The `linspace` function in MATLAB generates a linearly spaced vector containing a specified number of points between two given limits.
Here's a code snippet example:
% Create a vector of 5 points linearly spaced between 1 and 10
vector = linspace(1, 10, 5);
Understanding `linspace`
What is `linspace`?
The `linspace` function in MATLAB is a highly efficient tool for generating vectors of linearly spaced values. The function can be utilized by calling it with the following syntax:
linspace(start, stop, num_points)
In this syntax:
- start: Sets the beginning value of the sequence.
- stop: Sets the end value.
- num_points: Optional and specifies how many evenly spaced points to generate between the start and stop values.
If the third parameter is omitted, `linspace` defaults to generating 100 points between the start and stop values.
Importance of Linearly Spaced Vectors
Linearly spaced vectors are critical in various applications, particularly in data visualization and numerical analysis. For instance, they are often used in plotting continuous functions or simulating systems. By having values that are evenly distributed, you can better represent trends and relationships within your data, leading to clearer graphs and more accurate numerical results.
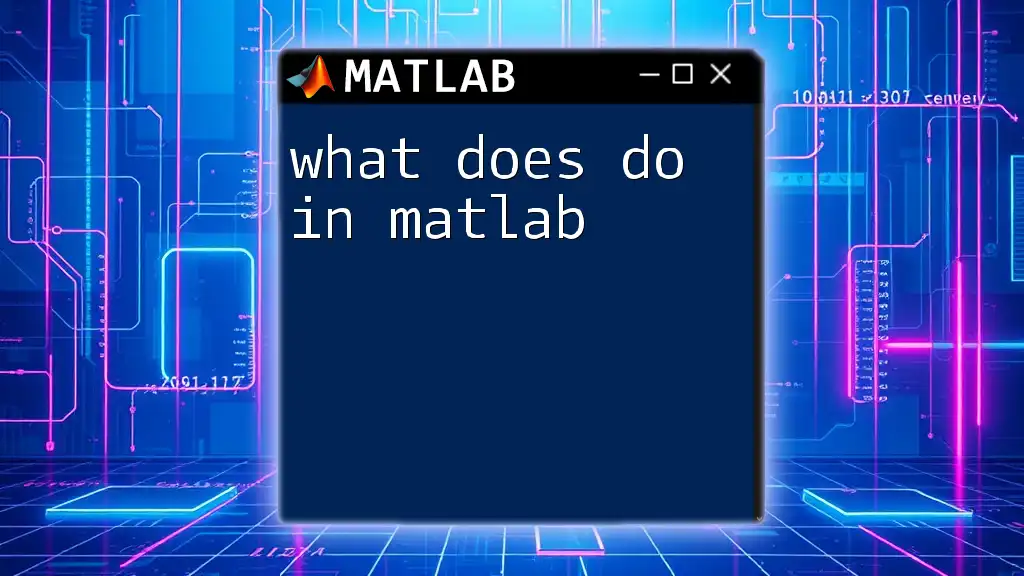
Syntax and Parameters
Basic Syntax
A straightforward example of `linspace` usage without specifying the number of points is:
x = linspace(1, 10);
In this example, MATLAB generates 100 points spanning the interval from 1 to 10. The default behavior of `linspace` to create a dense vector allows for a smooth representation of various functions when plotted.
Detailed Breakdown of Parameters
Start Parameter
The start value is where the generated vector begins. This parameter is inclusive, meaning the vector will include the start value itself.
Stop Parameter
Similarly, the stop value is the endpoint of the generated vector and is also inclusive. The `linspace` function ensures that the stop value appears in the resulting vector, providing a precise ending point.
Number of Points Parameter
When specifying the number of points, you dictate how many equally spaced values will be created between the start and stop. If omitted, MATLAB will default to 100 points.
Example of Specifying Number of Points
Consider the command:
y = linspace(0, 1, 5);
This creates a vector `y` containing 5 equally spaced points between 0 and 1, resulting in the values [0, 0.25, 0.5, 0.75, 1]. Such flexibility allows for tailored data generation, suitable for varying analytical needs.
