The `audioread` function in MATLAB allows you to read audio files into a matrix for further processing or analysis. Here's a simple example:
[y, fs] = audioread('example.wav');
Understanding Audio Data in MATLAB
Different Audio File Formats
MATLAB's `audioread` function supports various audio file formats, allowing for flexibility in audio processing tasks. Some of the most commonly used formats include:
- WAV: A lossless format that preserves audio quality and is ideal for analysis.
- MP3: A lossy format that compresses audio data, suitable for smaller file sizes but may sacrifice some quality.
- FLAC: A lossless compression format that retains high quality while reducing file size.
Choosing the correct format is crucial depending on your project requirements, such as sound quality, file size, and compatibility with other software.
Sampling Rates and Bit Depth
Sampling rate refers to how many samples of audio are taken per second. A higher sampling rate results in better audio quality but also larger file sizes. Common sampling rates include:
- 44.1 kHz: Standard for CDs.
- 48 kHz: Often used in video production.
- 96 kHz and above: Utilized in professional audio applications.
Bit depth affects the dynamic range of audio; commonly used depths are 16-bit and 24-bit. A greater bit depth enhances the audio's fidelity, allowing for a more extensive range of loudness levels.
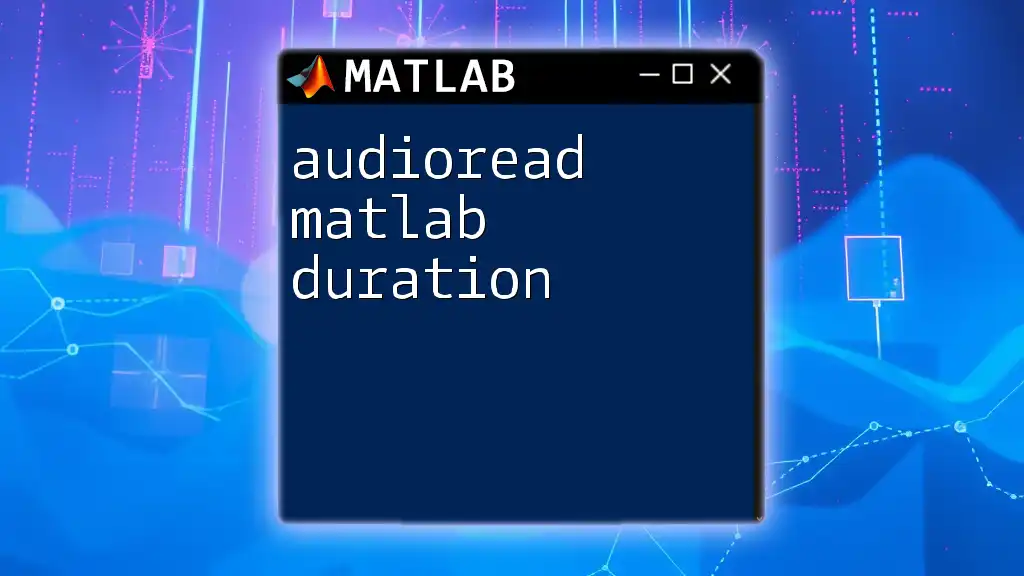
Basic Syntax of audioread
Function Signature
The basic syntax of the `audioread` function is:
y = audioread(filename)
- Here, `filename` is a string that specifies the name of the audio file you want to read.
- `y` will store the audio data, which is returned as a matrix.
Additional Parameters
`audioread` allows for additional input parameters such as start and end samples. This is particularly useful for large files where you may want to load only a section of the audio.
