Downsampling in MATLAB involves reducing the number of samples in a signal by selecting every nth sample, which can be efficiently achieved using the `downsample` function.
Here's a quick code snippet demonstrating how to downsample a signal by a factor of 2:
% Original signal
t = 0:0.01:1; % Time vector
x = sin(2*pi*5*t); % Example signal
% Downsampling the signal
downsampled_x = downsample(x, 2); % Downsample by a factor of 2
What is Downsampling?
Downsampling refers to the process of reducing the sample rate of a signal, which results in a lower resolution version of the data. This technique is crucial in various fields, such as signal processing, image processing, and data compression, where managing the size and quality of data is essential.
By reducing the number of samples, downsampling can help in minimizing computational load, accelerating processing times, and conserving storage space. However, it is pertinent to understand that downsampling can introduce unwanted artifacts if not executed properly.
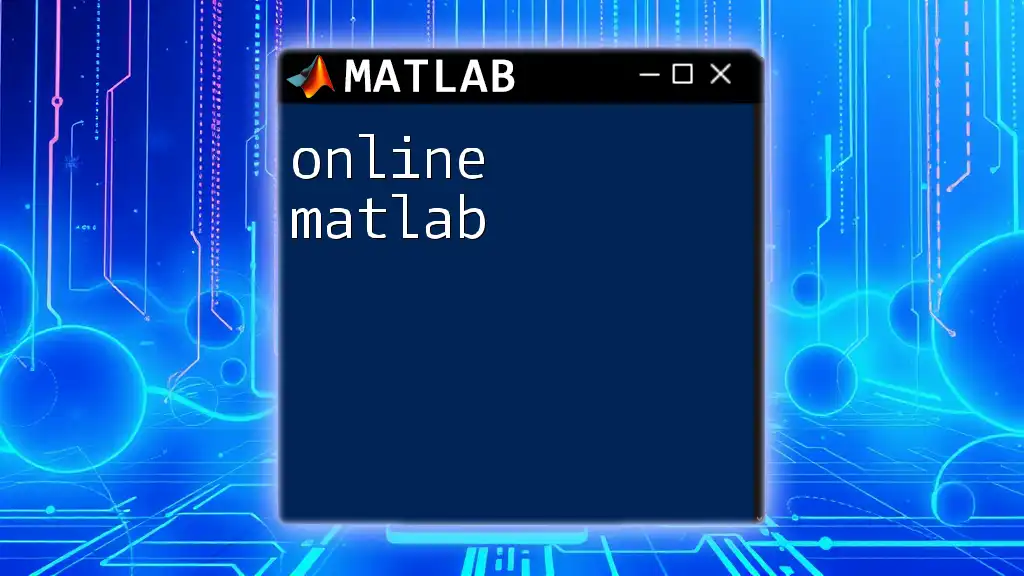
Why Use MATLAB for Downsampling?
MATLAB is a powerful tool for engineers and researchers, offering a wide range of built-in functions for data manipulation and analysis. Its high-level programming environment and extensive libraries make it an ideal choice for implementing downsampling techniques. Here are a few compelling reasons to use MATLAB for downsampling:
- Ease of Use: MATLAB’s syntax is designed for mathematical computations, making it user-friendly.
- Visualization: It provides excellent plotting capabilities, allowing you to visualize data before and after downsampling.
- Performance: With optimized functions and rich toolboxes, MATLAB efficiently handles large datasets.
