MATLAB primarily uses its own high-level programming language, which is designed specifically for numerical computing, mathematical modeling, and data analysis.
% Example of a simple MATLAB command
x = 0:0.1:10; % Create a vector x from 0 to 10 with increments of 0.1
y = sin(x); % Compute the sine of each element in vector x
plot(x, y); % Plot y versus x
What is MATLAB?
Understanding MATLAB
MATLAB, short for Matrix Laboratory, is a high-level programming environment widely used for numerical computation, algorithm development, and data visualization. It is a powerful tool that provides an interactive environment for performing mathematical computations with matrices, which is essential in numerous scientific and engineering applications.
History of MATLAB
MATLAB was created in the 1980s for use in numerical computing and was originally intended for use as a straightforward interface to FORTRAN libraries. Over the years, it has evolved significantly, incorporating more features that have established it as a comprehensive programming language widely utilized in academia and industry alike.
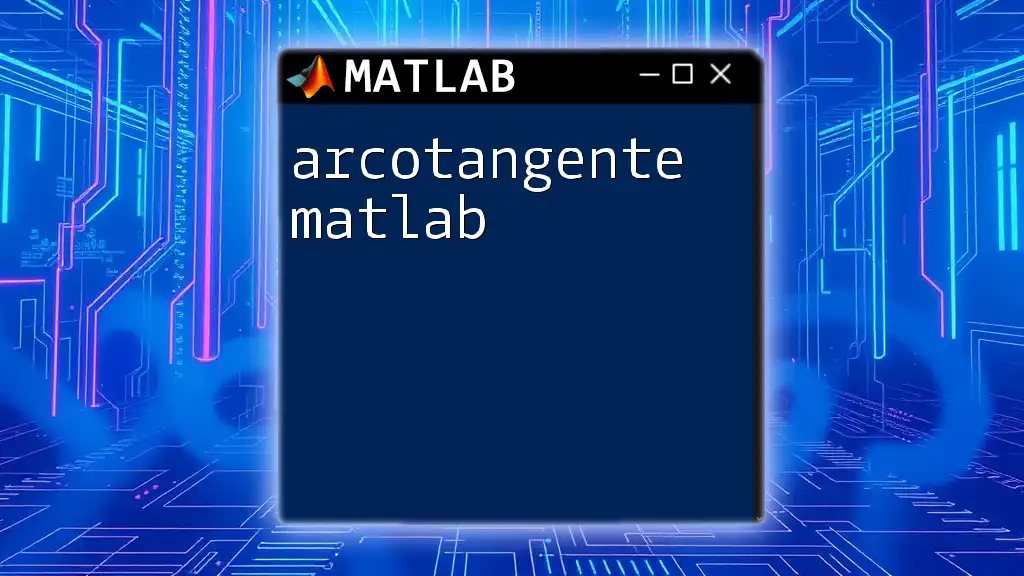
The Core Language of MATLAB
MATLAB Language Overview
The core of MATLAB's functionality is its high-level programming language. This language is designed to allow users to express their ideas in a straightforward and readable manner while managing complex tasks with ease. A hallmark of MATLAB is its interpreted nature, meaning that code can be executed line by line without requiring prior compilation. This feature encourages rapid prototyping and iterative development.
Key Features of MATLAB Language
Interpreted Language
MATLAB is classified as an interpreted language, which means that commands are executed immediately without the need for a compilation step. Benefits of this include:
- Immediate feedback: Users can see the results of their commands and code changes quickly, facilitating an interactive programming style.
- Debugging ease: Errors can be identified and corrected on the fly, streamlining the development process.
Dynamically Typed
In MATLAB, variables do not need to be explicitly declared with a data type. Instead, MATLAB uses dynamic typing, which automatically determines the type of variable at runtime. This allows for more flexibility in coding:
- For example, if you assign a value to a variable, you can later change it to a different type without any issues.
Multi-Paradigm
MATLAB supports multiple programming paradigms, including: Procedural programming: Focusing on writing procedures or routines. Object-oriented programming: Enabling users to define their data types. Functional programming: Allowing functions to be treated as first-class citizens. This versatility makes MATLAB suitable for a diverse range of applications.
