The `linspace` command in MATLAB generates a linearly spaced vector of specified length between two given endpoints.
Here's a code snippet that demonstrates its use:
% Generate 5 linearly spaced points between 1 and 10
vector = linspace(1, 10, 5);
Understanding `linspace`
What is `linspace`?
The `linspace` command in MATLAB is a powerful tool used for generating evenly spaced vectors. Unlike the colon operator or other methods, `linspace` provides a straightforward way to specify both the start and end points, alongside the exact number of points you wish to create. This becomes particularly valuable when you need precise control over the data you generate, whether for analysis or visualization.
Syntax of `linspace`
The basic syntax of the `linspace` command is as follows:
linspace(start, end, numPoints)
This syntax is comprised of three key components:
- `start`: The beginning value of the interval.
- `end`: The ending value of the interval.
- `numPoints`: (Optional) The total number of points to generate between the start and end values, inclusive.
By specifying these parameters, you can create a vector tailored to your specific needs.
Parameters Explained
`start`
The `start` parameter determines where the generated vector begins. This can be any real number, and the choice of the starting point greatly affects the results you get.
For example, if you choose a start value of `0`, you signal that the vector should begin at zero.
`end`
The `end` parameter marks the conclusion of your vector. Similar to the start value, this can also be any real number, and it acts as the upper limit of your generated set.
`numPoints`
The `numPoints` parameter specifies how many values you wish to generate in the interval. If you omit this parameter, MATLAB defaults to generating 100 points. It's important to understand that the specified endpoints (`start` and `end`) are included in this resultant vector, regardless of the value of `numPoints`.
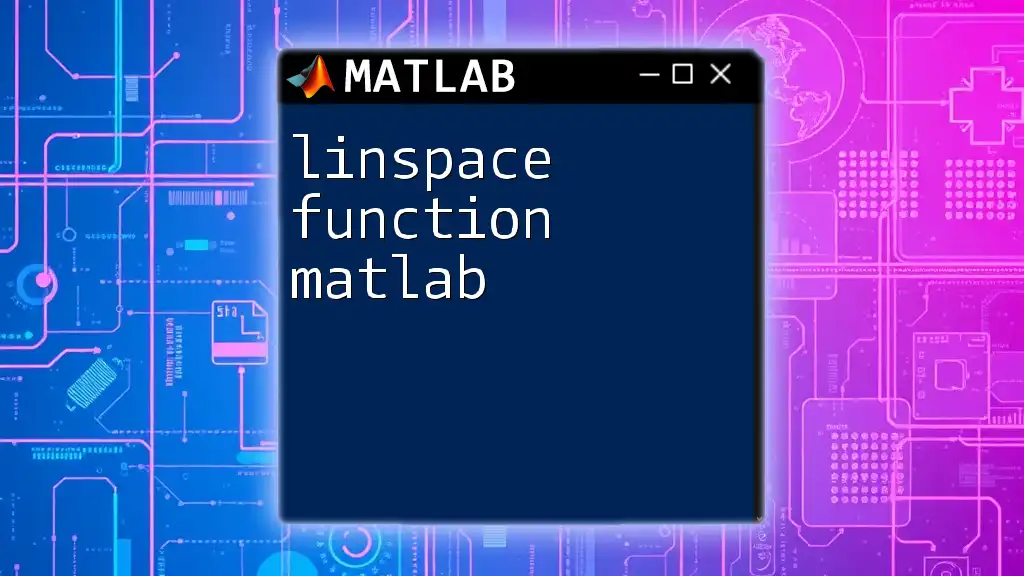
Practical Applications of `linspace`
Generating a Simple Vector
To illustrate the use of `linspace`, consider the following example: generating a vector from `0` to `1` with `5` points. This simple command creates a vector with evenly distributed values:
vec = linspace(0, 1, 5);
The output in this case would be:
vec = 0 0.25 0.5 0.75 1
Each value represents a quarter of the total range, showcasing how `linspace` operates effectively in producing uniform segments.
