A cell array in MATLAB is a versatile data structure that allows you to store different types of data in each cell and is particularly useful for holding collections of various sizes or types.
% Creating a cell array
myCellArray = {1, 'Hello', [1, 2, 3]; true, {4, 5}, 'World'};
Understanding Cell Arrays in MATLAB
What is a Cell Array?
A cell array in MATLAB is a versatile data structure that allows you to store arrays of different types and sizes in a single variable. Unlike regular arrays, which can only hold one data type (such as all numeric or all strings), cell arrays can hold a mix of data types. This flexibility makes cell arrays incredibly useful for organizing complex data sets.
For example, a single cell array can contain numeric values, character strings, or even other cell arrays. This capability is a significant advantage when working with heterogeneous data.
When to Use Cell Arrays
You should consider using cell arrays when you need to handle variable data sizes or types. Some common use cases include:
- Storing text data: When you have strings of varying lengths or formats.
- Collecting data of different types: For instance, when combining numbers, strings, and even other arrays or structures.
- Passing multiple outputs from functions: Functions can return multiple outputs in the form of cell arrays, which simplifies data organization.
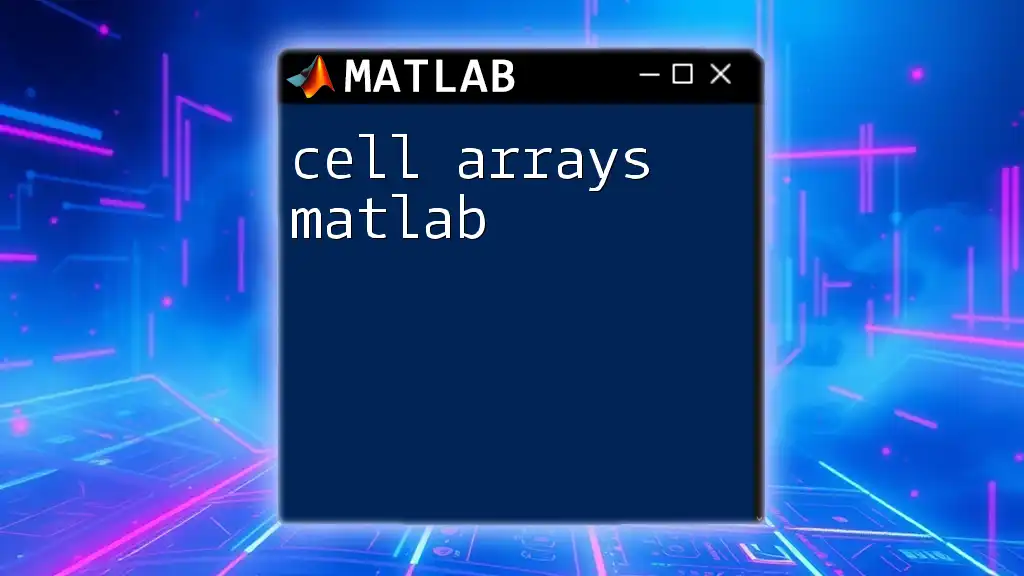
Creating Cell Arrays
Syntax for Creating a Cell Array
Creating a cell array is simple. You use curly braces `{}` to define it. Here’s the basic syntax to create a cell array:
myCellArray = {1, 'text', [1, 2, 3]};
In this example, `myCellArray` contains an integer, a string, and a numeric array. Each element can be accessed or modified independently due to the cell array's structure.
Preallocating Cell Arrays for Efficiency
To enhance performance, especially when dealing with large datasets, preallocate your cell arrays. Preallocating a cell array assigns memory in advance, which reduces the time taken to dynamically resize the array later.
Here’s how to preallocate an empty cell array:
myCellArray = cell(1, 5); % Creates a 1-by-5 cell array
This command prepares a cell array with five empty placeholders, allowing you to fill them at a later stage without overhead costs from repeated resizing.
