MATLAB provides a robust function called `lu` to facilitate LU decomposition. Here's a quick overview of the syntax:
[L, U] = lu(A)
- L is the lower triangular matrix.
- U is the upper triangular matrix.
- Matrix \( A \) can include an optional third output for the permutation matrix \( P \) if reordering was needed.
Code Example: Basic LU Decomposition
Here is a simple example demonstrating the use of the `lu` function:
A = [4, 3; 6, 3]; % Sample square matrix
[L, U] = lu(A); % Perform LU decomposition
disp(L); % Displaying L
disp(U); % Displaying U
In this code, we define a \( 2 \times 2 \) matrix \( A \) and use the `lu` function to obtain \( L \) and \( U \). Utilizing the `disp` function, we can visualize the resulting matrices.
Step-by-Step Example
Example Matrix
Consider the matrix:
\[ A = \begin{bmatrix} 2 & 3 & 1 \\ 4 & 7 & 1 \\ 6 & 18 & 2 \end{bmatrix} \]
Detailed Process
To understand LU decomposition more practically, let’s break it down step by step:
-
Calculate \( L \) and \( U \) Manually
- For the matrix \( A \), let’s conduct Gaussian elimination to determine \( L \) and \( U \).
-
Verify with MATLAB
Let's see how this can be achieved in MATLAB:
A = [2, 3, 1; 4, 7, 1; 6, 18, 2]; % Define the matrix
[L, U, P] = lu(A); % Perform LU decomposition with pivoting
% Display results
disp('Lower Triangular Matrix L:');
disp(L);
disp('Upper Triangular Matrix U:');
disp(U);
disp('Permutation Matrix P:');
disp(P);
In the above MATLAB code, we define the matrix \( A \), apply the `lu` function, and obtain \( L \), \( U \), and the permutation matrix \( P \). Displaying these matrices helps confirm our results.
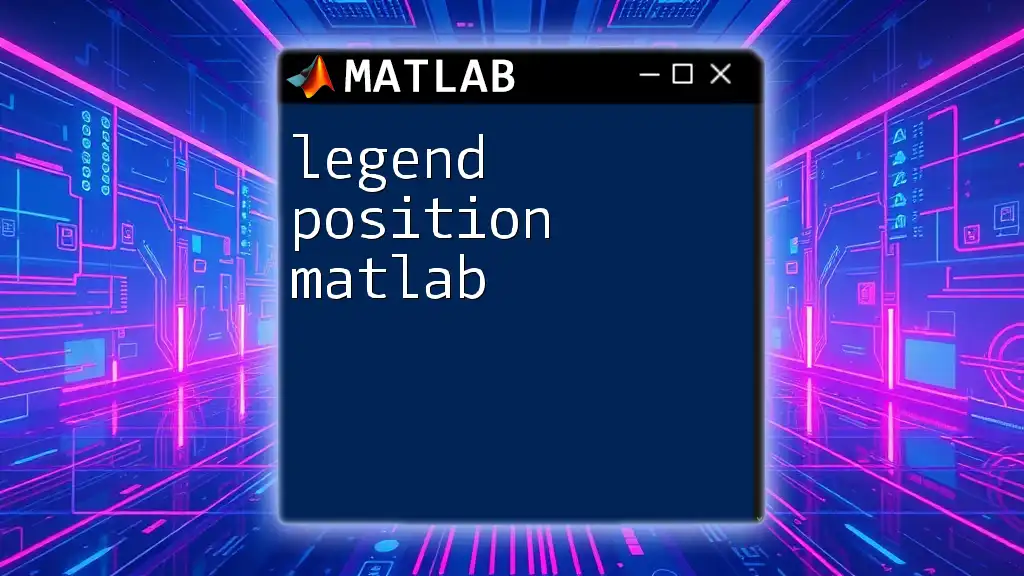
Applications of LU Decomposition
Solving Linear Systems
With LU decomposition, solving systems of linear equations becomes remarkably more efficient.
Expressing Systems as Ax = b
For the system \( Ax = b \), we can substitute our LU factorization to get:
\[ LUx = b \]
This means we can break it down into two steps:
-
Step 1: Solve \( Ly = Pb \)
- This is a forward substitution where we manipulate the equation to find \( y \).
-
Step 2: Solve \( Ux = y \)
- This is a back substitution to retrieve \( x \).
Code Example for Solving
Here’s how this can be executed in MATLAB:
b = [5; 11; 21]; % Corresponding vector for the system
y = L \ (P*b); % Forward substitution to find y
x = U \ y; % Back substitution to find x
In this example, we first compute \( y \) by solving the equation \( Ly = Pb \) and then derive \( x \) by solving \( Ux = y \).
Matrix Inversion
LU decomposition can also be leveraged to find the inverse of a matrix, a critical operation in many applications. By rearranging the `LU` decomposition, you can express the inverse \( A^{-1} \) as follows:
\[ A^{-1} = U^{-1} L^{-1} \]
Code Example: Matrix Inversion
Using LU decomposition for matrix inversion in MATLAB looks like this:
A_inv = U \ (L \ P); % Inverting matrix using LU
In this snippet, \( A^{-1} \) is obtained by first solving for \( L^{-1}y \) and then \( U^{-1}x \), effectively yielding the inverse of matrix \( A \).
