The `linspace` function in MATLAB generates a vector of evenly spaced points between a specified start and end value, which is particularly useful for creating ranges of numbers for analysis or plotting.
Here’s a code snippet demonstrating the use of `linspace` to create a vector of 10 linearly spaced points between 1 and 5:
% Create a vector of 10 linearly spaced points between 1 and 5
vector = linspace(1, 5, 10);
What is the Linspace Function?
The linspace function in MATLAB is an essential tool for generating linearly spaced vectors. It is particularly useful for creating sequences in computational simulations, data visualization, and mathematical modeling. The function is versatile and often serves as an alternative to the more commonly known colon operator (`:`), yet it brings unique advantages, especially when precise control over the number of points is required in a specific range.

Syntax of the Linspace Function
The syntax for the linspace function in MATLAB is straightforward:
y = linspace(a, b, n)
Here:
- `a` is the start value of the sequence.
- `b` is the end value of the sequence.
- `n` (optional) is the number of points to generate between `a` and `b`. When `n` is not specified, the default value is 100.
This functionality allows users to create arrays, making it a critical part of many MATLAB operations.
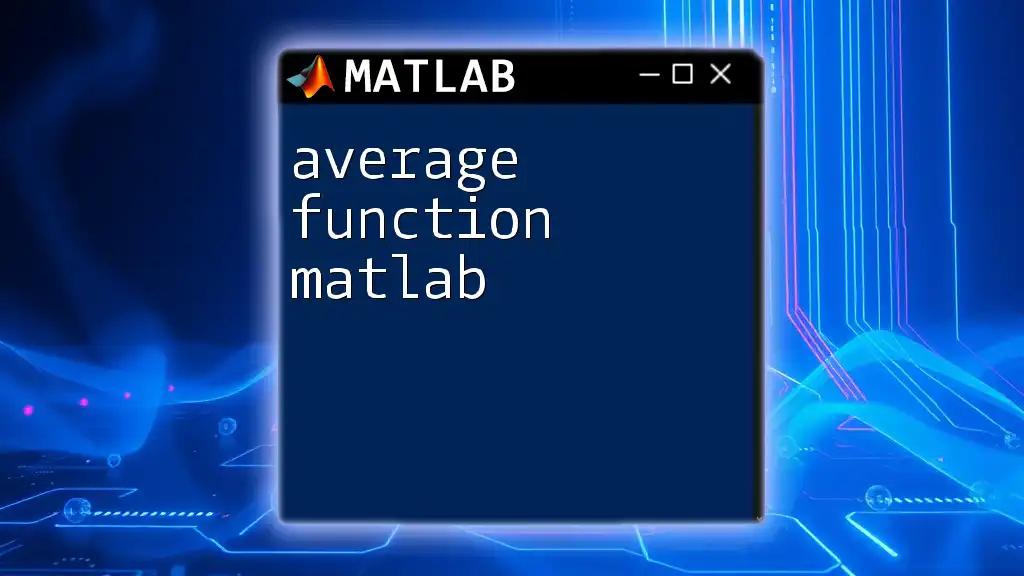
Understanding the Parameters
Start Value
The start value is the first number in the array generated by linspace. Choosing an appropriate start value sets the lower limit of your desired range. For instance:
linspace(1, 10, 5) % Starts at 1
This command generates a vector that starts at 1 and ends at 10, spreading a total of 5 points evenly between these values. The resultant vector will be:
`[1, 3.25, 5.5, 7.75, 10]`
End Value
The end value signifies where the sequence will conclude. It defines the upper limit of the output array. For example:
linspace(0, 100, 5) % Ends at 100
The above command produces an array starting from 0 to 100 with 5 equally spaced points:
`[0, 25, 50, 75, 100]`
Number of Points
The number of points parameter allows you to specify how many elements will be generated within the defined range. This is particularly useful for high-resolution applications, such as in plotting functions. Consider:
linspace(0, 1, 5) % Generates 5 points between 0 and 1
The output will consist of:
`[0, 0.25, 0.5, 0.75, 1]`
Controlling the number of points gives you flexibility depending on your computational needs.

Practical Applications of Linspace
Data Visualization
One of the primary applications of the linspace function in MATLAB is in data visualization. By generating a smooth set of values, linspace allows for the creation of smooth curves in plots. For example, if you want to visualize a sine wave, you can use:
x = linspace(0, 2*pi, 100);
y = sin(x);
plot(x, y);
This code produces a continuous and smooth sine wave by distributing 100 points between 0 and 2π, demonstrating how linspace aids in generating high-quality plots.
