The command `clear` in MATLAB is used to remove all variables from the workspace, allowing you to start fresh without any existing data.
clear
Understanding the MATLAB Workspace
What is a Workspace?
In MATLAB, the workspace serves as a vital area where all the variables created during a session are stored. It allows you to access and manipulate these variables while working on your scripts or functions. The workspace can contain both local variables (accessible only within specific scripts or functions) and global variables (accessible throughout the entire MATLAB environment).
Why Clear the Workspace?
Clearing the workspace becomes essential for several reasons:
- Avoiding Conflicts: Old variable names can clash with new variables, leading to unexpected behavior in your scripts.
- Improving Performance: A cluttered workspace can slow down MATLAB. By clearing unnecessary variables, you can enhance performance.
- Clarity: Regularly clearing your workspace helps keep your development environment clean and organized, making it easier to debug and manage code.
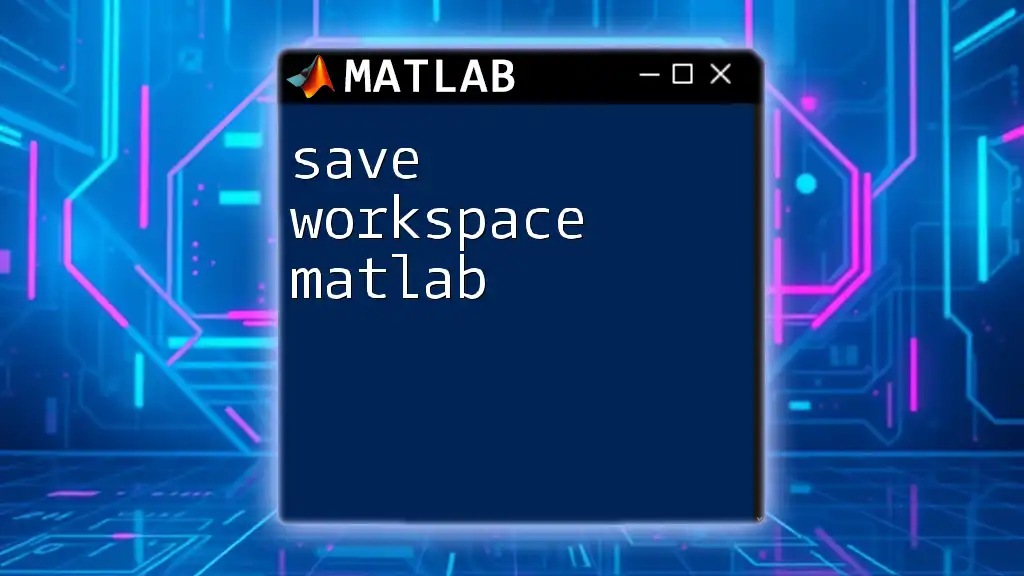
The clear Command
What Does the clear Command Do?
The clear command is the primary tool for managing variables in MATLAB. It removes specified variables from the workspace, essentially 'cleaning' your environment.
Basic Syntax
To clear all variables from the workspace, simply use:
clear
Clearing Specific Variables
If you want to clear only certain variables, specify their names:
clear variableName
For example, you can have:
a = 10;
b = 20;
clear a % Only 'a' will be removed from the workspace
In this case, after executing `clear a`, the variable `a` will no longer exist, while `b` will remain intact.
Clearing All Variables
To clear all variables simultaneously, use:
clear all
Be cautious with this command as it also clears global variables and all user-defined states, which may be crucial for your current project.