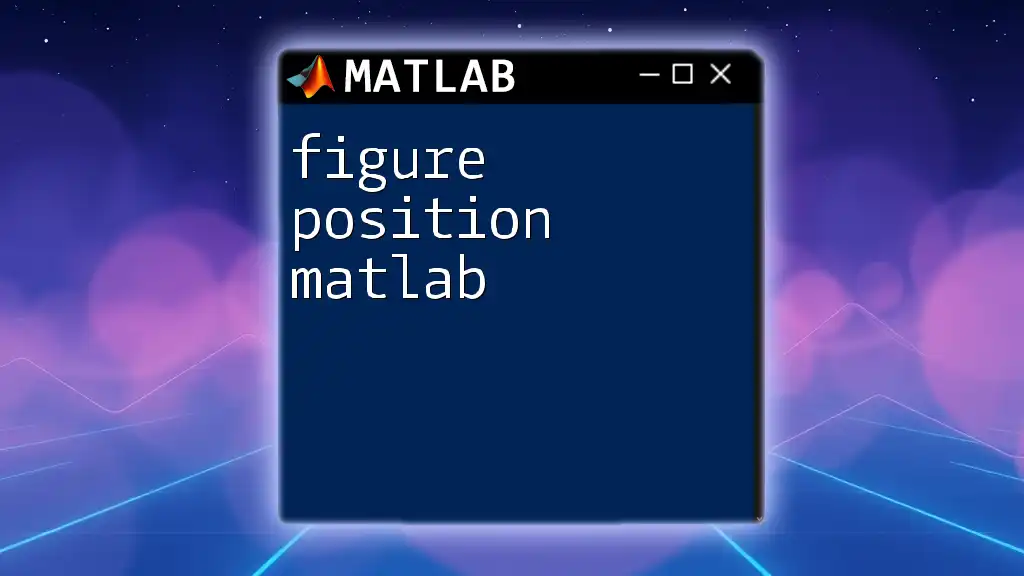
Performance Considerations
Computational Efficiency
LU decomposition is generally more efficient than direct methods. Instead of solving \( n^3 \) operations for larger matrices, it is possible to reduce this to a complexity of \( O(n^2) \), specifically when leveraging triangular systems.
Limitations and Common Pitfalls
While LU decomposition boasts excellent performance, certain matrices like singular or ill-conditioned ones may present challenges. If \( A \) is singular, LU decomposition fails because we cannot perform division by zero.
Resource Management
When dealing with very large matrices, be cautious of memory requirements. MATLAB can struggle with enormous matrices, so ensure that your system can handle the computational load or consider breaking the problem down into manageable parts.
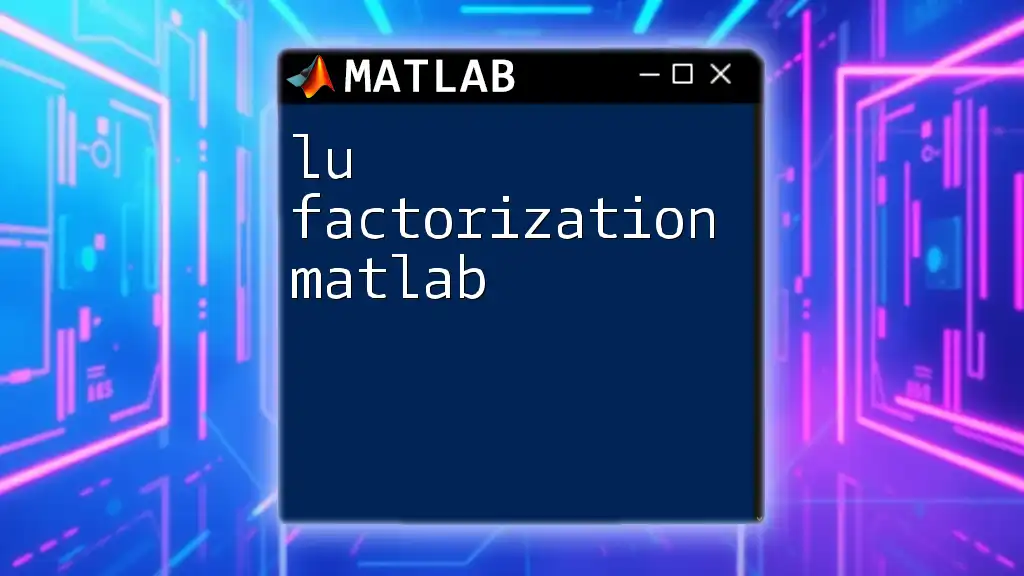
Troubleshooting Common Errors
Common MATLAB Errors
When executing LU decomposition in MATLAB, you may encounter error messages related to matrix dimensions or singularity. Understanding these messages can significantly expedite your troubleshooting process.