Using `linspace` for Plotting
The `linspace` command shines when applied to plotting functions. For example, if you want to plot the sine function, you first need a range of x-values:
x = linspace(0, 2*pi, 100);
y = sin(x);
plot(x, y);
title('Sine Function');
xlabel('x (radians)');
ylabel('sin(x)');
In this code, `linspace` generates `100` evenly spaced values between `0` and `2π`. The sine function is then plotted over this range, yielding a smooth curve visualizing the periodic nature of the sine wave.
Creating Non-Unit Intervals
`linspace` isn’t limited to unit intervals. For instance, if we want a vector ranging from `2` to `10` with `5` points, we can use:
vec = linspace(2, 10, 5);
This results in:
vec = 2 4 6 8 10
Here, `linspace` produced a vector with two-unit spacing between each number, illustrating its flexibility in generating vectors over any arbitrary range.
Vectorization and Performance
One of the biggest advantages of using `linspace` is the efficiency it offers in vectorized operations. For larger datasets, using `linspace` considerably enhances code performance compared to loops or other methods.
Example: Consider the difference in creating a vector of `1,000,000` points ranging from `0` to `1`. Utilizing `linspace` is computationally more efficient than iterating through a loop to generate the same data. Time comparisons can show marked reductions in execution time:
% Using linspace
tic;
large_vec = linspace(0, 1, 1000000);
toc;
% Using a loop (not efficient)
tic;
large_vec = zeros(1, 1000000);
for i = 1:1000000
large_vec(i) = (i-1)/999999;
end
toc;
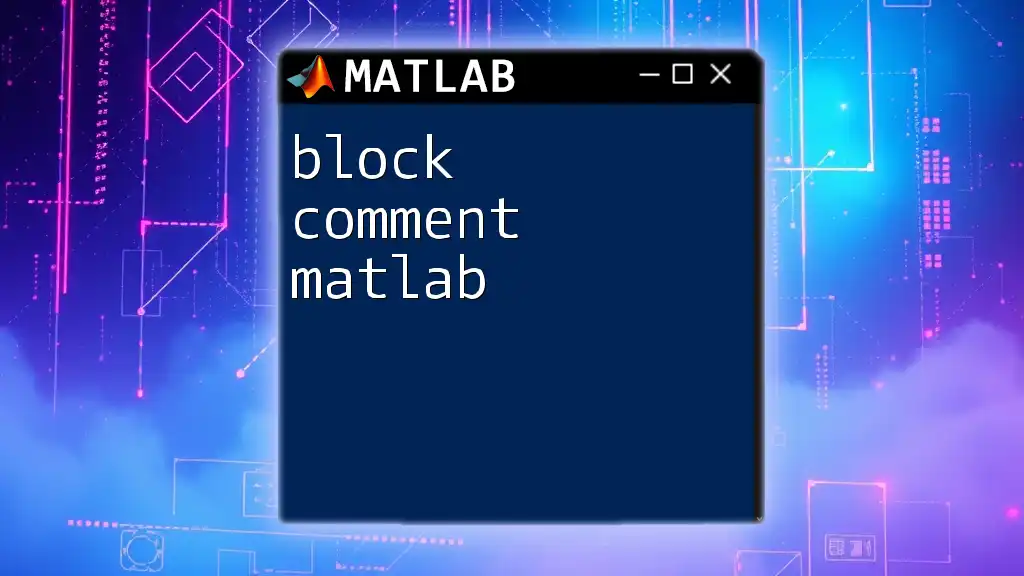
Advanced Usage of `linspace`
Using `linspace` with Complex Numbers
The versatility of `linspace` extends beyond real numbers. You can also generate complex numbers. If you want to create a vector of complex numbers ranging from `0` to `1 + 1i` over `5` points, simply employ:
z = linspace(0, 1+1i, 5);
This results in the following vector of complex numbers:
z = 0 + 0i 0.25 + 0.25i 0.5 + 0.5i 0.75 + 0.75i 1 + 1i
The ability to combine real and imaginary parts using `linspace` makes it useful for simulations involving complex dynamics.