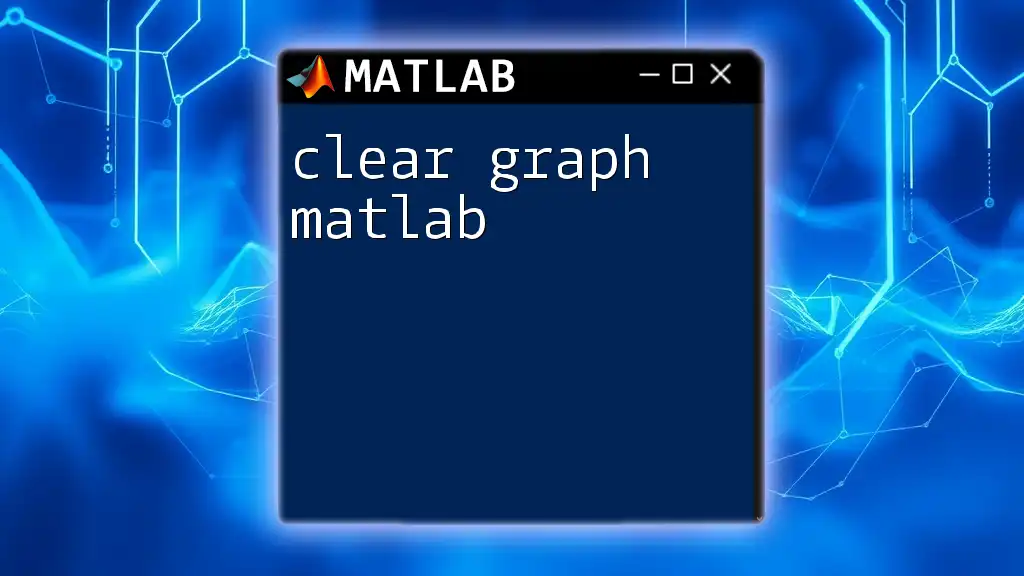
The clearvars Function
Introduction to clearvars
The clearvars function provides enhanced flexibility in managing variables in the workspace.
Syntax for Clearing Specific Variables
To clear specific variables, the usage is like:
clearvars variableName
Clearing Except Certain Variables
If you wish to keep some variables intact, you can use:
clearvars -except variableToKeep
For example:
x = 5;
y = 10;
clearvars -except x % This will preserve 'x' and remove 'y'
This command is especially useful when you have a large number of variables and only want to retain a few.
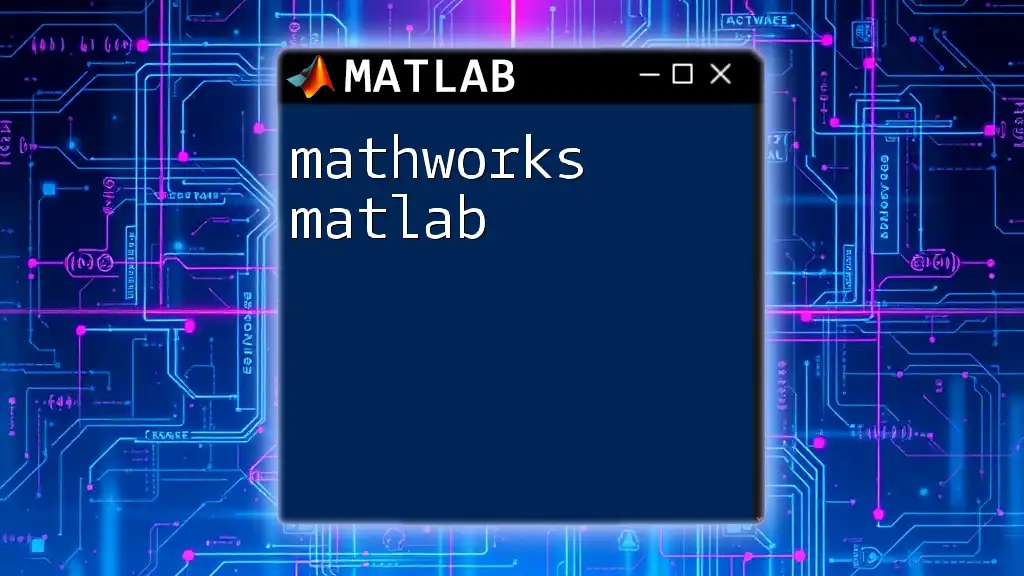
Clearing Functions and MEX Files
Usage of clear functions
When you clear functions, you're telling MATLAB to revert to the default state, effectively refreshing any cached function files. This can help avoid issues with updated function definitions:
clear functions
Working with MEX Files
MATLAB allows integration with other programming languages through MEX files. If you want to clear these files, use:
clear mex
This command is particularly important when you have made changes to MEX files and need to ensure that your session recognizes the updates.
