MATLAB is a high-level programming language and interactive environment used for numerical computation, visualization, and programming, allowing users to analyze data, develop algorithms, and create models.
Here's a simple code snippet to demonstrate MATLAB syntax:
% Calculate the sum of an array
array = [1, 2, 3, 4, 5];
total = sum(array);
disp(total); % Display the result
The Origins of MATLAB
Historical Background
MATLAB was developed in the late 1970s by Cleve Moler at The MathWorks. It was originally created as a simple tool to provide access to LINPACK and EISPACK, two libraries for numerical analysis and linear algebra. Over the years, MATLAB has evolved significantly and is now widely used across various industries, including engineering, finance, and data science. Its flexibility and powerful computational capabilities have made it a favorite among researchers and professionals.
What does MATLAB stand for?
The name MATLAB stands for MATrix LABoratory. This highlights the language's primary focus on matrix calculations and linear algebra, which are fundamental for many engineering and scientific computations. The matrix-centric design offers a unique advantage, allowing users to work efficiently with complex sets of data and perform high-level mathematical operations with ease.

Key Features of MATLAB
High-Level Programming Language
MATLAB is characterized as a high-level programming language, which means it abstracts away much of the complexity associated with lower-level languages such as C or Assembly. This simplicity allows users to express their ideas and algorithms concisely and clearly. MATLAB code is typically much shorter than equivalent code in languages like Python or C++, making it easier to write, read, and maintain.
Built-in Functions and Toolboxes
One of the standout features of MATLAB is its extensive collection of built-in functions and specialized toolboxes. Built-in functions can handle a wide range of tasks, from basic arithmetic to advanced numerical methods. For example, the `sum`, `mean`, and `fft` functions allow users to perform aggregate analysis, statistical calculations, and Fourier transforms with a single command.
Specialized Toolboxes are available for specialized tasks, covering areas such as signal processing, image processing, and machine learning. By installing the relevant toolbox, users gain access to functions that enable them to perform sophisticated analyses tailored to their domains.
Visualization Capabilities
MATLAB excels in data visualization, allowing users to create a variety of plots and graphs effortlessly. Its intuitive plotting functions help users interpret their data quickly and effectively. For example, to plot a simple sine wave, you can use the following code:
% Example of plotting a sine wave
x = 0:0.1:10; % Generate x-values
y = sin(x); % Compute y-values
plot(x, y); % Create the plot
title('Sine Wave');
xlabel('x');
ylabel('sin(x)');
This snippet demonstrates how MATLAB makes it simple to visualize data and identify trends, patterns, or anomalies within datasets.
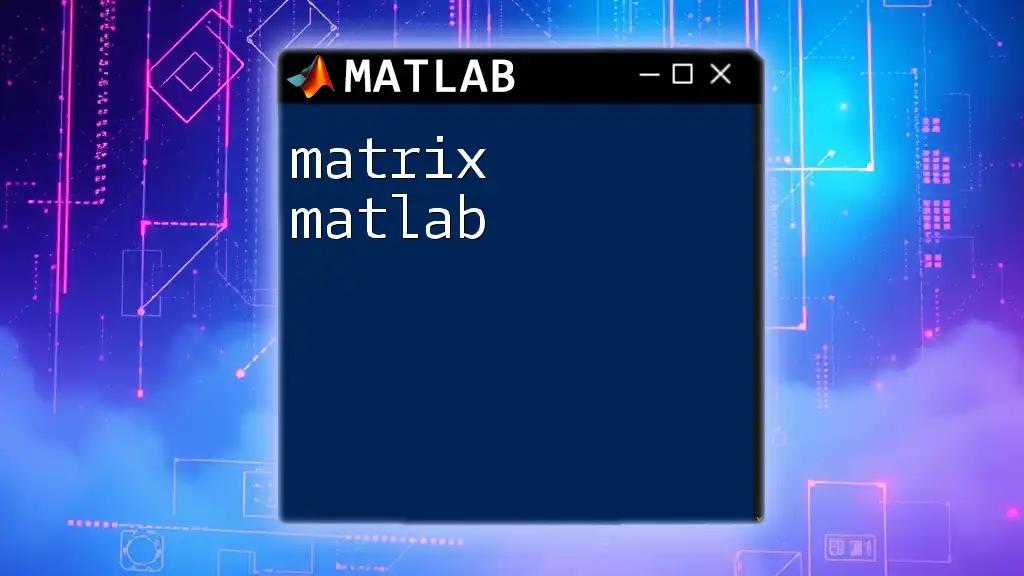
Core Applications of MATLAB
Engineering Applications
MATLAB has a prominent presence in the engineering field. Professionals use it to design control systems, analyze signals, and optimize communications. For instance, aerospace and automotive engineers rely on MATLAB’s robust capabilities to simulate and validate their designs, ensuring safety and efficiency.