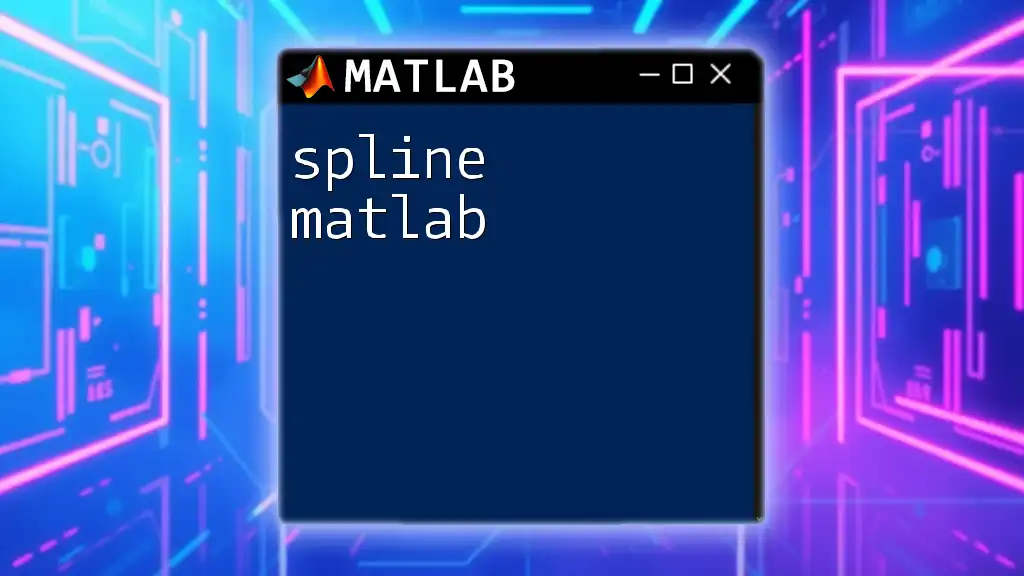
Understanding the Basics of Downsampling
The Concept of Sampling Rate
Sampling rate, measured in samples per second (Hz), dictates how frequently data points are collected from a continuous signal. The importance of the sampling rate is highlighted by the Nyquist theorem, which states that to accurately reconstruct a signal, it must be sampled at least twice its highest frequency component. If you downsample without considering this theorem, you may introduce aliasing, which distorts the signal.
Downsampling Techniques
Two primary techniques for downsampling include:
-
Decimation: Involves removing samples from the original signal at regular intervals. This often comes with the added step of low-pass filtering to prevent aliasing.
-
Simple Downsampling: Just reduces the number of samples without additional processing, potentially leading to issues such as aliasing if not done cautiously.
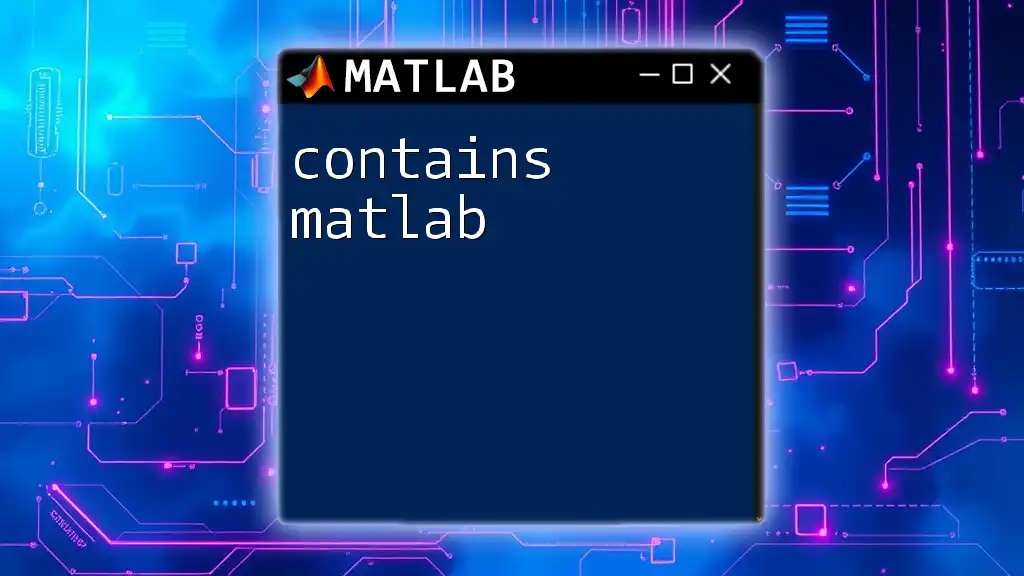
Methods for Downsampling in MATLAB
Using the `downsample` Function
The `downsample` function in MATLAB is straightforward for reducing the number of samples in a signal. It takes two arguments: the input signal and the downsampling factor.