Debugging Tips
Verifying Input Dimensions: Always double-check that your matrix \( A \) is square.
Step-by-Step Validation: In complex matrices, validate each part of the decomposition by confirming intermediate outputs. This can help identify where issues may arise.
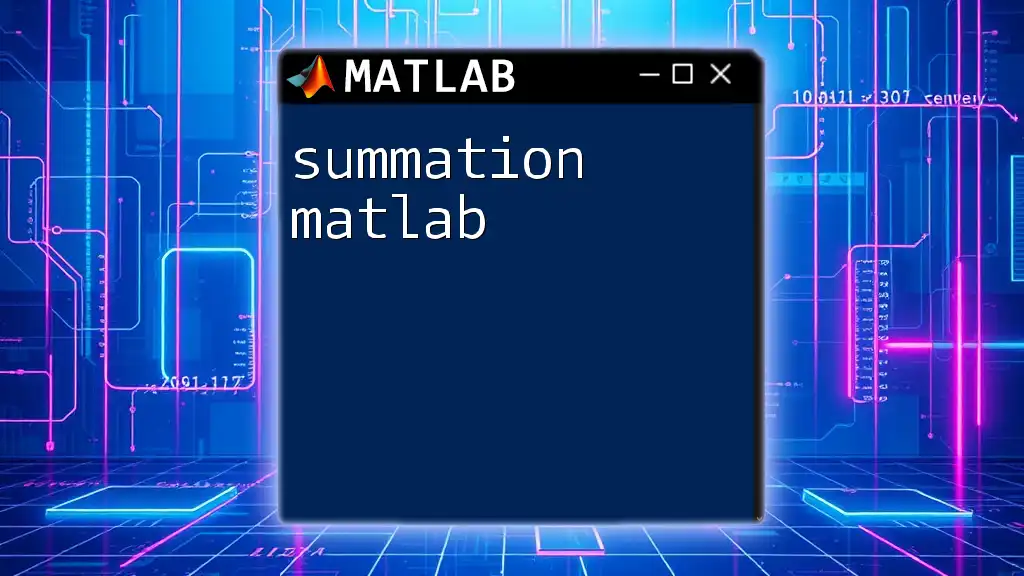
Conclusion
LU decomposition in MATLAB is a powerful mathematical tool that enhances the efficiency of solving linear systems and performing matrix operations. Its implementation within MATLAB not only simplifies complex calculations but also ensures accurate numerical results. By leveraging the built-in `lu` function, users can effectively break down their matrices for various applications.
As you dive deeper into MATLAB and linear algebra, consider exploring further computational techniques, such as Cholesky and QR factorizations, to broaden your mathematical skillset. Embrace the challenge and keep expanding your knowledge—the possibilities are endless!
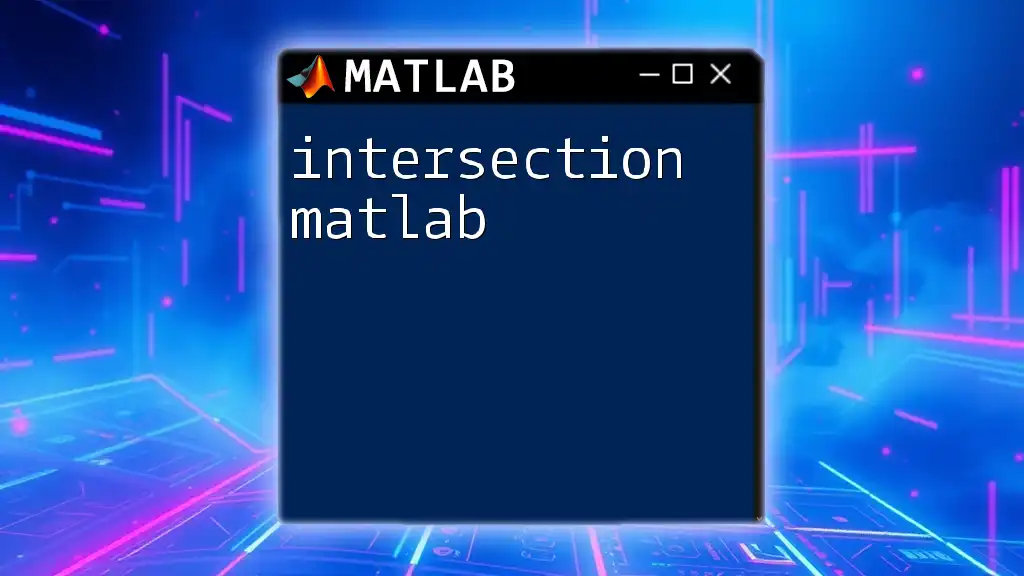
Additional Resources
For further exploration, refer to the official [MATLAB documentation on `lu`](https://www.mathworks.com/help/matlab/ref/lu.html). Additionally, online tutorials and community forums can provide valuable insights and peer support. Whether you're solving simple equations or handling large datasets, understanding LU decomposition equips you with the tools necessary for success in computational mathematics.