Engineering Calculations
The linspace function is also widely used in engineering calculations and simulations. In scenarios such as generating frequency values, linspace can create a range that meets specific analytical requirements. For example:
frequencies = linspace(20, 20000, 100);
This generates 100 frequencies evenly distributed between 20 Hz and 20,000 Hz, which can be crucial for signal processing and analysis.
Interpolating Functions
Interpolation is a method to estimate unknown values that fall between known data points. The linspace function is valuable when creating interpolation points for smoother estimations. For example:
x = linspace(0, 10, 6);
y = x.^2; % y values based on a quadratic function
This generates x values and computes corresponding y values from the quadratic function, making it easier to visualize and analyze the relationship.
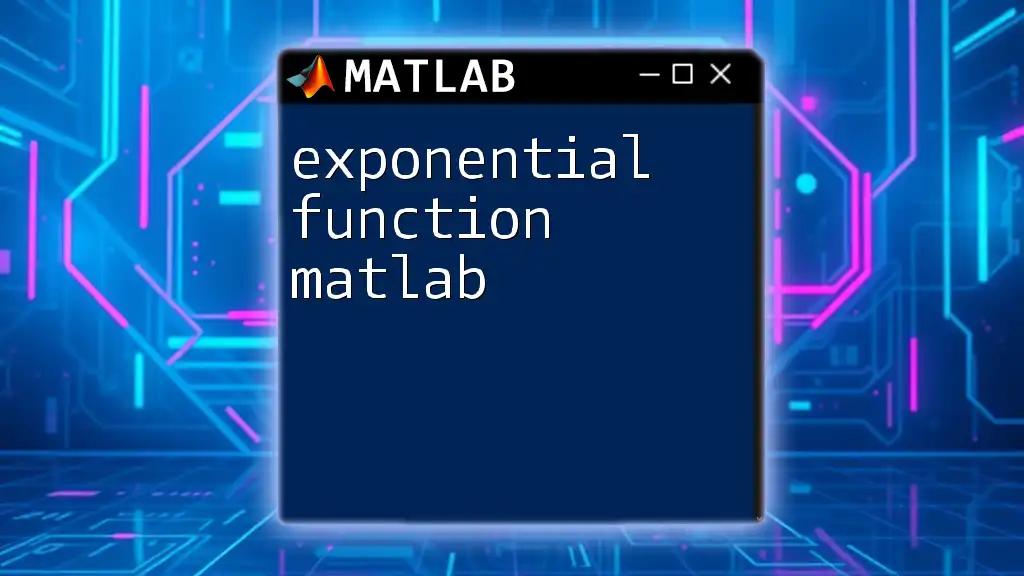
Advanced Options and Customization
Creating Custom Spacing
While linspace is typically used for uniform spacing, there are cases where creating custom intervals is beneficial. By using the parameters effectively, you can still manage to generate points in a specific fashion. For instance, consider this case that demonstrates custom spacing:
custom_points = linspace(1, 10, 5);
This constructs an array of 5 points that explore the range between 1 and 10, showcasing that even within uniform distributions, the method remains flexible and adaptable.
Return Type
The linspace function returns vectors, but it can also be reshaped into matrices, which can be particularly advantageous in multidimensional analysis. For example:
grid_x = linspace(-5, 5, 100);
grid_y = linspace(-5, 5, 100);
[X, Y] = meshgrid(grid_x, grid_y);
This creates 2D grids essential for various mathematical computations, including contour plots and surface graphs.