Syntax and Parameters
y = downsample(x, factor)
Here, `x` is the input signal, and `factor` indicates the downsampling rate (e.g., a factor of 5 means retaining every 5th sample).
Example Code Snippet
% Example of downsampling a signal
fs = 1000; % Original sampling frequency
t = 0:1/fs:1; % Time vector
x = sin(2*pi*50*t) + randn(size(t)); % Signal with noise
% Downsample by a factor of 5
factor = 5;
y = downsample(x, factor);
Explanation of Example
In this example, a noisy sine wave signal is created with an original sampling frequency of 1000 Hz. By downsampling it using a factor of 5, the resulting signal `y` retains every 5th sample. This reduces the data size while maintaining a representation of the signal.
Using the `decimate` Function
MATLAB’s `decimate` function is another powerful option that combines downsampling and low-pass filtering to mitigate aliasing effects.
Syntax and Parameters
y = decimate(x, factor)
Similar to `downsample`, `decimate` takes an input signal and a downsampling factor but automatically applies a low-pass filter.
Example Code Snippet
% Example of decimating a signal
fs = 1000; % Original sampling frequency
f = 50; % Frequency of the signal
t = 0:1/fs:1; % Time vector
x = sin(2*pi*f*t); % Original signal
% Decimate by a factor of 5
y = decimate(x, factor);
Explanation of Example
Here, a pure sine wave is generated, and `decimate` is applied with a factor of 5. The function effectively filters the signal to eliminate higher frequency components that could lead to aliasing, making it a preferred method in signal processing.
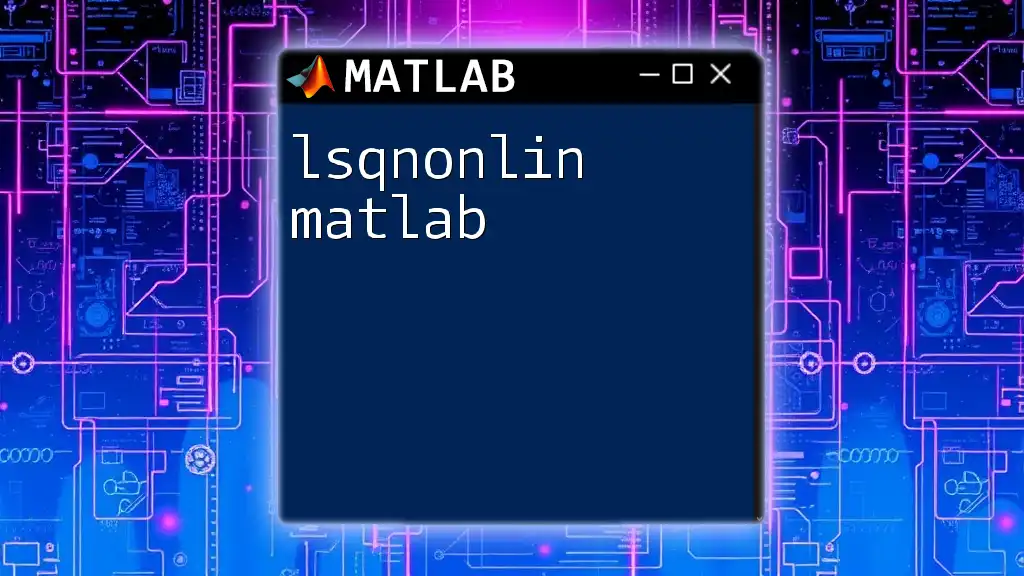
Visualizing Downsampled Data
Visualizing the original and downsampled signals can provide valuable insights into the effects of downsampling. This step is crucial to understand how well the key characteristics of the original signal are retained.
Example Code Snippet
% Plot original and downsampled signals
figure;
subplot(2,1,1);
plot(t, x); % Original signal
title('Original Signal');
subplot(2,1,2);
t_downsampled = downsample(t, factor); % Adjust time vector for downsampled signal
plot(t_downsampled, y); % Downsampled signal
title('Downsampled Signal');
Explanation of Visualization Choices
In this plot, the original signal appears in the top subplot, while the downsampled signal is in the bottom subplot. Observing the two together allows visualization of how the downsampling process has maintained the essential features of the original wave while reducing data complexity.