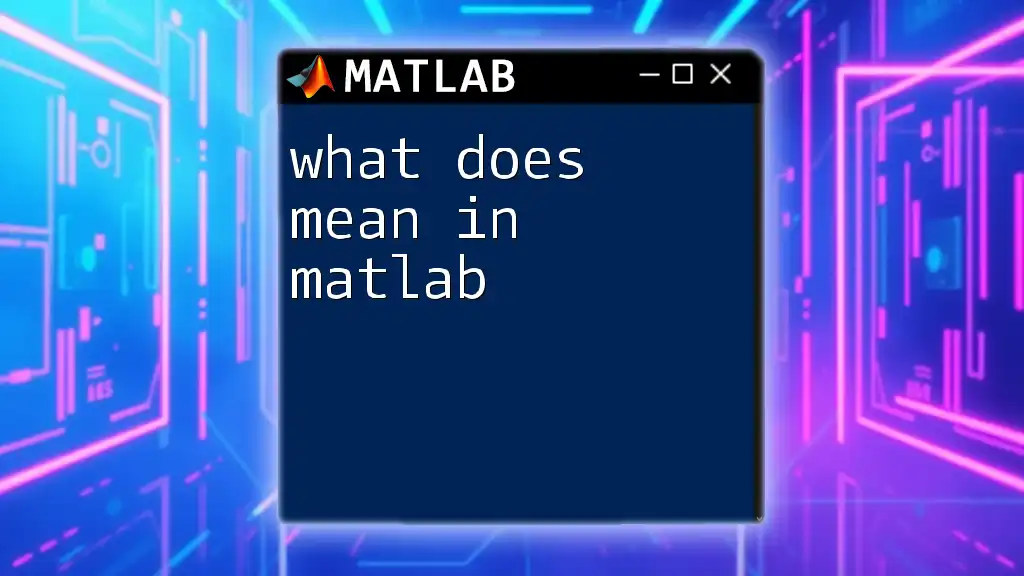
Practical Examples
Creating a Simple Vector
Here's how you can create a basic vector using `linspace`:
vec = linspace(-5, 5);
This generates a vector of 100 points ranging from -5 to 5. This approach is advantageous when you want to create a smooth transition across a range, which is important when analyzing data or forming visualizations.
Plotting with `linspace`
`linspace` can be seamlessly integrated with MATLAB’s plotting capabilities. For example:
t = linspace(0, 2*pi, 100);
y = sin(t);
plot(t, y);
title('Sine Function');
xlabel('Time');
ylabel('Amplitude');
In this example, `linspace` generates 100 points from 0 to 2π, which are used to compute the sine function. The resulting plot demonstrates the periodic nature of the sine wave, showcasing how `linspace` can create precise vectors for such representations.
Using `linspace` for Function Representation
You can also use `linspace` to represent functions effectively. For instance:
x = linspace(-10, 10, 200);
y = x.^2; % Quadratic function
plot(x, y);
title('Quadratic Function: y = x^2');
In this case, we create 200 points between -10 and 10 to demonstrate the quadratic relationship. The plot produced outlines a parabola, clearly illustrating how spaced values can produce a smooth curve.
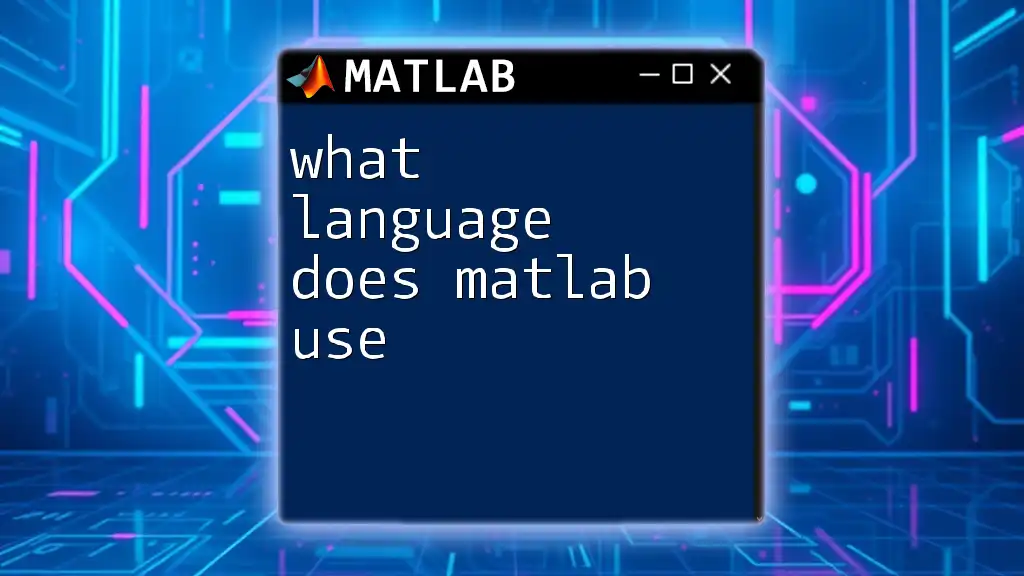
Advanced Usage
Creating Multidimensional Arrays with `linspace`
`linspace` is not limited to one-dimensional output; it can also generate multidimensional arrays. For example:
[X, Y] = meshgrid(linspace(-1, 1, 5));
This command generates two matrices (`X` and `Y`) from 5 linearly spaced values between -1 and 1. Using `meshgrid` allows you to work with surfaces and more complex plots efficiently.
Combining `linspace` with Other Functions
`linspace` can be combined effectively with other MATLAB functions for sophisticated computations. For example:
f = linspace(0, 100, 1000);
spectrum = abs(fft(sin(2*pi*10*f)));
Here, `linspace` helps generate a frequency vector for further processing, showcasing its versatility in numerical computing.