Data Analysis and Visualization
In the realm of data science, MATLAB serves as a powerful tool for statistical analysis and data visualization. By leveraging MATLAB's built-in functions, users can extract meaningful insights from large data sets, making informed decisions based on concrete evidence. Statistical models and algorithms can be employed to forecast trends and analyze complex relationships.
Machine Learning and AI
With the rise of machine learning and AI, MATLAB has positioned itself as a valuable asset in this space. The Statistics and Machine Learning Toolbox allows users to develop algorithms and build predictive models with relative ease. Users can apply supervised or unsupervised learning methods to train their models and validate results through cross-validation techniques.
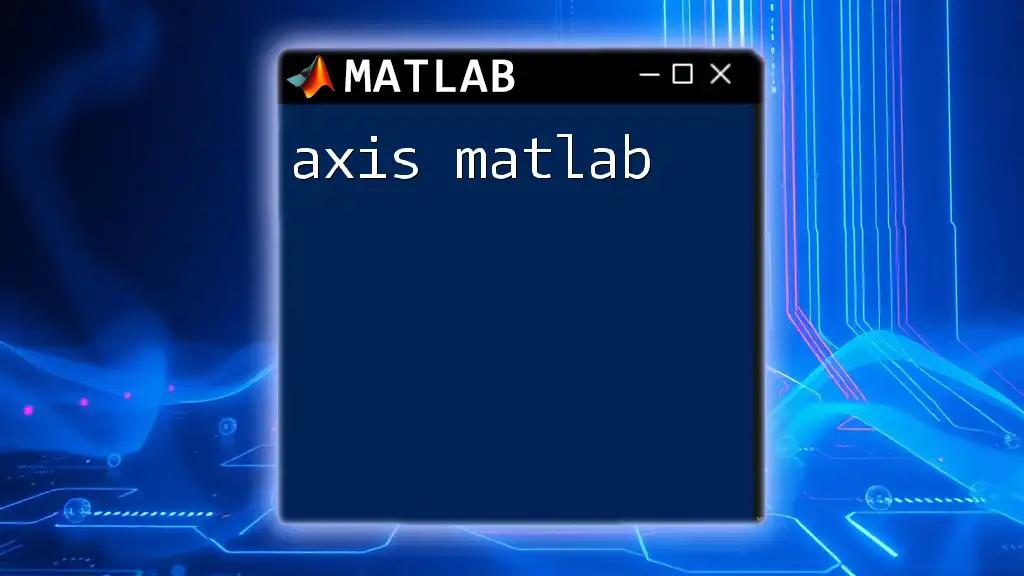
Getting Started with MATLAB
Installing MATLAB
Getting started with MATLAB is straightforward. You can easily install MATLAB on various platforms, including Windows, Mac, and Linux. Simply download the installer from The MathWorks website, follow the prompts, and you will have MATLAB up and running in no time.
MATLAB Editor and Command Window
Upon installation, users can explore the MATLAB Editor and Command Window. The Editor is where you can write and save scripts, while the Command Window allows you to execute commands interactively. For instance, you can display text in the Command Window using:
% Simple command to display 'Hello, World!'
disp('Hello, World!');
Creating Scripts and Functions
To maximize your productivity in MATLAB, it is essential to understand the difference between scripts and functions. Scripts are files containing a series of MATLAB commands intended to be executed together, whereas functions can take inputs and return outputs. Below is an example of a simple function that adds two numbers:
function result = addNumbers(a, b)
result = a + b;
end
Using functions allows for more organized and reusable code, enhancing your MATLAB programming experience.