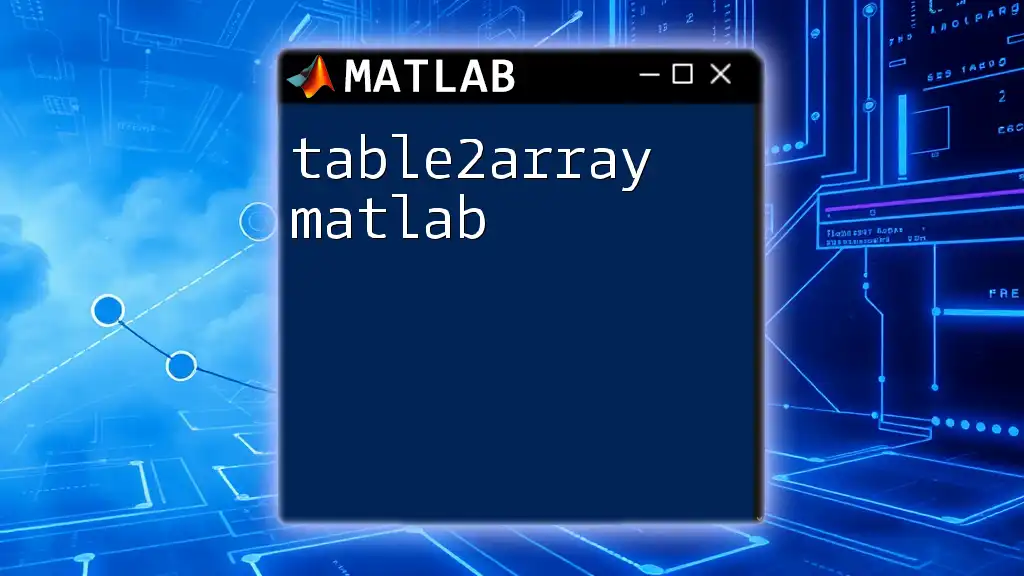
Accessing Data in Cell Arrays
Indexing Cell Arrays
Accessing elements in a cell array is performed using curly braces. Each element can be indexed just like elements in standard arrays.
For instance, to access a single element of your cell array, you might use:
element = myCellArray{2}; % Accessing the second element
This command retrieves the second element stored in `myCellArray`.
Modifying Elements in Cell Arrays
You can also modify elements within a cell array easily. Each element can be reassigned to a new value, which can either be a scalar, a string, or even another cell array.
Here's an example of updating an existing element:
myCellArray{1} = {10, 20}; % Updating the first element to a new cell array
In this case, the first element of `myCellArray` now contains another cell array with the numbers 10 and 20.
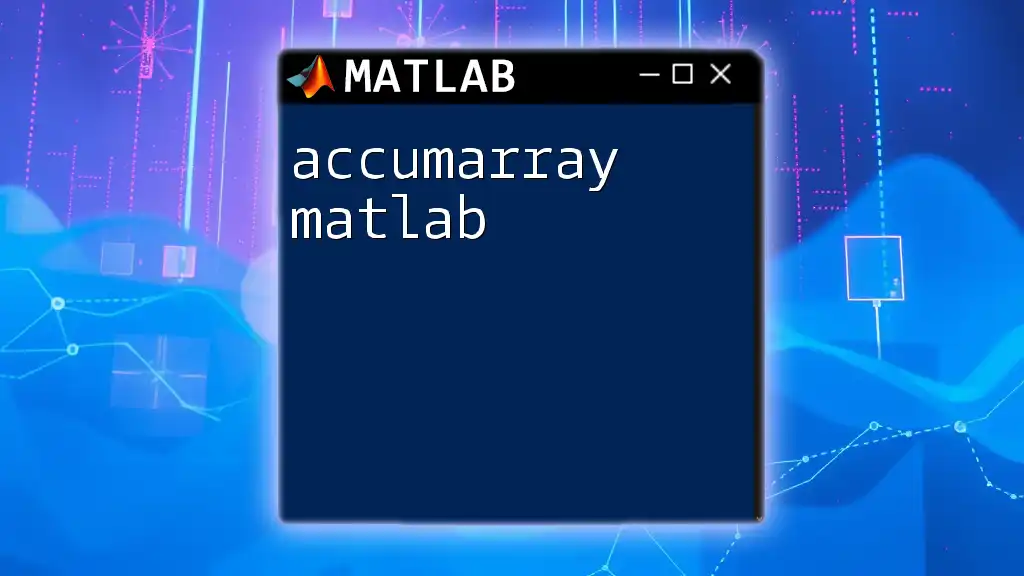
Working with Cell Array Functions
Key Functions in MATLAB for Cell Arrays
MATLAB provides several functions specifically designed for working with cell arrays. Some essential functions include:
- `cellfun`: Applies a function to each cell in a cell array, returning the results in a new cell array.
- `num2cell`: Converts numeric arrays into cell arrays.
- `cell2mat`: Converts a cell array into a regular matrix.
Using `cellfun` can streamline your calculations. Here’s a straightforward example that doubles the values of each element in a cell array:
myCellArray = {1, 2, 3};
doubled = cellfun(@(x) x*2, myCellArray, 'UniformOutput', false);
In this example, `doubled` now contains the values {2, 4, 6} as a new cell array.
Converting Between Cell Arrays and Regular Arrays
Often, you might need to convert between cell arrays and regular numeric arrays. Here's how you can convert a cell array back to a matrix:
matrix = cell2mat(myCellArray(1:3)); % Converts first three cells to a matrix
This command takes the contents of the first three cells from `myCellArray` and transforms them into a regular matrix.