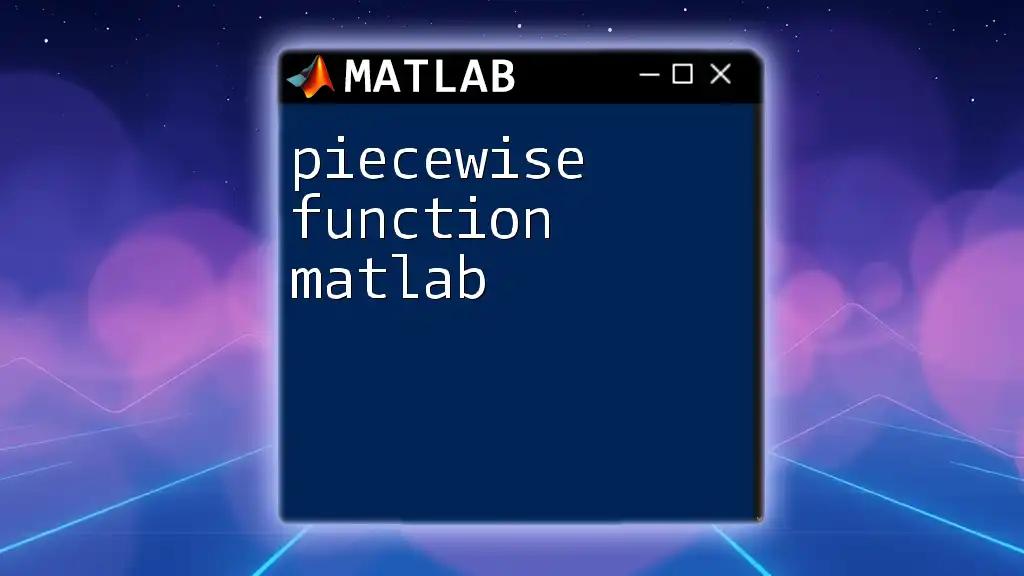
Common Mistakes and Troubleshooting
When using linspace, beginners often run into common pitfalls. One frequent mistake is misjudging the number of points. For example, omitting the third parameter defaults to 100 points, which may not be intended for your analysis, leading to overly dense or sparse output.
Another common issue is confusion between the linspace function and the colon operator. While both can create sequences, the `linspace` function offers more control over the number of points, while the colon operator is limited to defined intervals.
To avoid these mistakes, always verify your outputs by inspecting them visually or analyzing their dimensions and lengths using:
length(vector); % Check the length of your output
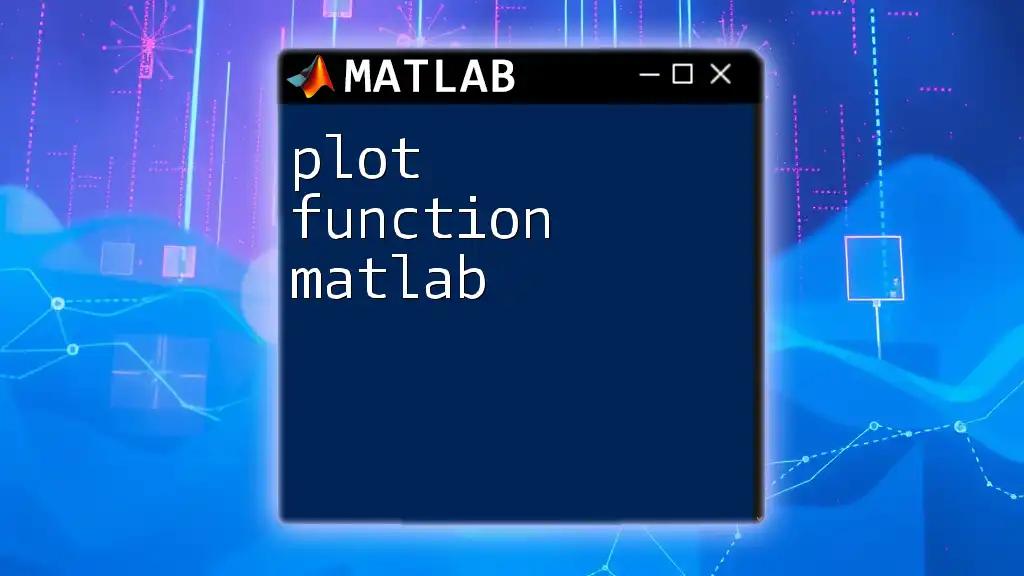
Conclusion
In summary, the linspace function in MATLAB is a powerful tool for generating linearly spaced vectors that enhance data visualization, facilitate engineering calculations, and streamline interpolation tasks. Its flexibility in handling parameters makes it an invaluable asset in both academic and professional contexts. Practice using linspace and experiment with its diverse applications to truly appreciate its functionality and power.