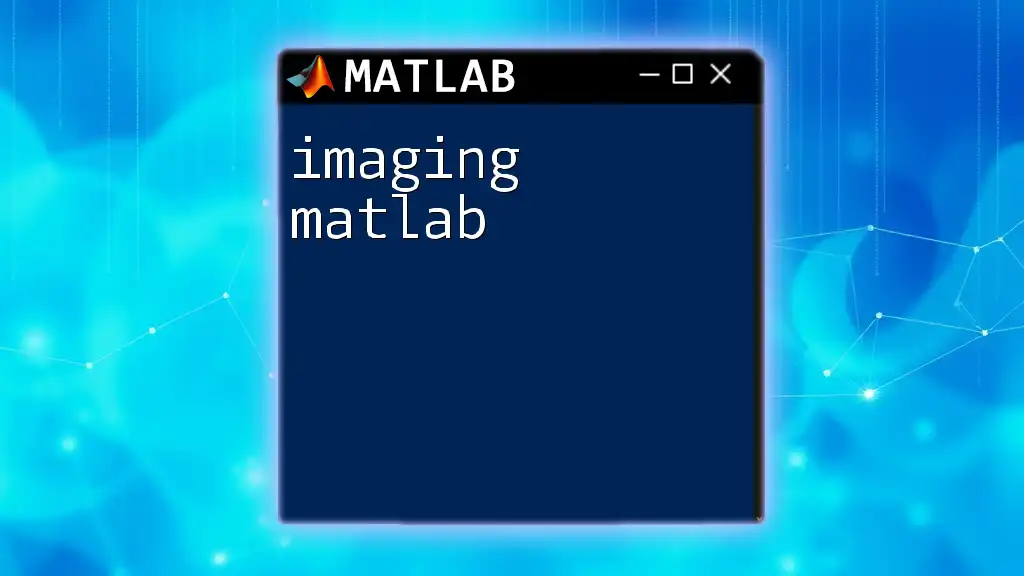
Practical Applications of Downsampling
Signal Processing and Audio Engineering
In audio applications, downsampling reduces the bitrate of recordings, making them easier to manage and store without sacrificing discernable sound quality. For instance, an audio file can be converted from a high sampling rate (e.g., 192 kHz) to a standard CD quality rate (44.1 kHz).
Image Processing
Downsampling plays a crucial role in image processing, particularly in applications where data size must be minimized. For example, when resizing images for web use, downsampling can reduce storage and increase loading speed without a significant loss in perceptual quality.
Machine Learning and Data Reduction
In machine learning, downsampling can help manage large datasets by reducing the number of samples, thereby speeding up model training times. A smaller but representative subset of the data can often yield similar results while improving computational efficiency.
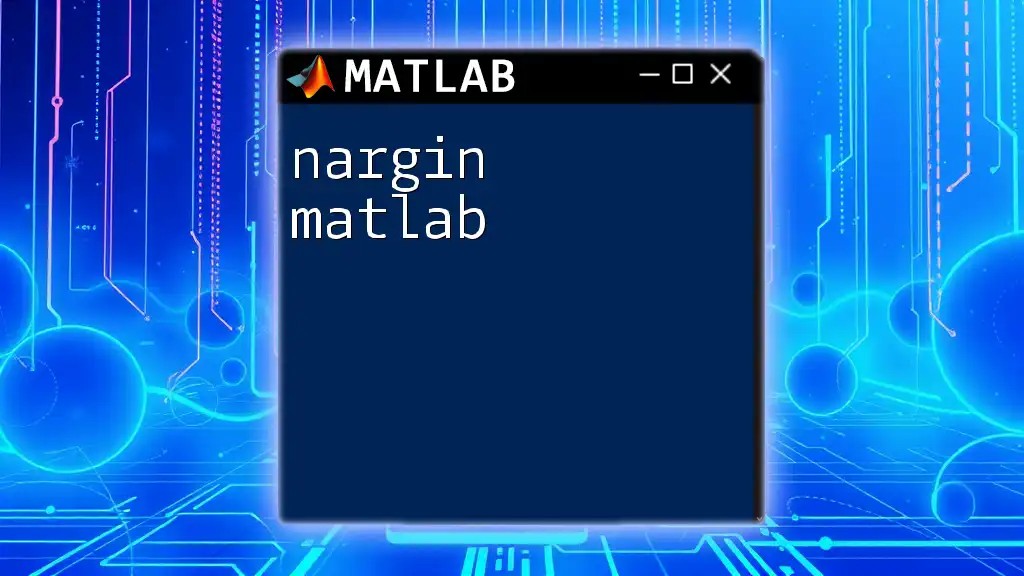
Common Pitfalls and Best Practices
Avoiding Aliasing
Aliasing occurs when higher frequency components are misrepresented after downsampling. To avoid this, it’s essential to apply a low-pass filter before downsampling, especially if the signal contains frequencies higher than half the new sampling rate.