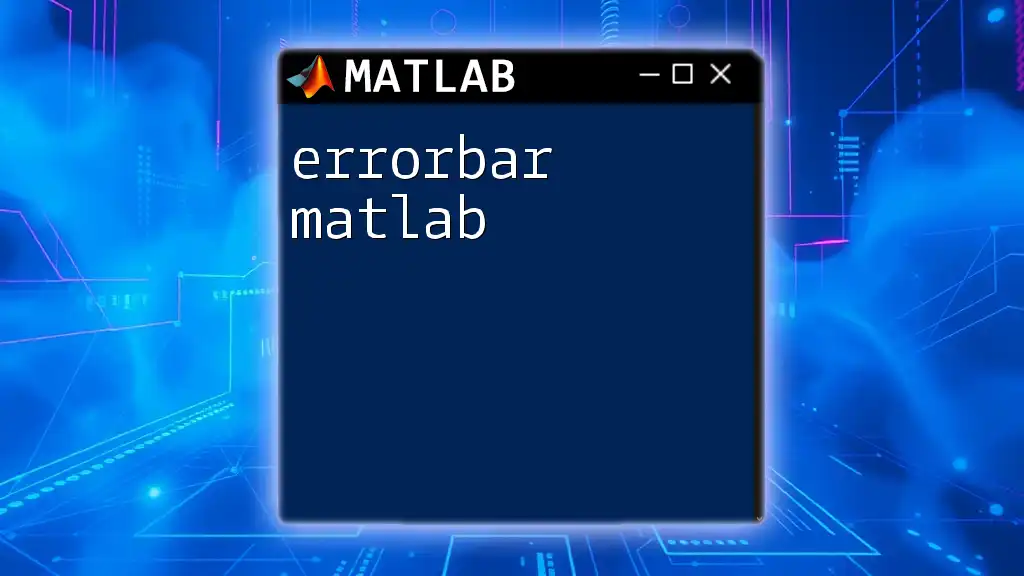
Best Practices for Clearing Workspace
When to Clear the Workspace
It's a good practice to clear your workspace at the beginning of a new script or function. This ensures that no leftover variables from previous computations interfere with your current work and leads to more predictable results.
Using Scripts and Functions
Adhering to well-defined scripts and functions minimizes workspace clutter. Creating modular code with specific input and output helps encapsulate variable storage and reduces the need for extensive workspace cleaning.
Regular Maintenance
Conduct routine cleaning of the workspace after substantial computations or data processing. By doing this regularly, you prevent excessive buildup of unused variables that can clutter your workspace and impede performance.