The syntax for this functionality is as follows:
y = audioread(filename, [startSample endSample])
Example
To read the entire file:
[audioData, sampleRate] = audioread('example.wav'); % Read entire file
To read only the first 100,000 samples:
[audioPartial, sampleRate] = audioread('example.wav', [1 100000]); % Read first 100000 samples
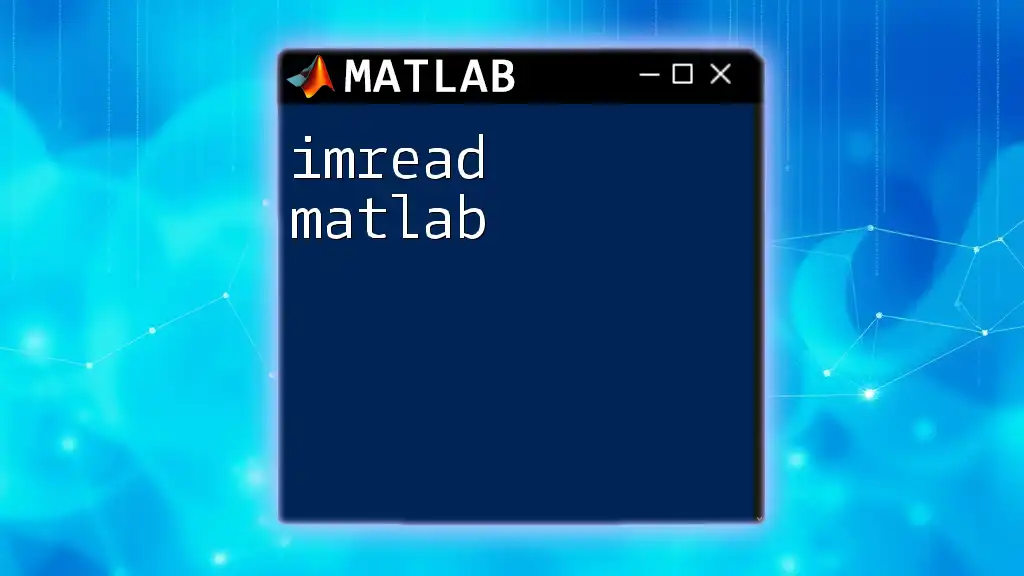
Practical Examples of Using audioread
Loading Audio Files
Loading an audio file is straightforward. You can specify the file format, and MATLAB will handle the rest. An example code snippet is shown below:
[audioData, sampleRate] = audioread('audiofile.mp3');
disp(['Sample Rate: ', num2str(sampleRate)]);
This code reads an MP3 file named `audiofile.mp3` and displays its sample rate.
Visualizing Audio Data
Visualizing audio data can provide insights into the waveform and help in understanding the audio's characteristics. MATLAB includes the `plot` function for this purpose. Here's an example:
time = (0:length(audioData)-1) / sampleRate; % Time vector
plot(time, audioData);
xlabel('Time (s)');
ylabel('Amplitude');
title('Audio Signal Waveform');
Playing Audio Files
You can play the loaded audio using the `sound` function. Here’s how:
sound(audioData, sampleRate);
This command will play your audio file according to the specified sample rate.
Extracting Specific Channels
When dealing with stereo audio files, you may want to analyze each channel separately. You can easily extract the left and right channels as follows:
leftChannel = audioData(:,1); % Left channel
rightChannel = audioData(:,2); % Right channel
This way, you can process or analyze each channel independently.
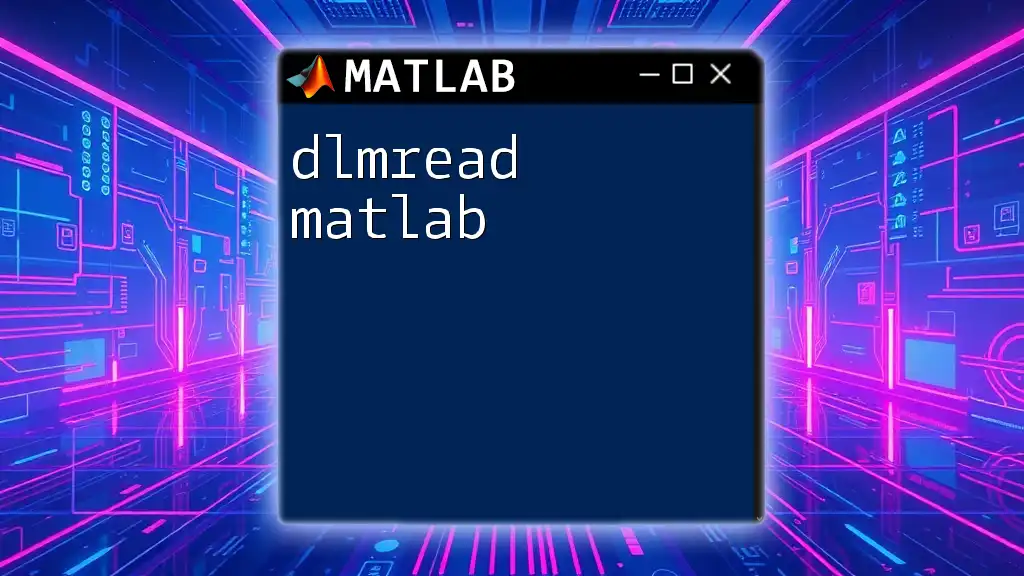
Advanced Usage of audioread
Reading Portions of a File
In practical applications, especially when dealing with lengthy audio files, you might only need a specific segment of the audio. Here’s how to achieve this:
y = audioread('largefile.wav', [fs*10 fs*20]); % Read from 10 to 20 seconds
The above command allows you to load audio data from the 10-second mark to the 20-second mark.