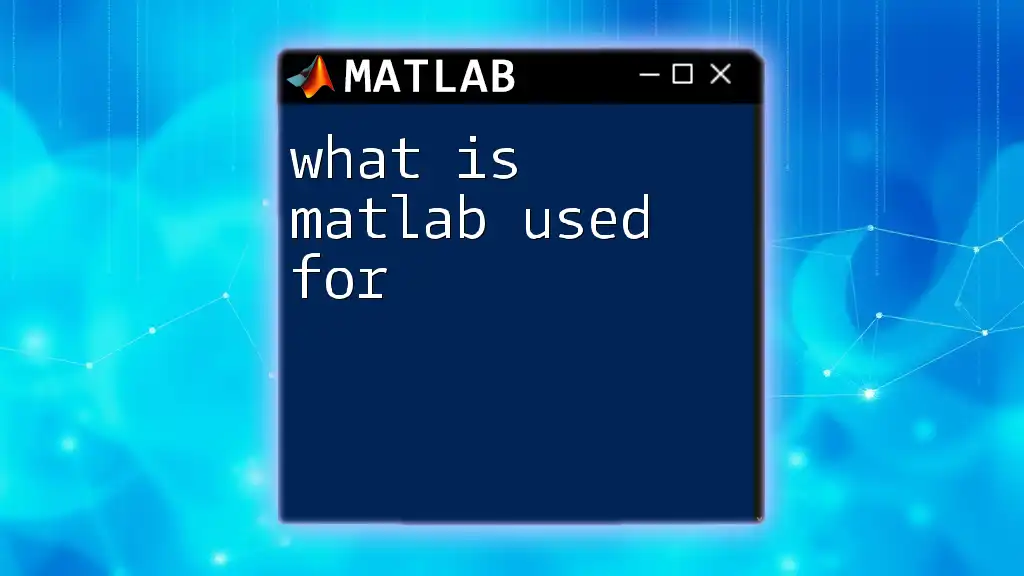
Syntax and Commands in MATLAB
Basic Syntax
Understanding the basic syntax of MATLAB is crucial for effective coding. MATLAB is case-sensitive, meaning that `Variable` and `variable` are recognized as distinct identifiers. Commands usually end with a new line and do not require semicolons to terminate. An essential feature is that if a command is entered without a semicolon, MATLAB outputs the result to the command window.
For example:
x = 5; % Assigning a value to a variable
Common Commands
To get started with MATLAB, familiarize yourself with a few common commands:
- `clc`: Clears the command window.
- `clear`: Removes all variables from memory, freeing up space.
- `plot`: Generates plots for visualizing data.
Here’s an example of a simple program demonstrating these commands:
x = 0:0.1:10; % Create an array of values
y = sin(x); % Calculate sine values
plot(x, y); % Plot the graph
This code snippet creates a series of values, computes their sine, and produces a plot.
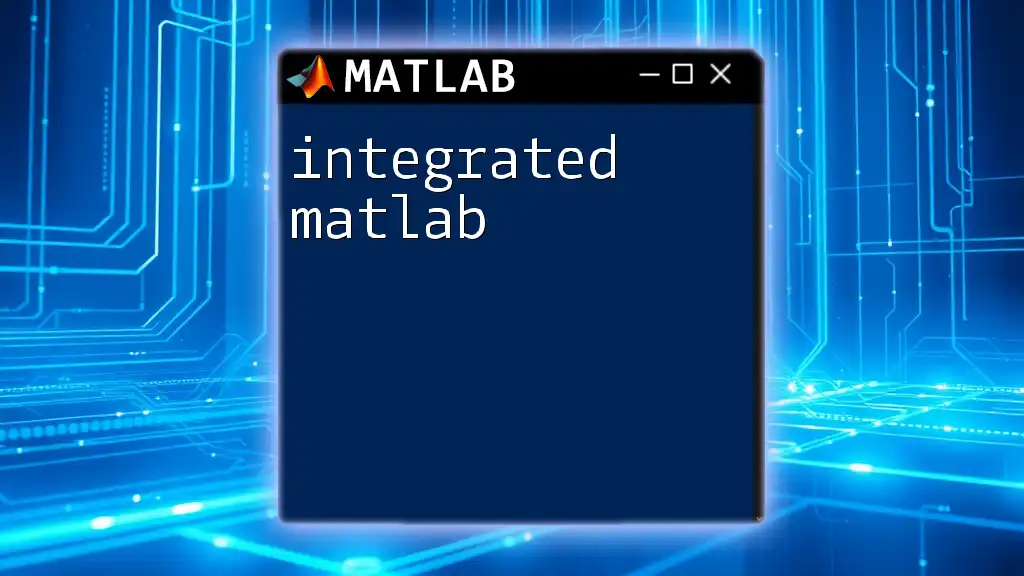
MATLAB Programming Constructs
Variables and Data Types
MATLAB supports various data types, including:
- Arrays and Matrices: Fundamental units, as MATLAB is short for "Matrix Laboratory".