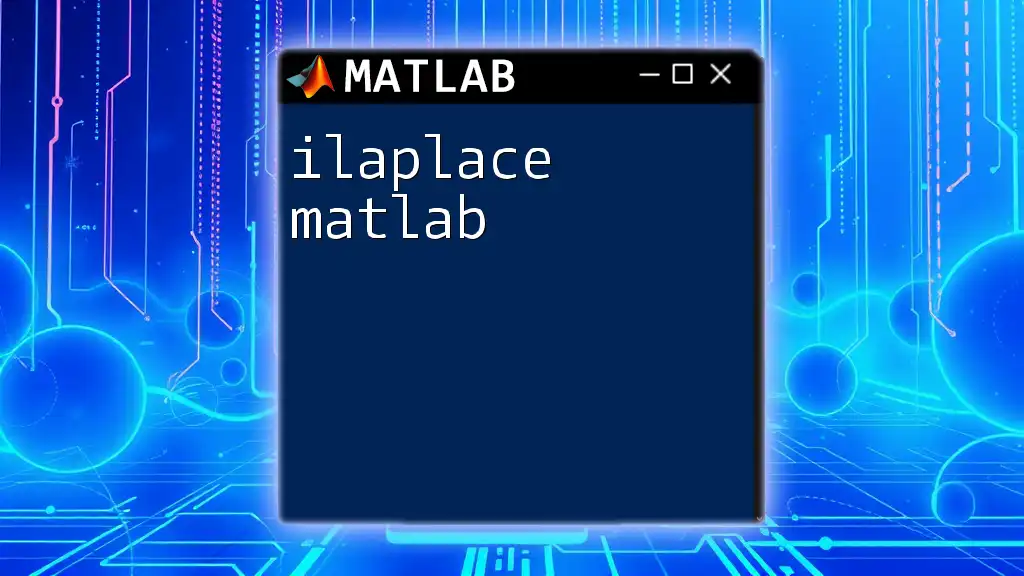
Additional Tips with Workspace Management
Using the clc Command
The clc command clears the command window, which is separate from clearing the workspace. This is valuable for maintaining a clear view of your output or errors:
clc
Use this command when you want to remove all previous command window output without affecting any variables in the workspace.
Displaying Workspace Contents
To view the current variables in your workspace, you can use:
whos
This command displays a list of all variables, along with their types and sizes, allowing you to assess what needs to be cleared.
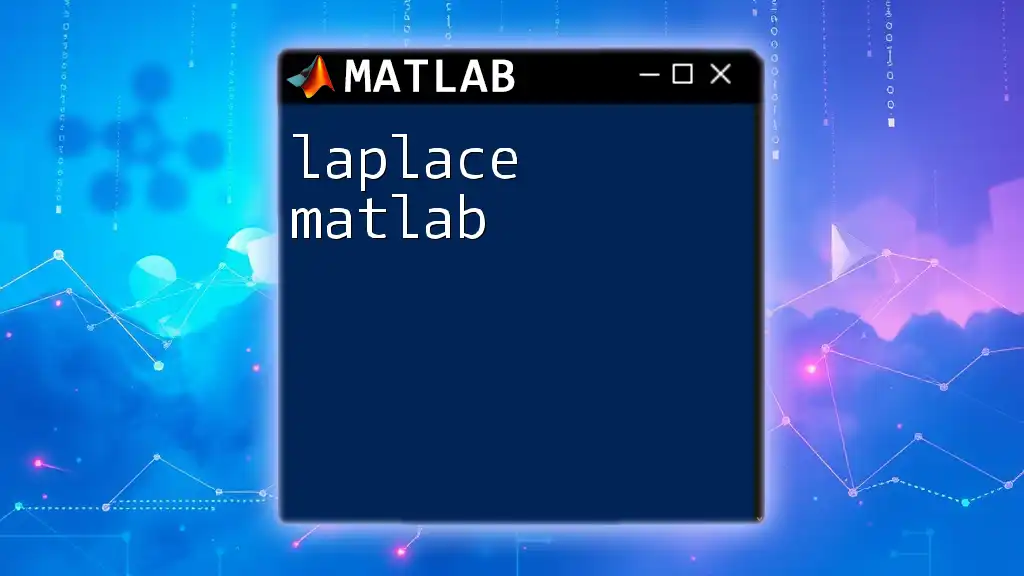
Conclusion
Managing your MATLAB workspace effectively is crucial for a smooth programming experience. By utilizing commands like clear, clearvars, and clc, you can maintain a well-organized coding environment that enhances productivity and reduces potential errors. Regularly practicing these workspace commands can significantly improve your workflow and foster better program management.
By grasping the essentials of the clear workspace MATLAB commands and principles, you'll set yourself up for success in your MATLAB endeavors.