Nested `linspace` for Multi-Dimensional Data
When you need to create multi-dimensional grids, `linspace` can be integrated with the `meshgrid` function effectively. For example, if you want to create surfaces or mesh plots, using `linspace` together with `meshgrid` can be quite advantageous:
[X,Y] = meshgrid(linspace(-5, 5, 100), linspace(-5, 5, 100));
Z = X.^2 + Y.^2;
surf(X, Y, Z);
Here, `linspace` generates a grid in the x and y dimensions, and the z-values are computed from the function \(Z = X^2 + Y^2\). This creates a surface plot representing a quadratic function, demonstrating how `linspace` can support complex visualizations.
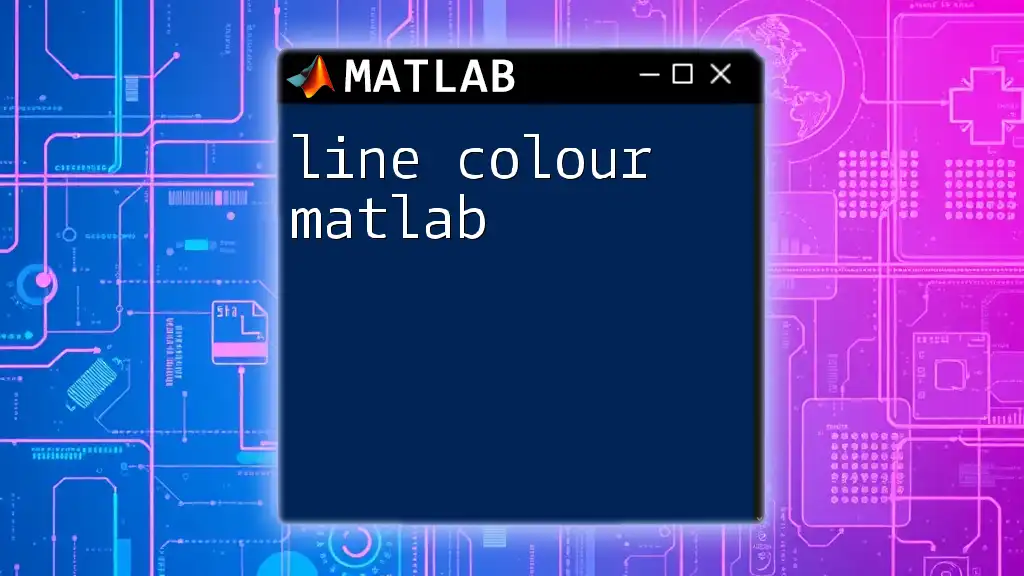
Common Mistakes in Using `linspace`
Misunderstanding the `numPoints` Parameter
A common mistake when utilizing `linspace` is misunderstanding how the `numPoints` parameter affects the endpoints. Some users may assume that the function should always create equal spacing, but they may forget that the endpoints are included in the total count of points. For instance, calling:
vec = linspace(0, 1, 3);
would produce:
vec = 0 0.5 1
This shows that it divides the interval into two segments, not three.
Not Accounting for Floating-Point Precision
When working with very large or very small numbers, floating-point precision can lead to unexpected values. If you're generating a large number of points with very small spacing, you might encounter precision errors. To manage this effectively, be aware of the representation and rounding issues in MATLAB, especially when dealing with limits close to the limits of floating-point representations.