- Strings: Handle text and characters.
- Structures and Cells: Allow more complex data structures.
Creating variables in MATLAB is intuitive:
A = [1, 2, 3; 4, 5, 6]; % A 2D matrix
B = 'Hello, MATLAB!'; % A string variable
Control Statements
Control flow is essential in programming. MATLAB includes several control statements such as `if`, `for`, and `while`. Here’s an example demonstrating a for loop:
for i = 1:5
disp(i); % Display numbers from 1 to 5
end
This loop prints the numbers from 1 to 5 in the command window.
Functions in MATLAB
Functions are critical for writing modular and reusable code. They allow for encapsulating logic that can be invoked as needed. An example of a simple function is as follows:
function area = circleArea(radius)
area = pi * radius^2; % Calculate area of a circle
end
This function takes a radius as input and returns the area of a circle, demonstrating MATLAB’s capability for mathematical computations.
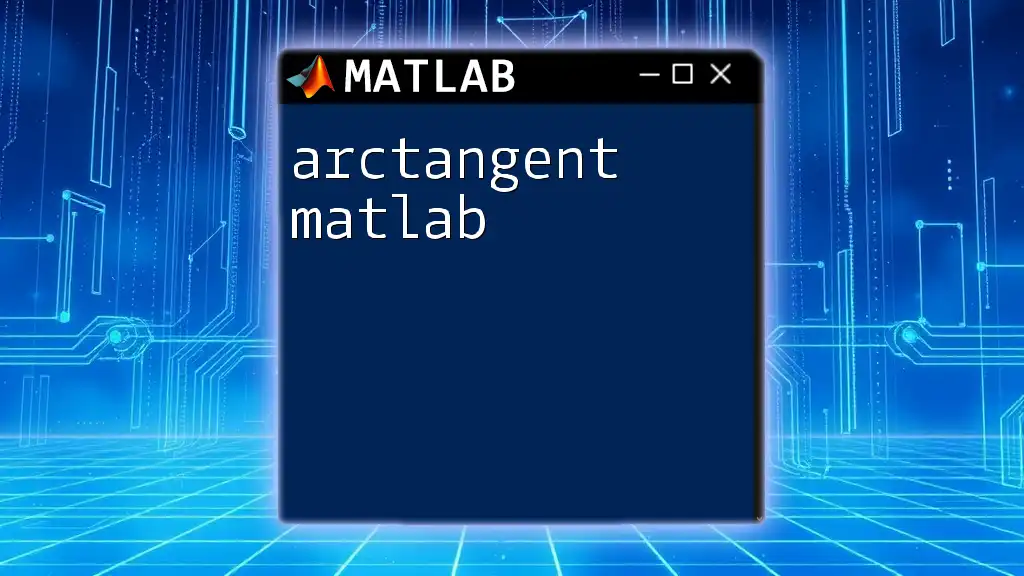
Advanced Features of the MATLAB Language
Object-Oriented Programming
MATLAB supports Object-Oriented Programming (OOP), enabling users to create classes and objects. OOP can make code more organized and manageable. Here is an example of defining a class in MATLAB:
classdef Circle
properties
Radius
end
methods
function obj = Circle(r)
obj.Radius = r;
end
function area = Area(obj)
area = pi * obj.Radius^2; % Calculate area of a circle
end
end
end
With this class, one can create circle objects and compute their area easily.
Integration with Other Languages
Another powerful feature of MATLAB is its ability to interface with other programming languages, such as C, C++, and Python. This integration allows MATLAB users to leverage compiled code for performance-intensive tasks or to use existing libraries. Benefits of such integration include:
- Efficiency: It improves execution speed for specific compute-heavy functions.
- Access to vast libraries: Users can utilize functionalities from languages with extensive libraries.