Working with Multichannel Audio
In scenarios involving multichannel audio files (like surround sound), `audioread` efficiently loads all channels into a matrix. Each column of the matrix corresponds to a channel. Here’s an example of how to manipulate these channels:
[audioData, sampleRate] = audioread('multichannelfile.wav');
channel1 = audioData(:, 1); % First channel
channel2 = audioData(:, 2); % Second channel
% You can continue analyzing or processing channels as needed.
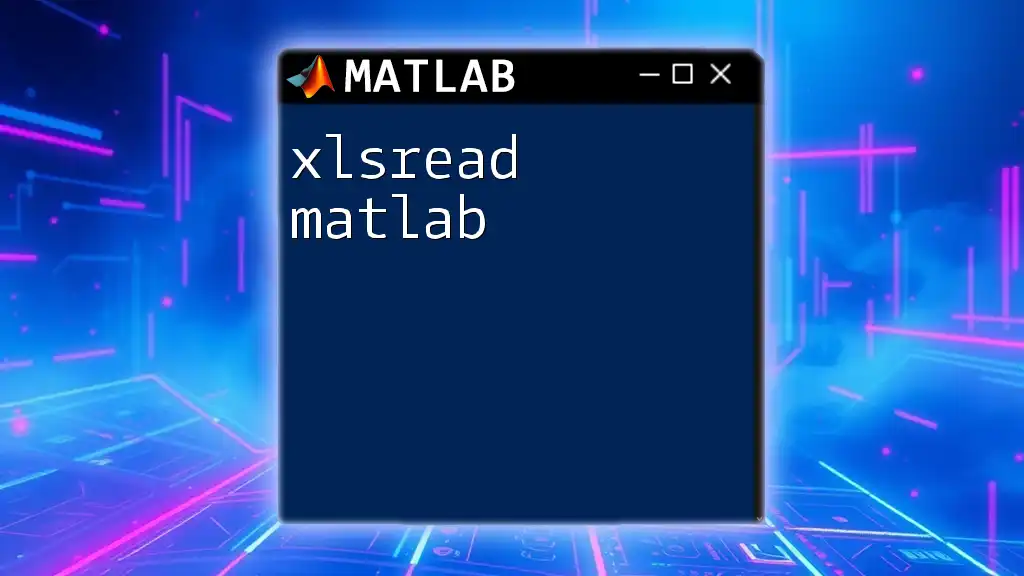
Common Issues and Troubleshooting
File Format Unsupported
If you encounter an "unsupported format" error, consider converting your audio files to a compatible format using audio editing software or online converters. Tools like Audacity can help re-encode your files.
Incorrect Sampling Rates
Playing audio may not work correctly if the sample rate of the audio file does not match the playback device's capabilities. Ensure to check the sample rate and adjust it if necessary.