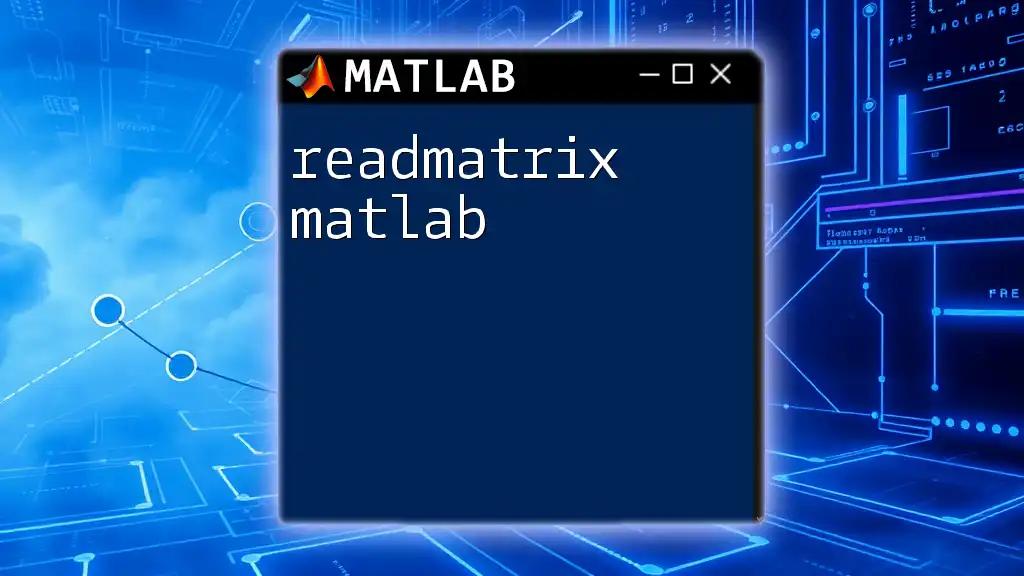
Practical Examples in MATLAB
Basic Numerical Computations
MATLAB can handle a variety of basic numerical computations. Users can perform operations like sums, products, and square roots with simple syntax. For example, calculating the square root of a number can be done using:
% Calculate the square root
number = 16;
sqrt_result = sqrt(number);
disp(['The square root of ', num2str(number), ' is ', num2str(sqrt_result)]);
Data Visualization Techniques
Visualizing data in MATLAB involves more than just basic plots; it provides advanced options for creating insightful graphics. For example, you can create a 3D surface plot with the following code:
% Example of a 3D surface plot
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
surf(X, Y, Z);
title('3D Surface Plot');
This showcases one of the many visualization techniques available in MATLAB, helping users present their findings effectively.
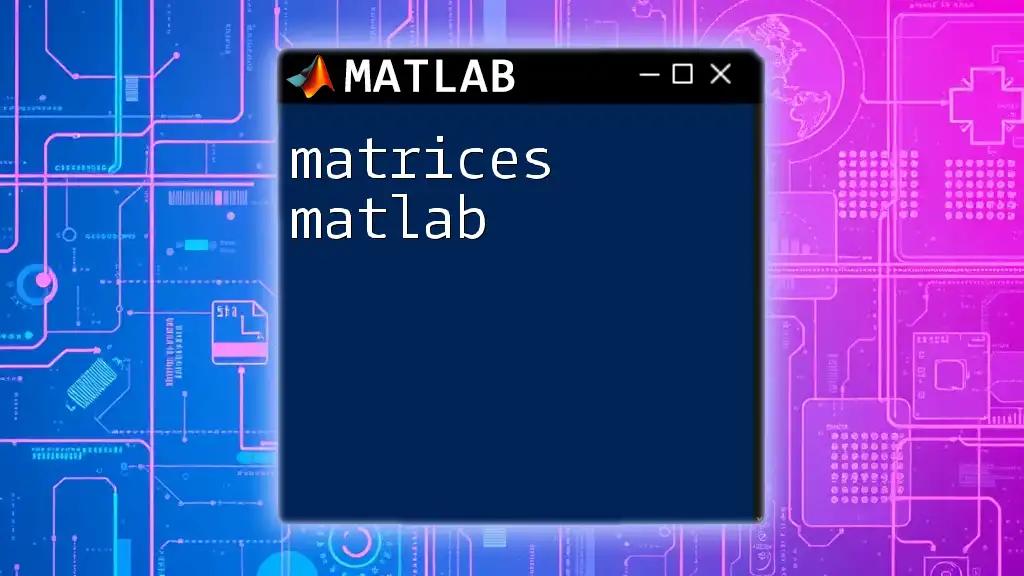
Advantages of Using MATLAB
Ease of Learning
One of the primary advantages of using MATLAB is its ease of learning. With numerous tutorials and documentation available, newcomers can quickly grasp the fundamentals. The intuitive syntax is designed to help users focus on their problems rather than getting bogged down by complex programming constructs.
Extensive Community and Support
MATLAB has a large and active user community, which is invaluable for learners and professionals alike. Users can share code, ask questions, and access solutions via online forums. Additionally, resources like the File Exchange allow users to share their scripts and functions with the community, promoting collaboration and continuous improvement.