Choosing the Right Downsampling Factor
Selecting an appropriate downsampling factor is crucial for maintaining the integrity of the data. If the factor chosen is too large, important information could be lost. A good practice is to experiment with different factors while monitoring the signal’s key characteristics.
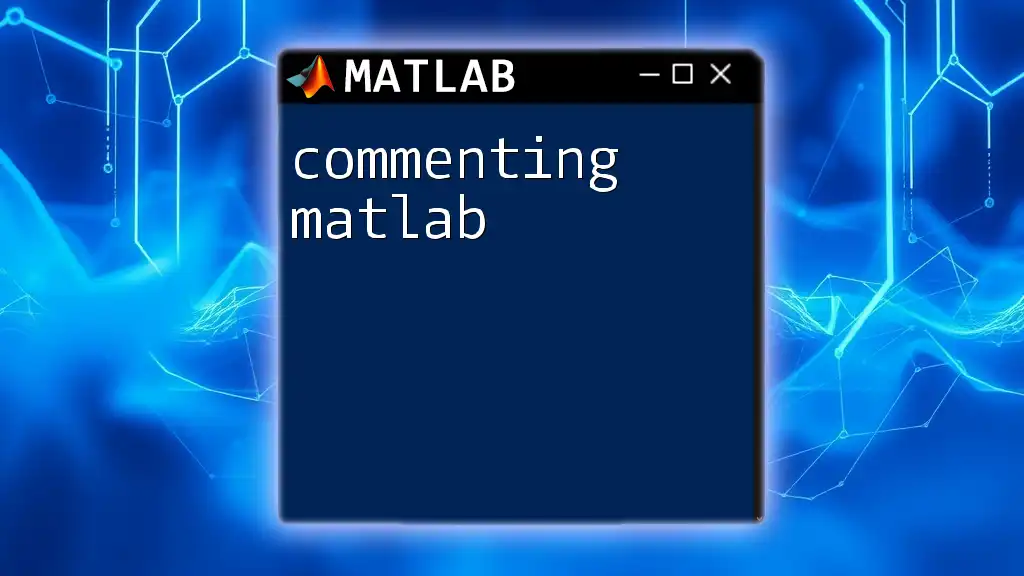
Summary
In summary, downsampling in MATLAB is a straightforward yet powerful process that enables efficient data management across various applications. Understanding the functions `downsample` and `decimate`, alongside the visual representation of data, will help you leverage the full potential of downsampling.
By keeping in mind the importance of avoiding aliasing and selecting optimal downsampling factors, you can harness downsampling effectively in your MATLAB projects.