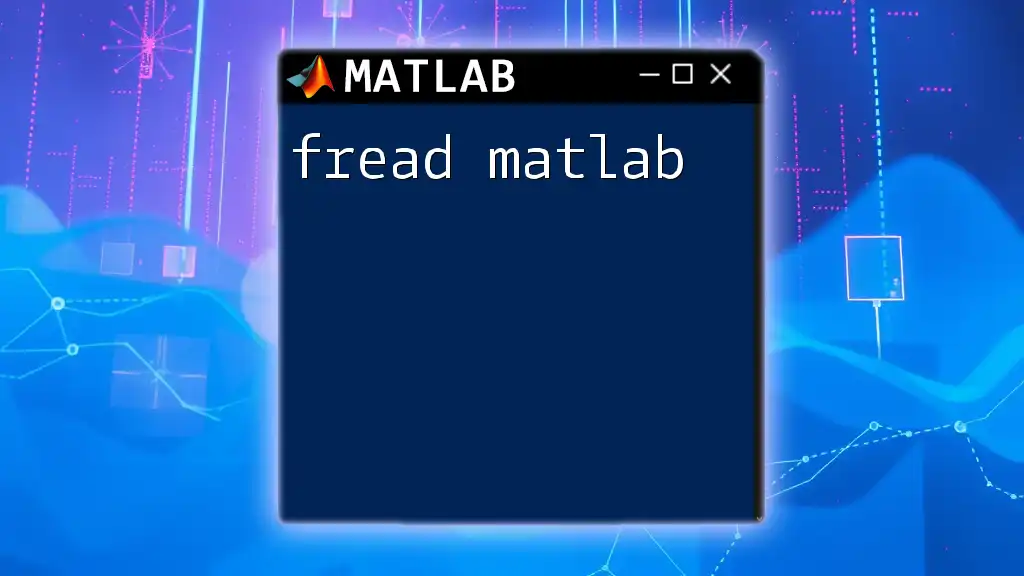
Conclusion
The `audioread` function in MATLAB is a potent tool for anyone looking to engage with audio data. It simplifies the process of loading, manipulating, and analyzing audio files of various formats. By mastering this function, you pave the way for deeper explorations into audio processing, analysis, and potential applications in fields such as machine learning and music technology.
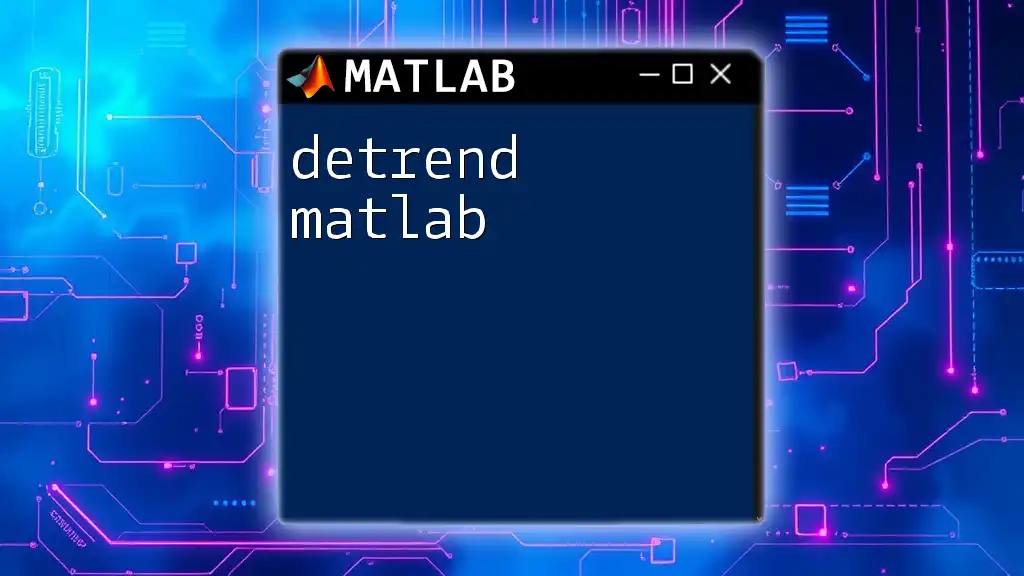
Additional Resources
For further learning, consider accessing the official MATLAB documentation for audioread. Also, explore additional functions in MATLAB related to audio processing, such as `audiowrite` for saving audio data and `fft` for spectral analysis.