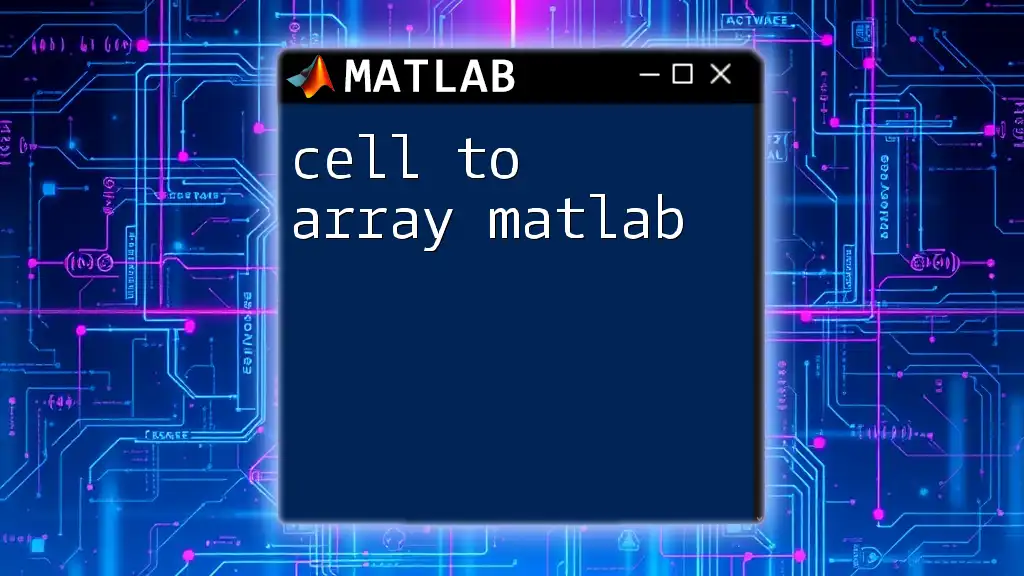
Advanced Cell Array Techniques
Nested Cell Arrays
Cell arrays can contain other cell arrays, resulting in nested cell arrays. Understanding how to create and access these structures is crucial for managing complex data.
Here’s how to create a nested cell array:
nestedCell = {1, {2, 3}, 'Text'};
To access an inner element, you would use double curly braces:
innerElement = nestedCell{2}{1}; % Accessing the inner element
This retrieves the value `2` from the nested structure.
Cell Arrays with Variable Length Data
Cell arrays are particularly beneficial for storing data of varying lengths. For instance, when storing strings of different lengths, a cell array provides a practical solution.
strings = {'short', 'a bit longer', 'this is definitely a longer string'};
Each string can be of different length without the constraints that a standard matrix would impose.
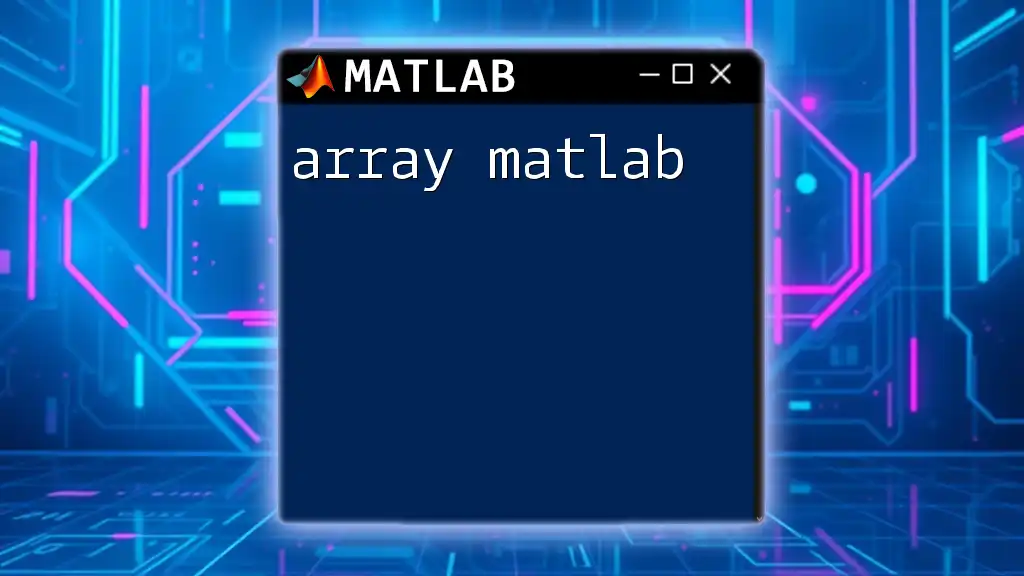
Best Practices in Using Cell Arrays
Performance Tips
To manage performance effectively, avoid unnecessary nesting within your cell arrays. While nesting can be convenient, each layer adds complexity and can lead to slower execution times when accessing or modifying data.