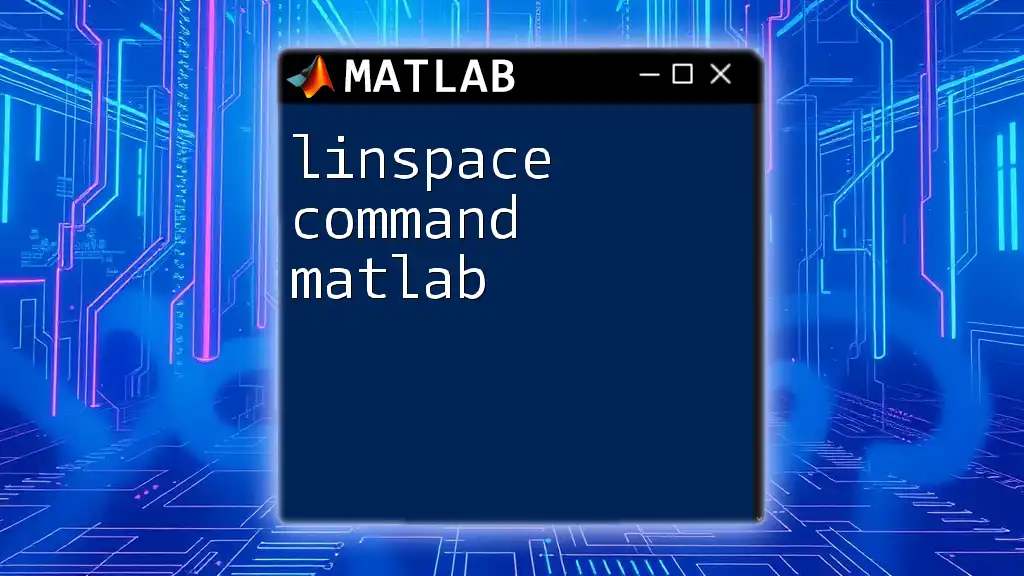
Tips for Optimizing Usage of `linspace`
Best Practices
One common guideline is to utilize `linspace` when you require an exact number of points, particularly in analytical contexts or when preparing visuals. In some cases, though, you may prefer the colon operator (`:`) for simpler ranges where the interval is known.
Common Pitfalls
While `linspace` is straightforward, users often forget that specifying fewer points can lead to a loss of detail in graphs. For smooth curves, ensure that the number of points adequately captures the nuances of the function being plotted.
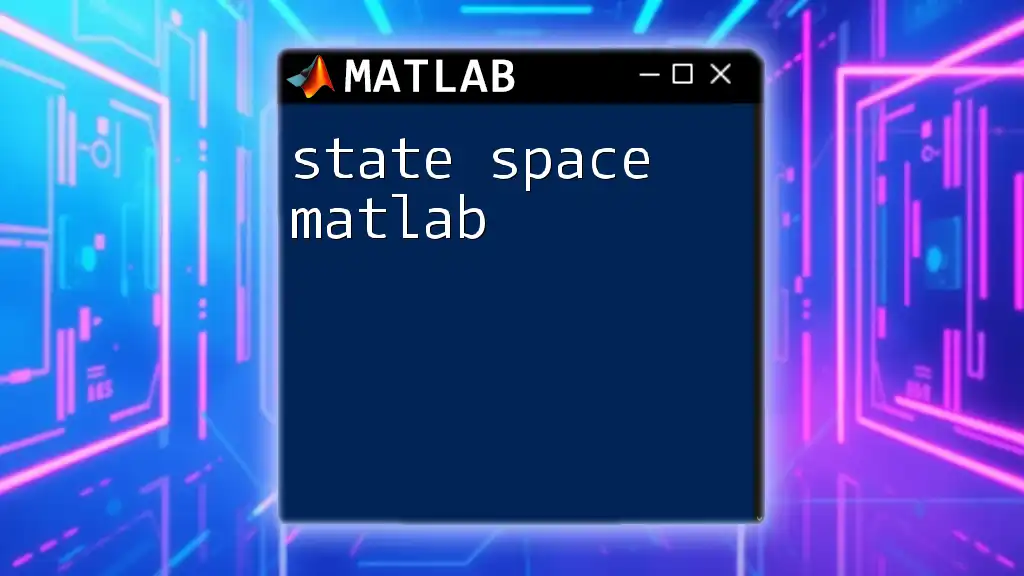
Conclusion
In summary, understanding what does linspace do in MATLAB enriches your MATLAB experience significantly. It serves as a powerful function in generating linearly spaced vectors essential for data analysis and visualization. Integrating `linspace` into your MATLAB toolkit will undoubtedly enhance your ability to model, simulate, and analyze mathematical functions effectively.
By practicing with various `linspace` applications, you'll gain a deeper understanding of its utility in MATLAB, empowering your projects with precise and effective data representation.