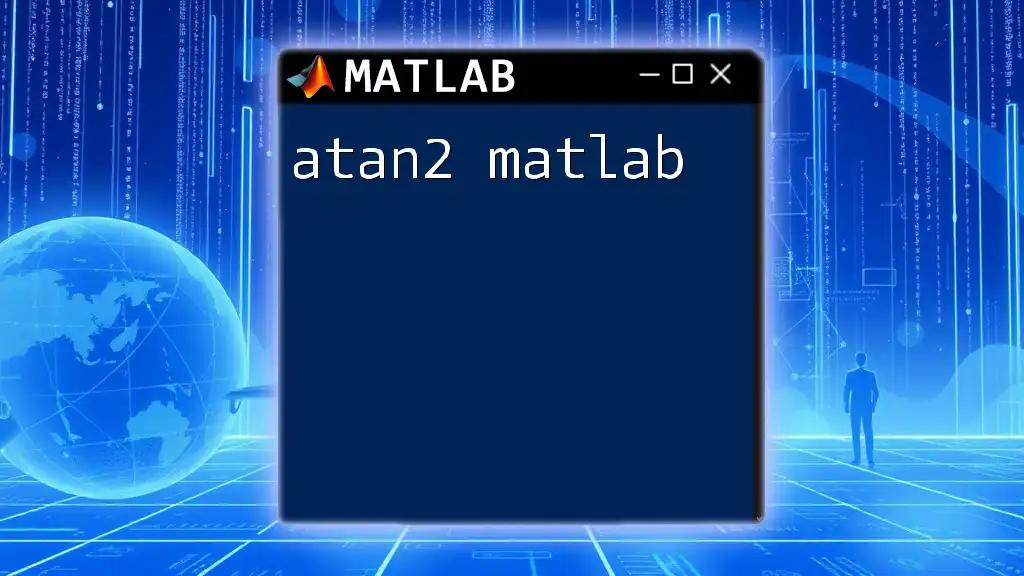
Conclusion
MATLAB is a powerful tool that has become integral in various fields, including engineering, data science, and machine learning. With its easy-to-learn syntax and rich feature set, MATLAB empowers users to tackle complex problems efficiently. Whether you’re a beginner or an experienced programmer, MATLAB offers the resources you need to succeed.
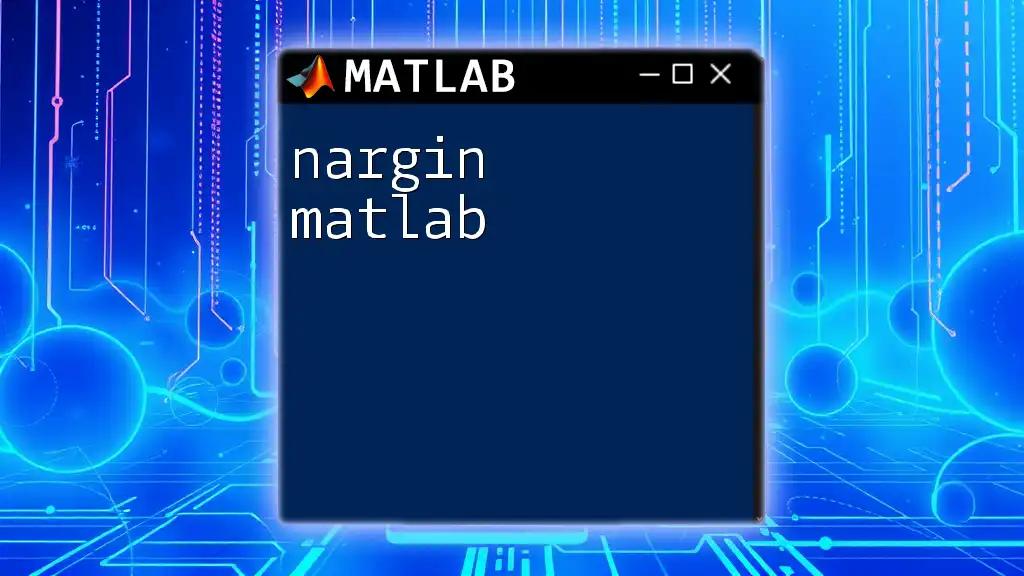
Call to Action
Join our MATLAB community today! Subscribe to our tutorials and courses to deepen your understanding of MATLAB and enhance your skills. We encourage you to share your experiences and questions in the comments section—let's learn together!