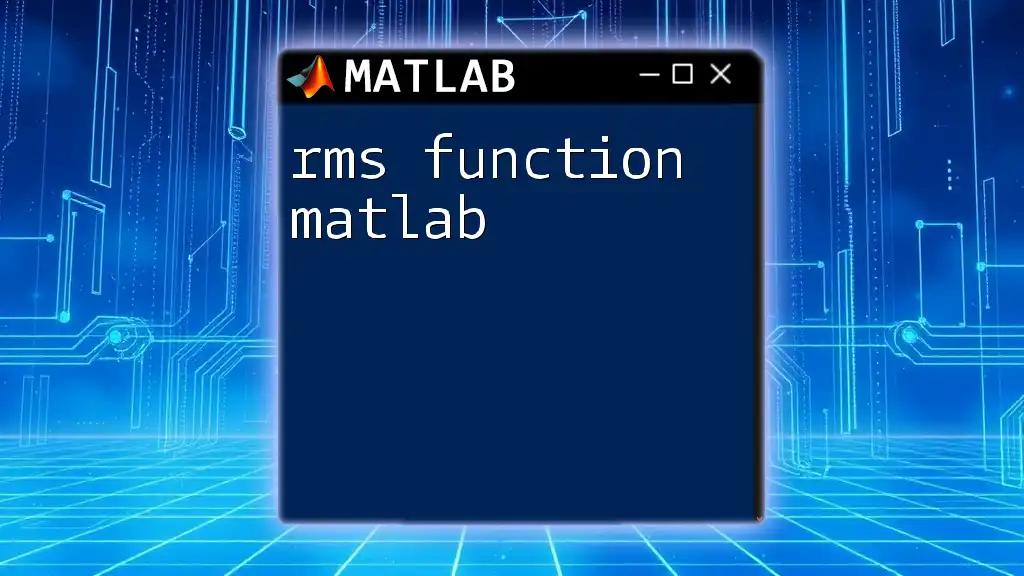
Additional Resources
For further exploration of the linspace function and other MATLAB commands, check out the official MATLAB documentation. Investing in books and online courses can also deepen your understanding and proficiency with MATLAB tools.
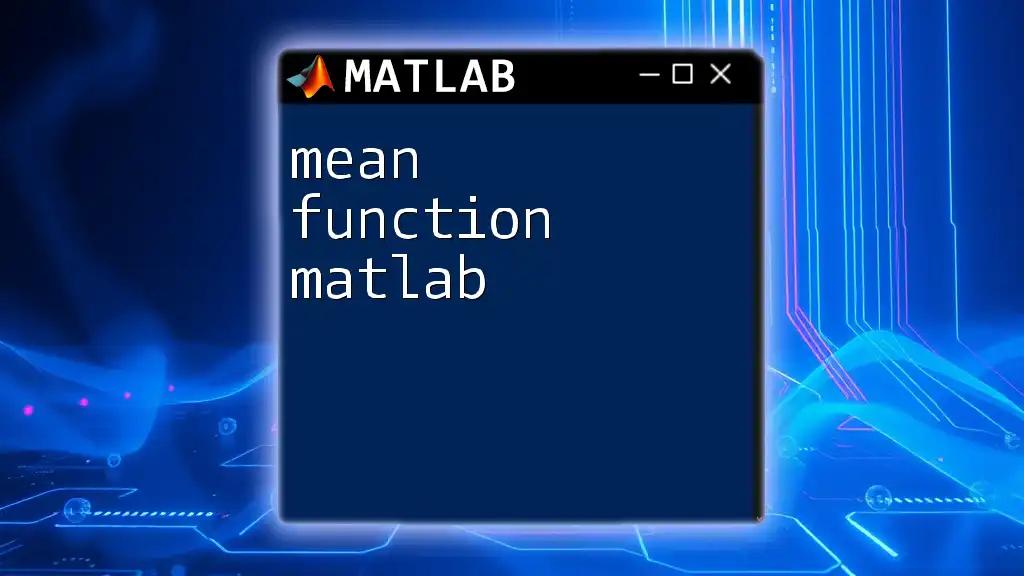
Code Snippets Section
Here are some quick code snippets that illustrate the usage of the linspace function in various contexts:
% Basic Usage
a = linspace(1, 10, 5);
% Generating Points for Plotting
x = linspace(0, 2*pi, 100);
y = cos(x);
% Multi-Dimensional Mesh Grid
[X, Y] = meshgrid(linspace(-1, 1, 10), linspace(-1, 1, 10));
Feel free to adapt and use these snippets as necessary in your projects!