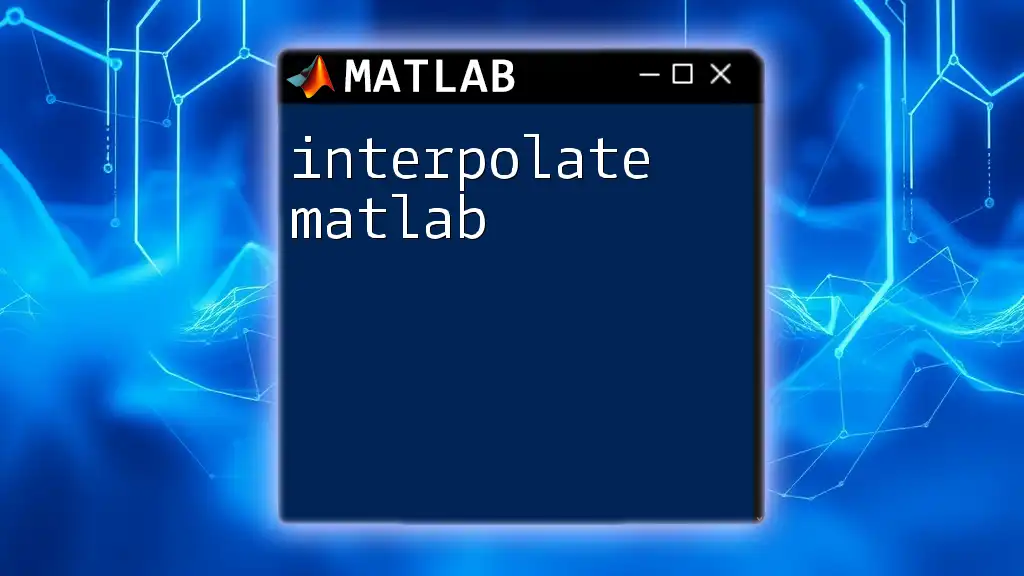
Conclusion
In conclusion, the `linspace` command in MATLAB is an essential tool for anyone working with vectors and matrices. Its ability to generate evenly spaced points, both for real and complex numbers, provides a flexible and efficient means of creating data for analysis or visualization. Whether you’re plotting functions or generating complex datasets, mastering the `linspace` command will undoubtedly enhance your MATLAB experience.
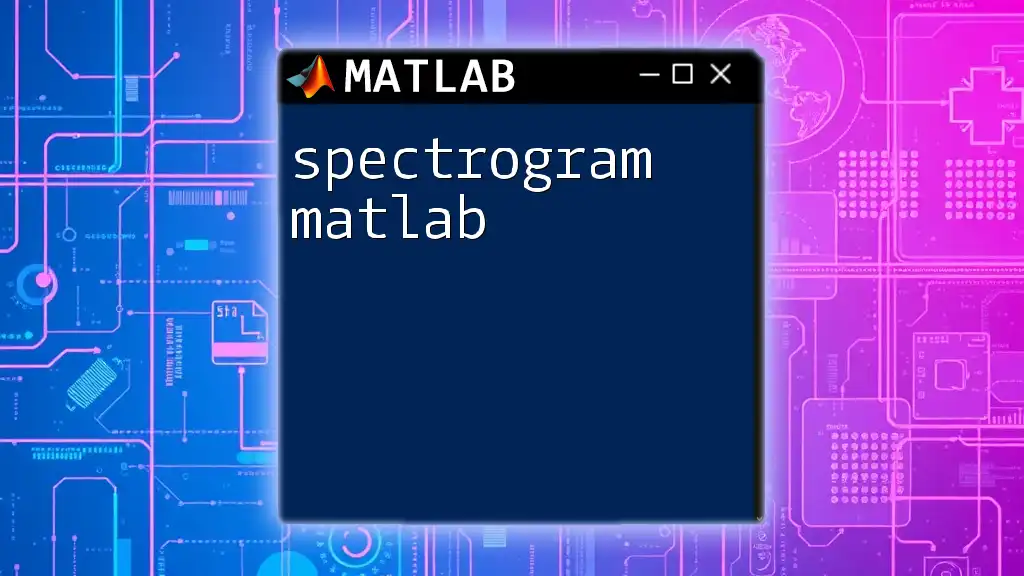
Further Resources
Recommended Readings
For a deeper understanding of MATLAB, consider exploring comprehensive MATLAB textbooks or online tutorials.
Online Platforms
Resources like MathWorks documentation and community forums will serve as effective tools for further exploration and assistance on various MATLAB topics.
Call to Action
Don’t miss out on more concise tutorials and tips on MATLAB commands. Be sure to engage with our community for additional learning and exploration!