Readability Considerations
Maintaining code readability is essential for future updates and debugging. Keep your code clean and understandable by using meaningful variable names and comments. A well-structured approach will make it easier for others (and yourself) to revisit the code later.
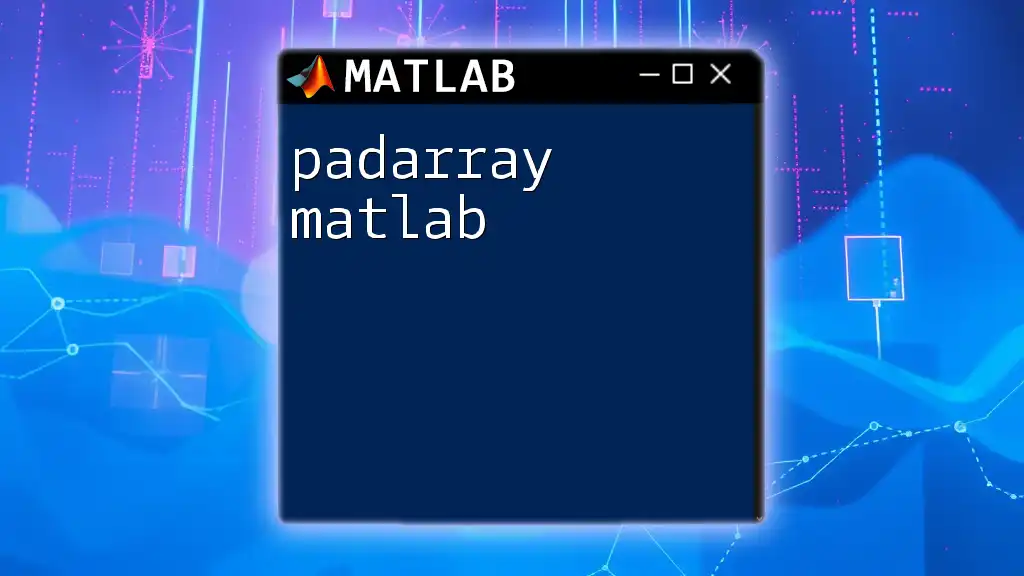
Conclusion
Cell arrays in MATLAB are powerful tools for handling mixed data types efficiently. Their flexibility allows you to work with complex datasets while maintaining a straightforward coding approach. By mastering the use of cell arrays—including their creation, indexing, and the various built-in functions—you will enhance your data management capabilities significantly.
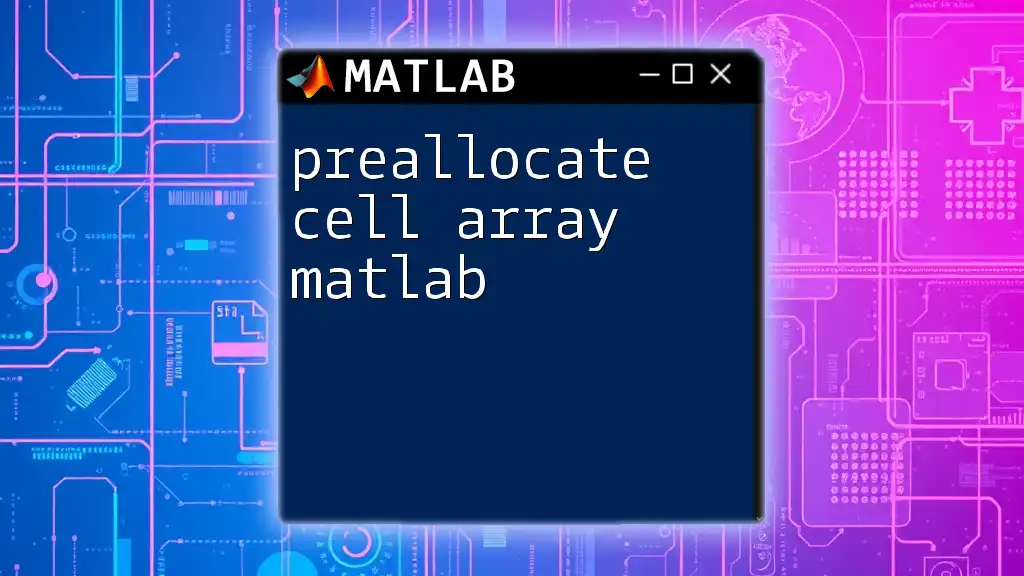
Additional Resources
For more in-depth understanding and advanced techniques, consider exploring the official MATLAB documentation. Online courses and video tutorials can provide further insights into how to master the use of cell arrays in your projects.
FAQs
If you have any common questions about cell arrays in MATLAB, FAQs help clarify their usage and provide solutions to common errors, ensuring you grasp the concept efficiently.