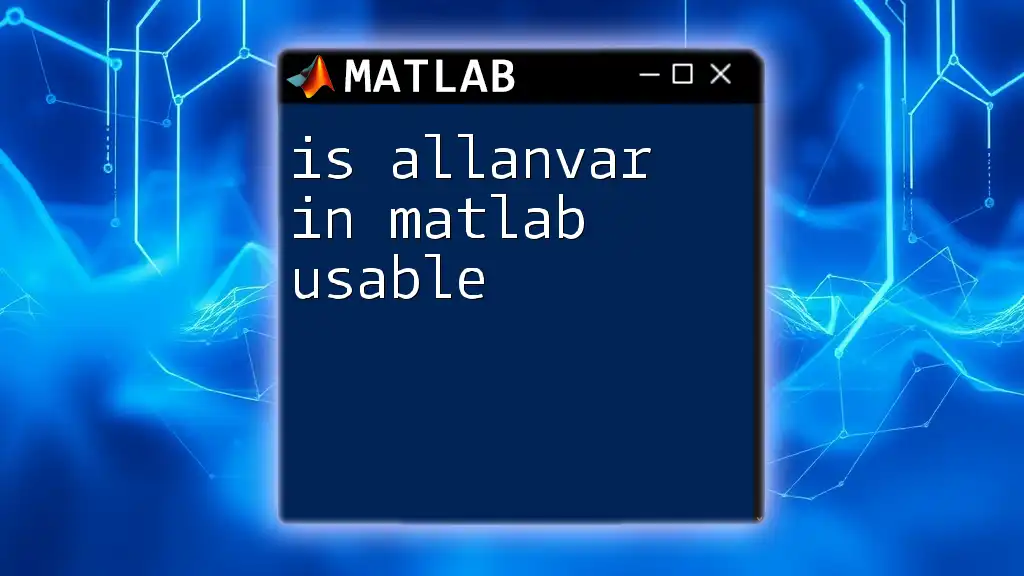
Conclusion
In summary, MATLAB uses a high-level language designed for easy numerical computing and data visualization. Understanding its core features—including being an interpreted and dynamically typed language—enables users to write effective, concise code for various applications. Whether engaging in simple calculations or complex data analysis, mastering MATLAB’s programming constructs and advanced capabilities will empower you to harness the full potential of this versatile tool.
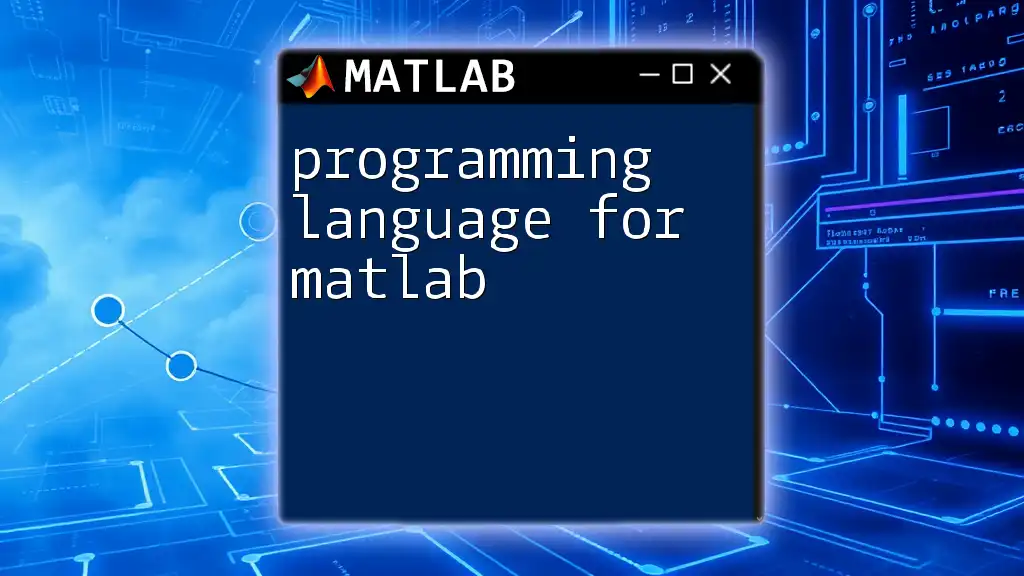
Call to Action
If you're eager to learn more about MATLAB and improve your coding skills quickly and effectively, consider subscribing to our resources or joining our upcoming courses. Let’s unlock the power of MATLAB together!