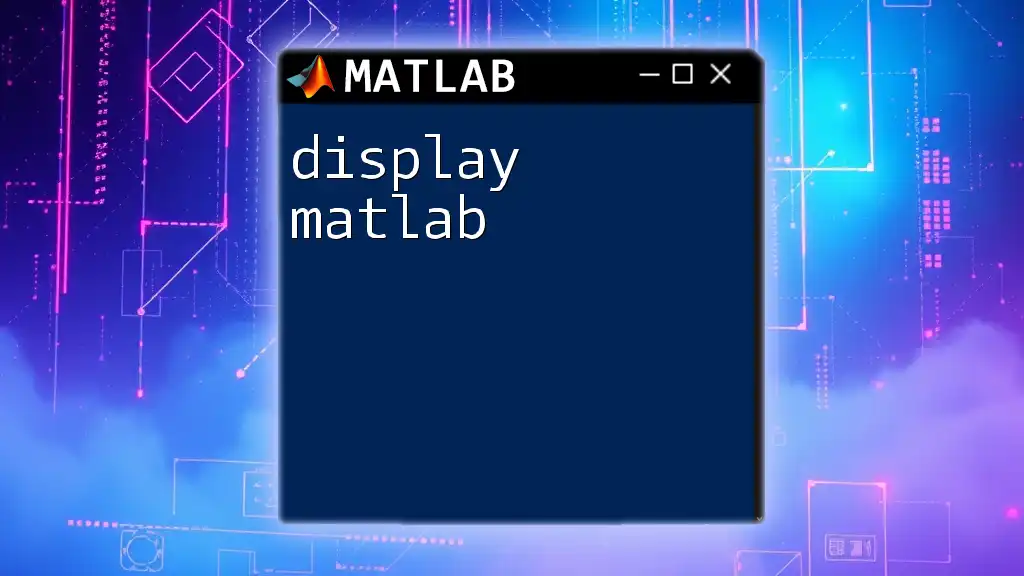
Additional Resources
For those looking to deepen their knowledge of downsampling in MATLAB, consider exploring the following:
- Official MATLAB Documentation: Get detailed information about the functions and their use cases.
- Online Tutorials and Courses: Engage with content dedicated to MATLAB and data processing techniques.
- Community Forums for MATLAB Users: Collaborate and learn from other MATLAB enthusiasts, sharing insights and solutions to challenges.
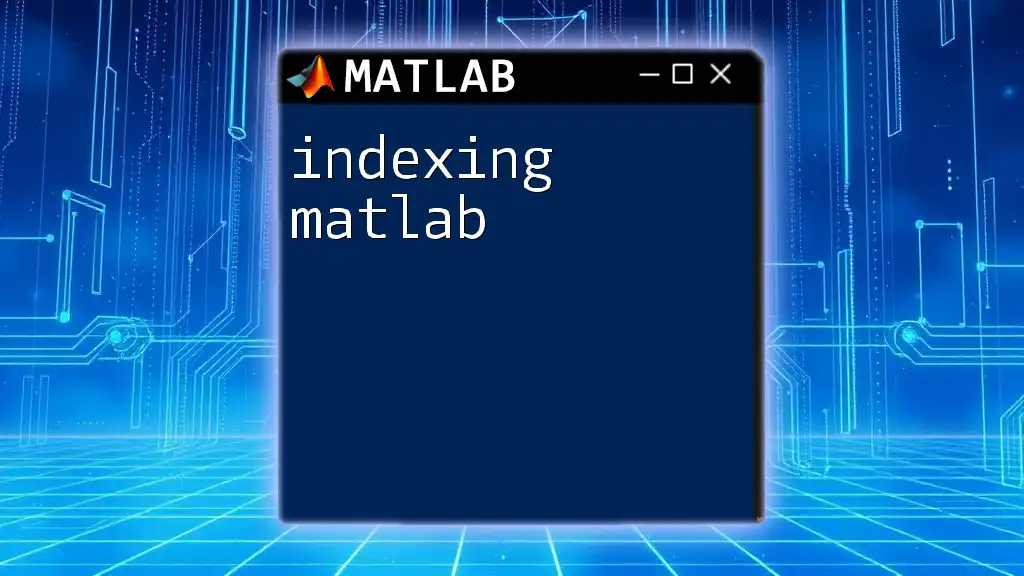
Conclusion
Now that you have a comprehensive understanding of downsampling in MATLAB, it’s time to put this knowledge into practice. Begin experimenting with the provided examples and refine your skills as you explore more complex datasets and applications. Your journey into the world of MATLAB downsampling has just begun!